7.21 修改Sales_data类使其隐藏实现的细节strcut-class
#ifndef ex7_21_h
#define ex7_21_h
#include <string>
#include <iostream>
using namespace std;
class Sales_data
{
friend istream &read(istream &is, Sales_data &item);
friend ostream &print(ostream &os, const Sales_data &item);
friend Sales_data add(const Sales_data &lhs, const Sales_data &rhs);
public:
Sales_data() = default;
Sales_data(const string &s):bookNo(s) {}
Sales_data(const string &s, unsigned &n, double &p) : bookNo(s), units_sold(n), revenue(n*p) {}
Sales_data(istream &is) {read(is, *this);}
string isbn() const {return bookNo;};
Sales_data& combine(const Sales_data&);
private:
string bookNo;
unsigned units_sold = 0;
double revenue = 0.0;
};
Sales_data& Sales_data::combine(const Sales_data &rhs)
{
units_sold += rhs.units_sold;
revenue += rhs.revenue;
}
istream &read(istream &is, Sales_data &item)
{
double price = 0;
is >> item.bookNo >> item.units_sold >> price;
item.revenue = price * item.units_sold;
}
ostream &print(ostream &os, const Sales_data &item)
{
os << item.isbn() << " " << item.units_sold << " " << item.revenue;
return os;
}
Sales_data add(const Sales_data &lhs, const Sales_data &rhs)
{
Sales_data sum = lhs;
sum.combine(rhs);
return sum;
}
#endif
7.22 修改Person类使其隐藏实现的细节strcut-class
#ifndef ex7_22_h
#define ex7_22_h
#include <string>
#include <iostream>
using namespace std;
class Person
{
friend std::istream &read(std::istream &is, Person &person);
friend std::ostream &print(std::ostream &os, const Person &person);
public:
Person() = default;
Person(const std::string sname, const std::string saddr):name(sname), address(saddr){ }
Person(std::istream &is){ read(is, *this); }
std::string getName() const { return name; }
std::string getAddress() const { return address; }
private://私有成员,封装实现细节
std::string name;
std::string address;
};
std::istream &read(std::istream &is, Person &person)
{
is >> person.name >> person.address;
return is;
}
std::ostream &print(std::ostream &os, const Person &person)
{
os << person.name << " " << person.address;
return os;
}
#endif
7.23-24 编写自己的Screen类
#ifndef ex7_24_h
#define ex7_24_h
#include <string>
using namespace std;
class Screen
{
public:
using pos = string::size_type;
Screen() = default;
Screen(pos ht, pos wd):height(ht), width(wd), contents(ht*wd, ' '){ }
Screen(pos ht, pos wd, char c):height(ht), width(wd), contents(ht*wd, c){}
char get() const { return contents[cursor]; }
char get(pos r, pos c) const { return contents[r*width+c]; }
private:
pos cursor = 0;
pos height = 0, width = 0;
string contents;
};
#endif
7.26 将Sales_data::avg_price定义成内联函数
#ifndef ex7_26_h
#define ex7_26_h
#include <string>
#include <iostream>
using namespace std;
class Sales_data
{
friend istream &read(istream &is, Sales_data &item);
friend ostream &print(ostream &os, const Sales_data &item);
friend Sales_data add(const Sales_data &lhs, const Sales_data &rhs);
public:
Sales_data() = default;
Sales_data(const string &s):bookNo(s) {}
Sales_data(const string &s, unsigned &n, double &p) : bookNo(s), units_sold(n), revenue(n*p) {}
Sales_data(istream &is) {read(is, *this);}
string isbn() const {return bookNo;};
Sales_data& combine(const Sales_data&);
private:
inline double avg_price() const;
private:
string bookNo;
unsigned units_sold = 0;
double revenue = 0.0;
};
inline
double Sales_data::avg_price() const
{
return units_sold ? revenue/units_sold : 0;
}
Sales_data& Sales_data::combine(const Sales_data &rhs)
{
units_sold += rhs.units_sold;
revenue += rhs.revenue;
}
istream &read(istream &is, Sales_data &item)
{
double price = 0;
is >> item.bookNo >> item.units_sold >> price;
item.revenue = price * item.units_sold;
}
ostream &print(ostream &os, const Sales_data &item)
{
os << item.isbn() << " " << item.units_sold << " " << item.revenue;
return os;
}
Sales_data add(const Sales_data &lhs, const Sales_data &rhs)
{
Sales_data sum = lhs;
sum.combine(rhs);
return sum;
}
#endif
7.27 给Screen类添加move、set、display函数,执行代码检验是否正确
#ifndef ex7_27_h
#define ex7_27_h
#include <string>
#include <iostream>
using namespace std;
class Screen
{
public:
using pos = string::size_type;
Screen() = default;
Screen(pos ht, pos wd):height(ht), width(wd), contents(ht*wd, ' '){ }
Screen(pos ht, pos wd, char c):height(ht), width(wd), contents(ht*wd, c){}
char get() const { return contents[cursor]; }
char get(pos r, pos c) const { return contents[r*width+c]; }
inline Screen& move(pos r, pos c);
inline Screen& set(char c);
inline Screen& set(pos r, pos c, char ch);
const Screen& display(std::ostream& os) const
{
do_display(os);
return *this;
}
Screen& display(std::ostream& os)
{
do_display(os);
return *this;
}
private:
void do_display(std::ostream& os) const { os << contents; }
private:
pos cursor = 0;
