<!-- 地图组件 -->
<template>
<div :id="id" class="container"></div>
</template>
<script>
import _ from "lodash";
import "maptalks/dist/maptalks.css";
import * as maptalks from "maptalks";
export default {
//import引入的组件需要注入到对象中才能使用
name: "Map",
props: {
id: {
type: String,
default: () => {
return "map";
},
},
mapSet: {
type: Object,
default: () => {
return {};
},
},
color: {
type: String,
default: () => {
return "";
},
},
},
components: {},
data() {
//这里存放数据
return {
dataLis: [],
layer: null,
layer2: null,
map: null,
polygon: null,
drawTool: null,
// 默认中心点点位
center: [121.7294793802647, 29.97249795779439],
// 缩放层级
zoom: 14,
// 倾斜度
pitch: 0,
// 轴承
// bearing: 90,
// 最小缩放层级
minZoom: 1,
// 最大缩放层级
maxZoom: 18.5,
symbol: {
polygonFill: "#ff0000", // 图形覆盖颜色
polygonOpacity: 0.3, // 图形覆盖区域透明度
lineColor: "#ff0000", // 线条颜色
lineWidth: 2, // 线条宽度
},
pointSymbol: {
markerFile: "@/assets/logo/logo.png",
markerWidth: 20,
markerHeight: 20,
},
layerSwitcherControl: {
position: "top-right",
// title of base layers
baseTitle: "Base Layers",
// title of layers
overlayTitle: "Layers",
// layers you don't want to manage with layer switcher
excludeLayers: [],
// css class of container element, maptalks-layer-switcher by default
containerClass: "maptalks-layer-switcher",
},
drawMap: {
Point: "点",
LineString: "线",
Polygon: "多边形",
Circle: "圆",
Ellipse: "椭圆",
Rectangle: "长方形",
},
};
},
//监听属性 类似于data概念
computed: {},
//监控data中的数据变化
watch: {
mapSet: {
deep: true,
immediate: true,
async handler(val) {
if (Object.keys(val).length) {
let { drawMapType, drawMapArray, editable, draggable } = val;
if (this.map) this.map.remove();
await this.init();
if (drawMapArray && drawMapArray.length > 0) {
// console.log("地图获取到所有点位", drawMapArray);
drawMapArray.map(i => {
// console.log(123, i[0]);
let items = i[0]
let obj = {
drawMapArray: () => {
this.startEdit(items, {
drawMapType, //点位
editable: true,//可编辑的
draggable: true, //拖动
drawMapArray: items //数据
});
},
drawMapType: () => {
this.drawMapType({
drawMapType, //点位
editable: true,//可编辑的
draggable: true, //拖动
drawMapArray: items //数据
});
},
};
let arrayKeys = Object.keys({
drawMapType, //点位
editable: true,//可编辑的
draggable: true, //拖动
drawMapArray: items //数据
});
arrayKeys.map((items) => {
if (obj[items]) return obj[items]();
});
})
// let items2 = [121.720448, 29.978434]
// console.log(9992, items2);
// let obj2 = {
// drawMapArray: () => {
// this.startEdit(items2, {
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: items2 //数据
// });
// },
// drawMapType: () => {
// this.drawMapType({
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: items2 //数据
// });
// },
// };
// let arrayKeys2 = Object.keys({
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: items2 //数据
// });
// arrayKeys2.map((items2) => {
// if (obj2[items2]) return obj2[items2]();
// });
// 出现
// this.drawMapType({
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: undefined //数据
// });
}
this.drawMapType({
drawMapType, //点位
editable: true,//可编辑的
draggable: true, //拖动
drawMapArray: undefined //数据
});
// if (drawMapArray && drawMapArray.length > 0) {
// drawMapArray.forEach((item, indexs) => {
// console.log(999, item);
// let obj = {
// drawMapArray: () => {
