简介
动画有下面两种情况
同一个图形通过视图在界面上进行透明度,缩放,旋转,平移的变化(View动画)
在界面的同一个位置上不断切换显示不同的图片(Drawable动画)
动画的分类
View Animation
Drawable Animation
Android中提供了两种实现动画的方式
纯编码的方式
Xml配置的方式
View Animation
Animation公用的方法和属性
setDuration(long durationMillis) : 设置持续时间(单位ms)
setStartOffset(long startOffset) : 设置开始的延迟的时间(单位ms)
setFillBefore(boolean fillBefore) : 设置最终是否固定在起始状态
setFillAfter(boolean fillAfter) : 设置最终是否固定在最后的状态
setAnimationListener(AnimationListener listener) : 设置动画监听
坐标类型:
Animation.ABSOLUTE :这个是绝对坐标,就是直接写坐标值
Animation.RELATIVE_TO_SELF :这个是相对坐标,而且是相对自己的,以自己的左上顶点为参考点
Animation.RELATIVE_TO_PARENT "这个是相对父节点的左上顶点为参考点
启动动画 : view.startAnimation(animation);
结束动画: view.clearAnimation()
动画监听器 : AnimationListener
onAnimationStart(Animation animation) : 动画开始的回调
onAnimationEnd(Animation animation) : 动画结束的回调
onAnimationRepeat(Animation animation) : 动画重复执行
分类
单一动画(Animation)
缩放动画(ScaleAnimation)
透明度动画(AlphaAnimation)
旋转动画(RotateAnimation)
平移动画(TranslateAnimation)
复合动画(AnimationSet)
由多个单一动画组合在一起的动画
从下面的关系可以看出Animation和AnimationSet的关系就好像之前
View和Viewgroup的关系一样
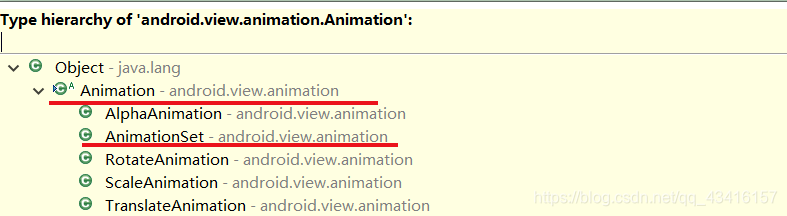
ScaleAnimation
编码方式
public ScaleAnimation(float fromX, float toX, float fromY, float toY,
int pivotXType, float pivotXValue, int pivotYType, float pivotYValue)
fromX : 开始时X轴上的缩放比例
toX : 结束时X轴上的缩放比例
fromY :开始时Y轴上的缩放比例
toY :结束时Y轴上的缩放比例
pivotXType : X轴坐标的类型(计算x轴上的偏移量的方式)
pivotXVlaue : 中心点在X轴相对视图左顶点在x轴上的偏移量
pivotYType : Y轴坐标的类型(计算x轴上的偏移量的方式)
pivotYValue : 中心点相对视图左顶点在y轴上的偏移量
/*
* 编码实现: 缩放动画
* ScaleAnimation
* //1. 创建动画对象
* //2. 设置
* //3. 启动动画
*/
public void startCodeScale(View v)
{
tv_animation_msg.setText("Code缩放动画: 宽度从0.5到1.5, 高度从0.0到1.0, "
+ "缩放的圆心为顶部中心点,延迟1s开始,持续2s,最终还原");
// 1. 创建动画对象
ScaleAnimation animation = new ScaleAnimation(0.5f, 1.5f, 0, 1,
Animation.ABSOLUTE, iv_animation.getWidth() / 2,Animation.ABSOLUTE, 0);
animation = new ScaleAnimation(0.5f, 1.5f, 0, 1,
Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0);
// 2. 设置
// 延迟1s开始
animation.setStartOffset(1000);
// 持续2s
animation.setDuration(2000);
// 最终还原
animation.setFillBefore(true);
// 3. 启动动画
iv_animation.startAnimation(animation);
}
文件方式
Animation.ABSOLUTE :
数值(默认以px为单位) 100
Animation.RELATIVE_TO_SELF :
百分数,如:50% (以当前视图的宽度或高度其为基数来计算)
Animation.RELATIVE_TO_PARENT :
百分数+p,如:50%p (以父视图的宽度或高度其为基数来计算)
<?xml version="1.0" encoding="utf-8"?>
<scale xmlns:android="http://schemas.android.com/apk/res/android"
android:fromXScale="0"
android:fromYScale="0"
android:toXScale="1.5"
android:toYScale="1"
android:startOffset="1000"
android:duration="3000"
android:pivotX="100%"
android:pivotY="100%"
android:fillAfter="true">
</scale>
/*
* xml实现: 缩放动画
* <scale>
*/
/*
1. 定义动画文件
2. 加载动画文件得到动画对象
3. 启动动画
*/
public void startXmlScale(View v)
{
tv_animation_msg.setText("Xml缩放动画: Xml缩放动画: "
+ "宽度从0.0到1.5, 高度从0.0到1.0, 延迟1s开始,持续3s,圆心为右下角, 最终固定");
// 1. 定义动画文件
// 2. 加载动画文件得到动画对象
Animation animation = AnimationUtils.loadAnimation(this,R.anim.scale_test);
// 3. 启动动画
iv_animation.startAnimation(animation);
