1.成绩录入
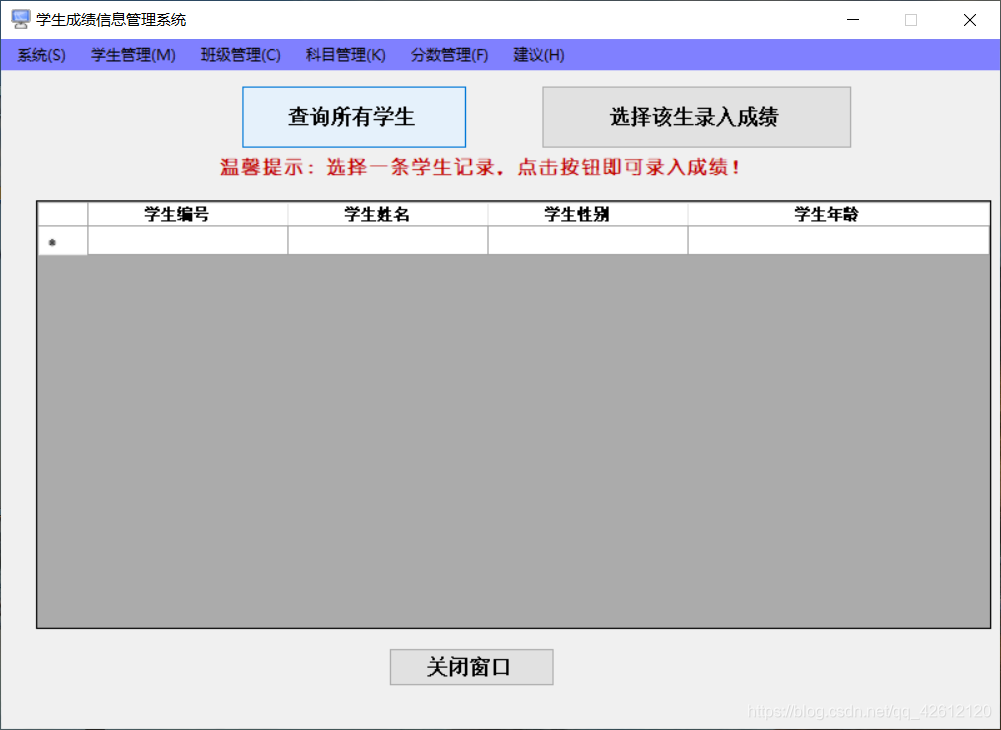
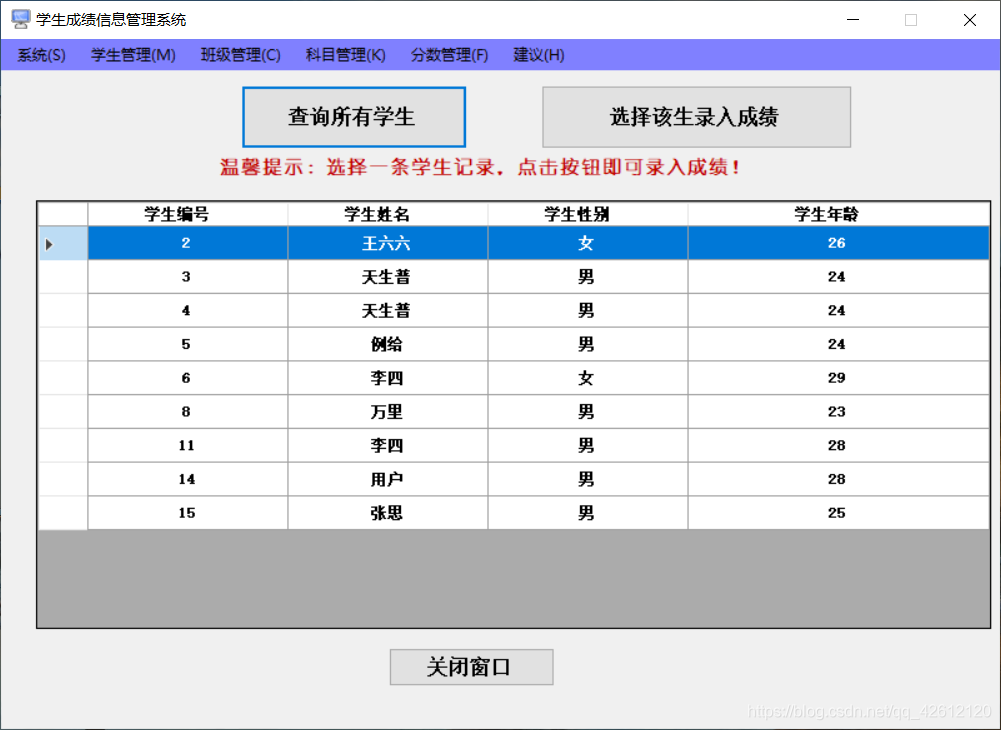
private StudentService studentService = new StudentService();
private ScoreService scoreService = new ScoreService();
private CourseService courseService = new CourseService();
public AddScoreForm()
{
InitializeComponent();
}
//关闭窗口
private void button2_Click(object sender, EventArgs e)
{
this.Close();
}
//查询所有学生信息
private void btnListStudent_Click(object sender, EventArgs e)
{
this.dgvStuScoreList.AutoGenerateColumns = false;
// this.dgvStuScoreList.DataSource = studentService.GetStudentNoScoreList();
this.dgvStuScoreList.DataSource = studentService.GetStudentList();
}
//选择该生录入成绩
private void btnChoiceStu_Click(object sender, EventArgs e)
{
//判断用户是否选中
if (this.dgvStuScoreList.RowCount == 0 || this.dgvStuScoreList.CurrentRow == null)
{
MessageBox.Show("没选中学生信息!", "信息提示");
return;
}
//获取要修改的StudentId
string studentId = this.dgvStuScoreList.CurrentRow.Cells["StudentId"].Value.ToString();
//学生成绩记录数与科目数是否相等???
if (scoreService.QueryScoreExist(studentId) ==courseService.QueryCourseNum())
{
MessageBox.Show("该生成绩已经录入完毕!!", "信息提示");
return;
}
Student student = studentService.QueryStuByStudentId(studentId);
// 显示待录入学生的成绩科目----打开编辑窗口
AddStuScoreForm addStuScoreForm = new AddStuScoreForm(student);
DialogResult result = addStuScoreForm.ShowDialog();
if (result == DialogResult.OK)
{
btnListStudent_Click(null, null);
}
}
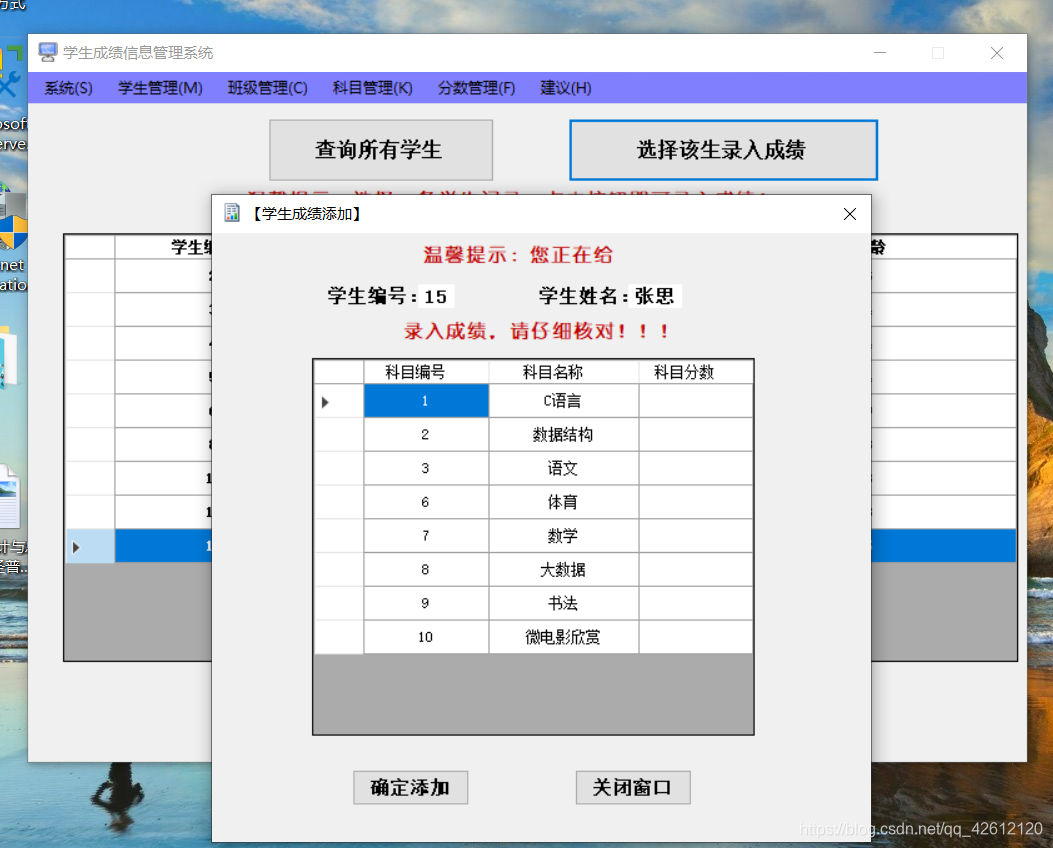