// this.startEdit(item, {
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: item //数据
// });
// },
// drawMapType: () => {
// this.drawMapType({
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: item //数据
// });
// },
// };
// let arrayKeys = Object.keys({
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: item //数据
// });
// arrayKeys.map((item) => {
// if (obj[item]) return obj[item]();
// });
// })
// } else {
// let obj = {
// // 绘制图形
// drawMapType: () => {
// this.drawMapType({
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: item //数据
// });
// },
// };
// let arrayKeys = Object.keys({
// drawMapType, //点位
// editable: true,//可编辑的
// draggable: true, //拖动
// drawMapArray: item //数据
// });
// arrayKeys.map((item) => {
// if (obj[item]) return obj[item]();
// });
// }
// ----------------
// // 循环插入多边形
// if (drawMapArray && drawMapArray.length > 0) {
// drawMapArray.forEach(item => {
// let obj = {
// // 编辑图形
// drawMapArray: () => {
// this.startEdit(item, {
// drawMapType,
// editable,
// draggable,
// drawMapArray: item
// });
// },
// // 绘制图形
// drawMapType: () => {
// this.drawMapType({
// drawMapType,
// editable,
// draggable,
// drawMapArray: item
// });
// },
// };
// let arrayKeys = Object.keys({
// drawMapType,
// editable,
// draggable,
// drawMapArray: item
// });
// arrayKeys.map((item) => {
// if (obj[item]) return obj[item]();
// });
// })
// } else {
// let obj = {
// // 编辑图形
// // drawMapArray: () => {
// // this.startEdit(item, {
// // drawMapType,
// // editable,
// // draggable,
// // drawMapArray
// // });
// // },
// // 绘制图形
// drawMapType: () => {
// this.drawMapType({
// drawMapType,
// editable,
// draggable,
// drawMapArray
// });
// },
// };
// let arrayKeys = Object.keys({
// drawMapType,
// editable,
// draggable,
// drawMapArray
// });
// arrayKeys.map((item) => {
// if (obj[item]) return obj[item]();
// });
// }
} else {
// console.log("销毁地图");
if (this.map != undefined) this.map.remove();
}
},
},
color: {
immediate: true,
handler(val) {
if (val) {
let { drawTool, polygon } = this;
if (drawTool) this.changeSymbol("drawTool", drawTool, val);
if (polygon) this.changeSymbol("Point", polygon, val);
// if (drawTool) {
// drawTool.setSymbol({
// polygonFill: val, // 图形覆盖颜色
// polygonOpacity: 0.3, // 图形覆盖区域透明度
// lineColor: val, // 线条颜色
// lineWidth: 2 // 线条宽度
// });
// }
// if (polygon) {
// polygon.updateSymbol({
// polygonFill: val, // 图形覆盖颜色
// lineColor: val // 线条颜色
// });
// }
}
},
},
},
//生命周期 - 创建完成(可以访问当前this实例)
created() { },
//生命周期 - 挂载完成(可以访问DOM元素)
mounted() {
// let a = "rgb(255, 69, 0)";
// console.log(a.slice(5, a.length - 1));
},
// beforeDestroy() {
// let { map } = this;
// // console.log(
// // "🚀 ~ Date:2023/07/11 14:37 ~ File: index.vue ~ Line:154 ----- ",
// // 销毁地图
// // );
// if (map) map.remove();
// },
//方法集合
methods: {
// 获取到图层所有的点位
extractCoordinates(data) {
// console.log("获取到图层所有的点位", data);
let datas = []
data.forEach(res => {
datas.push({
"lngWgs": res._coordinates.x,
"latWgs": res._coordinates.y
})
})
// console.log(111111, datas);
return datas;
},
// getSent(data) {
// let array = []
// data.forEach(res => {
// let arrray2 = []
