1.学生信息展示
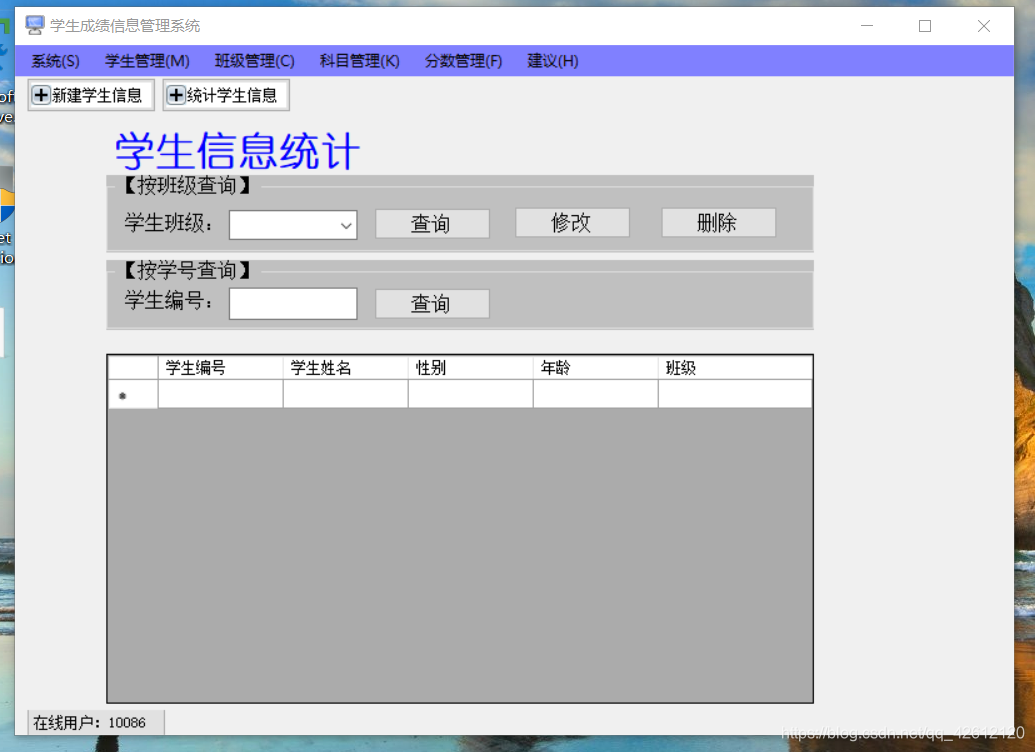
public partial class ListStudentForm : Form
{
private ClassService classService = new ClassService();
private StudentService studentService = new StudentService();
public ListStudentForm()
{
InitializeComponent();
//下拉班级
this.comBClass.DataSource = classService.GetClassList();
this.comBClass.DisplayMember = "ClassName";
this.comBClass.ValueMember = "ClassId";
this.comBClass.SelectedIndex = -1;
}
private void groupBox1_Enter(object sender, EventArgs e)
{
}
private void comBClass_SelectedIndexChanged(object sender, EventArgs e)
{
}
private void ListStudentForm_Load(object sender, EventArgs e)
{
}
private void groupBox2_Enter(object sender, EventArgs e)
{
}
//按班级查询
private void queryStudentList_Click(object sender, EventArgs e)
{
if (this.comBClass.SelectedIndex == -1)
{
MessageBox.Show("请选择班级!", "信息提示");
this.comBClass.Focus();
return;
}
List<StudentVo> list = studentService.GetStudentListByClassId(this.comBClass.SelectedValue.ToString());
this.dgvStudentClass.AutoGenerateColumns = false;
this.dgvStudentClass.DataSource = list;
}
//按学号查询一个学生
private void queryStudent_Click(object sender, EventArgs e)
{
if (this.txtStuId.Text.Trim().Length == 0)
{
MessageBox.Show("请输入学生编号!", "信息提示");
this.txtStuId.Focus();
return;
}
//根据Id查询
StudentVo studentVo = studentService.QueryStudentByStudentId(this.txtStuId.Text.Trim());
if (studentVo.StudentName == null)
{
MessageBox.Show("您输入的学号不正确!", "信息提示");
this.txtStuId.Focus();
this.txtStuId.SelectAll();
}
else
{
//打开学生信息窗体
StudentInfoForm studentInfoForm = new StudentInfoForm(studentVo);
studentInfoForm.Show();
}
}
//修改学生信息
private void btnEditStu_Click(object sender, EventArgs e)
{
//判断用户是否选中
if (this.dgvStudentClass.RowCount == 0 || this.dgvStudentClass.CurrentRow == null)
{
MessageBox.Show("没有要修改的信息!", "信息提示");
return;
}
//获取要修改的StudentId
