Python—第8天—字符串
字符串
字符串是 Python 中最常用的数据类型。我们可以使用引号('
或"
)来创建字符串。
所谓字符串,就是由零个或多个字符组成的有限序列。在Python程序中,如果我们把单个或多个字符用单引号或
者双引号包围起来,就可以表示一个字符串。字符串中的字符可以是特殊符号、英文字母、中文字符、日文的平假
名或片假名、希腊字母、Emoji字符等。
字符串的运算
注意:字符串也是不变数据类型,只能进行读操作
a = 'hello, world'
# 获取字符串长度
print(len(a))
# 循环遍历字符串的每个字符
for i in range(len(a)):
print(a[i])
for i in a:
print(i)
# 重复运算
print(a * 5)
# 成员运算
print('or' in a)
print('ko' in a)
b = 'hello,World'
# 比较运算(比较字符串的内容 ---> 字符编码大小)
print(a == b)
print(a != b)
c = 'goodbye,world'
print(b > c)
d = 'hello,everybody'
print(b >= d)
e = '!!!'
print(d + e)
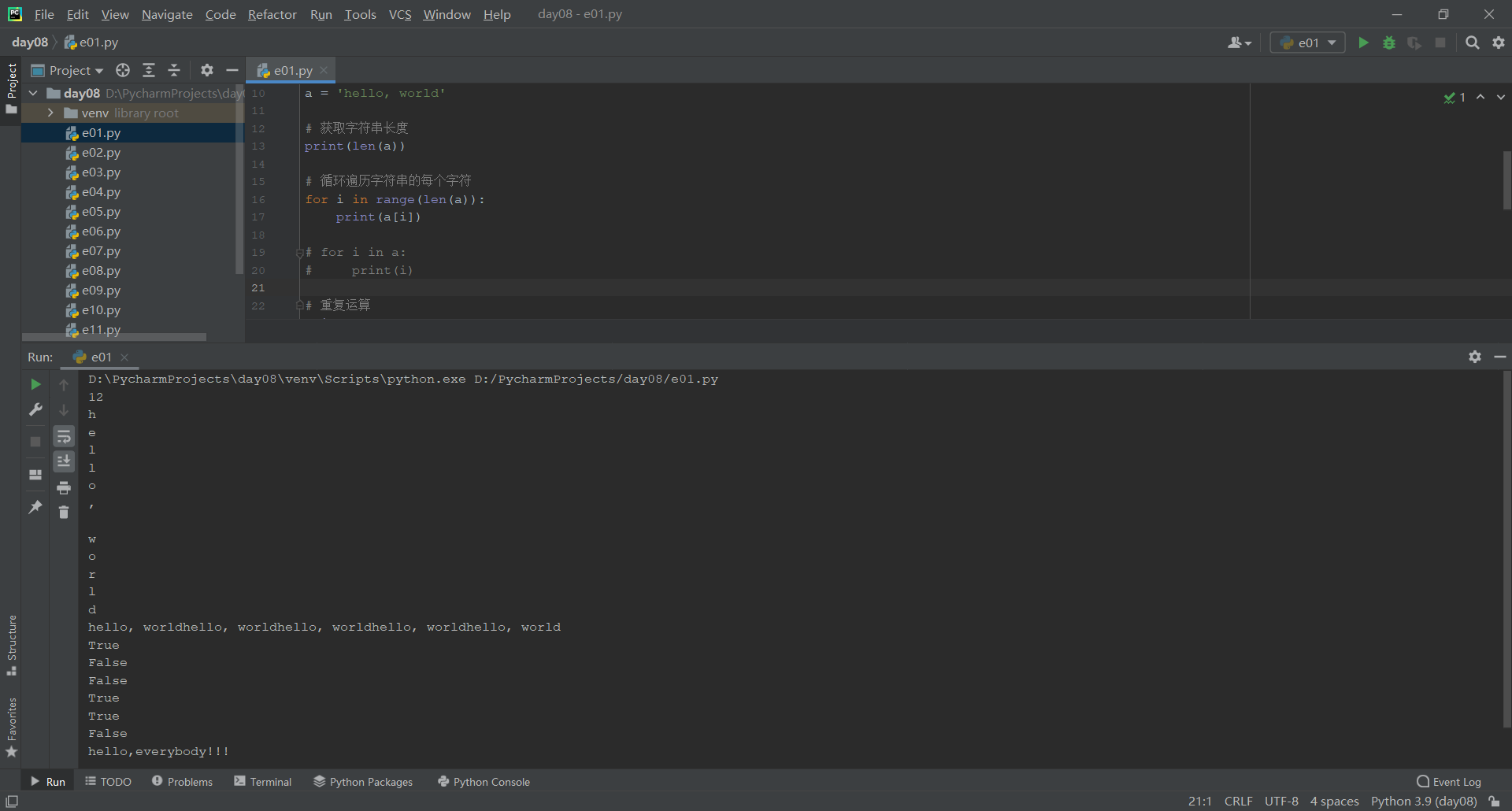