pos height = 0, width = 0;
string contents;
};
inline Screen& Screen::move(pos r, pos c)
{
cursor = r * width + c;
return *this;
}
inline Screen& Screen::set(char c)
{
contents[cursor] = c;
return *this;
}
inline Screen& Screen::set(pos r, pos c, char ch)
{
contents[r * width + c] = ch;
return *this;
}
#endif
#include "ex7_27.h"
using namespace std;
int main()
{
Screen myScreen(5, 5, 'X');
myScreen.move(4, 0).set('#').display(cout);
cout << "\n";
myScreen.display(cout);
cout << "\n";
system("pause");
return 0;
}
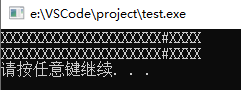
7.32 定义Screen和Window_mgr,其中clear是Window_mgr的成员,是Screen的友元
#ifndef ex7_32_h
#define ex7_32_h
#include <string>
#include <iostream>
#include <vector>
using namespace std;
class Window_mgr
{
public:
using ScreenIndex = vector<Screen>::size_type;
inline void clear(ScreenIndex);
private:
vector<Screen> screens;
};
class Screen
{
friend void Window_mgr::clear(ScreenIndex);
public:
using pos = string::size_type;
Screen() = default;
Screen(pos ht, pos wd):height(ht), width(wd), contents(ht*wd, ' '){ }
Screen(pos ht, pos wd, char c):height(ht), width(wd), contents(ht*wd, c){}
char get() const { return contents[cursor]; }
char get(pos r, pos c) const { return contents[r*width+c]; }
inline Screen& move(pos r, pos c);
inline Screen& set(char c);
inline Screen& set(pos r, pos c, char ch);
const Screen& display(std::ostream& os) const
{
do_display(os);
return *this;
}
Screen& display(std::ostream& os)
{
do_display(os);
return *this;
}
private:
void do_display(std::ostream& os) const { os << contents; }
private:
pos cursor = 0;
pos height = 0, width = 0;
string contents;
};
inline void Window_mgr::clear(ScreenIndex i)
{
if (i >= screens.size())
return;
Screen& s = screens[i];
s.contents = std::string(s.height * s.width, ' ');
}
inline Screen& Screen::move(pos r, pos c)
{
cursor = r * width + c;
return *this;
}
inline Screen& Screen::set(char c)
{
contents[cursor] = c;
return *this;
}
inline Screen& Screen::set(pos r, pos c, char ch)
{
contents[r * width + c] = ch;
return *this;
}
#endif
7.41 委托构造函数,给每个构造函数体添加一条语句,令其一旦执行就打印一条信息,理解委托构造函数的执行顺序
#ifndef ex7_41_h
#define ex7_41_h
#include <string>
#include <iostream>
#include <vector>
using namespace std;
class Sales_data
{
friend istream &read(istream &is, Sales_data &item);
friend ostream &print(ostream &os, const Sales_data &item);
friend Sales_data add(const Sales_data &lhs, const Sales_data &rhs);
public:
Sales_data(const string &s, unsigned &n, double &p) : bookNo(s), units_sold(n), revenue(n*p)
{ std::cout << "Sales_data(const std::string&, unsigned, double)" << std::endl; }
Sales_data() : Sales_data("", 0, 0.0f)
{ std::cout << "Sales_data()" << std::endl; }
Sales_data(const std::string &s) : Sales_data(s, 0, 0.0f)
{ std::cout << "Sales_data(const std::string&)" << std::endl; }
Sales_data(std::istream &is);
string isbn() const {return bookNo;};
Sales_data& combine(const Sales_data&);
private:
inline double avg_price() const;
private:
string bookNo;
unsigned units_sold = 0;
double revenue = 0.0;
};
inline
double Sales_data::avg_price() const
{
return units_sold ? revenue/units_sold : 0;
}
Sales_data& Sales_data::combine(const Sales_data &rhs)
{
units_sold += rhs.units_sold;
revenue += rhs.revenue;
}
istream &read(istream &is, Sales_data &item)
{
double price = 0;
is >> item.bookNo >> item.units_sold >> price;
item.revenue = price * item.units_sold;
}
ostream &print(ostream &os, const Sales_data &item)
{
os << item.isbn() << " " << item.units_sold << " " << item.revenue;
return os;
}
Sales_data add(const Sales_data &lhs, const Sales_data &rhs)
{
Sales_data sum = lhs;
sum.combine(rhs);
return sum;
}
#endif
#include "ex7_41.h"
using namespace std;
int main()
{
cout << "1. default way: " << endl;
cout << "----------------" << endl;
Sales_data s1;
cout << "\n2. use std::string as parameter: " << endl;
cout << "----------------" << endl;
Sales_data s2("CPP-Primer-5th");
cout << "\n3. complete parameters: " << endl;
cout << "----------------" << endl;
Sales_data s3("CPP-Primer-5th", 3, 25.8);
cout << "\n4. use istream as parameter: " << endl;
cout << "----------------" << endl;
Sales_data s4(std::cin);
system("pause");
return 0;
}
7.57 定义账户类-含有static成员
#ifndef ex7_57_h
#define ex7_57_h
#include <string>
#include <iostream>
#include <vector>
using namespace std;
class Account
{
public:
void caculate() { amount += amount * interestRate; }
static double rate() { return interestRate; }
static void rate(double newRate) { interestRate = newRate}
private:
string owner;
double amount;
static double interestRate;
static constexpr double todayRate = 42.42;
static double initRate() { return todayRate; }
};
double Account::interestRate = initRate();
#endif