string studentId = this.dgvStudentClass.CurrentRow.Cells["StudentId"].Value.ToString();
StudentVo studentVo = studentService.QueryStudentByStudentId(studentId);
//显示待修改学生的信息----打开编辑窗口
EditStudentForm editStudentForm = new EditStudentForm(studentVo);
DialogResult result = editStudentForm.ShowDialog();
if (result == DialogResult.OK)
{
queryStudentList_Click(null, null);
}
}
//删除学生信息
private void btnDelStu_Click(object sender, EventArgs e)
{
if (this.dgvStudentClass.RowCount == 0 || this.dgvStudentClass.CurrentRow == null)
{
MessageBox.Show("没有要删除的学生信息", "信息提示");
}
//删除确认
DialogResult result = MessageBox.Show("确认要删除吗?", "信息提示", MessageBoxButtons.OKCancel, MessageBoxIcon.Question);
if (result == DialogResult.Cancel)
{
return;
}
//获取要删除的StudentId
string studentId = this.dgvStudentClass.CurrentRow.Cells["StudentId"].Value.ToString();
//根据学号删除
try
{
if (studentService.DeleteStudent(studentId) == 1)
{
MessageBox.Show("删除成功!","信息提示");
queryStudentList_Click(null, null);
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message,"删除信息");
}
}
}
班级查询:
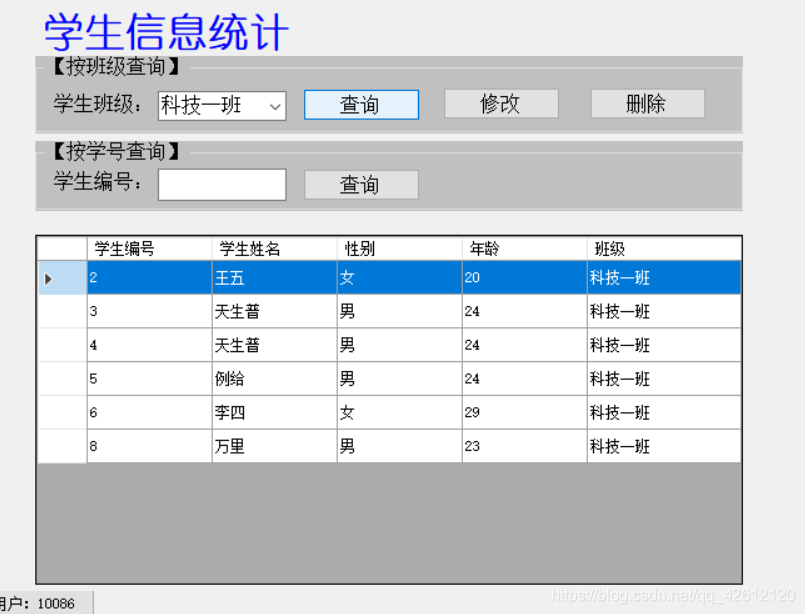
public List<StuClass> GetClassList()
{
List<StuClass> list = new List<StuClass>();
string sql = "select ClassId,ClassName from Class";
SqlDataReader dataReader = SQLHelper.GetReader(sql);
while (dataReader.Read())
{
StuClass stuClass = new StuClass();
stuClass.ClassId = Convert.ToInt32(dataReader["ClassId"]);
stuClass.ClassName = dataReader["ClassName"].ToString();
list.Add(stuClass);
}
dataReader.Close();
return list;
}
学号查询:
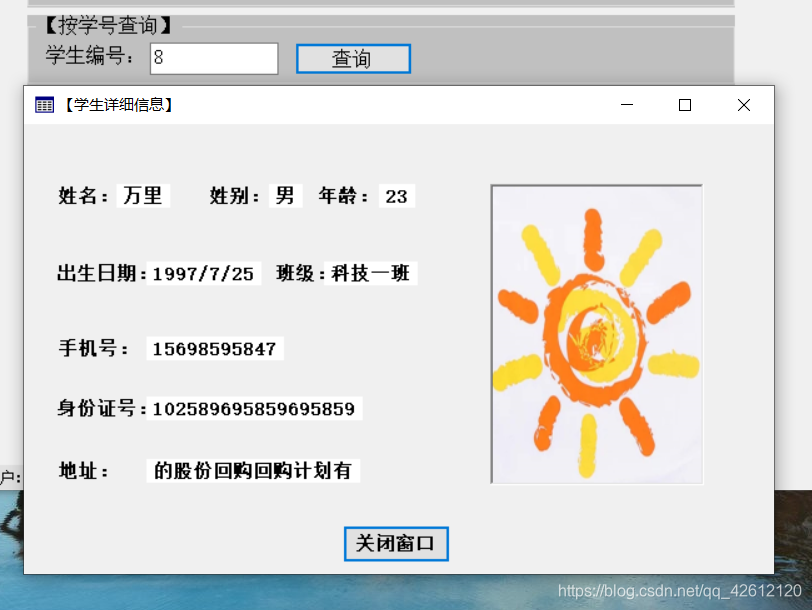
public StudentInfoForm()
{
InitializeComponent();
}
public StudentInfoForm(StudentVo studentVo)
{
InitializeComponent();
int x = (System.Windows.Forms.SystemInformation.WorkingArea.Width - this.Size.Width) / 2;
int y = (System.Windows.Forms.SystemInformation.WorkingArea.Height - this.Size.Height) / 2;
