- 单例设计模式是在类中预先实例化一个static对象,并将类的构造器设置为private,以保证该类在程序运行过程中只存在一个对象,调用该对象时通过static方法获取该对象。
- 单例设计模式分为饿汉式和懒汉式。
- 饿汉式是当类被加载时就实例化对象,优点是线程安全的,缺点是对象长时间占用内存。
- 懒汉式是当获取对象时才实例化对象,优点是对象占用内存的时间短,但需要利用同步代码块使其变成线程安全的。
public class InstanceDesignModel {
public static void main(String[] args) {
Test1 test11 = Test1.getInstance();
test11.setValue(1);
System.out.println("test11.value = " + test11.getValue());
Test1 test12 = Test1.getInstance();
test12.setValue(2);
System.out.println("test11.value = " + test11.getValue());
Test2 test21 = Test2.getInstance();
test21.setValue(1);
System.out.println("test21.value = " + test21.getValue());
Test2 test22 = Test2.getInstance();
test22.setValue(2);
System.out.println("test21.value = " + test21.getValue());
}
}
class Test1 {
private static Test1 instance = new Test1();
private int value;
private Test1() {
System.out.println("-----饿汉式单例-----");
}
public static Test1 getInstance() {
return instance;
}
public int getValue() {
return value;
}
public void setValue(int value) {
this.value = value;
}
}
class Test2 {
private static Test2 instance = null;
private int value;
private Test2() {
System.out.println("-----懒汉式单例-----");
}
public static Test2 getInstance() {
if (instance == null) {
synchronized (Test2.class) {
if (instance == null) {
instance = new Test2();
}
}
}
return instance;
}
public int getValue() {
return value;
}
public void setValue(int value) {
this.value = value;
}
}
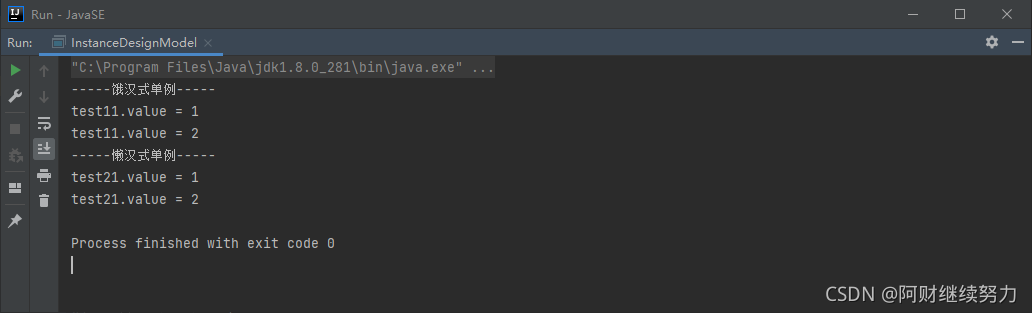