字符串的索引和切片
索引值以 0 为开始值,-1 为从末尾的开始位置。
a = 'hello, world'
print(a[0], a[-len(a)])
print(a[len(a) - 1], a[-1])
print(a[6])
print(a[2:5]) # 获取索引为 2 - 5 的元素,包括索引为 2 的元素
print(a[1:10:2]) # 获取索引为 1 - 10 的元素,步长为 2
print(a[::-1]) # 将字符串反转
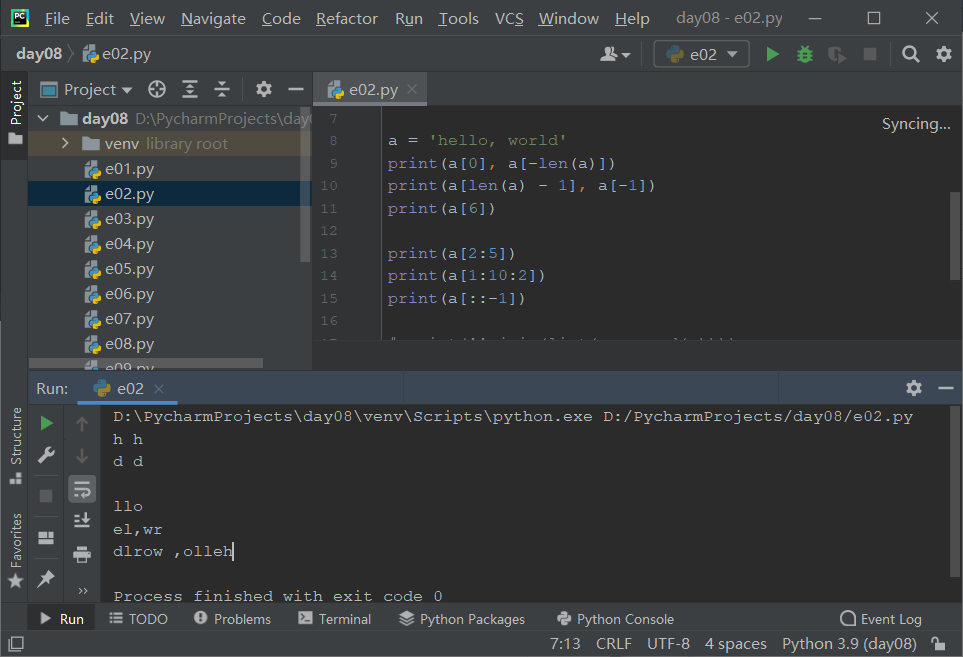
跑马灯文字效果
OS —> Operating System —> 操作系统 —> macOS / clear win / cls
程序得进入终端Termina里面运行,输入Python 文件名.py 按回车键开始运行,按Ctrl + c 停止运行
import time
import os
content = '拼搏到无能为力,坚持到感动自己 '
while True:
# system - 调用系统命令
os.system('cls')
print(content)
# 休眠 - 让程序暂停300ms
time.sleep(0.3)
content = content[1:] + content[0]
