代码部分:
part1:
logo.png
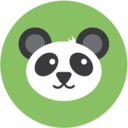
mobile.png

secret.png

btn_shape.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<solid android:color="#32C999"/>
<corners android:radius="30dp"/>
</shape>
效果图:
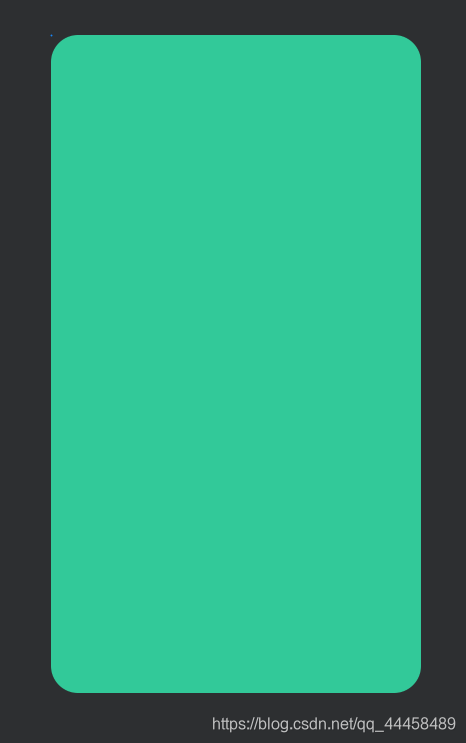
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="50dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:srcCompat="@drawable/logo" />
<LinearLayout
android:id="@+id/linearLayout"
android:layout_width="0dp"
android:layout_height="60dp"
android:layout_marginStart="20dp"
android:layout_marginLeft="20dp"
android:layout_marginTop="30dp"
android:layout_marginEnd="20dp"
android:layout_marginRight="20dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView">
<ImageView
android:id="@+id/imageView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical"
app:srcCompat="@drawable/mobile" />
<EditText
android:id="@+id/et_mobile"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:background="@null"
android:ems="10"
android:hint="请输入手机号"
android:inputType="phone" />
</LinearLayout>
<View
android:id="@+id/view"
android:layout_width="0dp"
android:layout_height="1dp"
android:background="#DFDDDD"
app:layout_constraintEnd_toEndOf="@+id/linearLayout"
app:layout_constraintStart_toStartOf="@+id/linearLayout"
app:layout_constraintTop_toBottomOf="@+id/linearLayout" />
<LinearLayout
android:id="@+id/linearLayout2"
android:layout_width="match_parent"
android:layout_height="60dp"
android:layout_marginStart="20dp"
android:layout_marginLeft="20dp"
android:layout_marginTop="30dp"
android:layout_marginEnd="20dp"
android:layout_marginRight="20dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="@+id/view">
<ImageView
android:id="@+id/imageView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical"
app:srcCompat="@drawable/secret" />
<EditText
android:id="@+id/et_password"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:background="@null"
android:ems="10"
android:hint="请输入密码"
android:inputType="textPassword" />
</LinearLayout>
<View
android:id="@+id/view2"
android:layout_width="0dp"
android:layout_height="1dp"
android:background="#DFDDDD"
app:layout_constraintEnd_toEndOf="@+id/linearLayout2"
app:layout_constraintStart_toStartOf="@+id/linearLayout2"
app:layout_constraintTop_toBottomOf="@+id/linearLayout2" />
<Button
android:id="@+id/button"
android:layout_width="0dp"
android:layout_height="50dp"
android:layout_marginStart="20dp"
android:layout_marginLeft="20dp"
android:layout_marginTop="40dp"
android:layout_marginEnd="20dp"
android:layout_marginRight="20dp"
android:background="@drawable/btn_shape"
android:onClick="login"
android:text="登录"
android:textColor="#FFFFFF"
