微信公众测试号使用:
1、首先创建微信测试号,通过二维码来添加测试号。添加完后可以通过二维码进入测试号。
然后设置域名才能重定向(没有设置域名的话会controller调用重定向接口的时候会失败):
2、获取accessToken:
请求方式: GET
请求接口: https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=APPID&secret=APPSECRET
3、创建自定义菜单:
请求方式:POST
请求接口: https://api.weixin.qq.com/cgi-bin/menu/create?access_token=ACCESS_TOKEN
json模板:
{
"button":[
{
"type":"click",
"name":"今日歌曲",
"key":"V1001_TODAY_MUSIC"
},
{
"name":"菜单",
"sub_button":[
{
"type":"view",
"name":"重定向接口",
"url":"http://你的域名/redirect"
},
//可以省略这块、这个是跳转微信小程序的一个菜单,需要关联小程序。
{
"type":"miniprogram",
"name":"wxa",
"url":"http://mp.weixin.qq.com",
"appid":"wx286b93c14bbf93aa",
"pagepath":"pages/lunar/index"
},
{
"type":"click",
"name":"赞一下我们",
"key":"V1001_GOOD"
}]
}]
}
4、服务器接口(通过这个接口来实现重定向):
@GetMapping("/redirect")
public void redirect(HttpServletResponse response) throws IOException {
String url = WeiXinUtils.REDIRECT_URL;
String appid = WeiXinUtils.APPID;
//你的回调页
String redirectUri = "http://你的域名/openId";
url = url.replace("APPID", appid);
url = url.replace("REDIRECT_URI", redirectUri);
response.sendRedirect(url);
}
5、获取code以及openId:
GetMapping("/openId")
public void openId(HttpServletRequest request) throws IllegalAccessException, NoSuchMethodException, InvocationTargetException, IOException {
//首先获得code,通过code来获得openid
String code = request.getParameter("code");
System.out.println(code);
if (code != null) {
//获取openid和access_token的连接
WechatEntity openid = WeiXinUtils.getOpenid(code);
String templateUrl = WeiXinUtils.TemplateMessage_Url.replace("ACCESS_TOKEN", WeiXinUtils.getAccessToken());
System.out.println("openId=" + openid);
System.out.println("accessToken=" + WeiXinUtils.getAccessToken());
JSONObject jsonObject = new JSONObject();
jsonObject.put("touser", "接收者的openId");
jsonObject.put("template_id", "模板id");
jsonObject.put("url", "http://www.baidu.com");
JSONObject data = new JSONObject();
JSONObject first = new JSONObject();
first.put("value", "hello");
first.put("color", "#173177");
JSONObject keyword1 = new JSONObject();
keyword1.put("value", "hello");
keyword1.put("color", "#173177");
JSONObject keyword2 = new JSONObject();
remark.put("value", "hello");
remark.put("color", "#173177");
data.put("first", first);
data.put("keyword1", keyword1);
data.put("remark", remark);
jsonObject.put("data", data);
String string = WeiXinUtils.sendPostJsonStr(templateUrl, jsonObject.toJSONString());
JSONObject result = JSON.parseObject(string);
int errcode = result.getIntValue("errcode");
if (errcode == 0) {
// 发送成功
System.out.println("发送成功");
} else {
// 发送失败
System.out.println("发送失败");
}
}
}
6、获取模板id:
{{first.DATA}}
信息:{{keyword1.DATA}}
{{remark.DATA}}
复制以上模板消息到第5步里面的template_id
7、测试成功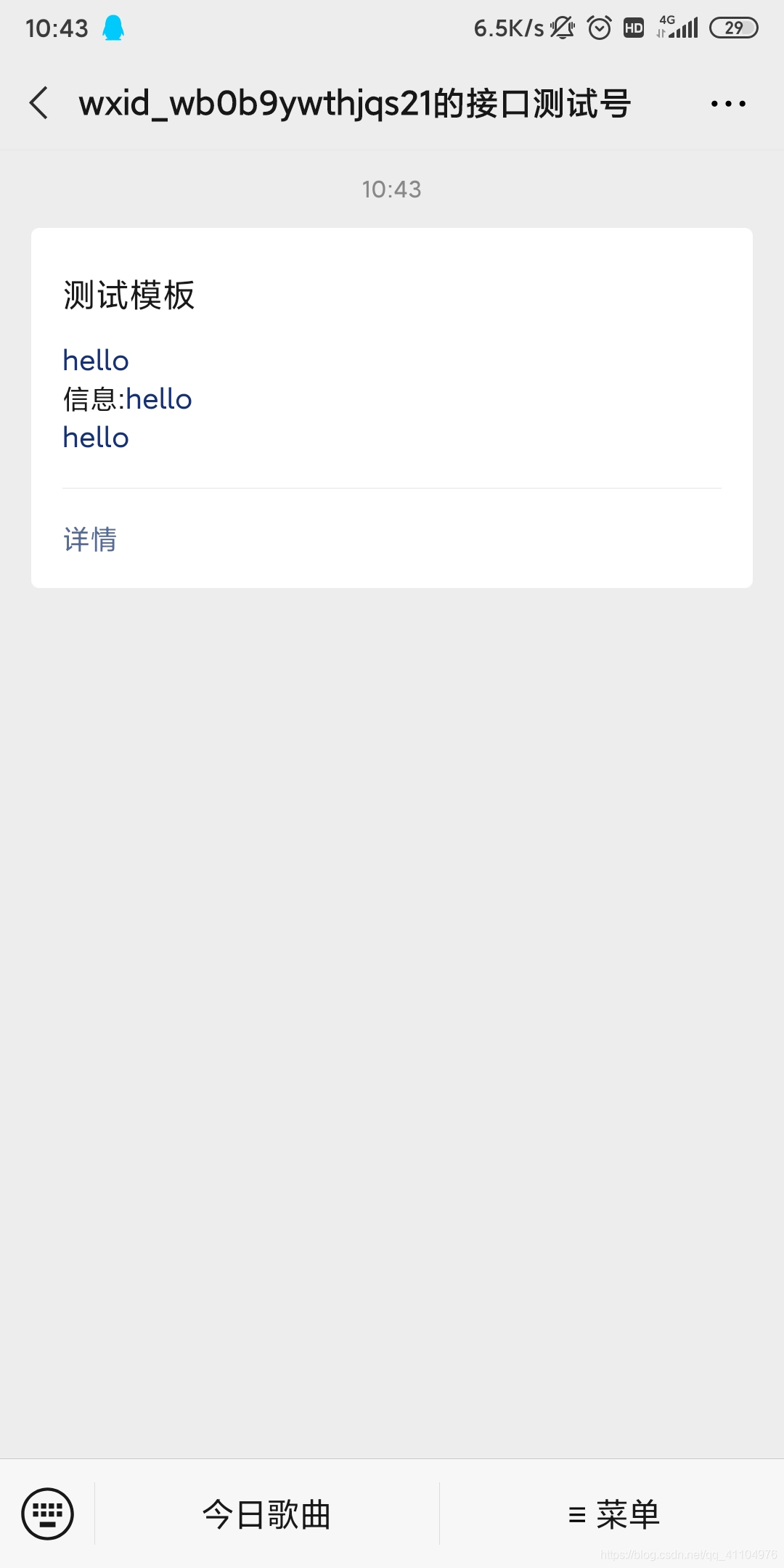
8、微信工具类(只需改下appId和appSecret)
@Slf4j
public class WeiXinUtils {
/**
* 微信公众号的APPID和Appsecret,这个是每个微信公众号都唯一的,以后配置不同的公众号配置这里即可
*/
public static final String APPID = "appId";
private static final String APPSECRET = "appSecret";
/**
* 获取access_token的URL
*/