this.StartPosition = FormStartPosition.Manual; //窗体的位置由Location属性决定
this.Location = (Point)new Size(x, y);
this.laStudentName.Text = studentVo.StudentName.ToString();
this.laGender.Text = studentVo.Gender.ToString();
this.laAge.Text = studentVo.Age.ToString();
this.laBirthday.Text = studentVo.Birthday.ToShortDateString();
this.laClassName.Text = studentVo.ClassName.ToString();
this.laPhone.Text = studentVo.PhoneNumber.ToString();
this.laStundetNo.Text = studentVo.StudentIdNo.ToString();
this.laAddress.Text = studentVo.StudentAddress.ToString();
this.laStuImage.Image =studentVo.StuImage.Length ==0?Image.FromFile("default.png") : (Image) new SerializeObjectToString().DeserializeObject(studentVo.StuImage.ToString());
}
private void label10_Click(object sender, EventArgs e)
{
}
private void StudentInfoForm_Load(object sender, EventArgs e)
{
}
//关闭窗口
private void button1_Click(object sender, EventArgs e)
{
this.Close();
}
2.学生信息添加
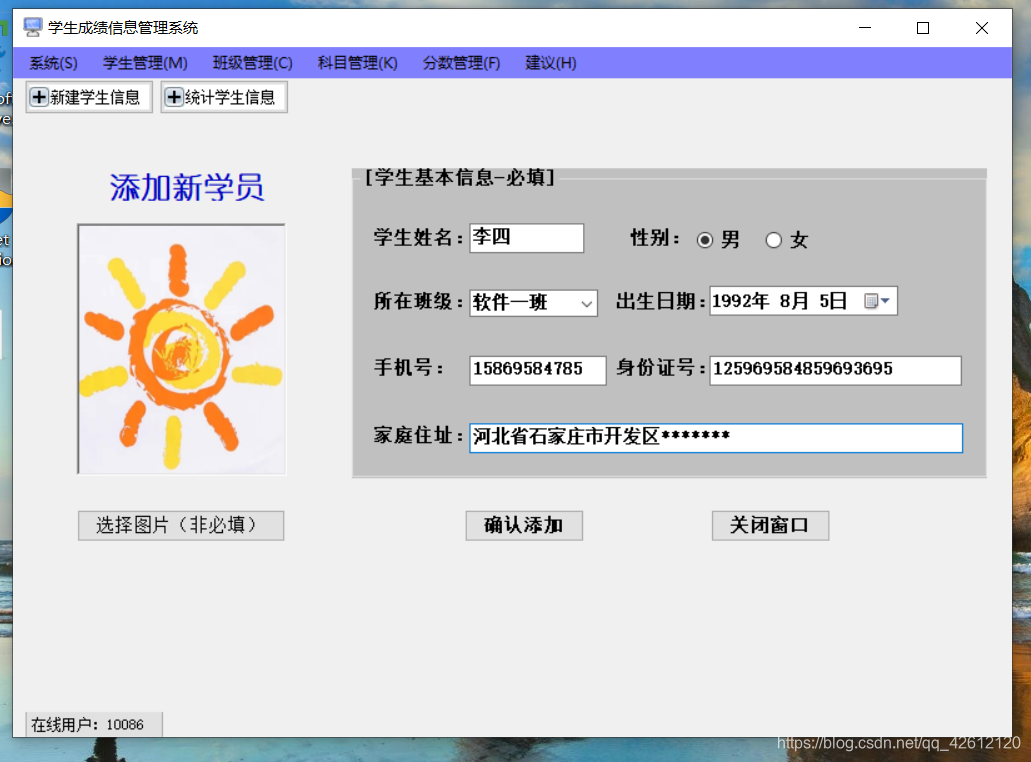
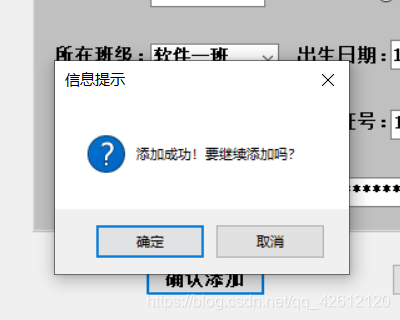
private ClassService classService = new ClassService();
private StudentService studentService = new StudentService();
public AddStudentForm()
{
InitializeComponent();
//班级下拉框
this.comStuClass.DataSource = classService.GetClassList();
this.comStuClass.DisplayMember = "ClassName";
this.comStuClass.ValueMember = "ClassId";
this.comStuClass.SelectedIndex = -1;
}
private void AddStudentForm_Load(object sender, EventArgs e)
{
}
//选择图片
private void btnPicture_Click(object sender, EventArgs e)
{
OpenFileDialog fileDialog = new OpenFileDialog();
DialogResult result = fileDialog.ShowDialog();
if (result == DialogResult.OK)
{
this.stuPicture.Image = Image.FromFile(fileDialog.FileName);
}
}
//添加学生
private void btnAddStu_Click(object sender, EventArgs e)
{
//数据验证
if (this.txtStuName.Text.Trim().Length == 0)