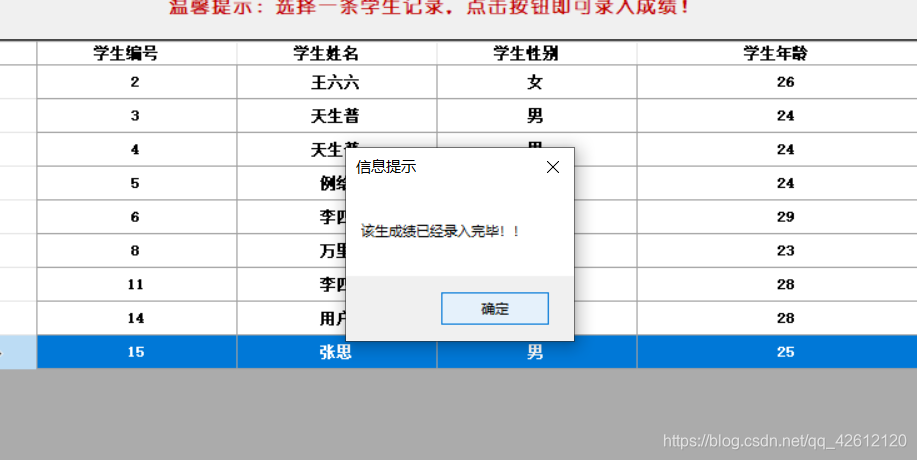
private CourseService courseService = new CourseService();
private ScoreService scoreService = new ScoreService();
public AddStuScoreForm()
{
InitializeComponent();
}
public AddStuScoreForm(Student student)
{
InitializeComponent();
int x = (System.Windows.Forms.SystemInformation.WorkingArea.Width - this.Size.Width) / 2;
int y = (System.Windows.Forms.SystemInformation.WorkingArea.Height - this.Size.Height) / 2;
this.StartPosition = FormStartPosition.Manual; //窗体的位置由Location属性决定
this.Location = (Point)new Size(x, y);
this.laStuId.Text = student.StudentId.ToString();
this.laStuName.Text = student.StudentName.ToString();
this.dgvStuScoreList.AutoGenerateColumns = false;
this.dgvStuScoreList.Columns[0].ReadOnly = true;
this.dgvStuScoreList.Columns[1].ReadOnly = true;
this.dgvStuScoreList.DataSource = courseService.HaveCourseScoreList(this.laStuId.Text);
}
private void AddStuScoreForm_Load(object sender, EventArgs e)
{
}
private void btnClose_Click(object sender, EventArgs e)
{
this.Close();
}
//控制一列输入分数----附加方法
private void ScoreInput(object sender, KeyPressEventArgs e)
{
if (e.KeyChar != 8 && !Char.IsDigit(e.KeyChar) && e.KeyChar != '.')
{
e.Handled = true;
}
}
//控制一列输入分数
private void dgvStuScoreList_EditingControlShowing(object sender, DataGridViewEditingControlShowingEventArgs e)
{
if (this.dgvStuScoreList.CurrentCell.ColumnIndex == 2)
{
