Java读取Resources目录下的文件发送至前端
JAVA读取resources目录下的templates文件,将文件以二进制流的方式发送至前端页面。
// 获取需要展示的文件位置,以二进制流发送至前端
@ApiOperation(value = "获取需要展示的政策文件", notes = "需要展示的政策文件", httpMethod = "GET")
@GetMapping("/readFile")
public void getReadFile(HttpServletResponse response, String fileName) {
try {
Resource resource = new ClassPathResource("templates/" + fileName);
File file = resource.getFile();
if (file.exists()) {
byte[] data = null;
FileInputStream input = new FileInputStream(file);
data = new byte[input.available()];
input.read(data);
// 根据文件类型,设置文件Content-Type
String fileType = fileName.substring(fileName.lastIndexOf(".")).toUpperCase();
// 设置Content-Type头
switch (fileType) {
case ".JPG":
response.setContentType("image/jpeg");
break;
case ".JPEG":
response.setContentType("image/jpeg");
break;
case ".PNG":
response.setContentType("image/png");
break;
case ".PDF":
response.setContentType("application/pdf");
break;
case ".DOC":
response.setContentType("application/msword");
break;
default:
response.setContentType("application/json");
}
response.getOutputStream().write(data);
input.close();
}
} catch (IOException e) {
e.printStackTrace();
// 抛出自定义异常
throw new CustomException("文件不存在");
}
}
接口调用
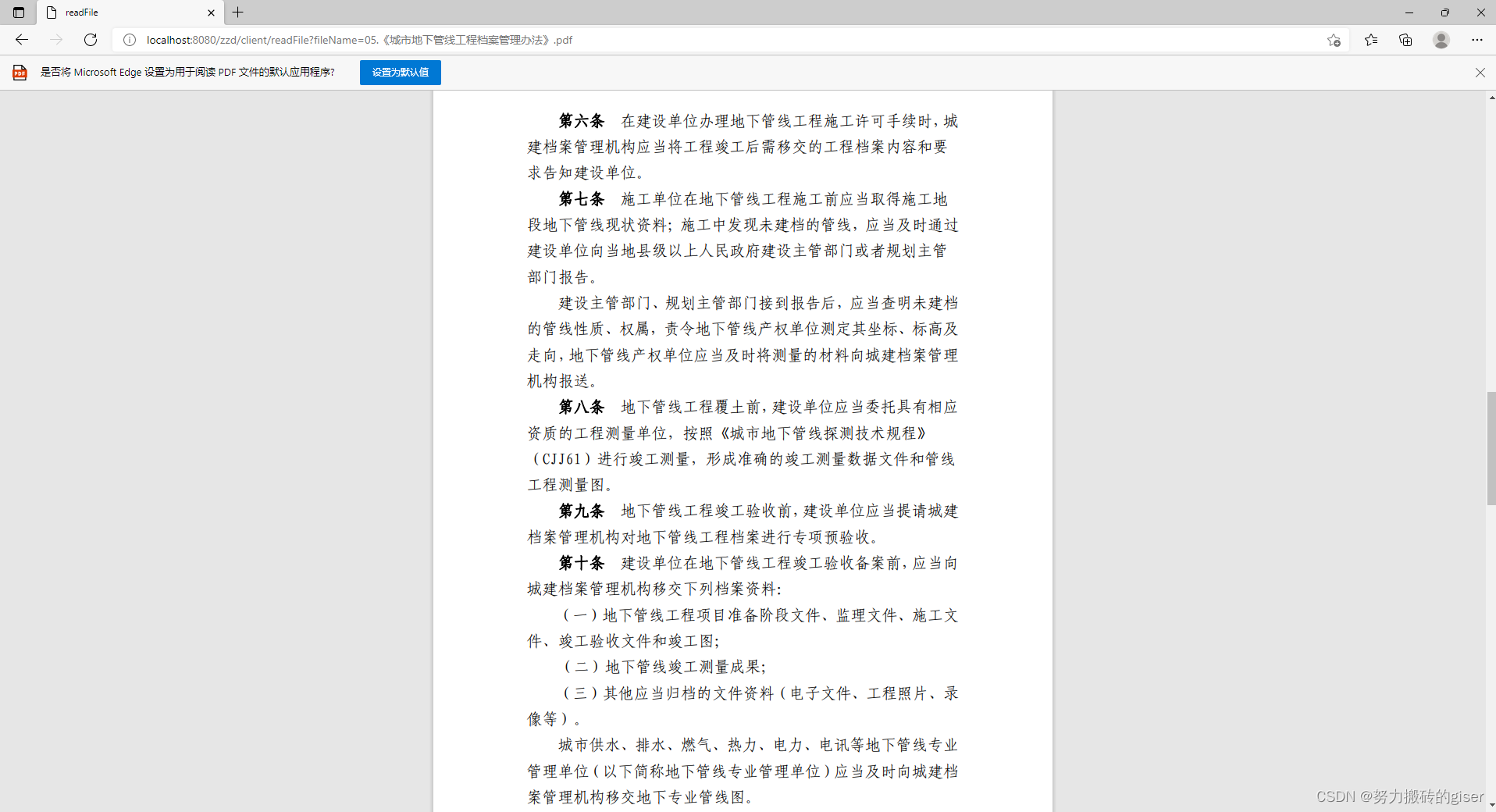