}
AlphaAnimation
编码方式
public RotateAnimation(float fromDegrees, float toDegrees, int pivotXType, float pivotXValue,
int pivotYType, float pivotYValue)
fromDegrees : 开始时的角度
toDegrees : 结束时的角度
pivotXType : X轴坐标的类型
pivotXVlaue : X轴坐标的值
pivotYType : Y轴坐标的类型
pivotYValue : Y轴坐标的值
/*
* 编码实现: 旋转动画 RotateAnimation
*/
public void startCodeRotate(View v)
{
tv_animation_msg.setText("Code旋转动画: 以图片中心点为中心, 从负90度到正90度, 持续5s");
// 1. 创建动画对象
RotateAnimation animation = new RotateAnimation(-90, 90,
Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF,0.5f);
// 2. 设置
animation.setDuration(5000);
// 3. 启动动画
iv_animation.startAnimation(animation);
}
文件方式
<?xml version="1.0" encoding="utf-8"?>
<rotate xmlns:android="http://schemas.android.com/apk/res/android"
android:fromDegrees="90"
android:toDegrees="-90"
android:duration="5000">
</rotate>
/*
* xml实现: 旋转动画
* <rotate>
*/
public void startXmlRotate(View v)
{
tv_animation_msg.setText("Xml旋转动画: 以左顶点为坐标, 从正90度到负90度, 持续5s");
// 1. 定义动画文件
// 2. 加载动画文件得到动画对象
Animation animation = AnimationUtils.loadAnimation(this,R.anim.rotate_test);
// 3. 启动动画
iv_animation.startAnimation(animation);
}
RotateAnimation
编码方式
/*
* 编码实现: 透明度动画
* 完全透明 : 0
* 完全不透明 : 1
* AlphaAnimation
*/
public void startCodeAlpha(View v)
{
tv_animation_msg.setText("Code透明度动画: 从完全透明到完全不透明, 持续2s");
// 1. 创建动画对象
AlphaAnimation animation = new AlphaAnimation(0, 1);
// 2. 设置
animation.setDuration(4000);
// 3. 启动动画
iv_animation.startAnimation(animation);
}
文件方式
<?xml version="1.0" encoding="utf-8"?>
<alpha xmlns:android="http://schemas.android.com/apk/res/android"
android:fromAlpha="1"
android:toAlpha="0"
android:duration="4000">
</alpha>
/*
* xml实现: 透明度动画
* <alpha>
*/
public void startXmlAlpha(View v)
{
tv_animation_msg.setText("Xml透明度动画: 从完全不透明到完全透明, 持续4s");
// 1. 定义动画文件
// 2. 加载动画文件得到动画对象
Animation animation = AnimationUtils.loadAnimation(this,
R.anim.alpha_test);
animation.setFillAfter(true);
// 3. 启动动画
iv_animation.startAnimation(animation);
}
TranslateAnimation
编码方式
/*
* 编码实现: 平移动画 TranslateAnimation
*/
public void startCodeTranslate(View v)
{
tv_animation_msg.setText("Code移动动画: 向右移动一个自己的宽度, 向下移动一个自己的高度, 持续2s");
// 1. 创建动画对象
TranslateAnimation animation = new TranslateAnimation(
Animation.ABSOLUTE, 0, Animation.RELATIVE_TO_SELF, 1,
Animation.ABSOLUTE, 0, Animation.RELATIVE_TO_SELF, 1);
// 2. 设置
animation.setDuration(2000);
// 3. 启动动画
iv_animation.startAnimation(animation);
}
文件方式
<?xml version="1.0" encoding="utf-8"?>
<translate
xmlns:android="http://schemas.android.com/apk/res/android"
android:fromXDelta="100%p"
android:toXDelta="0"
android:fromYDelta="0"
android:toYDelta="0"
android:duration="2000">
</translate>
/*
* xml实现: 平移动画
* <translate>
*/
public void startXmlTranslate(View v)
{
tv_animation_msg.setText("xml移动动画: 从屏幕的右边逐渐回到原来的位置, 持续2s");
// ***此效果用于界面切换的动画效果
// 1. 定义动画文件
// 2. 加载动画文件得到动画对象
Animation animation = AnimationUtils.loadAnimation(this,
R.anim.translate_test);