android:textSize="20sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/view2" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:background="@null"
android:text="忘记密码"
app:layout_constraintStart_toStartOf="@+id/button"
app:layout_constraintTop_toBottomOf="@+id/button" />
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:background="@null"
android:onClick="register"
android:text="注册"
app:layout_constraintEnd_toEndOf="@+id/button"
app:layout_constraintTop_toBottomOf="@+id/button" />
</androidx.constraintlayout.widget.ConstraintLayout>
效果图:
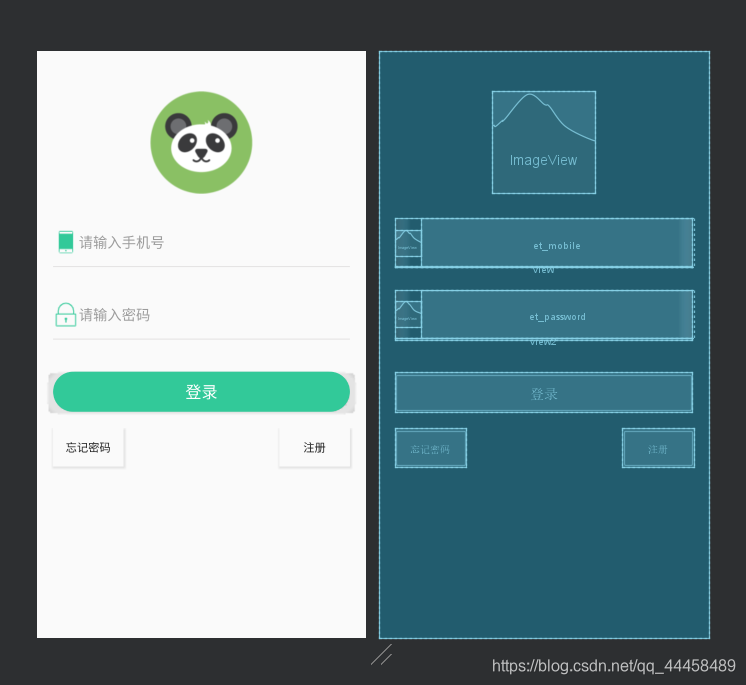
activity_register.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".RegisterActivity">
<ImageView
android:id="@+id/imageView4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="30dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:srcCompat="@drawable/logo" />
<LinearLayout
android:id="@+id/linearLayout3"
android:layout_width="0dp"
android:layout_height="50dp"
android:layout_marginStart="20dp"
android:layout_marginLeft="20dp"
android:layout_marginTop="30dp"
android:layout_marginEnd="20dp"
android:layout_marginRight="20dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView4">
<ImageView
android:id="@+id/imageView5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical"
app:srcCompat="@drawable/mobile" />
<EditText
android:id="@+id/et_mobile"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:background="@null"
android:ems="10"
android:hint="请输入手机号"
android:inputType="phone" />
</LinearLayout>
<View
android:id="@+id/view3"
android:layout_width="0dp"
android:layout_height="1dp"
android:background="#DFdddd"
app:layout_constraintEnd_toEndOf="@+id/linearLayout3"
app:layout_constraintStart_toStartOf="@+id/linearLayout3"
app:layout_constraintTop_toBottomOf="@+id/linearLayout3" />
<LinearLayout
android:id="@+id/linearLayout4"
android:layout_width="0dp"
android:layout_height="50dp"
android:layout_marginTop="20dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="@+id/linearLayout3"
app:layout_constraintStart_toStartOf="@+id/linearLayout3"
app:layout_constraintTop_toBottomOf="@+id/linearLayout3">
<ImageView
android:id="@+id/imageView6"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical"
app:srcCompat="@drawable/secret" />
<EditText
android:id="@+id/et_password"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:background="@null"
android:ems="10"
android:hint="请输入密码"
android:inputType="textPassword" />
</LinearLayout>
<View
android:id="@+id/view4"
android:layout_width="0dp"
android:layout_height="1dp"
android:background="#DFDDDD"
app:layout_constraintEnd_toEndOf="@+id/linearLayout4"
app:layout_constraintStart_toStartOf="@+id/linearLayout4"
app:layout_constraintTop_toBottomOf="@+id/linearLayout4" />
