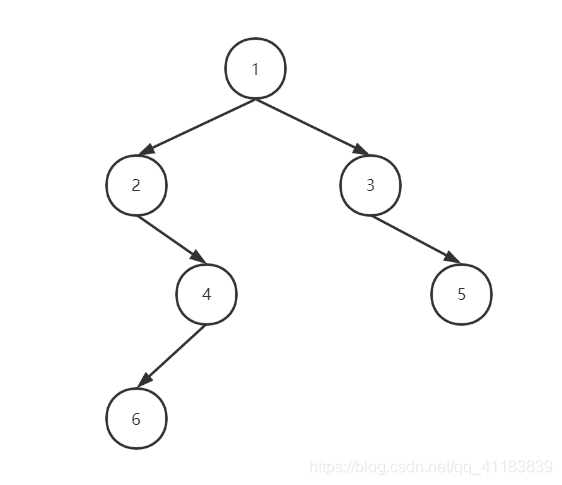
代码实现
#include "stdio.h"
#include "stdlib.h"
#include "stdbool.h"
typedef int DataType;
typedef struct Node {
DataType data;
struct Node* left;
struct Node* right;
}Node;
void create(int *arr, Node **pNode, int index, int len);
void preOrder(Node *pNode);
void inOrder(Node *pNode);
void postOrder(Node *pNode);
void inOrder2(Node *pNode);
void preOrder2(Node *pNode);
void postOrder2(Node *pNode);
void levelOrder(Node *pNode);
int main() {
DataType treeArr[] = {1, 2, 3, -1, 4, -1, 5, -1, -1, 6, -1};
int *ptr = treeArr;
Node* tree = NULL;
create(ptr, &tree, 1, 11);
printf("前序:");
preOrder(tree);
printf("NULL\n");
printf("中序:");
inOrder(tree);
printf("NULL\n");
printf("后序:");
postOrder(tree);
printf("NULL\n\n");
printf("前序:");
preOrder2(tree);
printf("NULL\n");
printf("中序:");
inOrder2(tree);
printf("NULL\n");
printf("后序:");
postOrder2(tree);
printf("NULL\n");
printf("\n层次:");
levelOrder(tree);
printf("NULL\n");
return 0;
}
void levelOrder(Node *pNode) {
Node* arr[30];
int front = 0;
int rear = 0;
arr[rear++] = pNode;
while (front != rear) {
Node *temp = arr[front++];
printf("%d --> ", temp->data);
if (temp->left != NULL)
arr[rear++] = temp->left;
if (temp->right != NULL)
arr[rear++] = temp->right;
}
}
void postOrder2(Node *pNode) {
Node* stack[30];
int top = -1;
Node* r = NULL;
Node *temp = pNode;
while (temp || top != -1) {
if (temp) {
stack[++top] = temp;
temp = temp->left;
} else {
if(stack[top]->right && r != stack[top]->right) {
Node* aa = stack[top]->right;
stack[++top] = aa;
temp = stack[top]->left;
} else {
Node *p = stack[top--];
printf("%d --> ", p->data);
r = p;
temp = NULL;
}
}
}
}
void inOrder2(Node *pNode) {
Node* stack[30];
int top = -1;
Node *temp = pNode;
while (temp || top != -1) {
if (temp) {
stack[++top] = temp;
temp = temp->left;
} else {
Node *p = stack[top--];
printf("%d --> ", p->data);
temp = p->right;
}
}
}
void preOrder2(Node *pNode) {
Node* stack[30];
int top = -1;
Node *temp = pNode;
while (temp || top != -1) {
if (temp) {
printf("%d --> ", temp->data);
stack[++top] = temp;
temp = temp->left;
} else {
Node *p = stack[top--];
temp = p->right;
}
}
}
void postOrder(Node *pNode) {
if(pNode == NULL) return;
postOrder(pNode->left);
postOrder(pNode->right);
printf("%d --> ", pNode->data);
}
void inOrder(Node *pNode) {
if(pNode == NULL) return;
inOrder(pNode->left);
printf("%d --> ", pNode->data);
inOrder(pNode->right);
}
void preOrder(Node *pNode) {
if(pNode == NULL) return;
printf("%d --> ", pNode->data);
preOrder(pNode->left);
preOrder(pNode->right);
}
void create(int *arr, Node **pNode, int index, int len) {
if (index > len || arr[index - 1] < 0) {
*pNode = NULL;
return;
}
*pNode = (Node *) malloc(sizeof(Node));
(*pNode)->data = arr[index - 1];
(*pNode)->left = NULL;
(*pNode)->right = NULL;
create(arr, &((*pNode)->left), index * 2, len);
create(arr, &((*pNode)->right), index * 2 + 1, len);
}
结果:
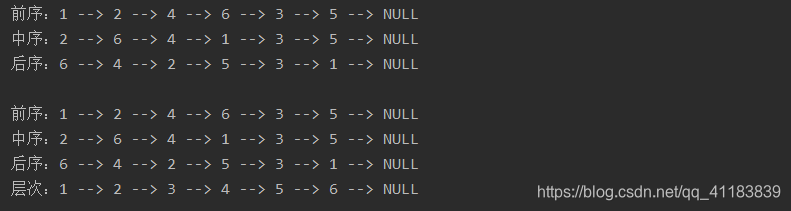