取
像
测
试
1
取像测试1
取像测试1
#include <stdio.h>
#include <process.h>
#include <conio.h>
#include "windows.h"
#include "MvCameraControl.h"
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
HWND g_hwnd = NULL;
bool g_bExit = false;
unsigned int g_nPayloadSize = 0;
void* handle = NULL;
void WaitForKeyPress(void)
{
while (!_kbhit())
{
Sleep(10);
}
_getch();
}
bool PrintDeviceInfo(MV_CC_DEVICE_INFO* pstMVDevInfo)
{
if (NULL == pstMVDevInfo)
{
printf("The Pointer of pstMVDevInfo is NULL!\n");
return false;
}
if (pstMVDevInfo->nTLayerType == MV_GIGE_DEVICE)
{
int nIp1 = ((pstMVDevInfo->SpecialInfo.stGigEInfo.nCurrentIp & 0xff000000) >> 24);
int nIp2 = ((pstMVDevInfo->SpecialInfo.stGigEInfo.nCurrentIp & 0x00ff0000) >> 16);
int nIp3 = ((pstMVDevInfo->SpecialInfo.stGigEInfo.nCurrentIp & 0x0000ff00) >> 8);
int nIp4 = (pstMVDevInfo->SpecialInfo.stGigEInfo.nCurrentIp & 0x000000ff);
printf("CurrentIp: %d.%d.%d.%d\n", nIp1, nIp2, nIp3, nIp4);
printf("UserDefinedName: %s\n\n", pstMVDevInfo->SpecialInfo.stGigEInfo.chUserDefinedName);
}
else if (pstMVDevInfo->nTLayerType == MV_USB_DEVICE)
{
printf("UserDefinedName: %s\n", pstMVDevInfo->SpecialInfo.stUsb3VInfo.chUserDefinedName);
printf("Serial Number: %s\n", pstMVDevInfo->SpecialInfo.stUsb3VInfo.chSerialNumber);
printf("Device Number: %d\n\n", pstMVDevInfo->SpecialInfo.stUsb3VInfo.nDeviceNumber);
}
else
{
printf("Not support.\n");
}
return true;
}
LRESULT CALLBACK WndProc(HWND hWnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
switch (msg)
{
case WM_DESTROY:
PostQuitMessage(0);
g_hwnd = NULL;
break;
}
return DefWindowProc(hWnd, msg, wParam, lParam);
}
static unsigned int __stdcall CreateRenderWindow(void* pUser)
{
HINSTANCE hInstance = ::GetModuleHandle(NULL);
WNDCLASSEX wc;
wc.cbSize = sizeof(wc);
wc.style = CS_HREDRAW | CS_VREDRAW;
wc.cbClsExtra = 0;
wc.cbWndExtra = 0;
wc.hInstance = hInstance;
wc.hIcon = ::LoadIcon(NULL, IDI_APPLICATION);
wc.hIconSm = ::LoadIcon(NULL, IDI_APPLICATION);
wc.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wc.hCursor = ::LoadCursor(NULL, IDC_ARROW);
wc.lpfnWndProc = WndProc;
wc.lpszMenuName = NULL;
wc.lpszClassName = "RenderWindow";
if (!RegisterClassEx(&wc))
{
return 0;
}
DWORD style = WS_OVERLAPPEDWINDOW;
DWORD styleEx = WS_EX_APPWINDOW | WS_EX_WINDOWEDGE;
RECT rect = { 0, 0, 640, 480 };
AdjustWindowRectEx(&rect, style, false, styleEx);
HWND hWnd = CreateWindowEx(styleEx, "RenderWindow", "Display", style, 0, 0,