{
MessageBox.Show("请填写学生姓名!", "输入提示");
this.txtStuName.Focus();
return;
}
if (!this.manStu.Checked && !womenStu.Checked)
{
MessageBox.Show("请选择性别!", "输入提示");
return;
}
if ((DateTime.Now.Year - Convert.ToDateTime(this.stuDate.Text).Year) < 18)
{
MessageBox.Show("学生年龄不能小于18岁,请修改!", "输入提示");
this.stuDate.Focus();
return;
}
if (this.comStuClass.SelectedIndex == -1)
{
MessageBox.Show("请选择班级!", "输入提示");
return;
}
if (this.txtStuPhone.Text.Trim().Length == 0)
{
MessageBox.Show("请填写学生手机号!", "输入提示");
this.txtStuPhone.Focus();
return;
}
//判断手机号格式
if (!DataValidate.IsPhone(this.txtStuPhone.Text.Trim()))
{
MessageBox.Show("手机号格式有误!", "输入提示");
this.txtStuPhone.Focus();
this.txtStuPhone.SelectAll();
return;
}
if (this.txtStuCId.Text.Trim().Length == 0)
{
MessageBox.Show("请填写学生身份证号!", "输入提示");
this.txtStuCId.Focus();
return;
}
//验证身份证号格式
if (!DataValidate.IsIdentityCard(this.txtStuCId.Text.Trim()))
{
MessageBox.Show("身份证格式有误!", "输入提示");
this.txtStuCId.Focus();
this.txtStuCId.SelectAll();
return;
}
if (this.stuAddress.Text.Trim().Length == 0)
{
MessageBox.Show("请填写学生地址!", "输入提示");
this.stuAddress.Focus();
return;
}
//封装对象
Student student = new Student();
student.StudentName = this.txtStuName.Text.Trim();
student.Gender = this.manStu.Checked ? "男" : "女";
student.Birthday = Convert.ToDateTime(this.stuDate.Text.Trim());
student.StudentIdNo = this.txtStuCId.Text.Trim();
student.StuImage = this.stuPicture.Image == null ? "" : new SerializeObjectToString().SerializeObject(this.stuPicture.Image);
student.PhoneNumber = this.txtStuPhone.Text.Trim();
student.StudentAddress = this.stuAddress.Text.Trim();
student.ClassId = Convert.ToInt32(this.comStuClass.SelectedValue);
student.Age = DateTime.Now.Year - Convert.ToDateTime(this.stuDate.Text).Year;
//提交学生对象
try
{
int result = studentService.AddStudent(student);
if (result == 1)
{
