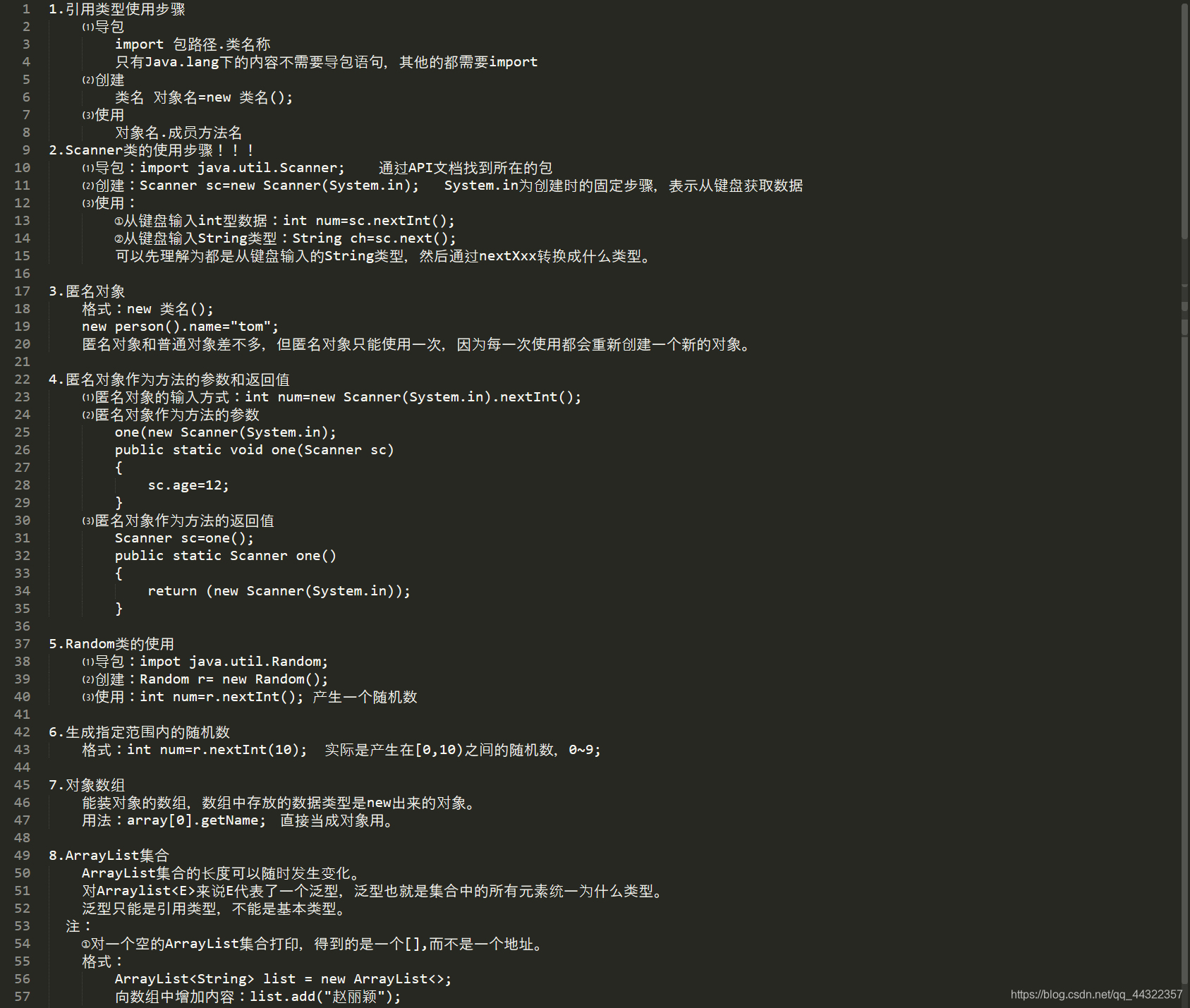
9.ArrayList集合常用方法
public boolean add(E e);
向集合中添加元素,返回值是布尔值,表示是否插入成功。public E get(int index);
从集合中获取元素,参数是索引编号,返回值就是对应位置的元素。public E remove(int index);
从集合中删除元素,参数是索引编号,返回值就是被删除的元素。public int size();
得到集合元素的个数。
例:
public class demo03ArrayListMethond {
public static void main(String[] args) {
//创建集合
ArrayList<String> list = new ArrayList<>();
//向集合中添加元素
list.add("迪丽热巴");
list.add("赵丽颖");
list.add("江疏影");
//访问集合中特定的元素
System.out.println("集合中的第三个元素是:"+list.get(2));
//删除集合中的元素
System.out.println("被删除的元素是:"+list.remove(1));
//显示集合中元素的个数
System.out.println("这个集合中还有多少个元素:"+list.size());
}
}
10.ArrayList集合存储基本类型
- ArrayList集合中不能存储基本数据类型,想要存储基本数据类型,需要转换成包装类
int -->Integer
char -->Character
byte -->Byte
short -->Short
long -->Long
float -->Float
boolean -->Boolean
double -->Double
例
public class demo04data {
public static void main(String[] args) {
//使用时只需要把基本数据类型改成包装类即可,其他照用
ArrayList<Integer> listC = new ArrayList<>();
listC.add(100);
listC.add(200);
System.out.println(listC.get(1));
}
}
11.集合作为方法的参数和返回值
集合不仅可以作为方法的参数,还能作为方法的返回值
public class demo04Special {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
list.add("赵丽颖");
//
specialPrint(list);
}
public static void specialPrint(ArrayList<String> list)
{
System.out.println(list);
}
}
12.字符串的特点
- 字符串的内容不可变。
- 字符串可以共享使用。
- 字符串表面上时char字符数组,但底层时byte字节数组。
13.字符串的构造方法和直接创建
14.字符串常量池
- 对于引用类型来说,==是进行地址值的比较。
- 直接写在双引号中的字符串会进入常量池,常量池中相同的字符串地址值都相等。new出来的字符床不会在常量池中,所以地址不相等。
public class demo04char {
public static void main(String[] args) {
String str1 = "abc";
String str2 = "abc";
char[] array = {'a','b','c'};
String str3 = new String(array);
System.out.println(str1==str2);//true
System.out.println(str1==str3);//false
System.out.println(str2==str3);//false
}
}
15.字符串的比较
- public boolean equals(Object obj); —Object表示参数可以是任何对象,
public class demo04equals {