e.Control.KeyPress += new KeyPressEventHandler(ScoreInput);
}
}
private void dgvStuScoreList_CellContentClick(object sender, DataGridViewCellEventArgs e)
{
}
private void btnAddScore_Click(object sender, EventArgs e)
{
string[] inputScoreNum = new string[10000];
string[] courseId = new string[10000];
for (int i = 0; i < this.dgvStuScoreList.Rows.Count; i++)
{
inputScoreNum[i] = this.dgvStuScoreList.Rows[i].Cells["ScoreNum"].Value.ToString();
courseId[i] = this.dgvStuScoreList.Rows[i].Cells["CourseId"].Value.ToString();
int StudentId = Convert.ToInt32(this.laStuId.Text.ToString());
Score score = new Score();
score.CourseId = Convert.ToInt32(courseId[i]);
score.StudentId = StudentId;
score.ScoreNum = Convert.ToInt32(inputScoreNum[i]);
int result = Convert.ToInt32(scoreService.AddScore(score));
if (result == 1)
{
// MessageBox.Show("成绩添加成功!","信息提示");
this.DialogResult = DialogResult.OK;
}
}
}
2.分数统计
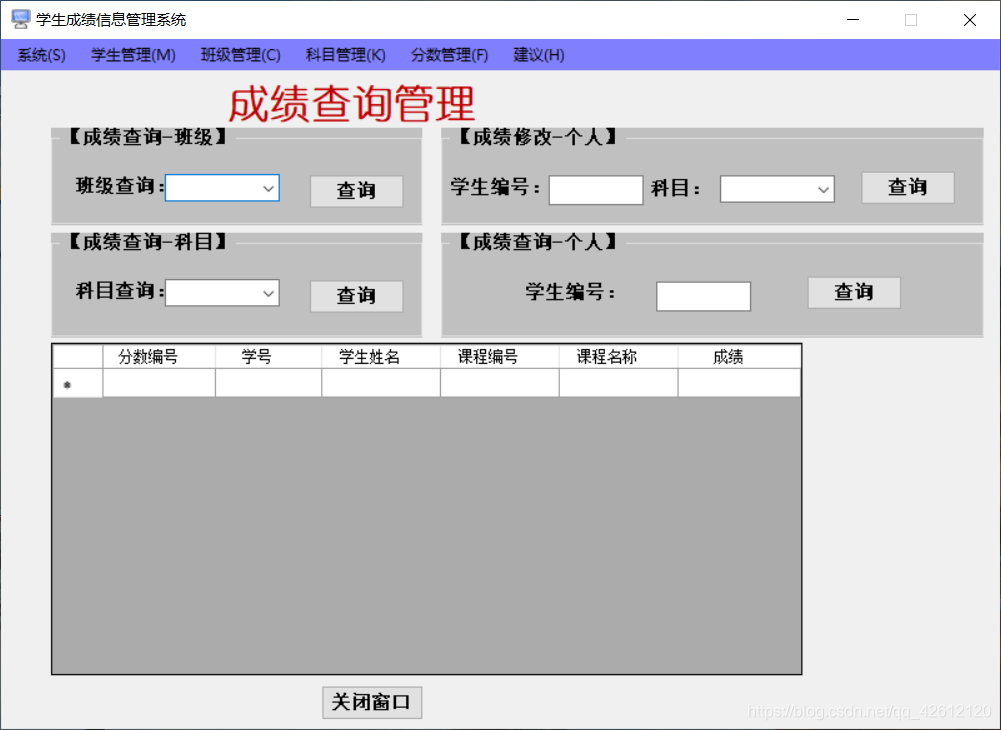
private ClassService classService = new ClassService();
private CourseService courseService = new CourseService();
private ScoreService scoreService = new ScoreService();
private StudentService studentService = new StudentService();
public ListScoreForm()
{
InitializeComponent();
//班级下拉框
this.comListClass.DataSource = classService.GetClassList();
this.comListClass.DisplayMember = "ClassName";
this.comListClass.ValueMember = "ClassId";
this.comListClass.SelectedIndex = -1;
//科目下拉框
this.comListCourse.DataSource = courseService.GetCourseList();