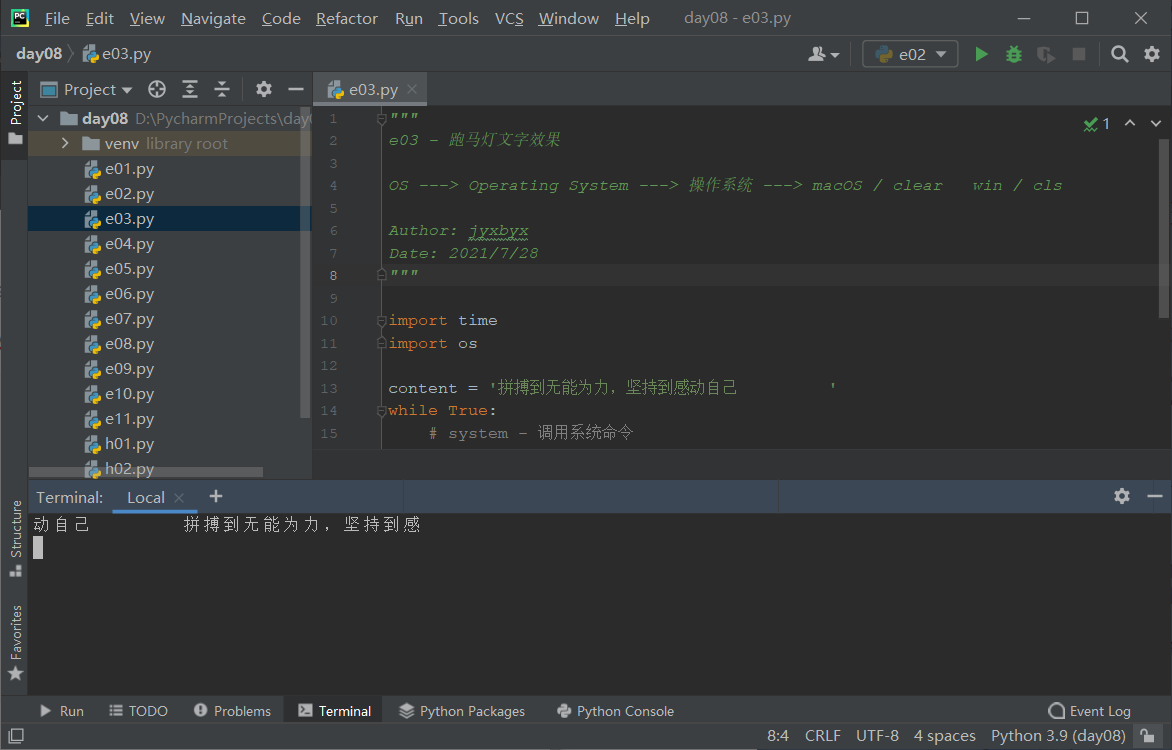
字符串的大小写字母转换
a = 'hello, World 123'
# 转大写
print(a.upper())
# 转小写
print(a.lower())
# 首字母大写
print(a.capitalize())
# 每个单词首字母大写
print(a.title())
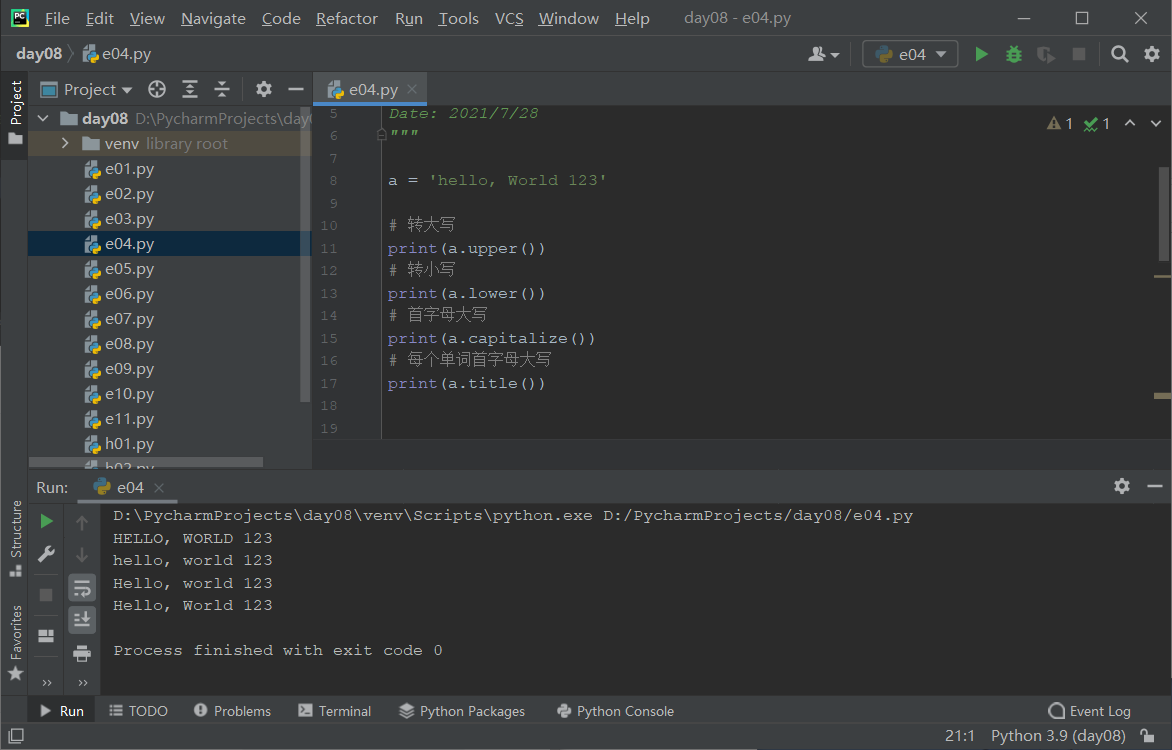
字符串的判断
b = 'abc123'
# 判断字符串是不是数字
print(b.isdigit())
# 判断字符串是不是字母
print(b.isalpha())
# 判断字符串是不是数字和字母
print(b.isalnum())
# 判断字符串是不是ASCII字符
print(b.isascii())
c = '你好呀'
print(c.isascii())
# 判断字符串是否以指定内容开头
print(c.startswith('你'))
# 判断字符串是否以指定内容结尾
print(c.endswith('啊'))
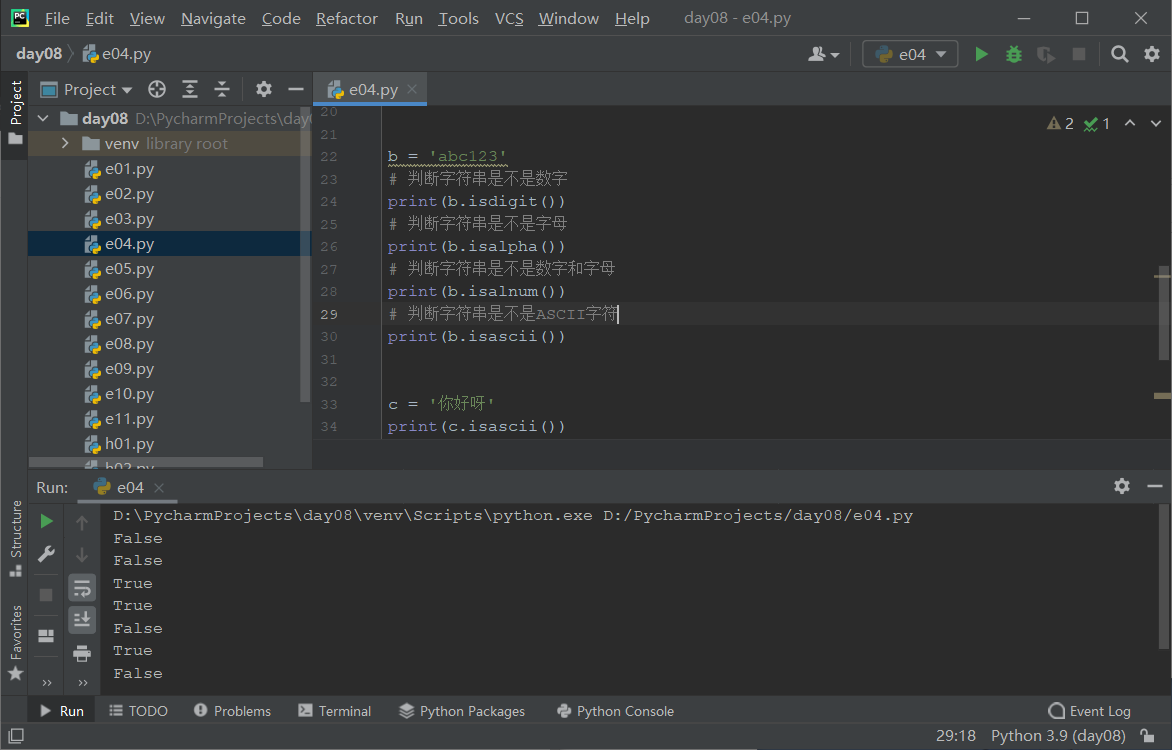
字符串的查找
在字符串中查找有没有某个子串的操作
- index / rindex
- find / rfind
a = 'Oh apple, i love apple.'