rect.right - rect.left, rect.bottom - rect.top, NULL, NULL, hInstance, NULL);
if (hWnd == NULL)
{
return 0;
}
::UpdateWindow(hWnd);
::ShowWindow(hWnd, SW_SHOW);
g_hwnd = hWnd;
MSG msg = { 0 };
while (msg.message != WM_QUIT)
{
if (PeekMessage(&msg, 0, 0, 0, PM_REMOVE))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
}
return 0;
}
static unsigned int __stdcall WorkThread(void* pUser)
{
int nRet = MV_OK;
MV_FRAME_OUT_INFO_EX stImageInfo = { 0 };
MV_DISPLAY_FRAME_INFO stDisplayInfo = { 0 };
unsigned char* pData = (unsigned char*)malloc(sizeof(unsigned char) * (g_nPayloadSize));
if (pData == NULL)
{
return 0;
}
unsigned int nDataSize = g_nPayloadSize;
while (1)
{
nRet = MV_CC_GetOneFrameTimeout(pUser, pData, nDataSize, &stImageInfo, 1000);
if (nRet == MV_OK)
{
printf("Get One Frame: Width[%d], Height[%d], nFrameNum[%d]\n",
stImageInfo.nWidth, stImageInfo.nHeight, stImageInfo.nFrameNum);
if (g_hwnd)
{
stDisplayInfo.hWnd = g_hwnd;
stDisplayInfo.pData = pData;
stDisplayInfo.nDataLen = stImageInfo.nFrameLen;
stDisplayInfo.nWidth = stImageInfo.nWidth;
stDisplayInfo.nHeight = stImageInfo.nHeight;
stDisplayInfo.enPixelType = stImageInfo.enPixelType;
MV_CC_DisplayOneFrame(pUser, &stDisplayInfo);
}
}
else
{
printf("No data[0x%x]\n", nRet);
}
if (g_bExit)
{
break;
}
}
free(pData);
return 0;
}
int RGB2BGR(unsigned char* pRgbData, unsigned int nWidth, unsigned int nHeight)
{
if (NULL == pRgbData)
{
return MV_E_PARAMETER;
}
for (unsigned int j = 0; j < nHeight; j++)
{
for (unsigned int i = 0; i < nWidth; i++)
{
unsigned char red = pRgbData[j * (nWidth * 3) + i * 3];
pRgbData[j * (nWidth * 3) + i * 3] = pRgbData[j * (nWidth * 3) + i * 3 + 2];
pRgbData[j * (nWidth * 3) + i * 3 + 2] = red;
}
}
return MV_OK;
}
bool Convert2Mat(MV_FRAME_OUT_INFO_EX* pstImageInfo, unsigned char* pData, cv::Mat& srcImage)
{
srcImage = cv::Mat(200, 200, CV_8UC3, pData);
return true;
}
int test_grab_a_frame()
{
unsigned char* pData = (unsigned char*)malloc(sizeof(unsigned char) * (g_nPayloadSize));
if (pData == nullptr)
{
return -1;
}
unsigned int nDataSize = g_nPayloadSize;
MV_FRAME_OUT_INFO_EX stImageInfo = { 0 };
int nRet = MV_OK;
nRet = MV_CC_GetOneFrameTimeout(handle, pData, nDataSize, &stImageInfo, 1000);
if (nRet == MV_OK)
{
printf("Get One Frame: Width[%d], Height[%d], nFrameNum[%d]\n",
stImageInfo.nWidth, stImageInfo.nHeight, stImageInfo.nFrameNum);
Mat srcImage;
srcImage = Mat(1000, 1000, CV_8UC3, pData);
imwrite("srcImage000000.bmp", srcImage);
}
}