// res._coordinates.forEach(res2 => {
// arrray2.push({
// "lngWgs": res2.x,
// "latWgs": res2.y,
// // "sort": index2
// })
// })
// array.push(arrray2)
// })
// return array;
// },
init() {
this.$nextTick(() => {
let { center, zoom, pitch, minZoom, maxZoom, symbol, layerSwitcherControl, id } = this;
this.map = new maptalks.Map(id, {
center,
zoom,
pitch,
minZoom,
maxZoom,
centerCross: true,
seamlessZoom: true,
attribution: true,
zoomControl: true, // add zoom control
scaleControl: true, // add scale control
layerSwitcherControl,
symbol,
// baseLayer 表示基础图层,它可以添加多个,逗号隔开
baseLayer: new maptalks.GroupTileLayer("Base TileLayer", [
new maptalks.TileLayer("卫星图", {
urlTemplate:
"https://t5.tianditu.gov.cn/DataServer?T=img_w&X={x}&Y={y}&L={z}&tk=074d4a21c8f34a3a20cd1f69f81b26bf",
subdomains: ["a", "b", "c", "d"],
}),
new maptalks.TileLayer("Carto light", {
visible: false,
urlTemplate:
"https://{s}.basemaps.cartocdn.com/light_all/{z}/{x}/{y}.png",
subdomains: ["a", "b", "c", "d"],
}),
new maptalks.TileLayer("Carto dark", {
visible: false,
urlTemplate:
"https://{s}.basemaps.cartocdn.com/dark_all/{z}/{x}/{y}.png",
subdomains: ["a", "b", "c", "d"],
}),
new maptalks.WMSTileLayer("survey", {
urlTemplate: "http://10.169.61.30:8080/geoserver/gwc/service/wms",
crs: "EPSG:3857",
layers: "haigang",
styles: "",
version: "1.1.1",
tileStackDepth: 0,
format: "image/png",
transparent: true,
uppercase: true,
zIndex: 1,
forceRenderOnMoving: true,
forceRenderOnZooming: true,
}),
]),
});
// this.layer = new maptalks.VectorLayer("vector").addTo(this.map)
this.layer = new maptalks.VectorLayer("vector").addTo(this.map)
this.layer2 = new maptalks.VectorLayer("vector2").addTo(this.map)
this.map.on("click", function (params) {
});
this.map.on("editrecord", function (params) {
// console.log(params);
});
});
},
// 绘制图形
drawMapType(val) {
// !this.layer ? this.layer = new maptalks.VectorLayer("vector").addTo(this.map) : null
// console.log('绘制图形', val, this.map);
let { drawMapType, drawMapArray } = val;
if (!drawMapArray) {
let { map, symbol, color } = this;
let that = this;
// var layer = new maptalks.VectorLayer("vector").addTo(map);
var drawTool = new maptalks.DrawTool({
mode: drawMapType,
// once: true,
// once: false,
symbol: drawMapType == "Point" ? null : symbol,
})
.addTo(map)
.disable();
drawTool.on("drawend", function (param) {
// console.log(param.geometry);
let { _coordinates } = param.geometry;
let array = null;
if (drawMapType == "Point") {
array = [_coordinates.x, _coordinates.y];
} else if (drawMapType == "Polygon") {
array = _coordinates.map((item) => {
return [item.x, item.y];
});
}
that.dataLis.push(array)
// console.log(123, array);
that.layer.addGeometry(param.geometry);
that.startEdit(array, val, that.dataLis.length - 1);
that.layer.remove();
that.layer.setZIndex(6);
// that.$nextTick(() => {
// console.log("绘制好了 发送", that.getSent(that.layer2._geoList));
// that.$emit("drawPointChange", that.getSent(that.layer2._geoList));
// })
// console.log(666666, that.extractCoordinates(that.layer._geoList));
that.$emit("drawPointChange", that.extractCoordinates(that.layer._geoList));
// console.log(666666, that.layer._geoList);
});
this.drawTool = drawTool;