# index - 从左向右寻找指定的子串,可以指定从哪开始找,默认是0
# 找到了返回子串对应的索引(下标),找不到直接报错(程序崩溃)
print(a.index('apple'))
print(a.index('apple', 10))
print(a.rindex('apple'))
print(a.find('apple'))
print(a.find('apple', 10))
print(a.rfind('apple'))
print(a.find('banana'))
print(a.rfind('banana'))
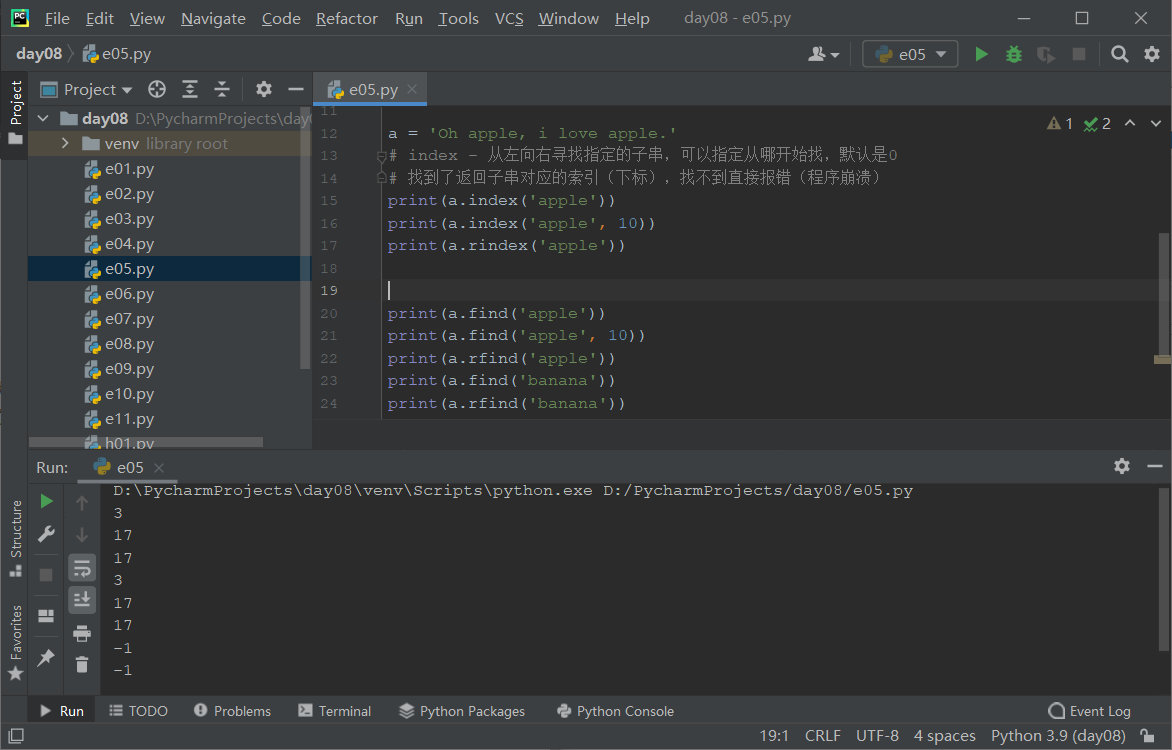
字符串的对齐和填充
a = 'hello, world'
# 居中
print(a.center(80, '='))
# 右对齐
print(a.rjust(80, '='))
#左对齐
print(a.ljust(80, '='))
b = '123'
# 零填充(在左边补0)
print(b.zfill(6))
c = 1234
d = 345
print('%d + %d = %d' % (c, d, c + d))