public static void main(String[] args) {
String str1 = "abc";
String str2 = "abc";
System.out.println(str1.equals(str2));//true
System.out.println("abc".equals(str1));//true
}
}
- public boolean equalsIgnoreCase(String str);—忽略大小写进行比较
public class demo04equals {
public static void main(String[] args) {
String str1 = "Abc";
String str2 = "abc";
System.out.println(str1.equalsIgnoreCase(str2));//true
System.out.println("abc".equalsIgnoreCase(str1));//true
}
}
注:
- equals方法是有对称性的,
a.equals(b)
和b.equals(a)
具有相同效果。 - 如果比较双方一个常量一个变量,推荐把常量字符串写在前面。—例如要写成
"abc".equals(str)
.而不是str.equals("abc");
因为str有可能为null,这时程序就错误了。
16.字符串获取常用方法
- public int length();—获取字符串的长度
- public String concat(String str):—拼接字符串
- public char charAt(int index):—获取指定索引的单个字符
- public int indexOf(String str):—查找参数字符串在本字符串首次出现的位置
例
public class demo05String {
public static void main(String[] args) {
String str1 = "hello";
String str2 = "world";
System.out.println("两个字符串拼接后的字符串是:"+str1.concat(str2));
//两个字符串拼接后的字符串是:helloworld
System.out.println("字符串中的第二个字母是:"+str1.charAt(1));
//字符串中的第二个字母是:e
System.out.println("字符串的长度是:"+str1.length());
//字符串的长度是:5
System.out.println("llo这个字符串第一次出现的位置是:"+str1.indexOf("llo"));
//llo这个字符串第一次出现的位置是:2
}
}
17.字符串的截取方法
public String substring(int index):—截取从参数位置一直到字符串末尾,返回新的字符串。
pubic String substring(int begin,int end):—截取从begin开始,一直到end结束中间的字符串。[begin,end),包含左边,不包含右边。
public class demo05substring {
public static void main(String[] args) {
String str1 = "helloworld";
System.out.println(str1.substring(2));//lloworld
System.out.println(str1.substring(2,5));//llo
}
}
18.字符串的转化相关方法
public char[] toCharArray():—将当前字符串拆分为字符数组作为返回值。
public byte[] getBytes():—获得当前字符串底层的字节数组。
public String replace(CharSequence oldString, CharSequence newString):—将所有出现的老字符串替换为新的字符串,返回替换之后的结果新字符串。
例:
public class demo05Stringconvert {
public static void main(String[] args) {