this.comListCourse.DisplayMember = "CourseName";
this.comListCourse.ValueMember = "CourseId";
this.comListCourse.SelectedIndex = -1;
//成绩修改----科目下拉框
this.comEditScoreCourse.DataSource = courseService.GetCourseList();
this.comEditScoreCourse.DisplayMember = "CourseName";
this.comEditScoreCourse.ValueMember = "CourseId";
this.comEditScoreCourse.SelectedIndex = -1;
}
private void btnClose_Click(object sender, EventArgs e)
{
this.Close();
}
//班级下拉框
private void comListClass_SelectedIndexChanged(object sender, EventArgs e)
{
}
//按【班级查询】
private void btnScoreClass_Click(object sender, EventArgs e)
{
if (this.comListClass.SelectedIndex == -1)
{
MessageBox.Show("请选择班级!", "信息提示");
this.comListClass.Focus();
return;
}
List<ScoreVo> list = scoreService.GetScoreVoByClassId(this.comListClass.SelectedValue.ToString());
this.dgvStuScoe.AutoGenerateColumns = false;
this.dgvStuScoe.DataSource = list;
}
//按【科目查询】
private void btnScoreCourse_Click(object sender, EventArgs e)
{
if (this.comListCourse.SelectedIndex == -1)
{
MessageBox.Show("请选择科目!", "信息提示");
this.comListCourse.Focus();
return;
}
string courseId = this.comListCourse.SelectedValue.ToString();
CourseScoreForm courseScoreForm = new CourseScoreForm(courseId);
courseScoreForm.Show();
}
private void ListScoreForm_Load(object sender, EventArgs e)
{
}
//查询学生个人成绩
private void btnShowStudentScore_Click(object sender, EventArgs e)
{
if (this.txtScoreStuId.Text.Trim().Length == 0)
