1、首先去阿里云申请号码隐私保护服务,申请号码池以及专属号码,获取有效的阿里云AK。
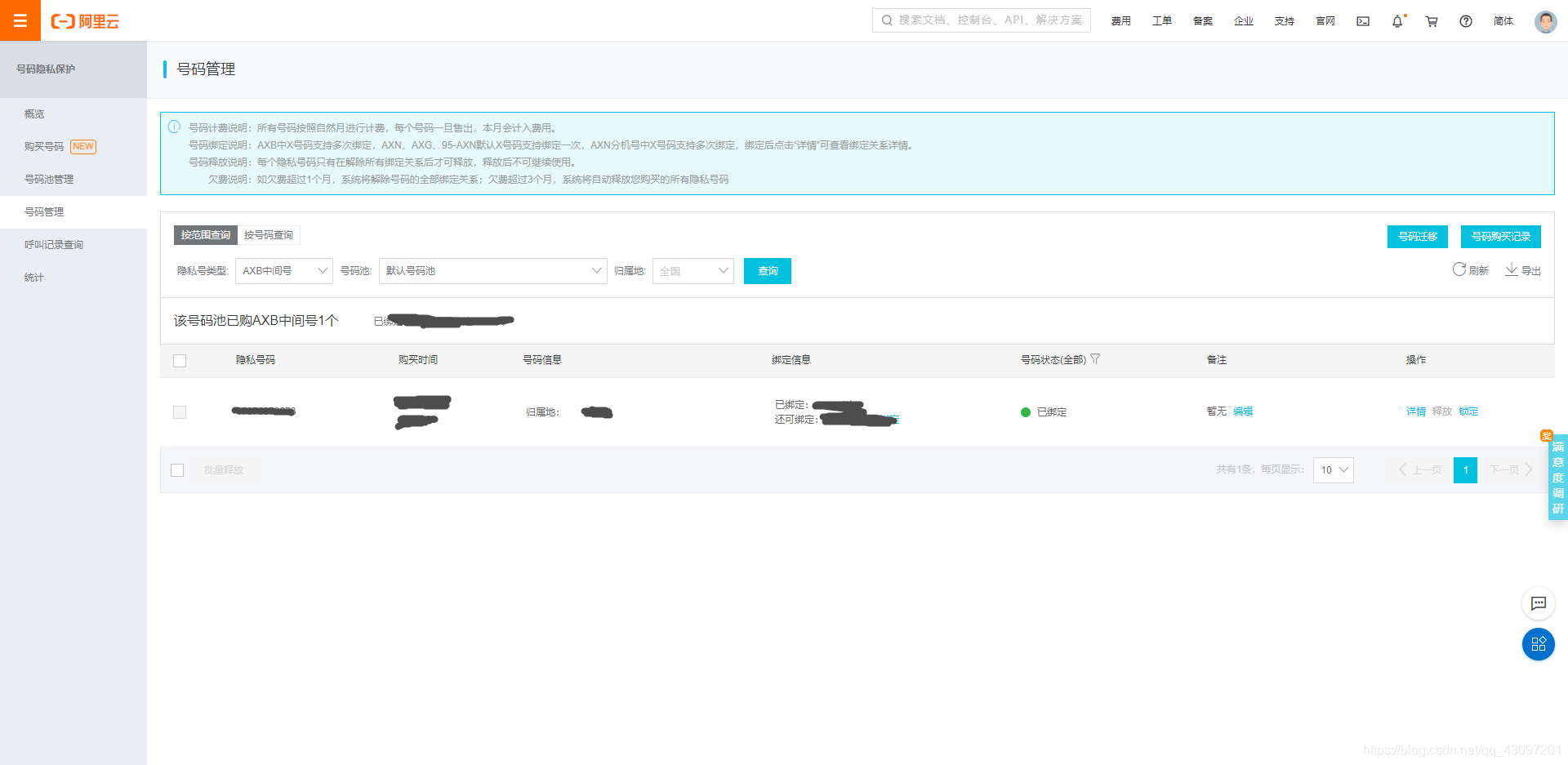
2、导入以下两个依赖
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>aliyun-java-sdk-dyplsapi</artifactId>
<version>1.2.0</version>
</dependency>
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>aliyun-java-sdk-core</artifactId>
<version>4.1.0</version>
</dependency>
3、在yml文件中写入有效的AK
oss:
product: Dyplsapi
domain: dyplsapi.aliyuncs.com
accessKeyId: XXXXXXXXXXXXXXXXXXXXXX
accessKeySecret: XXXXXXXXXXXXXXXX
4、调用代码
import com.aliyuncs.DefaultAcsClient;
import com.aliyuncs.IAcsClient;
import com.aliyuncs.dyplsapi.model.v20170525.*;
import com.aliyuncs.exceptions.ClientException;
import com.aliyuncs.profile.DefaultProfile;
import com.aliyuncs.profile.IClientProfile;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Configuration;
@Configuration
public class PhoneEncryption {
@Autowired
private RedisUtils redisUtils;
@Value("${oss.product}")
private String product;
@Value("${oss.domain}")
private String domain;
@Value("${oss.accessKeyId}")
private String accessKeyId;
@Value("${oss.accessKeySecret}")
private String accessKeySecret;
public BindAxbResponse bindAxb(String phoneA, String phoneB) throws ClientException {
System.setProperty("sun.net.client.defaultConnectTimeout", "10000");
System.setProperty("sun.net.client.defaultReadTimeout", "10000");
IClientProfile profile = DefaultProfile.getProfile("cn-hangzhou", accessKeyId, accessKeySecret);
DefaultProfile.addEndpoint("cn-hangzhou", "cn-hangzhou", product, domain);
IAcsClient acsClient = new DefaultAcsClient(profile);
BindAxbRequest request = new BindAxbRequest();
request.setPoolKey("XXXXXXXXXXXXXX");
request.setPhoneNoA(phoneA);
request.setPhoneNoB(phoneB);
request.setPhoneNoX("XXXXXXXXXXXXXX");
