springboot实现上传文件
一、项目目录
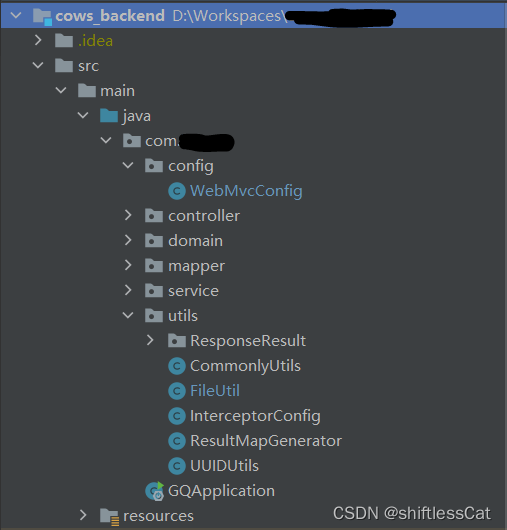
一、实现
1.WebMvcConfig.java
package com.hhu.gq.config;
import lombok.extern.slf4j.Slf4j;
import org.springframework.boot.system.ApplicationHome;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.InterceptorRegistration;
import org.springframework.web.servlet.config.annotation.InterceptorRegistry;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
import java.io.File;
@Configuration
@Slf4j
public class WebMvcConfig implements WebMvcConfigurer {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
String property = System.getProperty("user.dir");
File file3 = new File(property);
String filePath = file3.getParent()+File.separator+"files"+File.separator;
log.info(filePath);
registry.addResourceHandler("/images/**").addResourceLocations("file:"+filePath);
}
}
2.FileUtil.java
package com.hhu.gq.utils;
import lombok.extern.slf4j.Slf4j;
import org.springframework.boot.system.ApplicationHome;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Component;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
import java.util.*;
@Component
@Configuration
@Slf4j
public class FileUtil {
private static String property = System.getProperty("user.dir");
public static String fileUpLoad(MultipartFile multipartFile, String folder) {
File file3 = new File(property);
String filePath = file3.getParent() + File.separator + "files" + File.separator;
if (folder != null) {
filePath += folder + File.separator;
}
log.info("需要插入的文件夹为:{}", filePath);
String trueFileName = UUID.randomUUID().toString();
String fileName = multipartFile.getOriginalFilename();
String type = fileName.indexOf(".") != -1 ? fileName.substring(fileName.lastIndexOf(".") + 1, fileName.length())
: null;
String path = null == type ? filePath + File.separator + trueFileName
: filePath + File.separator + trueFileName + "." + type;