# Python 3.6 引入的格式化字符串的便捷式语法
print(f'{c} + {d} = {c + d}')
print('{} + {} = {}'.format(c, d, c + d))
print('{2} + {1} = {0}'.format(c + d, d, c))
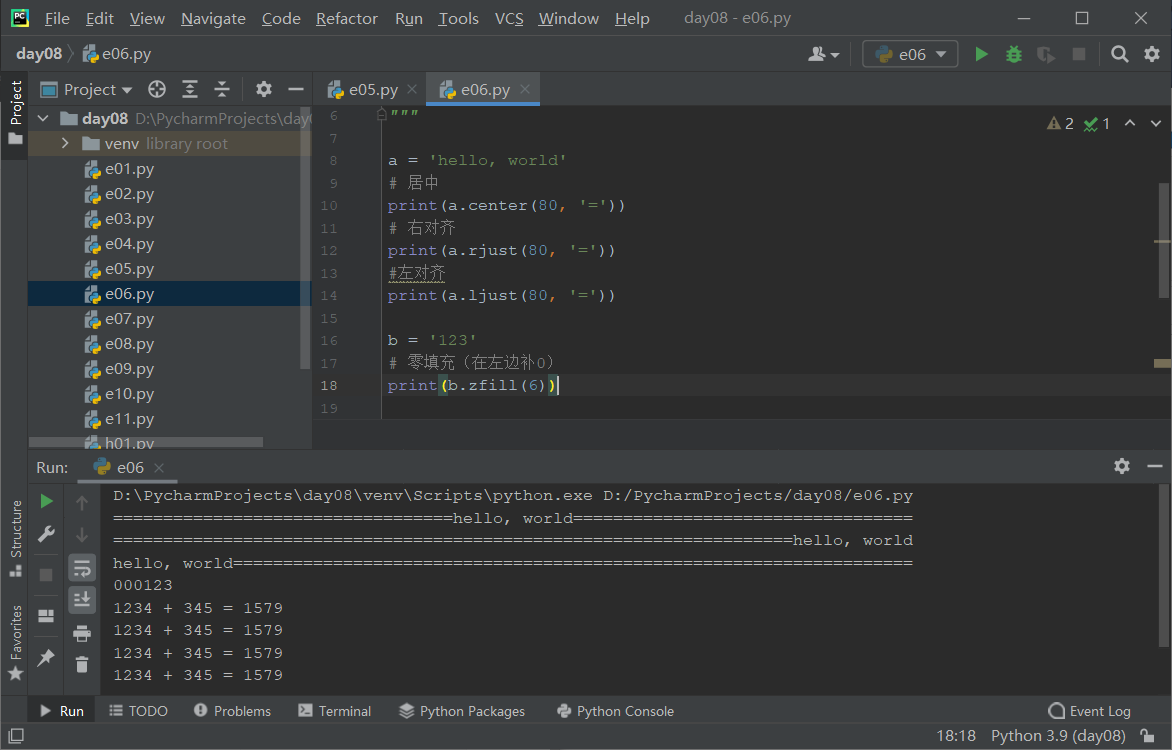
字符串的修剪与替换
email = ' 46548@123.com '
content = ' 张三是笨蛋 '
# 修剪字符串左右两端的空格
print(email.strip())
print(content.strip())
# 修剪字符串左端空格
print(email.lstrip())
# 修剪字符串右端空格
print(content.rstrip())
# 将指定的字符串替换为新的内容
print(content.strip().replace('张三', '*').replace('笨蛋', '*'))
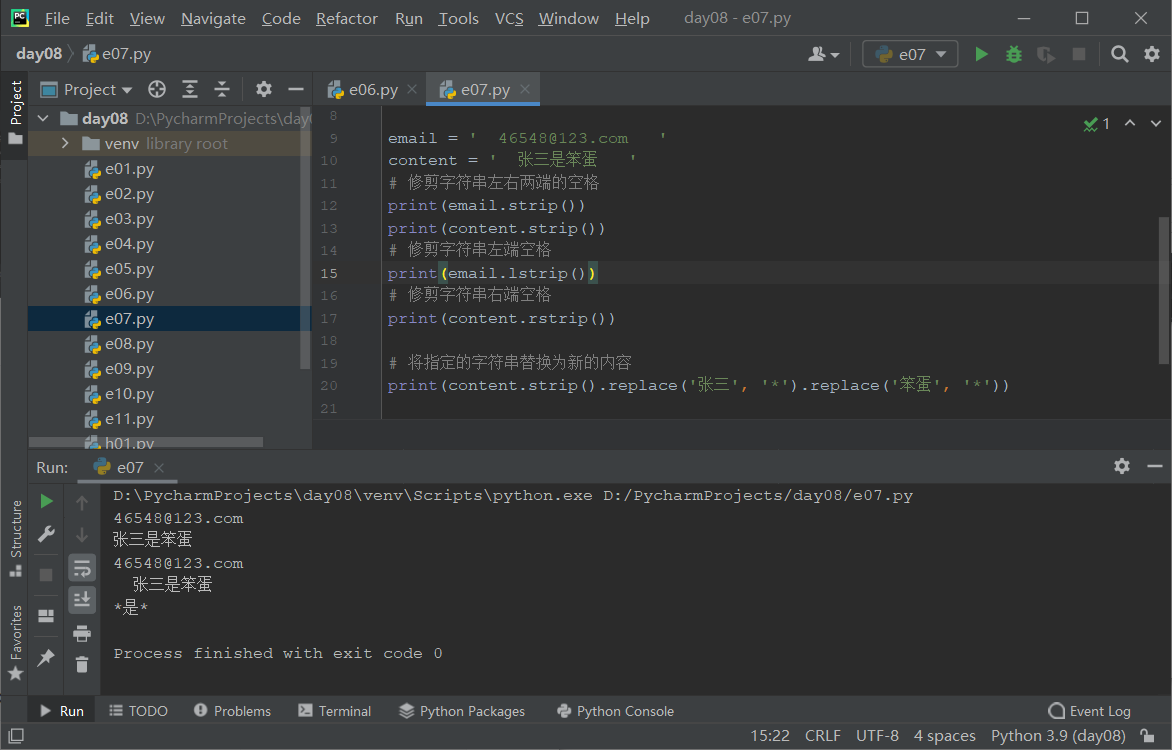
字符串的拆分和合并
split —> 拆分
join —> 合并
content = 'You go you way, I will go mine.'