private static final String ACCESS_TOKEN_URL = "https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=APPID&secret=APPSECRET";
private static final String OPENID_URL = "https://api.weixin.qq.com/sns/oauth2/access_token?appid=APPID&secret=APPSECRET&code=CODE&grant_type=authorization_code";
/**
* 模板消息请求路径
*/
public static String TemplateMessage_Url = "https://api.weixin.qq.com/cgi-bin/message/template/send?access_token=ACCESS_TOKEN";
public static String REDIRECT_URL = "https://open.weixin.qq.com/connect/oauth2/authorize?appid=APPID&redirect_uri=REDIRECT_URI&response_type=code&scope=snsapi_base&state=STATE#wechat_redirect";
/**
* 获取Openid
*
* @return 获取Openid
*/
public static WechatEntity getOpenid(String code) {
//首先获得code,通过code来获得openid
String url = OPENID_URL.replace("APPID", APPID).replace("APPSECRET", APPSECRET).replace("CODE", code);
String openIdRequest = getRequest(url);
JSONObject jsonObject = JSONObject.parseObject(openIdRequest);
return JSON.toJavaObject(jsonObject, WechatEntity.class);
}
public static String getAccessToken() {
String accessTokenUrl = ACCESS_TOKEN_URL;
accessTokenUrl = accessTokenUrl.replace("APPID", APPID);
accessTokenUrl = accessTokenUrl.replace("APPSECRET", APPSECRET);
String accessTokenRequest = getRequest(accessTokenUrl);
JSONObject jsonObject = JSONObject.parseObject(accessTokenRequest);
return jsonObject.getString("access_token");
}
public static String defaultEncoding = "utf-8";
/**
* 以jsonString形式发送HttpPost的Json请求,String形式返回响应结果
*
* @param url
* @param jsonString
* @return
*/
public static String sendPostJsonStr(String url, String jsonString) throws IOException {
if (jsonString == null || jsonString.isEmpty()) {
return sendPost(url);
}
String resp = "";
StringEntity entityStr = new StringEntity(jsonString,
ContentType.create("text/plain", "UTF-8"));
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(url);
httpPost.setEntity(entityStr);
CloseableHttpResponse response = null;
try {
response = httpClient.execute(httpPost);
HttpEntity entity = response.getEntity();
resp = EntityUtils.toString(entity, "UTF-8");
EntityUtils.consume(entity);
} catch (ClientProtocolException e) {
log.error(e.getMessage());
} catch (IOException e) {
log.error(e.getMessage());
} finally {
if (response != null) {
try {
response.close();
} catch (IOException e) {
log.error(e.getMessage());
}
}
}
if (resp == null || resp.equals("")) {
return "";
}
return resp;
}
/**
* 发送不带参数的HttpPost请求
*
* @param url
* @return
*/
public static String sendPost(String url) throws IOException {
// 1.获得一个httpclient对象
CloseableHttpClient httpclient = HttpClients.createDefault();
// 2.生成一个post请求
HttpPost httppost = new HttpPost(url);
CloseableHttpResponse response = null;
try {
// 3.执行get请求并返回结果
response = httpclient.execute(httppost);
} catch (IOException e) {
log.error(e.getMessage());
}
// 4.处理结果,这里将结果返回为字符串
HttpEntity entity = response.getEntity();
String result = null;
try {
result = EntityUtils.toString(entity);
} catch (ParseException | IOException e) {
log.error(e.getMessage());
}
return result;
}
/**
* 发送http get请求
*
* @param url 请求url