File file2 = new File(filePath);
if (!file2.exists()) {
file2.mkdirs();
}
try {
multipartFile.transferTo(new File(path));
Map<String, Object> resultMap = new HashMap<>();
resultMap.put("path", null == type ? trueFileName : trueFileName + "." + type);
log.info(resultMap.toString());
} catch (IllegalStateException | IOException e) {
e.printStackTrace();
}
log.info("文件夹位置:" + filePath + folder + File.separator);
return folder + File.separator + trueFileName + "." + type;
}
public static List<String> multiFileUpload(List<MultipartFile> files, String folder) {
File file3 = new File(property);
String filePath = file3.getParent() + File.separator + "files" + File.separator;
if (folder != null) {
filePath += folder + File.separator;
}
log.info("需要插入的文件夹为:{}", filePath);
MultipartFile multipartFile = null;
BufferedOutputStream stream = null;
List<String> locationOfStoredFiles = new ArrayList<>();
for (int i = 0; i < files.size(); ++i) {
multipartFile = files.get(i);
String trueFileName = UUID.randomUUID().toString();
String fileName = multipartFile.getOriginalFilename();
String type = fileName.indexOf(".") != -1 ? fileName.substring(fileName.lastIndexOf(".") + 1, fileName.length())
: null;
String path = null == type ? filePath + File.separator + trueFileName
: filePath + File.separator + trueFileName + "." + type;
File file2 = new File(filePath);
if (!file2.exists()) {
file2.mkdirs();
}
if (!multipartFile.isEmpty()) {
try {
byte[] bytes = multipartFile.getBytes();
stream = new BufferedOutputStream(new FileOutputStream(
new File(path)));
stream.write(bytes);
stream.close();
locationOfStoredFiles.add(folder + File.separator +trueFileName+'.'+type);
} catch (IllegalStateException | IOException e) {
stream = null;
log.info("第 " + i + " 个文件上传失败 ==> " + e.getMessage());
}
} else {
log.info("第 " + i + " 个文件上传失败因为文件为空");
}
}
