LeetCode:235. 二叉搜索树的最近公共祖先
解决方案:
1.思路
- 对于当前节点x,如果x比p和q的值都大,说明,p和q在x的右子树里面,那么去x的右子树里面去寻找;
- 对于当前节点x,如果x比p和q的值都小,说明,p和q在x的左子树里面,那么去x的左子树里面去寻找;
2.代码实现
class Solution {
public TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) {
TreeNode ancestor = root;
while (true) {
if (p.val < ancestor.val && q.val < ancestor.val) {
ancestor = ancestor.left;
} else if (p.val > ancestor.val && q.val > ancestor.val) {
ancestor = ancestor.right;
} else {
break;
}
}
return ancestor;
}
}
class Solution {
public TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) {
if(root.val>p.val && root.val>q.val){
return lowestCommonAncestor(root.left, p, q);
}
if(root.val<q.val && root.val<p.val){
return lowestCommonAncestor(root.right, p, q);
}
else{return root;}
}
}
3.复杂度分析
- 非递归
- 时间复杂度为
O
(
n
)
O(n)
O(n);
- 空间复杂度为
O
(
1
)
O(1)
O(1);
- 递归
- 时间复杂度为
O
(
n
)
O(n)
O(n);
- 空间复杂度为
O
(
n
)
O(n)
O(n);
5.疑问
- 问:该题有必要使用递归吗?
- 答:没有必要,浪费空间复杂度;
LeetCode:701.二叉搜索树中的插入操作
解决方案:
1.思路:
2.代码实现
class Solution {
public TreeNode insertIntoBST(TreeNode root, int val) {
if (root == null) {
return new TreeNode(val);
}
TreeNode pos = root;
while (pos != null) {
if (val < pos.val) {
if (pos.left == null) {
pos.left = new TreeNode(val);
break;
} else {
pos = pos.left;
}
} else {
if (pos.right == null) {
pos.right = new TreeNode(val);
break;
} else {
pos = pos.right;
}
}
}
return root;
}
}
3.复杂度分析
- 时间复杂度为
O
(
n
)
O(n)
O(n);
- 空间复杂度为
O
(
1
)
O(1)
O(1);
LeetCode:450.删除二叉搜索树中的节点
问题描述
解决方案:
1.思路:
2.代码实现
class Solution {
public TreeNode deleteNode(TreeNode root, int key) {
if (root == null) {
return null;
}
if (root.val > key) {
root.left = deleteNode(root.left, key);
return root;
}
if (root.val < key) {
root.right = deleteNode(root.right, key);
return root;
}
if (root.val == key) {
if (root.left == null && root.right == null) {
return null;
}
if (root.right == null) {
return root.left;
}
if (root.left == null) {
return root.right;
}
TreeNode successor = root.right;
while (successor.left != null) {
successor = successor.left;
}
root.right = deleteNode(root.right, successor.val);
successor.right = root.right;
successor.left = root.left;
return successor;
}
return root;
}
}
3.复杂度分析
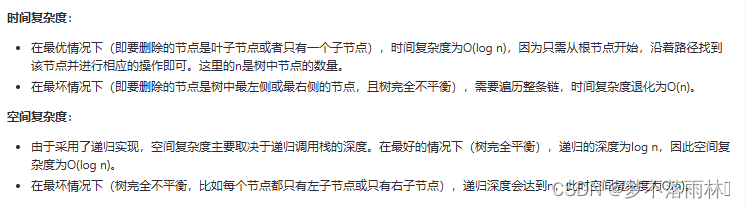