// 3. 启动动画
iv_animation.startAnimation(animation);
}
Code Animation
编码方式
/*
* 编码实现: 复合动画 AnimationSet
*/
public void startCodeAnimationSet(View v)
{
tv_animation_msg.setText("Code复合动画: 透明度从透明到不透明, 持续2s, "
+ "接着进行旋转360度的动画, 持续1s");
// 1. 创建透明动画并设置
AlphaAnimation alphaAnimation = new AlphaAnimation(0, 1);
alphaAnimation.setDuration(2000);
// 2. 创建旋转动画并设置
RotateAnimation rotateAnimation = new RotateAnimation(0, 360,
Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF,
0.5f);
rotateAnimation.setDuration(1000);
rotateAnimation.setStartOffset(2000);// 延迟
// 3. 创建复合动画对象
AnimationSet animationSet = new AnimationSet(true);
// 4. 添加两个动画
animationSet.addAnimation(alphaAnimation);
animationSet.addAnimation(rotateAnimation);
// 5. 启动复合动画对象
iv_animation.startAnimation(animationSet);
}
文件方式
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" >
<alpha
android:duration="2000"
android:fromAlpha="0"
android:toAlpha="1" >
</alpha>
<rotate
android:duration="2000"
android:fromDegrees="0"
android:startOffset="2000"
android:toDegrees="360" />
</set>
/*
* xml实现: 复合动画
* <set>
*/
public void startXmlAnimationSet(View v) {
tv_animation_msg.setText("Xml复合动画: 透明度从透明到不透明, 持续2s, 接着进行旋转360度的动画, 持续2s");
//1. 定义动画文件
//2. 加载动画文件得到动画对象
Animation animation = AnimationUtils.loadAnimation(this, R.anim.set_test);
//3. 启动动画
iv_animation.startAnimation(animation);
}
动画监听
在程序中可以对动画的特定时刻进行监听
Animation.setAnimationListener(AnimationListener listener) : 设置动画监听
动画监听器 : AnimationListener
onAnimationStart(Animation animation) : 动画开始的回调
onAnimationEnd(Animation animation) : 动画结束的回调
onAnimationRepeat(Animation animation) : 动画重复执行
Interpolator属性
Interpolator 被用来修饰动画效果,定义动画的变化率,
可以使存在的动画效果accelerated(加速),decelerated(减速),repeated(重复)等。
@android:anim/linear_interpolator : 线性变化
@android:anim/accelerate_interpolator : 加速变化
@android:anim/decelerate_interpolator : 减速变化
@android:anim/cycle_interpolator : 周期循环变化
Drawable Animation
首先新建drawable文件夹下面的xml文件
<?xml version="1.0" encoding="utf-8"?>
<animation-list xmlns:android="http://schemas.android.com/apk/res/android"
android:oneshot="false">
<item
android:drawable="@drawable/nv1"
android:duration="500"/>
<item
android:drawable="@drawable/nv2"
android:duration="500"/>
<item
android:drawable="@drawable/nv3"
android:duration="500"/>
<item
android:drawable="@drawable/nv4"
android:duration="500">
</item>
</animation-list>
然后在图片视图里面引用这个文件
<ImageView
android:id="@+id/iv_da_mm"
android:layout_width="80dp"
android:layout_height="80dp"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="160dp"
android:background="@drawable/drawable_animation_test"/>
然后还要得到背景动画图片对象来进行启动和停掉
@Override
public void onClick(View v)
{
if (v == btn_da_start)
{
if (animationDrawable == null)
{
// 得到背景动画图片对象
animationDrawable = (AnimationDrawable) iv_da_mm.getBackground();
// 启动
animationDrawable.start();
}
} else if (v == btn_da_stop)
{
if (animationDrawable != null)
{
animationDrawable.stop();
animationDrawable = null;
}
}
}