request.setExpiration(DateTimeHelper.timestampToLocalDateTimeStr((System.currentTimeMillis() + 100000L)));
request.setIsRecordingEnabled(false);
BindAxbResponse response = acsClient.getAcsResponse(request);
if (response.getCode() != null && response.getCode().equals("OK")) {
return response;
}
return null;
}
public UnbindSubscriptionResponse unbind(String subsId, String secretNo) throws ClientException {
System.setProperty("sun.net.client.defaultConnectTimeout", "10000");
System.setProperty("sun.net.client.defaultReadTimeout", "10000");
IClientProfile profile = DefaultProfile.getProfile("cn-hangzhou", accessKeyId, accessKeySecret);
DefaultProfile.addEndpoint("cn-hangzhou", "cn-hangzhou", product, domain);
IAcsClient acsClient = new DefaultAcsClient(profile);
UnbindSubscriptionRequest request = new UnbindSubscriptionRequest();
request.setPoolKey("XXXXXXXXXXXXXXXXXXXX");
request.setSecretNo(secretNo);
request.setSubsId(subsId);
UnbindSubscriptionResponse response = acsClient.getAcsResponse(request);
return response;
}
public Boolean phoneEncryption(String phoneA, String phoneB) {
String cacheSubsId = redisUtils.get(CACHE_KEY);
if (GeneralUtil.isNotNullAndEmpty(cacheSubsId)) {
try {
unbind(cacheSubsId, "XXXXXXXXXXXXXXXXXXXX");
} catch (ClientException e) {
e.printStackTrace();
}
}
BindAxbResponse axbResponse = null;
try {
axbResponse = bindAxb(phoneA, phoneB);
} catch (ClientException e) {
e.printStackTrace();
}
String axbSubsId = axbResponse.getSecretBindDTO() == null ? null : axbResponse.getSecretBindDTO().getSubsId();
if (GeneralUtil.isObjNotNull(axbResponse) && GeneralUtil.isNotNullAndEmpty(axbSubsId)) {
redisUtils.set(CACHE_KEY, axbSubsId);
LogUtil.info("Processing phoneEncryption success!Code = %s , RequestId = %s , subsId = %s"
, axbResponse.getCode(), axbResponse.getRequestId(), axbSubsId);
return true;
}
return false;
}
}