int initDevice()
{
int nRet = MV_OK;
do
{
MV_CC_DEVICE_INFO_LIST stDeviceList = { 0 };
nRet = MV_CC_EnumDevices(MV_GIGE_DEVICE | MV_USB_DEVICE, &stDeviceList);
if (MV_OK != nRet)
{
printf("Enum Devices fail! nRet [0x%x]\n", nRet);
break;
}
if (stDeviceList.nDeviceNum > 0)
{
for (unsigned int i = 0; i < stDeviceList.nDeviceNum; i++)
{
printf("Device %d Info:\n", i);
MV_CC_DEVICE_INFO* pDeviceInfo = stDeviceList.pDeviceInfo[i];
if (NULL == pDeviceInfo)
{
break;
}
PrintDeviceInfo(pDeviceInfo);
}
}
else
{
printf("Find No Devices!\n");
return -1;
}
unsigned int nIndex = 0;
nRet = MV_CC_CreateHandle(&handle, stDeviceList.pDeviceInfo[nIndex]);
if (MV_OK != nRet)
{
printf("Create Handle fail! nRet [0x%x]\n", nRet);
break;
}
nRet = MV_CC_OpenDevice(handle);
if (MV_OK != nRet)
{
printf("Open Device fail! nRet [0x%x]\n", nRet);
break;
}
if (stDeviceList.pDeviceInfo[nIndex]->nTLayerType == MV_GIGE_DEVICE)
{
int nPacketSize = MV_CC_GetOptimalPacketSize(handle);
if (nPacketSize > 0)
{
nRet = MV_CC_SetIntValue(handle, "GevSCPSPacketSize", nPacketSize);
if (nRet != MV_OK)
{
printf("Warning: Set Packet Size fail nRet [0x%x]!", nRet);
}
}
else
{
printf("Warning: Get Packet Size fail nRet [0x%x]!", nPacketSize);
}
}
nRet = MV_CC_SetEnumValue(handle, "TriggerMode", 0);
if (MV_OK != nRet)
{
printf("Set Trigger Mode fail! nRet [0x%x]\n", nRet);
break;
}
MVCC_INTVALUE stParam = { 0 };
nRet = MV_CC_GetIntValue(handle, "PayloadSize", &stParam);
if (MV_OK != nRet)
{
printf("Get PayloadSize fail! nRet [0x%x]\n", nRet);
break;
}
g_nPayloadSize = stParam.nCurValue;
nRet = MV_CC_StartGrabbing(handle);
if (MV_OK != nRet)
{
printf("Start Grabbing fail! nRet [0x%x]\n", nRet);
break;
}
} while (0);
if (nRet != MV_OK)
{
if (handle != NULL)
{
MV_CC_DestroyHandle(handle);
handle = NULL;
}
return -1;
}
printf("加载设备成功!");
return 0;
}
int main()
{
initDevice();
test_grab_a_frame();
}
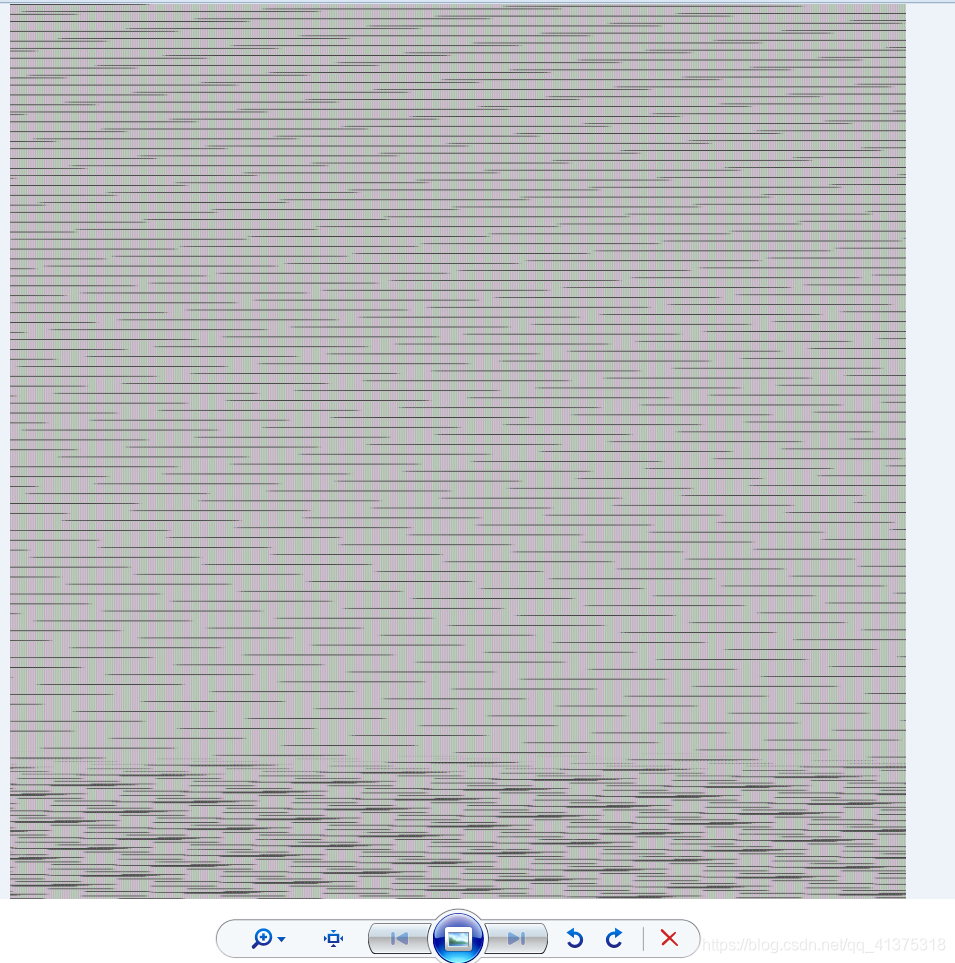
关闭相机
int closeDevice()
{
int nRet = MV_OK;
do
{
nRet = MV_CC_StopGrabbing(g_handle);
if (MV_OK != nRet)
{
printf("Stop Grabbing fail! nRet [0x%x]\n", nRet);
break;
}
nRet = MV_CC_CloseDevice(g_handle);
if (MV_OK != nRet)
{
printf("Close Device fail! nRet [0x%x]\n", nRet);
break;
}
nRet = MV_CC_DestroyHandle(g_handle);
if (MV_OK != nRet)
{
printf("Destroy Handle fail! nRet [0x%x]\n", nRet);
break;
}
} while (0);
if (nRet != MV_OK)
{
if (g_handle != NULL)
{
MV_CC_DestroyHandle(g_handle);
g_handle = NULL;
}
return -1;
}
return 0;
}