DialogResult dialogResult = MessageBox.Show("添加成功!要继续添加吗?", "信息提示", MessageBoxButtons.OKCancel, MessageBoxIcon.Question);
if (dialogResult == DialogResult.OK)
{
//清空文本框
foreach (Control item in this.stuGroupBox.Controls)
{
if (item is TextBox)
{
item.Text = "";
}
else if (item is RadioButton)
{
((RadioButton)item).Checked = false;
}
else if (item is ComboBox)
{
((ComboBox)item).SelectedIndex = -1;
}
else if (item is DateTimePicker)
{
((DateTimePicker)item).Value = DateTime.Now;
}
}
this.stuPicture.Image = null;//图片清空
this.txtStuName.Focus();//光标定位到名字
}
if (dialogResult == DialogResult.Cancel)
{
btuCloseAddStu_Click(null,null);
}
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, "信息提示", MessageBoxButtons.OK, MessageBoxIcon.Error);
throw;
}
//判断结果是否成功
}
//关闭
private void btuCloseAddStu_Click(object sender, EventArgs e)
{
this.Close();
}
3.学生信息修改
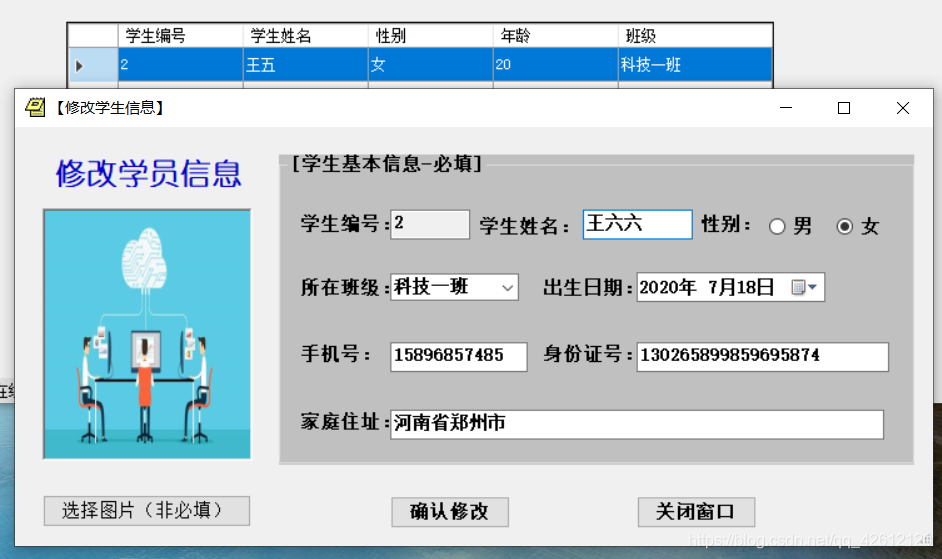
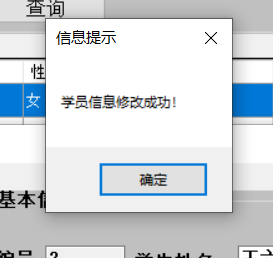
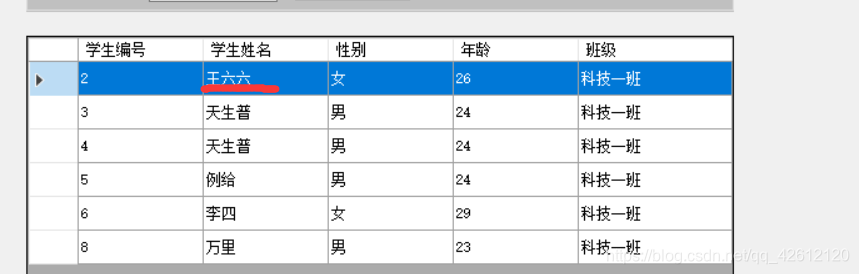
private ClassService classService = new ClassService();
private StudentService studentService = new StudentService();
public EditStudentForm()
{
InitializeComponent();
}
public EditStudentForm(StudentVo studentVo)
{
InitializeComponent();
//班级下拉框
this.comStuClass.DataSource = classService.GetClassList();
this.comStuClass.DisplayMember = "ClassName";
this.comStuClass.ValueMember = "ClassId";
this.comStuClass.Text = studentVo.ClassName;
this.txtStuId.Text = studentVo.StudentId.ToString();
this.txtStuCId.Text = studentVo.StudentId.ToString();
this.txtStuName.Text = studentVo.StudentName.ToString();
this.stuDate.Text = studentVo.Birthday.ToShortDateString();
this.txtStuPhone.Text = studentVo.PhoneNumber.ToString();
this.txtStuCId.Text = studentVo.StudentIdNo.ToString();
this.stuAddress.Text = studentVo.StudentAddress.ToString();
if (studentVo.Gender == "男")
{