content2 = content.replace(',', '').replace('.', '')
# 用空格拆分字符串得到一个列表
words = content2.split()
print(words, len(words))
for word in words:
print(word)
# 用空格拆分字符串, 最多允许拆分3次
words = content2.split(' ', maxsplit=3)
print(words, len(words))
# 从右向左拆分字符串, 最多允许拆分3次
words = content2.rsplit(' ', maxsplit=3)
print(words, len(words))
# 用逗号拆分字符串
items = content.split(',')
for item in items:
print(item)
contents = [
'123',
'456',
'789',
'123'
]
print('~'.join(contents))
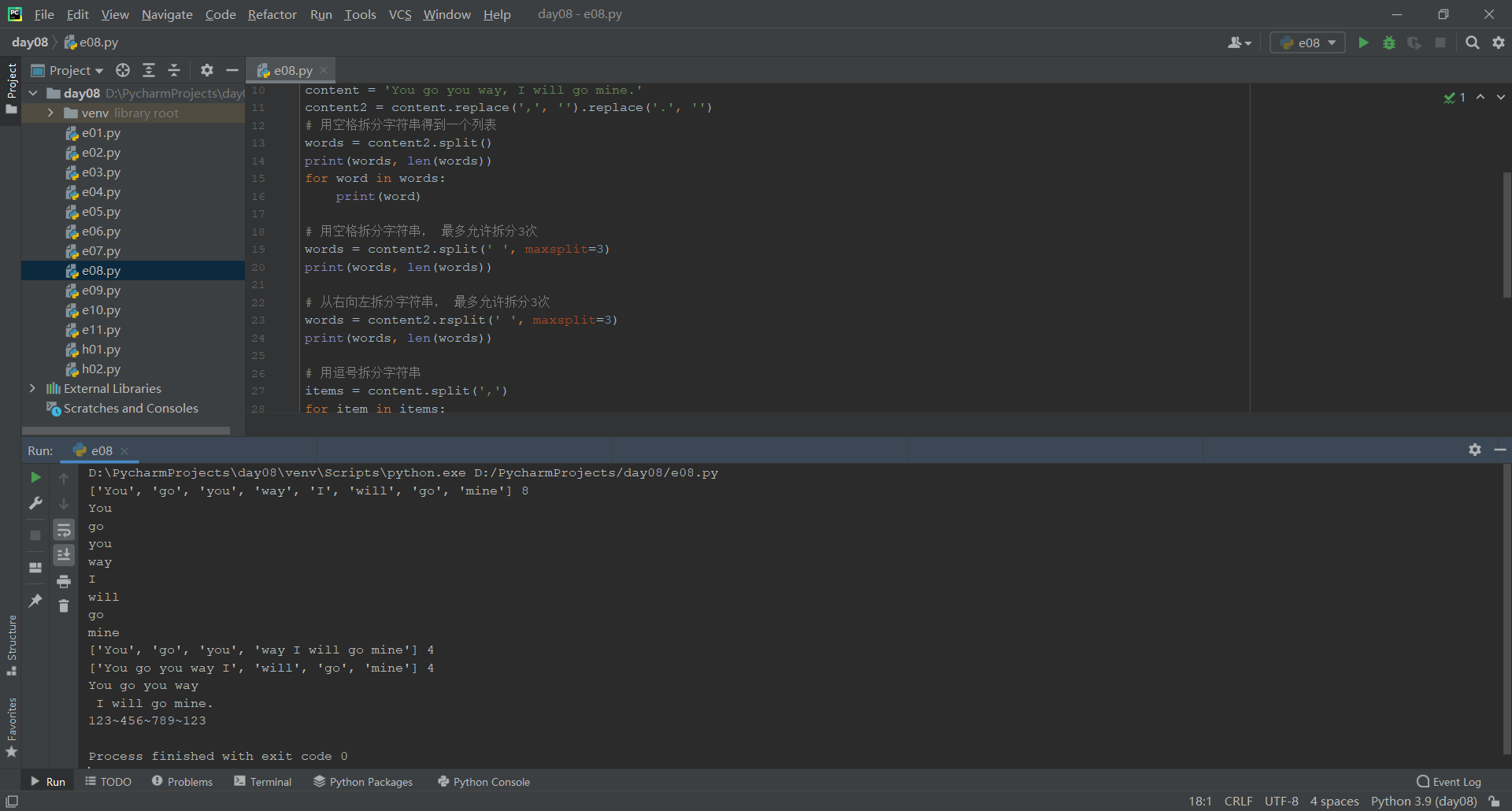
字符串的编码与解码
编码 —> 把一种字符集转换成另外一种字符集
解码 —> 把数码还原成它所代表的内容
str(字符串) —> encode(字符集) —> bytes(字节串)