drawTool.setMode(drawMapType).enable();
// 改变覆盖物设置
if (color) this.changeSymbol("drawTool", drawTool, color);
// if (color) {
// drawTool.setSymbol({
// polygonFill: color, // 图形覆盖颜色
// polygonOpacity: 0.3, // 图形覆盖区域透明度
// lineColor: color, // 线条颜色
// lineWidth: 2 // 线条宽度
// });
// }
}
},
// 编辑绘制图形
startEdit(array, val) {
// let nun = Math.random();
// console.log("编辑绘制图形", array, val);
let that = this;
let { drawMapType, editable, draggable } = val;
let { symbol, map, pointSymbol, color } = that;
let type = null;
let pointArray = _.clone(array);
// that.$emit("drawPointChange", pointArray);
that.$emit("drawPointChange", that.extractCoordinates(that.layer._geoList));
let objArray = [
[() => drawMapType == "Point",
() => {
type = "Marker";
},
() => {
// 更改位置
polygon.on("shapechange", function (param) {
console.log(110, param, that.extractCoordinates(that.layer2._geoList));
// that.extractCoordinates(that.layer._geoList)
// let { _coordinates } = param.target;
// pointArray = [_coordinates.x, _coordinates.y];
// that.$emit("drawPointChange", pointArray);
that.$emit("drawPointChange", that.extractCoordinates(that.layer2._geoList));
});
// 更改位置
polygon.on("positionchange", function (param) {
console.log(110, param, that.extractCoordinates(that.layer2._geoList));
// that.extractCoordinates(that.layer._geoList)
// let { _coordinates } = param.target;
// pointArray = [_coordinates.x, _coordinates.y];
// that.$emit("drawPointChange", pointArray);
that.$emit("drawPointChange", that.extractCoordinates(that.layer2._geoList));
});
// 更改位置
polygon.on("remove", function (param) {
console.log(110, param, that.extractCoordinates(that.layer2._geoList));
// that.extractCoordinates(that.layer._geoList)
// let { _coordinates } = param.target;
// pointArray = [_coordinates.x, _coordinates.y];
// that.$emit("drawPointChange", pointArray);
that.$emit("drawPointChange", that.extractCoordinates(that.layer2._geoList));
});
},
]
];
let state = objArray.find((m) => m[0]());
if (state) state[1]();
let polygon = new maptalks[type](array, {
visible: true,
editable,
cursor: "pointer",
draggable,
dragShadow: false,
drawOnAxis: null,
symbol: drawMapType == "Point" ? null : symbol,
});
var options = {
'items': [
{
item: '删除', click: function (e) {
that.layer2.removeGeometry(polygon);
}
}
]
};
polygon.setMenu(options).openMenu();
// new maptalks.VectorLayer("vectorEdit" + nun, polygon).addTo(map);
that.layer2.addGeometry(polygon);
// that.layer.addGeometry(polygon);
map.fitExtent(polygon.getExtent(), -1); // 自动适配区域
if (color) this.changeSymbol("Point", polygon, color);
if (state) state[2]();
that.polygon = polygon;
},
// 覆盖物设置更改
changeSymbol(type, mapObj, color) {
let obj = {
drawTool: () => {
mapObj.setSymbol({
polygonFill: color, // 图形覆盖颜色
polygonOpacity: 0.3, // 图形覆盖区域透明度
lineColor: color, // 线条颜色
lineWidth: 2, // 线条宽度
});
},
polygon: () => {
mapObj.updateSymbol({
polygonFill: color, // 图形覆盖颜色
lineColor: color, // 线条颜色
});
},
};
if (obj[type]) return obj[type]();
},
},
};
</script>
<style scoped>
.container {
width: calc(100% - 50px);
/* width: 550px; */
min-height: 550px;
margin: 15px;
}
</style>
maptalks 右键删除坐标点位
最新推荐文章于 2024-05-13 01:39:01 发布
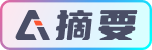