<LinearLayout
android:id="@+id/linearLayout5"
android:layout_width="0dp"
android:layout_height="50dp"
android:layout_marginTop="20dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="@+id/linearLayout4"
app:layout_constraintStart_toStartOf="@+id/linearLayout4"
app:layout_constraintTop_toBottomOf="@+id/view4">
<ImageView
android:id="@+id/imageView7"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical"
app:srcCompat="@drawable/secret" />
<EditText
android:id="@+id/et_repassword"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:background="@null"
android:ems="10"
android:hint="请确认密码"
android:inputType="textPassword" />
</LinearLayout>
<View
android:id="@+id/view5"
android:layout_width="0dp"
android:layout_height="1dp"
android:background="#DDDDDD"
app:layout_constraintEnd_toEndOf="@+id/linearLayout5"
app:layout_constraintStart_toStartOf="@+id/linearLayout5"
app:layout_constraintTop_toBottomOf="@+id/linearLayout5" />
<Button
android:id="@+id/button4"
android:layout_width="0dp"
android:layout_height="50dp"
android:layout_marginTop="30dp"
android:background="@drawable/btn_shape"
android:onClick="register"
android:text="注册"
android:textColor="#FFFFFF"
android:textSize="20sp"
app:layout_constraintEnd_toEndOf="@+id/linearLayout5"
app:layout_constraintStart_toStartOf="@+id/linearLayout5"
app:layout_constraintTop_toBottomOf="@+id/view5" />
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="@+id/button4"
app:layout_constraintStart_toStartOf="@+id/button4"
app:layout_constraintTop_toBottomOf="@+id/button4">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="已有账号,"
android:textColor="#111111" />
<Button
android:id="@+id/button5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@null"
android:text="立即登录"
android:textColor="#32C999" />
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
效果图:
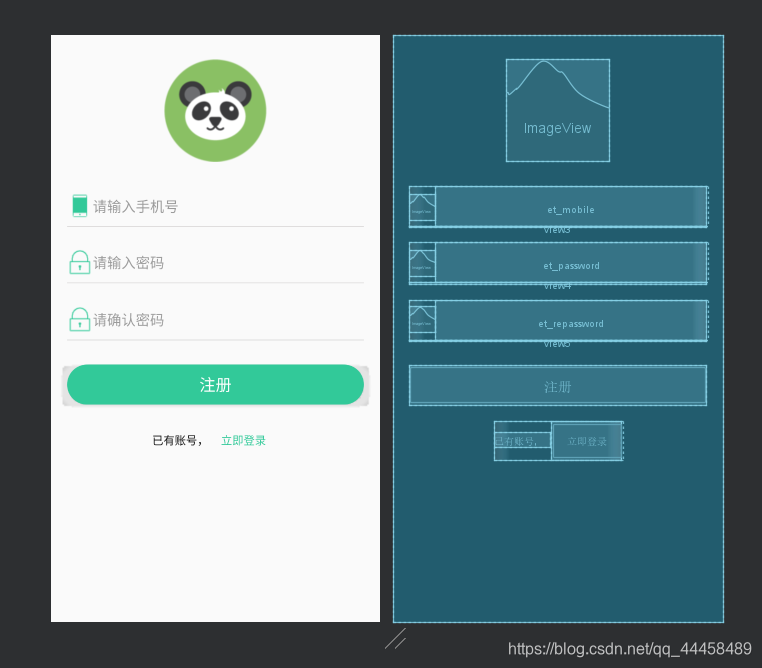
RegisterActivity.java
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.util.Log;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
public class RegisterActivity extends AppCompatActivity {
private EditText et_mobile;
private EditText et_password;
private EditText et_repassword;
private Handler handler = new Handler(){
@Override
public void handleMessage(@NonNull Message msg) {
if ("200".equals(msg.obj)) {
Toast.makeText(RegisterActivity.this,"注册成功", Toast.LENGTH_SHORT).show();
startActivity(new Intent(RegisterActivity.this, MainActivity.class));
} else {
Toast.makeText(RegisterActivity.this,"注册失败", Toast.LENGTH_SHORT).show();
}
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_register);
et_mobile = findViewById(R.id.et_mobile);
et_password = findViewById(R.id.et_password);
et_repassword = findViewById(R.id.et_repassword);
