Python--强化--Day01
🧸如何在列表,字典,集合中根据条件筛选数据?
🎈题目1:列表解析–过滤列表中部的负数
import timeit
from random import randint
data = [randint(-10, 10) for _ in range(10)]
print(data)
# 题目1:过滤列表中部的负数
# 方法1 使用filter + lambda
r = filter(lambda x: x >= 0, data)
print(list(r))
# 方法2 列表解析
r2 = [x for x in data if x >= 0]
print(r2)
# 使用计时器 计算运行时间
print(timeit.timeit("filter(lambda x: x >= 0, data)", 'from __main__ import data'))
print(timeit.timeit("[x for x in data if x >= 0]", 'from __main__ import data'))
🎈题目2:字典解析–过滤列表学生成绩大于90的
# 过滤列表学生成绩大于90的
from random import randint
student_id_and_score = {x: randint(60, 100) for x in range(20200001, 20200021)}
print(student_id_and_score)
# 字典解析
r = {k: v for k, v in student_id_and_score.items() if v > 90}
print(r)
🎈集合解析:
# 集合解析
from random import randint
data = [randint(0, 20) for _ in range(10)]
s = set(data)
print(s)
r = {x for x in s if x % 3 == 0}
print(r)
🧸如何为元祖中的每个元素命名,提高程序的可读性?
# 方法 1 定义一系列数值常量
# 使用拆包的方法
NAME, AGE, SEX, EMAIL = range(4)
student = ('Jim', 16, 'male', 'jim123@gmail.com')
print(student[0]) # Jim 这样调用下标的方式降低了程序的可读性,0,1,2...这种
print(student[NAME]) # Jim
# 方法 2
from collections import namedtuple
# 第一个参数是子类名,第二个参数对应索引名
Student = namedtuple('Student', ['name', 'age', 'sex', 'email'])
s = Student('Jim', 16, 'male', 'jim123@gmail.com')
print(s.name) # Jim
print(isinstance(s, tuple)) # True 说明s是内置类型的一个子类
🧸如何统计序列中元素出现的频度?
from random import randint
from collections import Counter
import re
# 例1
data = [randint(0, 20) for _ in range(30)]
print(data)
# 方法 1
# 创建字典
c = dict.fromkeys(data, 0)
# print(c)
for i in data:
c[i] += 1
print(c)
# 方法 2
# 使用collections下的Counter对象
c2 = Counter(data)
print(c2)
print(c2.most_common(3)) # [(6, 4), (4, 4), (16, 4)]
# 例2
# 进行词频统计
f = open(r'D:\test.txt', encoding='utf-8').read()
# print(f)
# print(re.split('\W+', f))
c3 = Counter(re.split('\W+', f))
print(c3)
print(c3.most_common(5))
# [('the', 14), ('to', 9), ('in', 9), ('of', 8), ('used', 6)]
🧸如何根据字典中值得大小,对字典中的项排序?
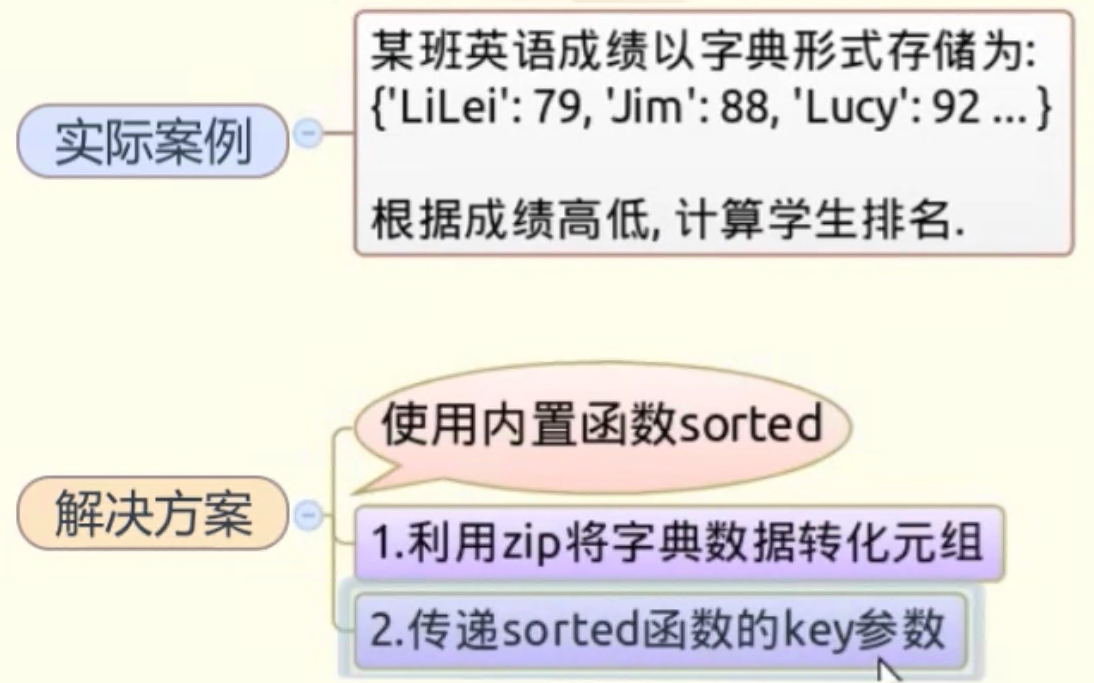
from random import randint
# 创建随机成绩表
stu_score = {x: randint(60, 100) for x in 'xyzabc'}
print(stu_score)
print(sorted(stu_score))
# 获得键
print(stu_score.keys())
# 获得值
print(stu_score.values())
# 按照成绩高低由小到达排序
# 1.使用zip函数
z = zip(stu_score.values(), stu_score.keys())
z1 = list(z)
print(sorted(z1))
# 2.sorted中的key
print(stu_score.items())