{
MessageBox.Show("请输入学生编号", "信息提示");
this.txtScoreStuId.Focus();
return;
}
Student student = studentService.QueryStuByStudentId(this.txtScoreStuId.Text.Trim().ToString());
if (student.StudentName == null)
{
MessageBox.Show("你输入的学号有误,不存在该学生!!", "");
return;
}
ShowStudentScoreForm showStudentScoreForm = new ShowStudentScoreForm(student);
showStudentScoreForm.Show();
}
//只能输入数字
private void txtScoreStuId_KeyPress(object sender, KeyPressEventArgs e)
{
if (e.KeyChar != '\b')//这是允许输入退格键
{
if ((e.KeyChar < '0') || (e.KeyChar > '9'))
//这是允许输入0-9数字
{
e.Handled = true;
}
}
}
private void txtEditScoreStuId_KeyDown(object sender, KeyEventArgs e) { }
//只能输入数字
private void txtEditScoreStuId_KeyPress(object sender, KeyPressEventArgs e)
{
if (e.KeyChar != '\b')//这是允许输入退格键
{
if ((e.KeyChar < '0') || (e.KeyChar > '9'))
//这是允许输入0-9数字
{
e.Handled = true;
}
}
}
private void comListCourse_SelectedIndexChanged(object sender, EventArgs e)
{
}
//成绩修改
private void btnShowEditScore_Click(object sender, EventArgs e)
{
if (this.txtEditScoreStuId.Text.Trim().Length == 0)
{
MessageBox.Show("请输入学生编号", "信息提示");
this.txtEditScoreStuId.Focus();
return;
}
Student student = studentService.QueryStuByStudentId(this.txtEditScoreStuId.Text.Trim().ToString());
if (student.StudentName == null)
{
MessageBox.Show("你输入的学号有误,不存在该学生!!", "");
return;
}
if (this.comEditScoreCourse.SelectedIndex == -1)
{
MessageBox.Show("请选择科目!", "信息提示");