this.manStu.Checked = true;
}
else
{
this.womenStu.Checked = true;
}
this.stuPicture.Image = studentVo.StuImage.Length == 0 ? Image.FromFile("default.png") : (Image)new SerializeObjectToString().DeserializeObject(studentVo.StuImage.ToString());
}
private void stuPicture_Click(object sender, EventArgs e)
{
}
private void EditStudentForm_Load(object sender, EventArgs e)
{
int x = (System.Windows.Forms.SystemInformation.WorkingArea.Width - this.Size.Width) / 2;
int y = (System.Windows.Forms.SystemInformation.WorkingArea.Height - this.Size.Height) / 2;
this.StartPosition = FormStartPosition.Manual; //窗体的位置由Location属性决定
this.Location = (Point)new Size(x, y);
}
private void btnPicture_Click(object sender, EventArgs e)
{
OpenFileDialog fileDialog = new OpenFileDialog();
DialogResult result = fileDialog.ShowDialog();
if (result == DialogResult.OK)
{
this.stuPicture.Image = Image.FromFile(fileDialog.FileName);
}
}
//提交修改信息
private void btnEditStu_Click(object sender, EventArgs e)
{
//数据验证
if (this.txtStuName.Text.Trim().Length == 0)
{
MessageBox.Show("请填写学生姓名!", "输入提示");
this.txtStuName.Focus();
return;
}
if (!this.manStu.Checked && !womenStu.Checked)
{
MessageBox.Show("请选择性别!", "输入提示");
return;
}
if ((DateTime.Now.Year - Convert.ToDateTime(this.stuDate.Text).Year) < 18)
{
MessageBox.Show("学生年龄不能小于18岁,请修改!", "输入提示");
this.stuDate.Focus();
return;
}
if (this.comStuClass.SelectedIndex == -1)
{
MessageBox.Show("请选择班级!", "输入提示");
return;
}
if (this.txtStuPhone.Text.Trim().Length == 0)
{
MessageBox.Show("请填写学生手机号!", "输入提示");
this.txtStuPhone.Focus();
return;
}
//判断手机号格式
if (!DataValidate.IsPhone(this.txtStuPhone.Text.Trim()))
{
MessageBox.Show("手机号格式有误!", "输入提示");
this.txtStuPhone.Focus();
this.txtStuPhone.SelectAll();
return;
}
if (this.txtStuCId.Text.Trim().Length == 0)
{
MessageBox.Show("请填写学生身份证号!", "输入提示");
this.txtStuCId.Focus();
return;
