1. 题目
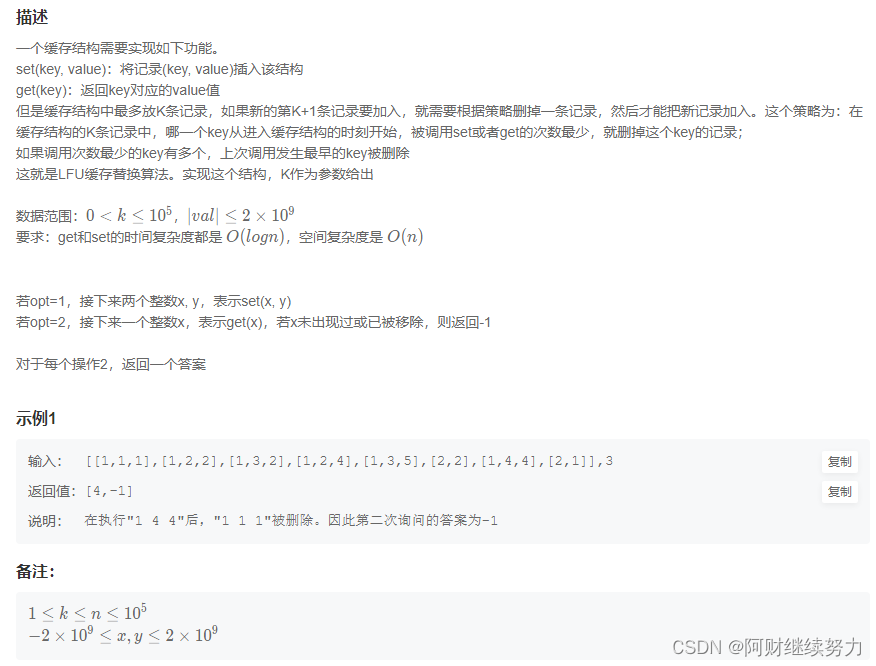
2. 思路
(1) 哈希表+优先队列
- 哈希表count存储key和调用该key的次数和时间,哈希表data存储真正的数据,优先队列存储key,按照调用次数从小到大排序,调用次数相等时,按照调用时间从小到大排序。
3. 代码
import java.util.ArrayList;
import java.util.HashMap;
import java.util.PriorityQueue;
public class Solution {
class Node {
public int count;
public int time;
public Node(int count, int time) {
this.count = count;
this.time = time;
}
}
public int[] LFU(int[][] operators, int k) {
HashMap<Integer, Node> count = new HashMap<>();
HashMap<Integer, Integer> data = new HashMap<>();
PriorityQueue<Integer> minHeap = new PriorityQueue<>((o1, o2) -> Integer.compare(count.get(o1).count, count.get(o2).count) == 0 ?
Integer.compare(count.get(o1).time, count.get(o2).time) : Integer.compare(count.get(o1).count, count.get(o2).count));
ArrayList<Integer> list = new ArrayList<>();
int time = 0;
for (int[] operator : operators) {
if (operator[0] == 1) {
int key = operator[1];
int value = operator[2];
if (data.size() == k) {
int removeKey = minHeap.poll();
count.remove(removeKey);
data.remove(removeKey);
}
data.put(key, value);
if (count.containsKey(key)) {
Node node = new Node(count.get(key).count + 1, time++);
count.put(key, node);
minHeap.remove(key);
minHeap.offer(key);
} else {
Node node = new Node(1, time++);
count.put(key, node);
minHeap.offer(key);
}
} else {
int key = operator[1];
if (data.containsKey(key)) {
list.add(data.get(key));
if (count.containsKey(key)) {
Node node = new Node(count.get(key).count + 1, time++);
count.put(key, node);
minHeap.remove(key);
minHeap.offer(key);
} else {
Node node = new Node(1, time++);
count.put(key, node);
minHeap.offer(key);
}
} else {
list.add(-1);
}
}
}
int n = list.size();
int[] res = new int[n];
for (int i = 0; i < n; i++) {
res[i] = list.get(i);
}
return res;
}
}