this.comEditScoreCourse.Focus();
return;
}
Score score = scoreService.GetScoreOne(student.StudentId.ToString(), this.comEditScoreCourse.SelectedValue.ToString());
if (score.ScoreId == 0)
{
MessageBox.Show("成绩不存在,请先录入成绩!");
return;
}
//显示待修改学生的成绩----打开编辑窗口
//把学生Id 课程id 传递过去
EditStudentScoreForm editStudentScoreForm = new EditStudentScoreForm(student.StudentId.ToString(), this.comEditScoreCourse.SelectedValue.ToString());
DialogResult result = editStudentScoreForm.ShowDialog();
if (result == DialogResult.OK)
{
ShowStudentScoreForm showStudentScoreForm = new ShowStudentScoreForm(student);
showStudentScoreForm.Show();
}
}
按班级查询:
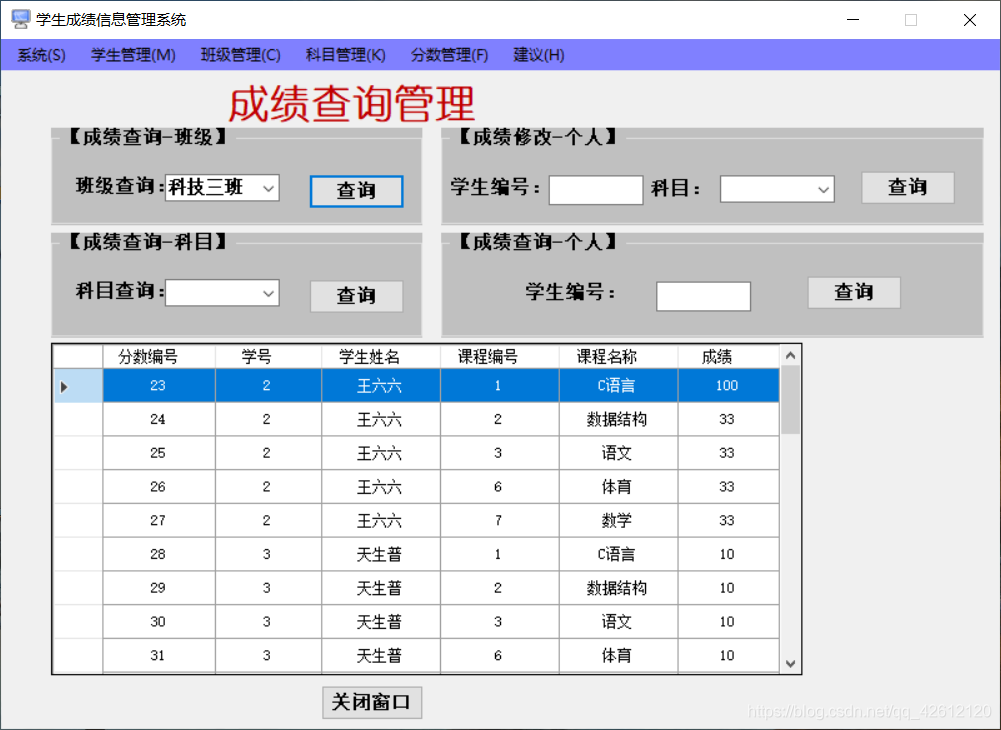
//按照班级查询成绩
public List<ScoreVo> GetScoreVoByClassId(string classId)
{
string sql = "select sc.*,s.StudentName,c.CourseName from Score sc ,Student s, Course c where sc.StudentId = s.StudentId and sc.CourseId = c.CourseId and s.ClassId=" + classId;
SqlDataReader dataReader = SQLHelper.GetReader(sql);
List<ScoreVo> list = new List<ScoreVo>();
while (dataReader.Read())
{
list.Add(new ScoreVo()
{
ScoreId = Convert.ToInt32(dataReader["ScoreId"]),
StudentId = Convert.ToInt32(dataReader["StudentId"]),
CourseId = Convert.ToInt32(dataReader["CourseId"]),
StudentName = dataReader["StudentName"].ToString(),
CourseName = dataReader["CourseName"].ToString(),
ScoreNum = Convert.ToInt32(dataReader["ScoreNum"])
});
}
dataReader.Close();
return list;
}
按科目查询:
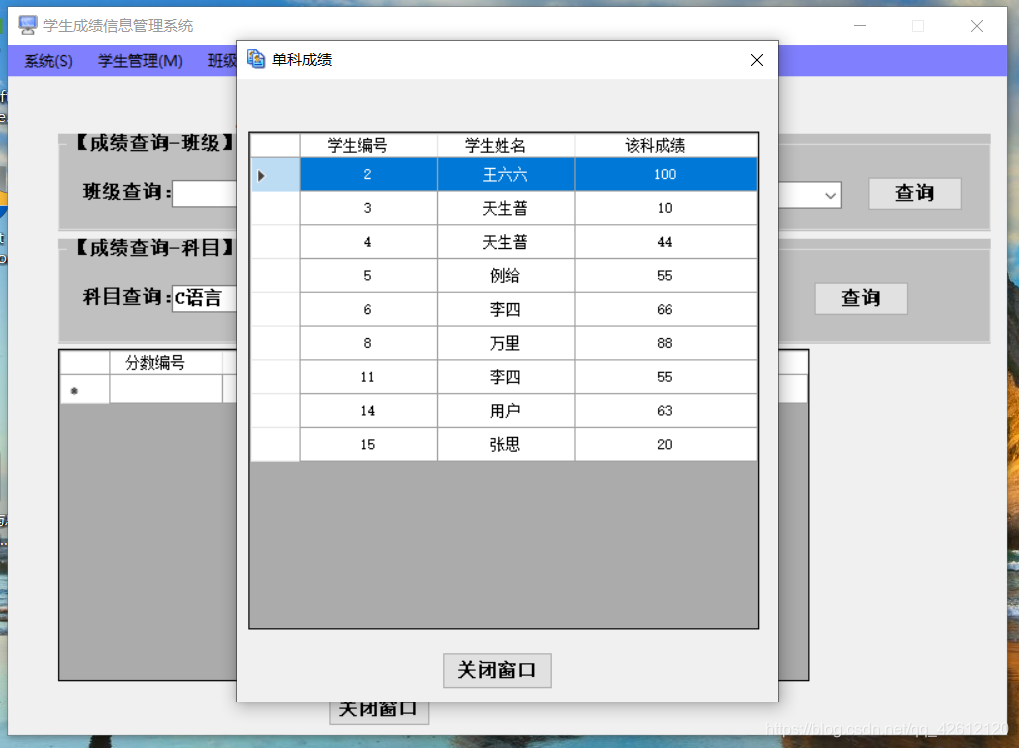