return locationOfStoredFiles;
}
public static ResponseEntity<byte[]> downloadFile(String folder, String fileName) throws IOException {
File file3 = new File(property);
String filePath = file3.getParent() + File.separator + "files" + File.separator;
if (folder != null) {
filePath += (folder + File.separator);
}
byte[] body = null;
if (fileName != null) {
File file = new File(filePath + fileName);
if (file.exists()) {
InputStream in = new FileInputStream(file);
body = new byte[in.available()];
try {
in.read(body);
fileName = new String(fileName.getBytes("gbk"), "iso8859-1");
} catch (Exception e) {
e.printStackTrace();
} finally {
if (in != null) {
try {
in.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
HttpHeaders headers = new HttpHeaders();
headers.add("Content-Disposition", "attachment;filename=" + fileName);
HttpStatus statusCode = HttpStatus.OK;
ResponseEntity<byte[]> response = new ResponseEntity<byte[]>(body, headers, statusCode);
return response;
}
}
3.controller.java
package com.gq.controller;
import com.gq.service.DemoService;
import com.gq.utils.FileUtil;
import com.gq.utils.ResponseResult.Result.ResponseResult;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.multipart.MultipartHttpServletRequest;
import javax.servlet.http.HttpServletRequest;
@RestController
@RequestMapping("/demo")
@CrossOrigin(origins = "*")
@ResponseResult
public class DemoController {
@PostMapping(value = "add_image")
public String addVehicle(HttpServletRequest httpServletRequest){
MultipartFile image = ((MultipartHttpServletRequest) httpServletRequest).getFile("image");
String url = "";
if (image != null){
url = FileUtil.fileUpLoad(image, "fav");
}
System.out.println(url);
return url;
}
}
二、测试结果
1.前端请求
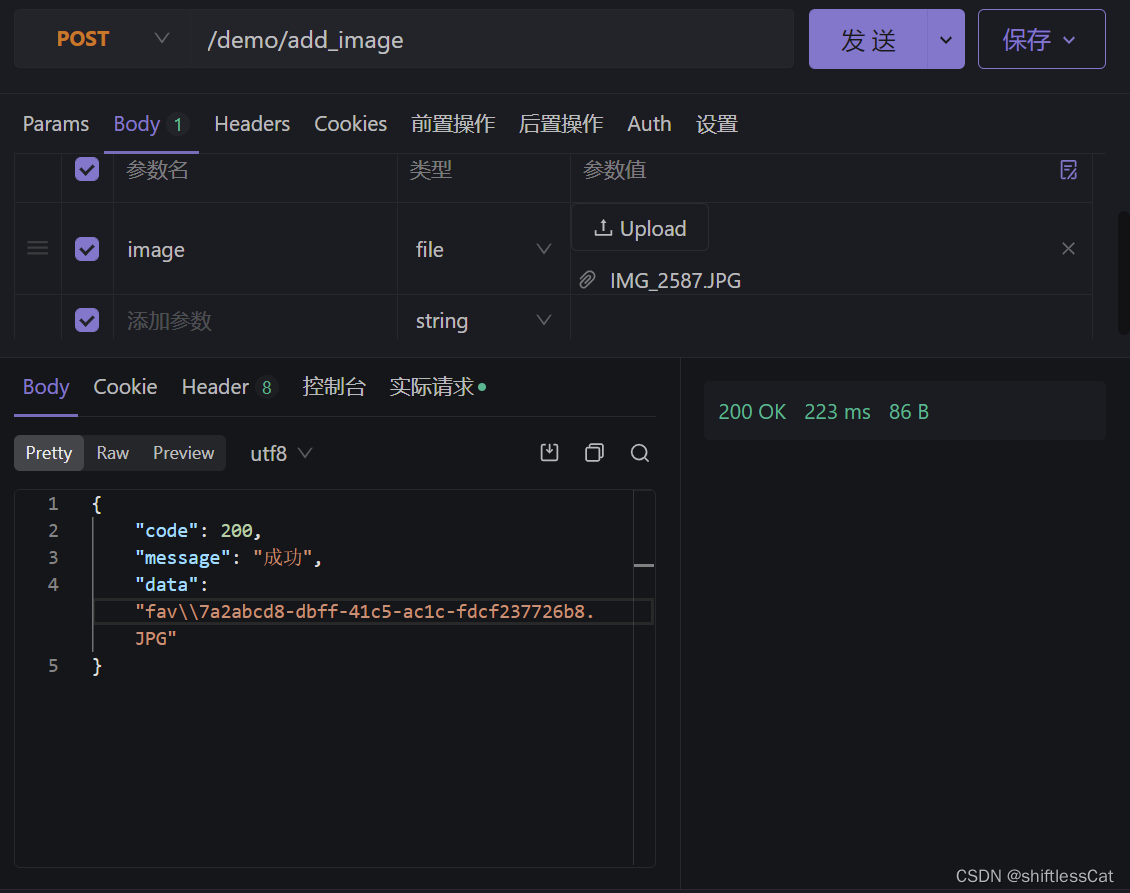
2.后台打印

3.本地磁盘
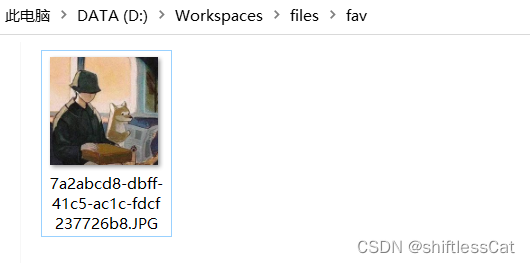
4.url访问
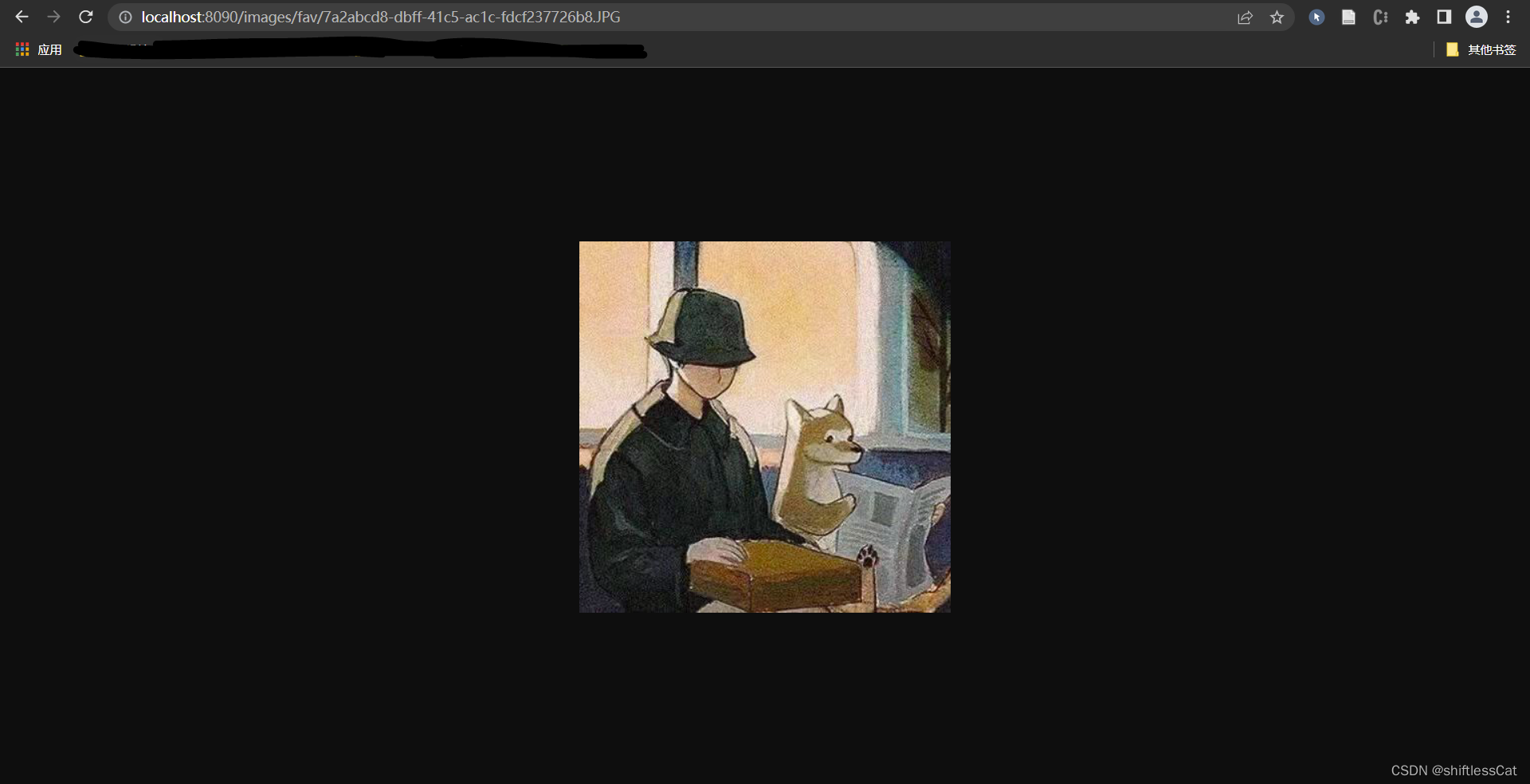
完