z2 = sorted(stu_score.items(), key=lambda x: x[1])
print(z2)
🧸如何快速找到多个字典的公共健(key)?
from functools import reduce
from random import randint, sample
# 假设球员abcdefg
# s使用sample取样
print(sample('abcdefg', 3))
s1 = {i: randint(1, 4) for i in sample('abcdefg', randint(3, 6))}
s2 = {i: randint(1, 4) for i in sample('abcdefg', randint(3, 6))}
s3 = {i: randint(1, 4) for i in sample('abcdefg', randint(3, 6))}
print(s1)
print(s2)
print(s3)
# 1.使用for循环
r = []
for k in s1:
if k in s2 and k in s3:
r.append(k)
print(r)
# 2.使用集合操作
r2 = s1.keys() & s2.keys() & s3.keys()
print(list(r2))
# 使用map和reduce
rm = map(dict.keys, [s1, s2, s3])
print(list(rm))
r3 = reduce(lambda x, y: x & y, map(dict.keys, [s1, s2, s3]))
print(r3)
🧸如何让字典保持有序?
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-5jYg3zcw-1653276902215)(https://raw.githubusercontent.com/kurry0123/Py-learn-imgs/main/img/202205231134740.png)]
# 🧸如何让字典保持有序?
# 1. 引入
d = {}
d['Jim'] = (1, 35)
d['Leo'] = (2, 45)
d['Bob'] = (3, 50)
for i in d: print(i)
# 使用OrderedDict
from collections import OrderedDict
d2 = OrderedDict()
d2['Jim'] = (1, 35)
d2['Leo'] = (2, 45)
d2['Bob'] = (3, 50)
for i in d2: print(i)
# 模拟系统
from time import time
from random import randint
from collections import OrderedDict
players = list('ABCDEFGH')
stat_time = time()
l = len(players)
d = OrderedDict()
for i in range(l):
print('请输入,完成提交:')
input() # 相当于阻断时间正常走,没输入一次将有一人交卷,出栈
# 出栈
p = players.pop(randint(0, l-1 - i))
end_time = time()
sum_time = end_time - stat_time
print(i + 1, p, sum_time)
d[p] = (i+1, sum_time)
print('='*20)
for k in d:
print(k, d[k])
🧸如何实现用户的历史记录功能:
🎈pickle的使用:
# pickle
import pickle
from collections import deque
q = deque([[20, 10, 50, 60, 70]])
print(q)
# 存储
pickle.dump(q, open('history_demo', 'wb'))
q2 = pickle.load(open('history_demo', 'rb'))
print(q2)
数字游戏实例:
from random import randint
from collections import deque
import pickle
N = randint(0, 100)
history = deque([], 5)
def guss(k):
if k == N:
print('Right')
return True
if k < N:
print('%s is less-than N' %k)
else:
print('%s is greater-than N'%k)
return False
while True:
line = input('Please input a number:')
if line.isdigit():
k = int(line)
history.append(k)
pickle.dump(history, open('history', 'wb'))
if guss(k):
break
elif line == 'history' or 'h?':
print(list(history))
print(pickle.load(open('history', 'rb')))