}
public void register(View view) {
final String mobile = et_mobile.getText().toString().trim();
if ("".equals(mobile)) {
Toast.makeText(this, "请输入手机号", Toast.LENGTH_SHORT).show();
return;
}
final String password = et_password.getText().toString().trim();
if ("".equals(password)) {
Toast.makeText(this, "请输入密码", Toast.LENGTH_SHORT).show();
return;
}
String repassword = et_repassword.getText().toString().trim();
if (!password.equals(repassword)) {
Toast.makeText(this, "两次输入的密码不一致", Toast.LENGTH_SHORT).show();
return;
}
new Thread(new Runnable() {
@Override
public void run() {
HttpURLConnection connection = null;
try {
URL url = new URL("http://121.36.161.94:8080/register");
connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
String param = "username="+mobile+"&password="+password;
OutputStream outputStream = connection.getOutputStream();
outputStream.write(param.getBytes());
outputStream.close();
InputStream inputStream = connection.getInputStream();
int i = inputStream.read();
StringBuffer response = new StringBuffer();
while(i != -1) {
response.append((char)i);
i = inputStream.read();
}
inputStream.close();
Log.i("RegisterActivity", response.toString());
JSONObject jsonObject = new JSONObject(response.toString());
String string = jsonObject.getString("code");
Message message = new Message();
message.obj = string;
handler.sendMessage(message);
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} catch (JSONException e) {
e.printStackTrace();
} finally {
if (connection != null) {
connection.disconnect();
}
}
}
}).start();
}
}
IndexActivity.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".IndexActivity">
</androidx.constraintlayout.widget.ConstraintLayout>
IndexActivity.java
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
public class IndexActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_index);
}
}
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.day15">
<uses-permission android:name="android.permission.INTERNET" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme"
android:usesCleartextTraffic="true">
<activity android:name=".IndexActivity"></activity>
<activity android:name=".RegisterActivity" />
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
MainActivity.java
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import org.json.JSONObject;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class MainActivity extends AppCompatActivity {
private EditText et_mobile;
private EditText et_password;
private Handler handler = new Handler(){
@Override
public void handleMessage(@NonNull Message msg) {
if ("200".equals(msg.obj)) {
Toast.makeText(MainActivity.this, "登录成功", Toast.LENGTH_SHORT).show();
