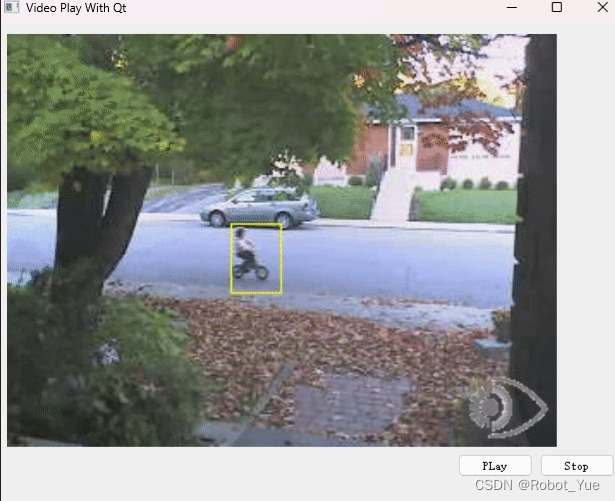
1. mainwindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include <opencv2/opencv.hpp>
#include <QTimer>
#include <QPixmap>
#include <QDebug>
#include <QImage>
using namespace cv;
using namespace std;
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow {
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void on_btnPlay_clicked();
void on_btnStop_clicked();
void importFrame();
private:
Ui::MainWindow *ui;
VideoCapture capture;
QTimer *timer;
Mat frame;
Ptr<BackgroundSubtractorMOG2>ptrMOG;
};
#endif
2. mainwindow.cpp
#include "mainwindow.h"
#include "ui_mainwindow.h"
MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) {
ui->setupUi(this);
this->setWindowTitle("Video Play With Qt");
ptrMOG = createBackgroundSubtractorMOG2(500, 16, false);
timer = new QTimer(this);
connect(timer, SIGNAL(timeout()), this, SLOT(importFrame()));
}
MainWindow::~MainWindow() {
delete ui;
}
void MainWindow::on_btnPlay_clicked() {
capture.open("D:\\BaiduNetdiskDownload\\bike.avi");
double rate = capture.get(CAP_PROP_FPS);
double timerDelay = 1000 / rate;
timer->start(timerDelay);
}
void MainWindow::on_btnStop_clicked() {
timer->stop();
capture.release();
}
void MainWindow::importFrame() {
Mat currentFrame;
Mat grayFrame, foreGround;
capture >> currentFrame;
if (!currentFrame.empty()) {
qDebug() << "Video is running...";
Mat result = currentFrame.clone();
ptrMOG->apply(currentFrame, foreGround, 0.05);
threshold(foreGround, foreGround, 25, 255, THRESH_BINARY);
Mat kernelErode = getStructuringElement(MORPH_RECT, Size(5, 5));
erode(foreGround, foreGround, kernelErode);
Mat kernelDilate = getStructuringElement(MORPH_RECT, Size(11, 11));
dilate(foreGround, foreGround, kernelDilate);
vector<vector<Point>>contours;
findContours(foreGround, contours, RETR_EXTERNAL, CHAIN_APPROX_NONE);
for (uint64 i = 0; i < contours.size(); i++) {
rectangle(result, boundingRect(contours[i]), Scalar(0, 255, 255), 1);
}
cvtColor(result, result, COLOR_BGR2RGB);
QImage showVideo = QImage((const unsigned char*)(result.data), result.cols, result.rows, result.step, QImage::Format_RGB888);
ui->labelVideo->setPixmap(QPixmap::fromImage(showVideo.scaled(ui->labelVideo->size(), Qt::KeepAspectRatio, Qt::SmoothTransformation)));
} else {
qDebug() << "Play is over.";
timer->stop();
}
}