//按照科目查询成绩
public List<ScoreVo> GetScoreVoByCourseId(string courseId)
{
string sql = "select sc.*,s.StudentName from Score sc,Student s where sc.StudentId = s.StudentId and sc.CourseId =" + courseId;
SqlDataReader dataReader = SQLHelper.GetReader(sql);
List<ScoreVo> list = new List<ScoreVo>();
while (dataReader.Read())
{
list.Add(new ScoreVo()
{
ScoreId = Convert.ToInt32(dataReader["ScoreId"]),
StudentId = Convert.ToInt32(dataReader["StudentId"]),
CourseId = Convert.ToInt32(dataReader["CourseId"]),
StudentName = dataReader["StudentName"].ToString(),
ScoreNum = Convert.ToInt32(dataReader["ScoreNum"])
});
}
dataReader.Close();
return list;
}
个人查询:
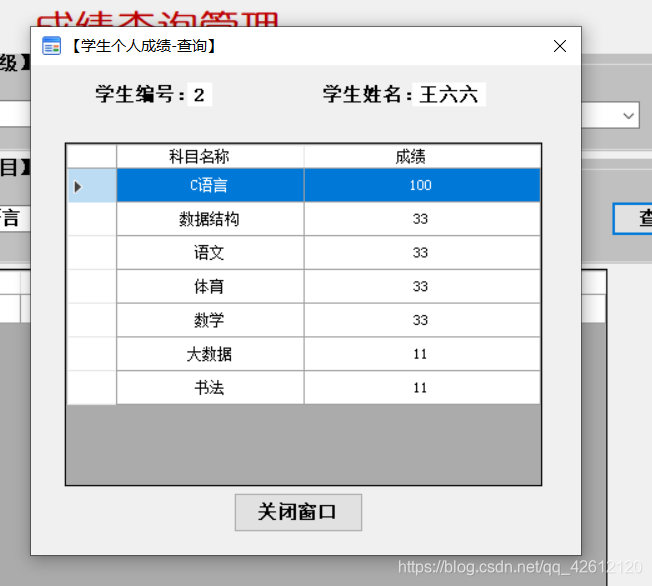
//按照学生id查询成绩
public List<ScoreVo> ShowStuScore(string studentId)
{
string sql = "select s.*,c.CourseName from Score s, Course c where s.CourseId =c.CourseId and StudentId=" + studentId;
SqlDataReader dataReader = SQLHelper.GetReader(sql);
List<ScoreVo> list = new List<ScoreVo>();
while (dataReader.Read())
{
list.Add(new ScoreVo()
{
CourseName = dataReader["CourseName"].ToString(),
ScoreNum = Convert.ToInt32(dataReader["ScoreNum"])
});
}
dataReader.Close();
return list;
}
成绩修改:
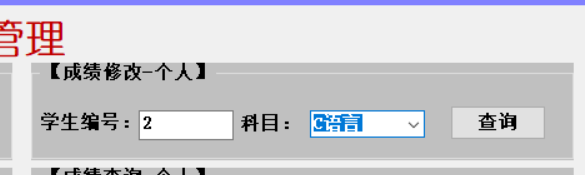
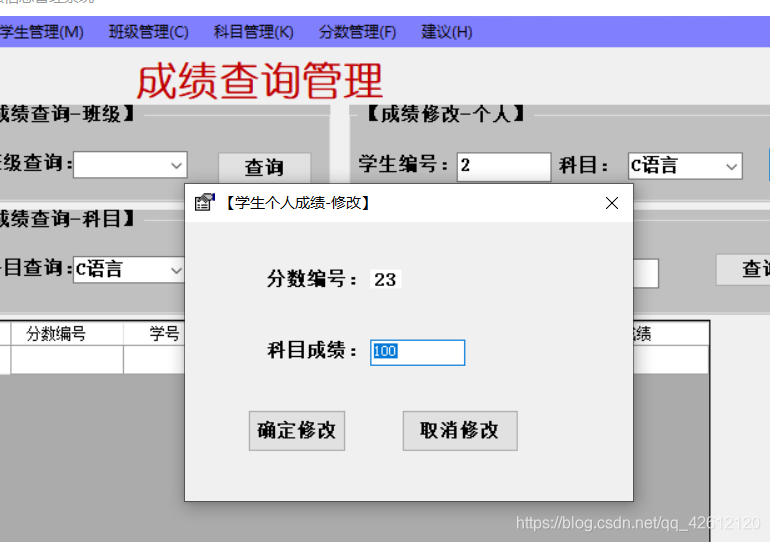
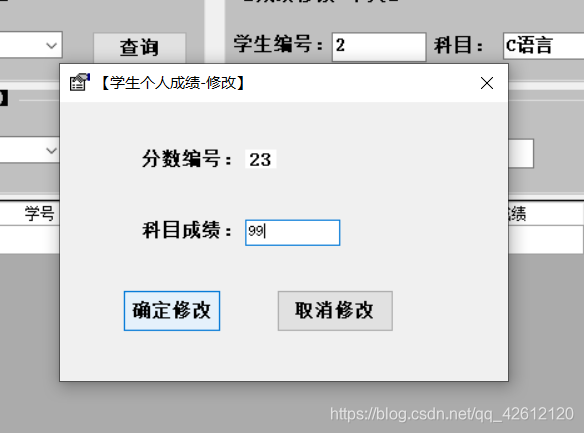
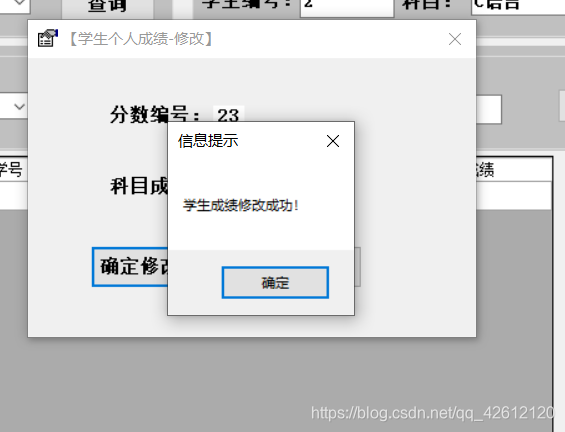
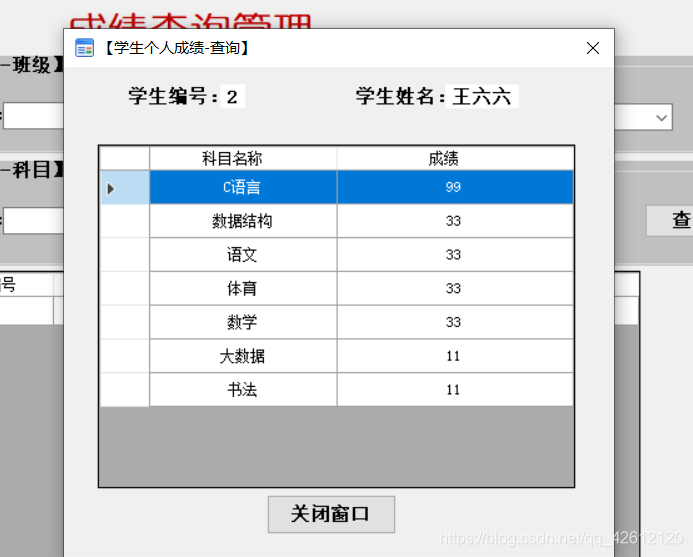
//更新该生成绩
public int EditScore(string scoreId, string scoreNum)