startActivity(new Intent(MainActivity.this, IndexActivity.class));
}
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
et_mobile = findViewById(R.id.et_mobile);
et_password = findViewById(R.id.et_password);
}
public void register(View view) {
Intent intent = new Intent(this, RegisterActivity.class);
startActivity(intent);
}
public void login(View view) {
final String username = et_mobile.getText().toString().trim();
if ("".equals(username)) {
Toast.makeText(this, "用户名不能为空", Toast.LENGTH_SHORT).show();
return;
}
final String password = et_password.getText().toString().trim();
if ("".equals(password)) {
Toast.makeText(this, "密码不能为空", Toast.LENGTH_SHORT).show();
}
new Thread(new Runnable() {
@Override
public void run() {
HttpURLConnection connection = null;
try {
URL url = new URL("http://121.36.161.94:8080/login");
connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
String param = "username="+username+"&password="+password;
OutputStream out = connection.getOutputStream();
out.write(param.getBytes());
out.close();
InputStream in = connection.getInputStream();
StringBuffer response = new StringBuffer();
int i = in.read();
while (i != -1) {
response.append((char)i);
i = in.read();
}
in.close();
JSONObject jsonObject = new JSONObject(response.toString());
Message message = new Message();
message.obj = jsonObject.getString("code");
handler.sendMessage(message);
} catch (Exception e) {
e.printStackTrace();
}
}
}).start();
}
}
part2:
rignt.png

add.png

book.png

back.png

btn_edit_shape.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<solid android:color="#32C999"/>
<corners android:radius="2dp"/>
</shape>
效果图:
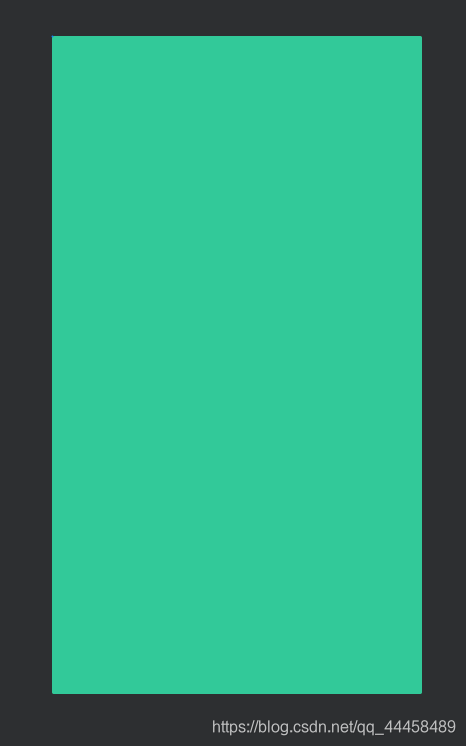
btn_delete_shape.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android">
<solid android:color="#E8BD48"/>
<corners android:radius="2dp"/>
</shape>
效果图:
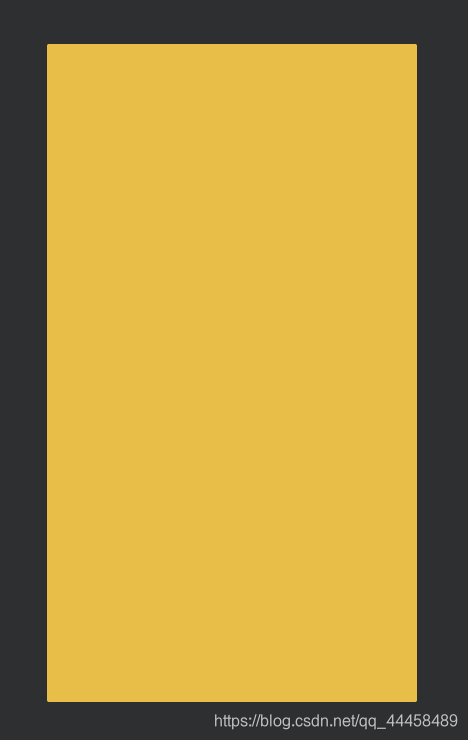
activity_index.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".IndexActivity">
<LinearLayout
android:id="@+id/linearLayout6"
android:layout_width="0dp"
android:layout_height="50dp"
android:layout_marginStart="20dp"
android:layout_marginLeft="20dp"
android:layout_marginEnd="20dp"
android:layout_marginRight="20dp"
android:onClick="bookList"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<ImageView
android:id="@+id/imageView8"
android:layout_width="24dp"
android:layout_height="24dp"
android:layout_gravity="center_vertical"
app:srcCompat="@drawable/book" />
<TextView
android:id="@+id/textView2"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginLeft="10dp"
android:layout_weight="1"
android:gravity="center_vertical"
android:text="书籍管理"
android:textColor="#111111"
android:textSize="20sp" />
<ImageView
android:id="@+id/imageView9"