bytes(字节串) —> decode(字符集) —> str(字符串)
a = '我爱你中国'
b = a.encode('gbk')
print(type(b))
print(b, len(b))
c = b'\xce\xd2\xb0\xae\xc4\xe3\xd6\xd0\xb9\xfa'
print(c.decode('gbk'))
c = '✔🎁'
d = c.encode()
print(d, len(b))
print(d.decode())
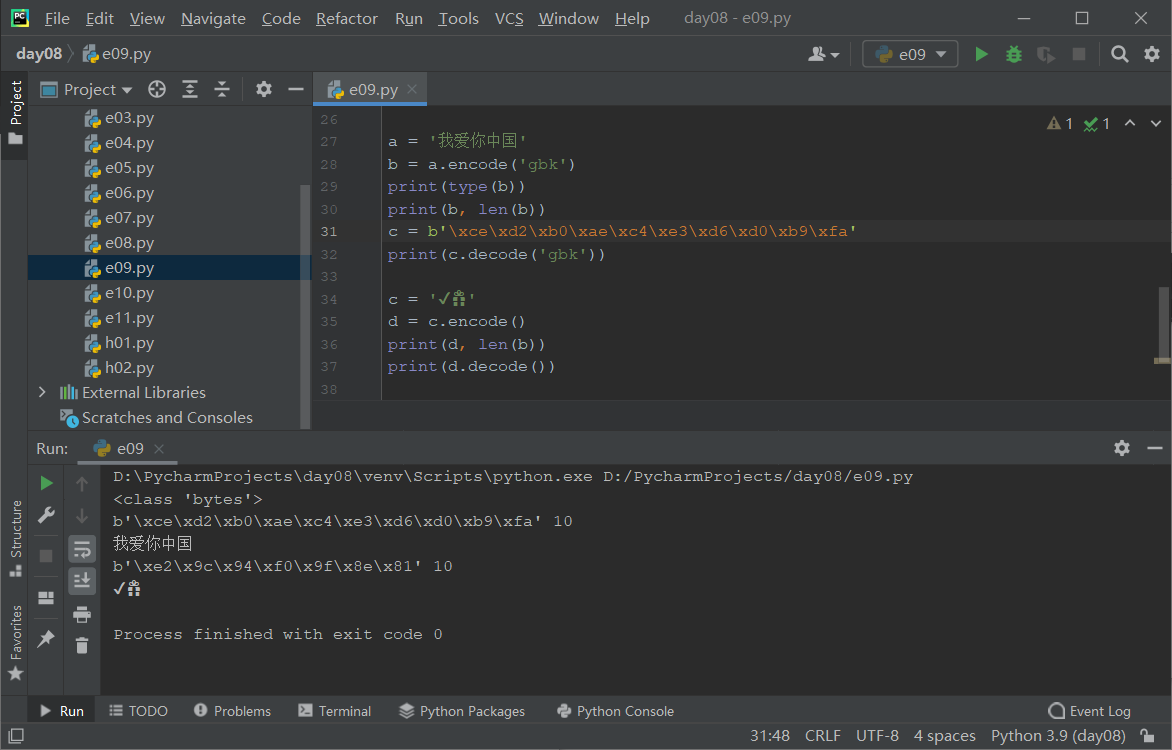
要点:
1,选择字符集(编码)的时候,最佳的选择(也是默认的)是utf-8编码。
2.编码和解码的字符集要保持一致,否则就会出现乱码现象。
3.不能用iso-8859-1编码中文,否则会出现编码黑洞,中文变成?。
4.utf—8是Unicode的一种实现方案,也是一种变长的编码,
最少1个字节(英文和数字),最多4个字节(Emoji),表示中文用3个字节。
字符串的加密解密
凯撒密码
明文: attack at dawn .
密文: dwwdfn dw gdzq.对称加密︰加密和解密使用了相同的密钥—>AES。
非对称加密:加密和解密使用不同的密钥(公钥、私钥) —>RSA —>适合互联网应用。
message = 'attack at dawn.'
