1、BOM编程
BOM(browser Object Model)浏览器对象模型 操作浏览器
window
window.location :Location 对象包含有关当前 URL 的信息。Location 对象是 Window 对象的一个部分,可通过 window.location 属性来访问。
window.history:History 对象包含用户(在浏览器窗口中)访问过的 URL。History 对象是 window 对象的一部分,可通过 window.history 属性对其进行访问。
window.navigator:Navigator 对象包含有关浏览器的信息。
window.screen:Screen 对象包含有关客户端显示屏幕的信息。
window.document:每个载入浏览器的 HTML 文档都会成为 Document 对象。Document 对象使我们可以从脚本中对 HTML 页面中的所有元素进行访问。提示:Document 对象是 Window 对象的一部分,可通过 window.document 属性对其进行访问。
window对象的常见方法:
alert()
<html>
<head>
<script type="text/javascript">
function display_alert()
{
alert("I am an alert box!!")
}
</script>
</head>
<body>
<input type="button" onclick="display_alert()"
value="Display alert box" />
</body>
</html>
confirm():显示带有一段消息以及确认按钮和取消按钮的对话框。
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript">
function disp_confirm() {
var r = confirm("Press a button")
if (r == true) {
document.write("You pressed OK!")
} else {
document.write("You pressed Cancel!")
}
}
</script>
</head>
<body>
<input type="button" onclick="disp_confirm()" value="Display a confirm box" />
</body>
</html>
prompt():prompt() 方法用于显示可提示用户进行输入的对话框。
<html>
<head>
<script type="text/javascript">
function disp_prompt()
{
var name=prompt("Please enter your name","")
if (name!=null && name!="")
{
document.write("Hello " + name + "!")
}
}
</script>
</head>
<body>
<input type="button" onclick="disp_prompt()"
value="Display a prompt box" />
</body>
</html>
close():关闭浏览器
function myClose() {
window.close();
}
print():打印内容
setInteval(fn, ms);setInterval() 方法可按照指定的周期(以毫秒计)来调用函数或计算表达式。setInterval() 方法会不停地调用函数,直到 clearInterval() 被调用或窗口被关闭。由 setInterval() 返回的 ID 值可用作 clearInterval() 方法的参数。
setTimeout(fn, ms);
setTimeout(function() {
// clearInterval(id)清除对应的setInterval
window.clearInterval(timer);
}, 5000);
clearInteval(id);clearInterval() 方法可取消由 setInterval() 设置的 timeout。clearInterval() 方法的参数必须是由 setInterval() 返回的 ID 值。
<html>
<body>
<input type="text" id="clock" size="35" />
<script language=javascript>
var int=self.setInterval("clock()",50)
function clock()
{
var t=new Date()
document.getElementById("clock").value=t
}
</script>
</form>
<button onclick="int=window.clearInterval(int)">
Stop interval</button>
</body>
</html>
clearTimeout(id);clearTimeout() 方法可取消由 setTimeout() 方法设置的 timeout。
<html>
<head>
<script type="text/javascript">
var c=0
var t
function timedCount()
{
document.getElementById('txt').value=c
c=c+1
t=setTimeout("timedCount()",1000)
}
function stopCount()
{
clearTimeout(t)
}
</script>
</head>
<body>
<form>
<input type="button" value="Start count!" onClick="timedCount()">
<input type="text" id="txt">
<input type="button" value="Stop count!" onClick="stopCount()">
</form>
</body>
</html>
scrollTo:scrollTo() 方法可把内容滚动到指定的坐标。
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript">
function scrollWindow() {
window.scrollTo(100, 500)
}
</script>
</head>
<body>
<input type="button" onclick="scrollWindow()" value="Scroll" />
<p>SCROLL SCROLL SCROLL SCROLL SCROLL SCROLL SCROLL SCROLL</p>
<div style="height: 1000px;">
</div>
<p>SCROLL SCROLL SCROLL SCROLL SCROLL SCROLL SCROLL SCROLL</p>
</body>
</html>
scrollBy:方法可把内容滚动指定的像素数。(谷歌浏览器不生效)
resizeBy:resizeBy() 方法用于根据指定的像素来调整窗口的大小。(谷歌浏览器不生效)
<html>
<head>
<script type="text/javascript">
function resizeWindow()
{
window.resizeBy(-100,-100)
}
</script>
</head>
<body>
<input type="button" onclick="resizeWindow()"
value="Resize window">
</body>
</html>
resizeTo:resizeTo() 方法用于把窗口大小调整为指定的宽度和高度。(谷歌浏览器不生效)
<html>
<head>
<script type="text/javascript">
function resizeWindow()
{