android:layout_width="24dp"
android:layout_height="24dp"
android:layout_gravity="center_vertical"
app:srcCompat="@drawable/right" />
</LinearLayout>
<View
android:id="@+id/view6"
android:layout_width="0dp"
android:layout_height="1dp"
android:background="#DDDDDD"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/linearLayout6" />
</androidx.constraintlayout.widget.ConstraintLayout>
效果图:
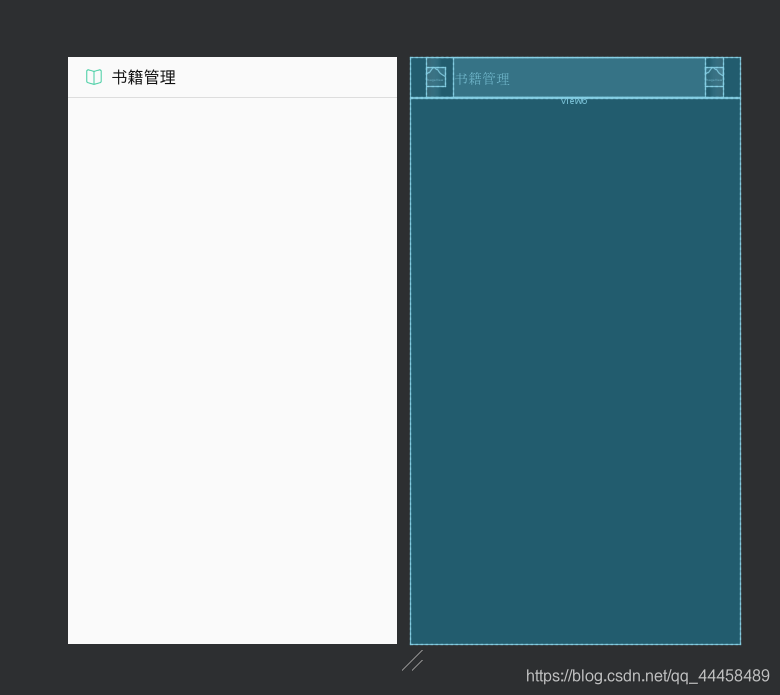
title.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="50dp"
android:background="#32C999"
>
<ImageButton
android:id="@+id/btn_back"
android:layout_width="24dp"
android:layout_height="24dp"
app:srcCompat="@drawable/back"
android:background="@null"
android:layout_centerVertical="true"
android:layout_marginLeft="20dp"/>
<TextView
android:id="@+id/tv_title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="书籍列表"
android:layout_centerInParent="true"
android:textColor="#111111"
android:textSize="20sp"
/>
<ImageButton
android:id="@+id/btn_edit"
android:layout_width="24dp"
android:layout_height="24dp"
app:srcCompat="@drawable/add"
android:layout_alignParentRight="true"
android:background="@null"
android:layout_centerVertical="true"
android:layout_marginRight="20dp"/>
</RelativeLayout>
效果图:
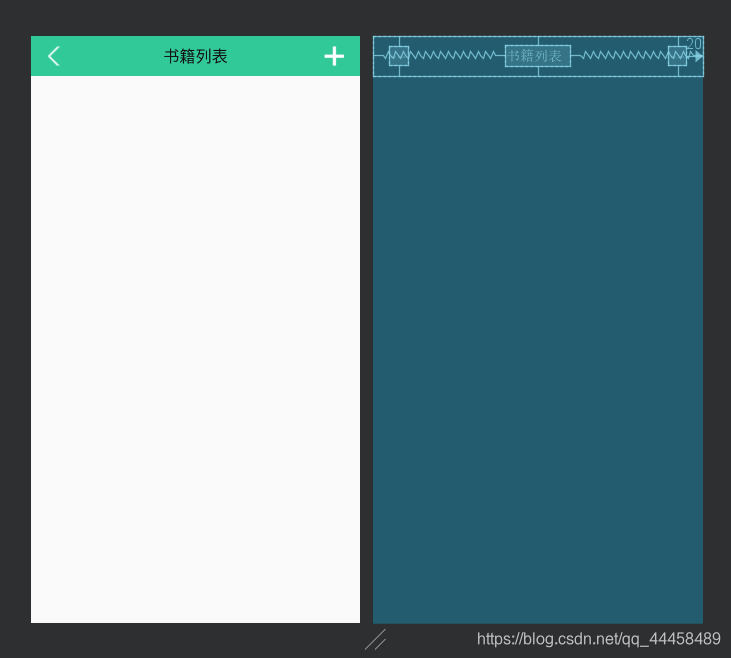
activity_book_list.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".BookListActivity">
<include
android:id="@+id/include"
layout="@layout/title" />
<ListView
android:id="@+id/bookListView"
android:layout_width="0dp"
android:layout_height="0dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/include" />
</androidx.constraintlayout.widget.ConstraintLayout>
效果图:
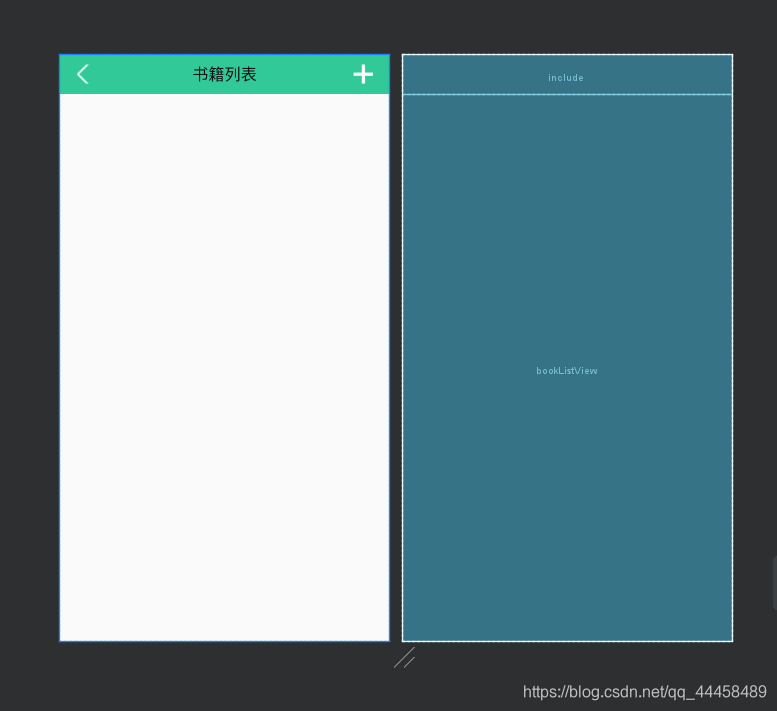
activity_book_add.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".BookAddActivity">
<include
android:id="@+id/include2"
layout="@layout/title" />
<LinearLayout
android:id="@+id/linearLayout7"
android:layout_width="0dp"
android:layout_height="50dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/include2">
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical"
android:layout_marginLeft="20dp"
android:text="书籍名称"
android:textColor="#111111"
android:textSize="16sp" />
<EditText
android:id="@+id/et_name"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_gravity="center_vertical"
android:layout_marginRight="20dp"
android:layout_weight="1"
android:background="@null"
android:ems="10"
android:gravity="right|center_vertical"
android:hint="请输入书籍名称"
android:inputType="textPersonName" />
</LinearLayout>
<View
android:id="@+id/view7"
android:layout_width="0dp"
android:layout_height="1dp"
android:background="#dddddd"
app:layout_constraintEnd_toEndOf="@+id/linearLayout7"