# 生成字符串转换的对照表
table = str.maketrans(
'abcdefghijklmnopqrstuvwxyz',
'defghijklmnopqrstuvwxyzabc'
)
# 通过字符串的translate方法实现字符串转义
print(message.translate(table))
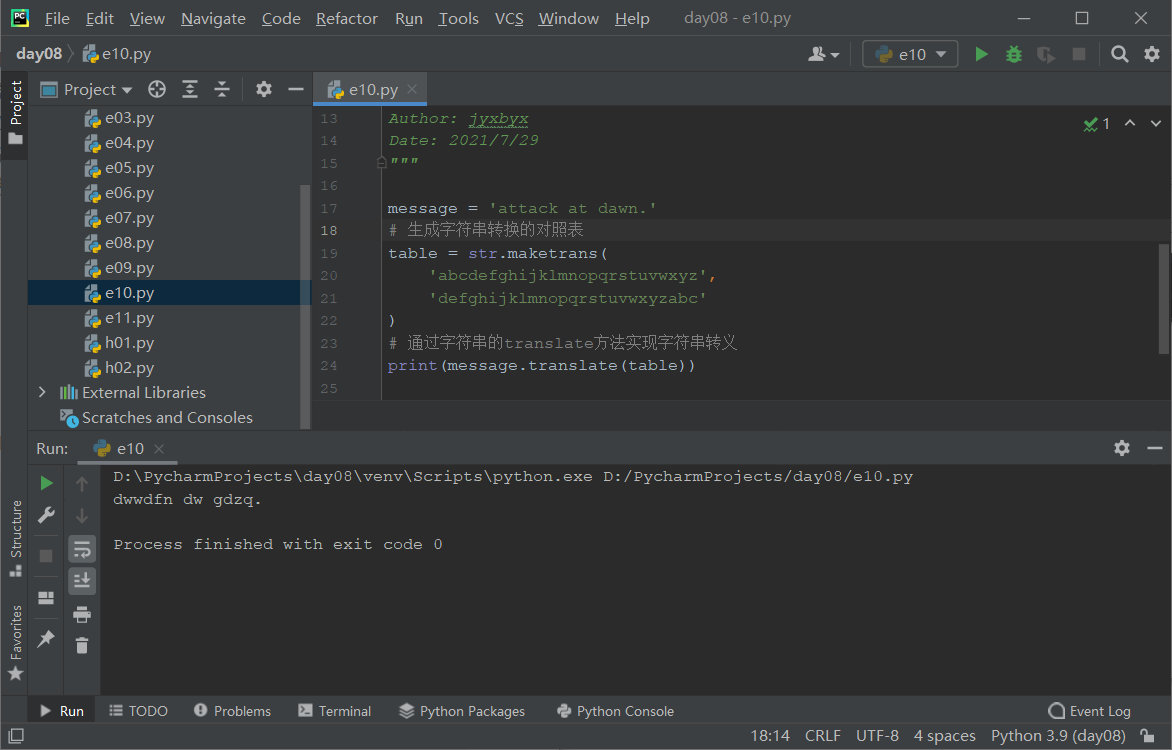
生成随机验证码
import random
import string
all_chars = string.ascii_letters + string.digits
for _ in range(10):
selected_chars = random.choices(all_chars, k=4)
print(''.join(selected_chars))
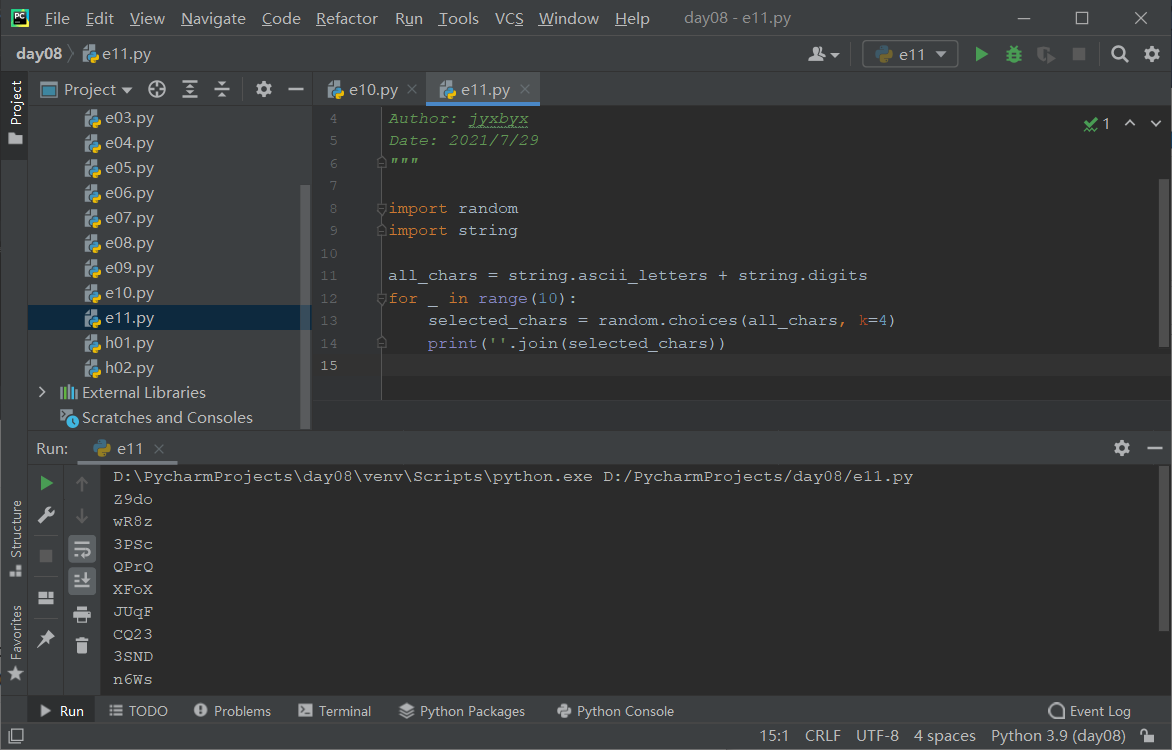