输入样例:
1248009005003600700
0
输出样例:
123456789
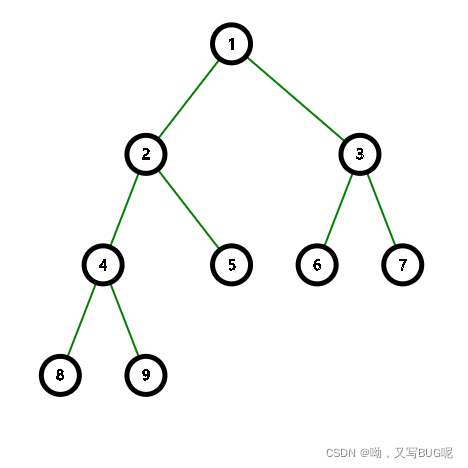
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define MAX_SIZE 100
#define ElemType BiTree
typedef struct BiTNode {
char data;
struct BiTNode *left, *right;
} BiTNode, *BiTree;
typedef struct SqQueue {
ElemType data[MAX_SIZE];
int front, rear;
} SqQueue;
void CreateBiTree(BiTree *T, char *str, int *cursor);
void DestroyBiTree(BiTree T);
void LevelOrderTraverseBiTree(BiTree T);
void InitQueue(SqQueue *Q);
bool IsEmptyQueue(SqQueue Q);
void EnQueue(SqQueue *Q, ElemType e);
void DeQueue(SqQueue *Q, ElemType *e);
int main() {
char str[MAX_SIZE];
while (scanf("%s", str) && str[0] != 48) {
BiTree T = NULL;
int cursor = 0;
CreateBiTree(&T, str, &cursor);
printf("\n");
LevelOrderTraverseBiTree(T);
DestroyBiTree(T);
}
return 0;
}
void CreateBiTree(BiTree *T, char *str, int *cursor) {
if (str[*cursor] == 48) {
*T = NULL;
} else {
*T = (BiTree) malloc(sizeof(BiTNode));
(*T)->data = str[*cursor];
(*cursor)++;
CreateBiTree(&((*T)->left), str, cursor);
(*cursor)++;
CreateBiTree(&((*T)->right), str, cursor);
}
}
void DestroyBiTree(BiTree T) {
if (T) {
DestroyBiTree(T->left);
DestroyBiTree(T->right);
free(T);
}
}
void LevelOrderTraverseBiTree(BiTree T) {
BiTree now_node;
SqQueue Q;
InitQueue(&Q);
EnQueue(&Q, T);
while (!IsEmptyQueue(Q)) {
DeQueue(&Q, &now_node);
printf("%c", now_node->data);
if (now_node->left) {
EnQueue(&Q, now_node->left);
}
if (now_node->right) {
EnQueue(&Q, now_node->right);
}
}
}
void InitQueue(SqQueue *Q) {
Q->front = Q->rear = 0;
}
bool IsEmptyQueue(SqQueue Q) {
return (Q.front == Q.rear) ? true : false;
}
void EnQueue(SqQueue *Q, ElemType e) {
if ((Q->rear + 1) % MAX_SIZE == Q->front) {
printf("full\n");
return;
}
Q->data[Q->rear] = e;
Q->rear = (Q->rear + 1) % MAX_SIZE;
}
void DeQueue(SqQueue *Q, ElemType *e) {
if (Q->rear == Q->front) {
printf("empty\n");
return;
}
*e = Q->data[Q->front];
Q->front = (Q->front + 1) % MAX_SIZE;
}