app:layout_constraintStart_toStartOf="@+id/linearLayout7"
app:layout_constraintTop_toBottomOf="@+id/linearLayout7" />
<LinearLayout
android:id="@+id/linearLayout8"
android:layout_width="0dp"
android:layout_height="50dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/view7">
<TextView
android:id="@+id/textView4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="20dp"
android:text="出版时间"
android:textColor="#111111"
android:textSize="16sp" />
<EditText
android:id="@+id/et_publishing"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginRight="20dp"
android:layout_weight="1"
android:background="@null"
android:ems="10"
android:gravity="right|center_vertical"
android:hint="请输入出版时间"
android:inputType="textPersonName" />
</LinearLayout>
<LinearLayout
android:id="@+id/linearLayout9"
android:layout_width="0dp"
android:layout_height="50dp"
android:orientation="horizontal"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/view8">
<TextView
android:id="@+id/textView5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="20dp"
android:text="书籍简介"
android:textColor="#111111"
android:textSize="16sp" />
<EditText
android:id="@+id/et_intro"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_marginRight="20dp"
android:layout_weight="1"
android:background="@null"
android:ems="10"
android:gravity="right|center_vertical"
android:hint="请输入书籍简介"
android:inputType="textPersonName" />
</LinearLayout>
<View
android:id="@+id/view8"
android:layout_width="0dp"
android:layout_height="1dp"
android:background="#DDDDDD"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/linearLayout8" />
<View
android:id="@+id/view9"
android:layout_width="0dp"
android:layout_height="1dp"
android:background="#DDDDDD"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/linearLayout9" />
<Button
android:id="@+id/button6"
android:layout_width="0dp"
android:layout_height="50dp"
android:layout_marginStart="20dp"
android:layout_marginLeft="20dp"
android:layout_marginTop="80dp"
android:layout_marginEnd="20dp"
android:layout_marginRight="20dp"
android:background="@drawable/btn_shape"
android:onClick="addBook"
android:text="保存"
android:textColor="#FFFFFF"
android:textSize="20sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/view9" />
</androidx.constraintlayout.widget.ConstraintLayout>
效果图:
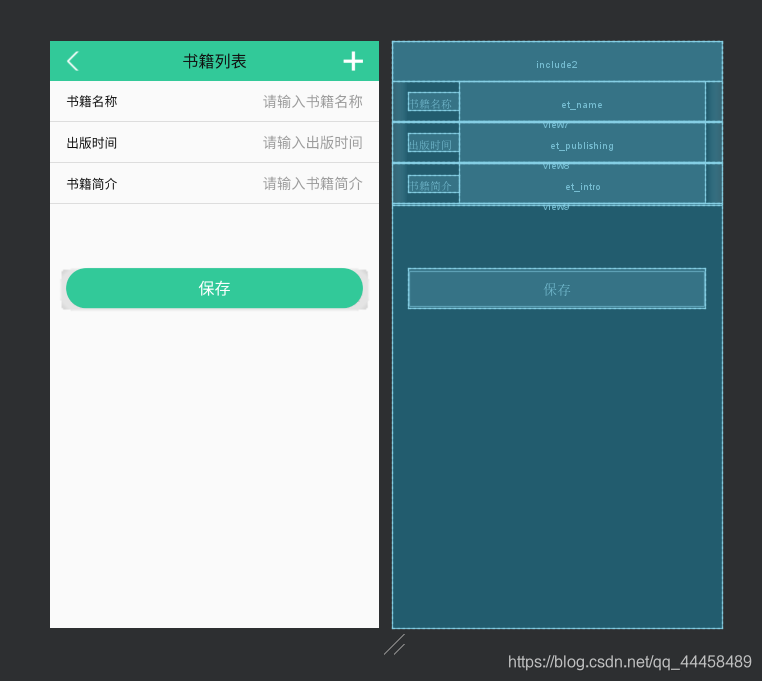
BookAddActivity.java
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import androidx.localbroadcastmanager.content.LocalBroadcastManager;
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.view.View;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import org.json.JSONObject;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class BookAddActivity extends AppCompatActivity {