String str1="hello world";
//将字符串转化为字符数组类型
char[] array = str1.toCharArray();
System.out.println(array[0]);//h
//将字符串转换为byte类型
byte[] bytes = str1.getBytes();
System.out.println(bytes);//[B@1540e19d
//字符串的替换
System.out.println(str1.replace("o","*"));//hell* w*rld
}
}
19.字符串的分割方法
public String[] split(String regex):—按照参数的规则,将字符串分成若干部分。返回值是字符串数组。
但是注意,参数不能是".",不能从点处分,如果从点处分,需要把点写成"\\.";
public class demo05Stringsplit {
public static void main(String[] args) {
String str1 = "aaa,bbb,ccc";
String[] array = new String[10];
array = str1.split(",");
for (int i = 0; i < array.length; i++) {
System.out.println(array[i]);
}
}
}
20.static关键字修饰成员变量和成员方法
- static修饰成员变量或成员方法时,可以通过类名.变量名或类名 .成员方法名来调用,也可以通过创建对象调用,但不推荐
- 如果一个变量定义了static关键字,那么这个变量不再属于对象自己,而是属于所在的类,多个对象共享一份数据。
- 静态的成员方法不能访问非静态的成员方法。
21.静态代码块的特点
- 特点:当第一次用到本类时,静态代码块只执行唯一的一次;静态内容总是优先于非静态内容。所以静态代码块比构造方法先执行;
- 静态代码块的典型用途:用来一次性地对静态成员变量进行赋值。
public class Person {
static {
System.out.println("静态代码块执行了");
}
}
22.Arrays数组工具类
- public static String toString(数组):将参数变成字符串;
- public static void sort(数组):按照默认升序元素对数组进行排序;
- 因为Arrays工具类提供了大量的静态方法,所以在使用的时候不用创建对象;
public class domo06Arrays {
public static void main(String[] args) {
int [] array = {1,3,2};
//对数组进行排序,原来的数组将不再保存
Arrays.sort(array);
//将数组转化为字符串进行输出
System.out.println(Arrays.toString(array));
}
}
23.Math数学工具类
- Math提供了大量的静态方法类,使用时不用创建对象,
- public static double abs (double num): 获取绝对值
- public static double ceil (double num): 向上取整
- public static double floor (double num): 向下取整
- public static long round (double num): 四舍五入
- Math.PI :得到Π的值
public class demo06Math {
public static void main(String[] args) {
//获取绝对值
double num = -3.4;
System.out.println(Math.abs(num));
//向上取整
System.out.println(Math.ceil(num));
//向下取整
System.out.println(Math.floor(num));
//四舍五入
System.out.println(Math.round(num));
//Π的值
System.out.println(Math.PI);
}
}