}
//验证身份证号格式
if (!DataValidate.IsIdentityCard(this.txtStuCId.Text.Trim()))
{
MessageBox.Show("身份证格式有误!", "输入提示");
this.txtStuCId.Focus();
this.txtStuCId.SelectAll();
return;
}
if (this.stuAddress.Text.Trim().Length == 0)
{
MessageBox.Show("请填写学生地址!", "输入提示");
this.stuAddress.Focus();
return;
}
//封装对象
Student student = new Student();
student.StudentId = Convert.ToInt32(this.txtStuId.Text.Trim().ToString());
student.StudentName = this.txtStuName.Text.Trim();
student.Gender = this.manStu.Checked ? "男" : "女";
student.Birthday = Convert.ToDateTime(this.stuDate.Text.Trim());
student.StudentIdNo = this.txtStuCId.Text.Trim();
student.StuImage = this.stuPicture.Image == null ? "" : new SerializeObjectToString().SerializeObject(this.stuPicture.Image);
student.PhoneNumber = this.txtStuPhone.Text.Trim();
student.StudentAddress = this.stuAddress.Text.Trim();
student.ClassId = Convert.ToInt32(this.comStuClass.SelectedValue);
student.Age = DateTime.Now.Year - Convert.ToDateTime(this.stuDate.Text).Year;
//进行判断
try
{
if (studentService.updateStudent(student) ==1)
{
MessageBox.Show("学员信息修改成功!","信息提示");
this.DialogResult = DialogResult.OK;
this.Close();
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
private void btuCloseEditStu_Click(object sender, EventArgs e)
{
this.Close();
}
4.学生信息删除
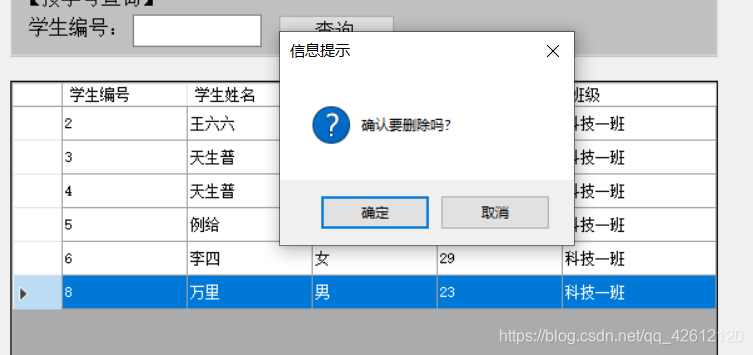
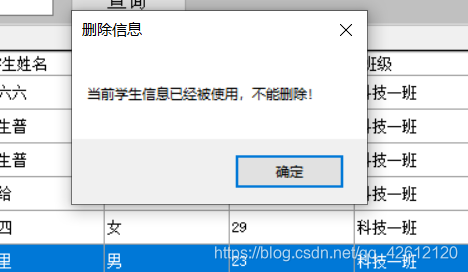
/// <summary>
/// 删除学生
/// </summary>
/// <param name="studentId"></param>
/// <returns></returns>
public int DeleteStudent(string studentId)
{
string sql = "delete from Student where StudentId=" + studentId;
try
{
return SQLHelper.Update(sql);
}
catch (SqlException ex)
{
if (ex.Number == 547)
{
throw new Exception("当前学生信息已经被使用,不能删除!");
}
else
{
throw new Exception("删除信息错误:" + ex.Message);
}
}
catch (Exception ex)
{
throw new Exception("删除学生信息错误!" + ex.Message);
}
}