private EditText et_name;
private EditText et_publishing;
private EditText et_intro;
private Handler handler = new Handler(){
@Override
public void handleMessage(@NonNull Message msg) {
if("200".equals(msg.obj)) {
Toast.makeText(BookAddActivity.this, "书籍添加成功", Toast.LENGTH_SHORT).show();
LocalBroadcastManager.getInstance(BookAddActivity.this).sendBroadcast(new Intent("day15.book.add"));
finish();
}
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_book_add);
et_name = findViewById(R.id.et_name);
et_publishing = findViewById(R.id.et_publishing);
et_intro = findViewById(R.id.et_intro);
findViewById(R.id.btn_edit).setVisibility(View.INVISIBLE);
TextView textView = findViewById(R.id.tv_title);
textView.setText("书籍添加");
findViewById(R.id.btn_back).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
}
public void addBook(View view) {
final String name = et_name.getText().toString().trim();
final String publishing = et_publishing.getText().toString().trim();
final String intro = et_intro.getText().toString().trim();
new Thread(new Runnable() {
@Override
public void run() {
HttpURLConnection connection = null;
try {
URL url = new URL("http://121.36.161.94:8080/book/addBook");
connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
String param = "name="+name+"&publishing="+publishing+"&intro="+intro;
OutputStream out = connection.getOutputStream();
out.write(param.getBytes());
out.close();
InputStream in = connection.getInputStream();
StringBuffer response = new StringBuffer();
int i = in.read();
while(i != -1) {
response.append((char)i);
i = in.read();
}
JSONObject jsonObject = new JSONObject(response.toString());
Message message = new Message();
message.obj = jsonObject.getString("code");
handler.sendMessage(message);
} catch (Exception e) {
e.printStackTrace();
}
}
}).start();
}
}
BookListActivity.java
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
public class BookListActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_book_list);
findViewById(R.id.btn_edit).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
startActivity(new Intent(BookListActivity.this, BookAddActivity.class));
}
});
findViewById(R.id.btn_back).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
}
}
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.day15">
<uses-permission android:name="android.permission.INTERNET" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.AppCompat.DayNight.NoActionBar"
android:usesCleartextTraffic="true">
<activity android:name=".BookAddActivity"></activity>
<activity android:name=".BookListActivity" />
<activity android:name=".IndexActivity" />
<activity android:name=".RegisterActivity" />
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>