from keras.datasets import cifar10
(x_train, y_train), (x_test, y_test) = cifar10.load_data()
x_train[0]
array([[[ 59, 62, 63],
[ 43, 46, 45],
[ 50, 48, 43],
...,
[158, 132, 108],
[152, 125, 102],
[148, 124, 103]],
[[ 16, 20, 20],
[ 0, 0, 0],
[ 18, 8, 0],
...,
[123, 88, 55],
[119, 83, 50],
[122, 87, 57]],
[[ 25, 24, 21],
[ 16, 7, 0],
[ 49, 27, 8],
...,
[118, 84, 50],
[120, 84, 50],
[109, 73, 42]],
...,
[[208, 170, 96],
[201, 153, 34],
[198, 161, 26],
...,
[160, 133, 70],
[ 56, 31, 7],
[ 53, 34, 20]],
[[180, 139, 96],
[173, 123, 42],
[186, 144, 30],
...,
[184, 148, 94],
[ 97, 62, 34],
[ 83, 53, 34]],
[[177, 144, 116],
[168, 129, 94],
[179, 142, 87],
...,
[216, 184, 140],
[151, 118, 84],
[123, 92, 72]]], dtype=uint8)
x_train.shape
(50000, 32, 32, 3)
统计各个通道的均值
r_mean = x_train[:,:,:,0].mean()
r_mean
125.306918046875
g_mean = x_train[:,:,:,1].mean()
g_mean
122.950394140625
b_mean = x_train[:,:,:,2].mean()
b_mean
113.86538318359375
统计各个通道的标准差
r_std = x_train[:,:,:,0].std()
g_std = x_train[:,:,:,1].std()
b_std = x_train[:,:,:,2].std()
print(r_std,g_std,b_std)
62.993219278136884 62.08870764001421 66.70489964063091
减均值除标准差
mean = [125.307, 122.95, 113.865]
std = [62.9932, 62.0887, 66.7048]
for i in range(3):
x_train[:,:,:,i] = (x_train[:,:,:,i] - mean[i]) / std[i]
总结
def normalize_preprocessing(x_train,x_test):
x_train = x_train.astype('float32')
x_test = x_test.astype('float32')
mean = np.array([125.307, 122.95, 113.865])
std = np.array([62.9932, 62.0887, 66.7048])
x_train = (x_train - mean) / std
x_test = (x_test - mean) / std
return x_train, x_test
(x_train, y_train), (x_test, y_test) = cifar10.load_data()
x_train, x_test = color_preprocessing(x_train, x_test)
'''现在整个数据集的均值为 0 方差为 1 了'''
x_train[0]
array([[[-1.0526057e+00, -9.8166007e-01, -7.6253873e-01],
[-1.3066014e+00, -1.2393559e+00, -1.0323844e+00],
[-1.1954782e+00, -1.2071439e+00, -1.0623672e+00],
...,
[ 5.1899254e-01, 1.4575927e-01, -8.7924674e-02],
[ 4.2374417e-01, 3.3017330e-02, -1.7787321e-01],
[ 3.6024526e-01, 1.6911339e-02, -1.6288179e-01]],
[[-1.7352191e+00, -1.6581117e+00, -1.4071699e+00],
[-1.9892148e+00, -1.9802315e+00, -1.7069985e+00],
[-1.7034696e+00, -1.8513836e+00, -1.7069985e+00],
...,
[-3.6622990e-02, -5.6290430e-01, -8.8247013e-01],
[-1.0012191e-01, -6.4343429e-01, -9.5742726e-01],
[-5.2497718e-02, -5.7901031e-01, -8.5248733e-01]],
[[-1.5923465e+00, -1.5936877e+00, -1.3921785e+00],
[-1.7352191e+00, -1.8674896e+00, -1.7069985e+00],
[-1.2113529e+00, -1.5453697e+00, -1.5870671e+00],
...,
[-1.1599664e-01, -6.2732828e-01, -9.5742726e-01],
[-8.4247179e-02, -6.2732828e-01, -9.5742726e-01],
[-2.5886920e-01, -8.0449420e-01, -1.0773586e+00]],
...,
[[ 1.3127290e+00, 7.5778693e-01, -2.6782176e-01],
[ 1.2016059e+00, 4.8398507e-01, -1.1972901e+00],
[ 1.1539817e+00, 6.1283296e-01, -1.3172214e+00],
...,
[ 5.5074203e-01, 1.6186525e-01, -6.5759879e-01],
[-1.1002299e+00, -1.4809458e+00, -1.6020585e+00],
[-1.1478541e+00, -1.4326278e+00, -1.4071699e+00]],
[[ 8.6823660e-01, 2.5850120e-01, -2.6782176e-01],
[ 7.5711352e-01, 8.0534868e-04, -1.0773586e+00],
[ 9.6348500e-01, 3.3903116e-01, -1.2572558e+00],
...,
[ 9.3173552e-01, 4.0345511e-01, -2.9780459e-01],
[-4.4936597e-01, -9.8166007e-01, -1.1972901e+00],
[-6.7161220e-01, -1.1266140e+00, -1.1972901e+00]],
[[ 8.2061243e-01, 3.3903116e-01, 3.2006722e-02],
[ 6.7773986e-01, 9.7441293e-02, -2.9780459e-01],
[ 8.5236186e-01, 3.0681917e-01, -4.0274459e-01],
...,
[ 1.4397268e+00, 9.8327076e-01, 3.9180091e-01],
[ 4.0786946e-01, -7.9724602e-02, -4.4771886e-01],
[-3.6622990e-02, -4.9848035e-01, -6.2761593e-01]]], dtype=float32)
import matplotlib.pyplot as plt
plt.imshow(x_train[0])
Clipping input data to the valid range for imshow with RGB data ([0..1] for floats or [0..255] for integers).
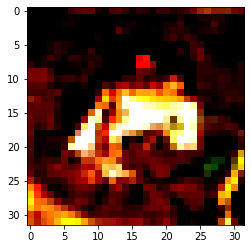