window.resizeTo(500,300)
}
</script>
</head>
<body>
<input type="button" onclick="resizeWindow()"
value="Resize window">
</body>
</html>
open():open() 方法用于打开一个新的浏览器窗口或查找一个已命名的窗口。
history 浏览器的历史记录 浏览器地址栏 renow(刷新)
<!DOCTYPE html>
<html>
<head>
<title></title>
<meta charset="utf-8"/>
<script type="text/javascript">
function gotos() {
window.history.forward();
}
function goback() {
window.history.back();
}
function gos() {
history.go(-1)
}
</script>
</head>
<body>
<button onclick="gotos()">前进</button>
<a href="02.回到顶部.html">回到顶部</a>
<button onclick="goback()">后退</button>
<button onclick="gos()">go</button>
</body>
</html>
sareen:Screen 对象包含有关客户端显示屏幕的信息。注释:没有应用于 screen 对象的公开标准,不过所有浏览器都支持该对象。
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<script type="text/javascript">
// 电脑的分辨率的宽和高
console.info(window.screen.height)
console.log(screen.width)
console.info(screen.availHeight)
console.info(screen.availWidth)
// 浏览器用户代理对象
console.info(navigator.userAgent);
</script>
</head>
<body>
</body>
</html>
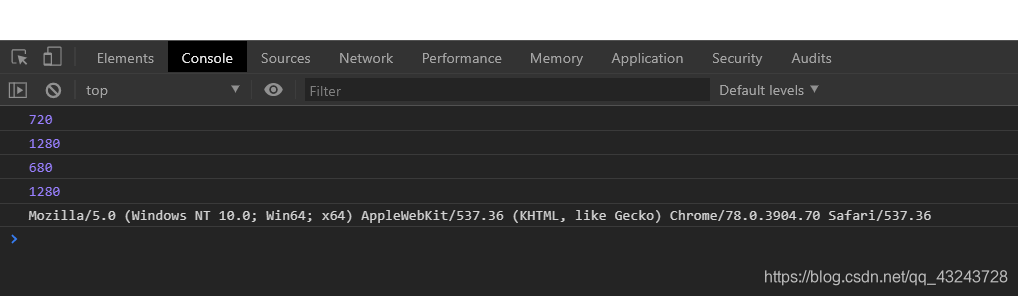
DOM编程
DOM全称Document Object Model,即文档对象模型。 一个html页面被浏览器加载的时候,浏览器就会对整个html页面上的所有标签都会创建一个对应的对象进行描述,我在浏览器上看到的信息只不过就是这些html对象的属性信息而已。我们只要能找到对应的对象操作对象的属性,则可以改变浏览器当前显示的内容。 DOM描绘了一个层次化的树,允许开发人员添加、删除、修改页面的某一部分浏览器在解析HTML页面标记的时候,其实不是按照一行一行读取并解析的, 而是将HTML页面中的每一个标记按照顺序在内存中组建一颗DOM树,组建好之后,按照树的结构将页面显示在浏览器的窗口中。
查找DOM对象的几种方式
1、直接使用id获取对象,虽然很简单 但是这种写法对于程序员阅读理解有难度,不推荐使用
console.info(show);
2、利用api getElementById('id') (重要)
var show = document.getElementById("show");
console.info(show)
show.innerHTML = "朕聪哥";
3、使用类名称来获取对应的标签 (掌握)
var acs = document.getElementsByClassName("active");
console.info(acs)
acs[0].innerHTML = "哈哈";
4、通过标签名称
var divs = document.getElementsByTagName("div");
console.info(divs)
5、通过name属性来获取对象
// 注意:该api主要用来获取表单对象
var unames = document.getElementsByName("username");
console.info(unames)
操作DOM对象的内容:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
</head>
<body>
<div id="box">div标签<span>这个是span中的内容</span></div>
<script type="text/javascript">
var box = document.getElementById("box");
console.info(box)
// innerHTML 获取DOM对象的子标签及文本内容
console.info(box.innerHTML)
box.innerHTML = "<b style='color: royalblue;'>我是李聪聪</b>"
// 只获取文本内容,包括子标签的文本内容,但是
// 注意:不要去子标签本身
console.info(box.innerText);
box.innerText = "licongcong"
box.innerText = "<b style='color: royalblue;'>licongcongcongcong</b>"
box.textContent = "<b style='color: royalblue;'>javaWeb</b>";
</script>
</body>
</html>
操作DOM对象的样式
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title></title>
<style type="text/css">
.show {
width: 200px;
}
.box {
width: 200px;
height: 200px;
background: red;
border-radius: 50%;
border: 1px solid red;
}
</style>
</head>
<body>
<div id="show" class="show" style="height: 50px;"></div>
<div id="box" class="box" onclick="run()"></div>
<script>
var div = document.getElementById("show");
// DOM对象.style.样式名称只能拿到标签样式
var h = show.style.height;
console.info(h)
console.info(typeof(h))
var w = show.style.width;
console.info(w)
// 使用getComputedStyle才能拿到任意的样式值
let w2 = getComputedStyle(div).width;
console.info(w2)
function run() {
var box = document.getElementById("box");
var h = getComputedStyle(box).height;
var w = getComputedStyle(box).width;
// 去掉单位
h = parseInt(h);
w = parseInt(w);
box.style.width = h + 10 + "px";
box.style.height = w + 10 + "px";
// JavaScript专门提供用来操作宽和高的api,获取是没有单位的数值
var h = box.offsetHeight;
var w = box.clientWidth;
box.style.width = h + 10 + "px";
box.style.height = w + 10 + "px";
}
</script>
</body>
</html>
————————————————————————————————————————————————————————————