{
string sql = "update score set scoreNum={0} where scoreId={1}";
sql = string.Format(sql, scoreNum,scoreId);
try
{
return (int)SQLHelper.Update(sql);
}
catch (Exception ex)
{
throw new Exception("更新学生成绩存在信息出错!" + ex.Message); ;
}
}
private ScoreService scoreService = new ScoreService();
public EditStudentScoreForm()
{
InitializeComponent();
}
public EditStudentScoreForm(string studentId, string courseId)
{
InitializeComponent();
int x = (System.Windows.Forms.SystemInformation.WorkingArea.Width - this.Size.Width) / 2;
int y = (System.Windows.Forms.SystemInformation.WorkingArea.Height - this.Size.Height) / 2;
this.StartPosition = FormStartPosition.Manual; //窗体的位置由Location属性决定
this.Location = (Point)new Size(x, y);
Score score = scoreService.GetScoreOne(studentId, courseId);
this.laScoreId.Text = score.ScoreId.ToString();
this.txtEditScore.Text = score.ScoreNum.ToString();
}
private void button2_Click(object sender, EventArgs e)
{
this.Close();
}
//修改学生的单科成绩成绩
private void button1_Click(object sender, EventArgs e)
{
if (this.txtEditScore.Text.Trim().Length == 0)
{
MessageBox.Show("请输入成绩!!!", "信息提示");
return;
}
string scoreId = this.laScoreId.Text;
string scoreNum = txtEditScore.Text.Trim().ToString();
//进行判断
try
{
if (scoreService.EditScore(scoreId, scoreNum) == 1)
{
MessageBox.Show("学生成绩修改成功!", "信息提示");
this.DialogResult = DialogResult.OK;
this.Close();
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}