* @return url返回内容
*/
public static String getRequest(String url) {
return getRequest(url, null);
}
/**
* 发送http get请求
*
* @param url 请求的url
* @param params 请求的参数
* @return
*/
public static String getRequest(String url, Map<String, Object> params) {
return getRequest(url, null, params);
}
/**
* 发送http get请求
*
* @param url 请求的url
* @param headersMap 请求头
* @param params 请求的参数
* @return
*/
public static String getRequest(String url, Map<String, String> headersMap, Map<String, Object> params) {
String result = null;
CloseableHttpClient httpClient = buildHttpClient();
try {
String apiUrl = url;
if (null != params && params.size() > 0) {
StringBuffer param = new StringBuffer();
int i = 0;
for (String key : params.keySet()) {
if (i == 0) {
param.append("?");
} else {
param.append("&");
}
param.append(key).append("=").append(params.get(key));
i++;
}
apiUrl += param;
}
HttpGet httpGet = new HttpGet(apiUrl);
if (null != headersMap && headersMap.size() > 0) {
for (Map.Entry<String, String> entry : headersMap.entrySet()) {
String key = entry.getKey();
String value = entry.getValue();
httpGet.addHeader(new BasicHeader(key, value));
}
}
CloseableHttpResponse response = httpClient.execute(httpGet);
try {
HttpEntity entity = response.getEntity();
if (null != entity) {
result = EntityUtils.toString(entity, defaultEncoding);
}
} finally {
response.close();
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (ParseException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
httpClient.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return result;
}
/**
* 创建httpclient
*
* @return
*/
public static CloseableHttpClient buildHttpClient() {
try {
RegistryBuilder<ConnectionSocketFactory> builder = RegistryBuilder
.create();
ConnectionSocketFactory factory = new PlainConnectionSocketFactory();
builder.register("http", factory);
KeyStore trustStore = KeyStore.getInstance(KeyStore
.getDefaultType());
SSLContext context = SSLContexts.custom().useTLS()
.loadTrustMaterial(trustStore, new TrustStrategy() {
@Override
public boolean isTrusted(X509Certificate[] chain,
String authType) throws CertificateException {
return true;
}
}).build();
LayeredConnectionSocketFactory sslFactory = new SSLConnectionSocketFactory(
context,
SSLConnectionSocketFactory.ALLOW_ALL_HOSTNAME_VERIFIER);
builder.register("https", sslFactory);
Registry<ConnectionSocketFactory> registry = builder.build();
PoolingHttpClientConnectionManager manager = new PoolingHttpClientConnectionManager(
registry);
ConnectionConfig connConfig = ConnectionConfig.custom()
.setCharset(Charset.forName(defaultEncoding)).build();
SocketConfig socketConfig = SocketConfig.custom()
.setSoTimeout(100000).build();
manager.setDefaultConnectionConfig(connConfig);
manager.setDefaultSocketConfig(socketConfig);
return HttpClientBuilder.create().setConnectionManager(manager)
.build();
} catch (KeyStoreException e) {
e.printStackTrace();
} catch (KeyManagementException e) {
e.printStackTrace();
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
return null;
}
}