1 线程概述
01 线程概述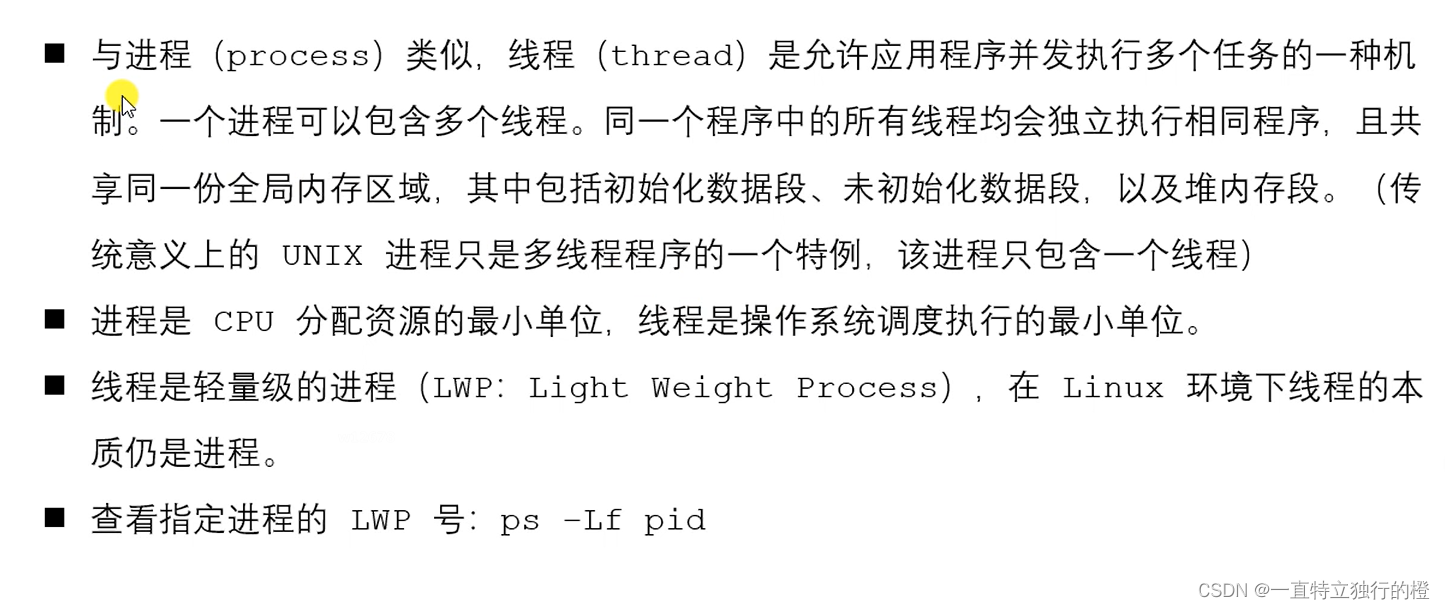
02 线程和进程的区别
创建进程虽然使用了写时复制技术,但是仍然要复制父进程的信息,而创建线程,线程间是共享虚拟地址空间
03 线程和进程虚拟地址空间
创建一个进程,就是把原先的地址空间拷贝一下,如果只读那么他们共享虚拟内存空间,如果要写就需要拷贝一个虚拟内存空间,与真实的内存做一个映射
创建一个线程,是和原来的共用一个虚拟空间,但是里面的栈空间会开辟成一个个的单独空间,代码段(.text段)也是会划分成一个个单独空间
04 线程之间共享和非共享资源
05 NPTL
2 创建线程
06 线程操作
线程的创建
/*
一般情况下,main函数所在的线程我们称之为主线程(main线程),其余创建的线程
称之为子线程。
程序中默认只有一个进程,fork()函数调用,2进行
程序中默认只有一个线程,pthread_create()函数调用,2个线程。
#include <pthread.h>
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine) (void *), void *arg);
- 功能:创建一个子线程
- 参数:
- thread:传出参数,线程创建成功后,子线程的线程ID被写到该变量中。
- attr : 设置线程的属性,一般使用默认值,NULL
- start_routine : 函数指针,这个函数是子线程需要处理的逻辑代码
- arg : 给第三个参数使用,传参
- 返回值:
成功:0
失败:返回错误号。这个错误号和之前errno不太一样。
获取错误号的信息: char * strerror(int errnum);
*/
#include<stdio.h>
#include<pthread.h>
#include<string.h>
#include<unistd.h>
void *callback(void *arg){
printf("child thread....\n");
printf("arg value : %d \n",*(int *)arg);
return NULL;
}
int main(){
pthread_t tid;
int num = 10;
// 创建一个子线程
int ret = pthread_create(&tid,NULL,callback,(void *)&num);
if(ret != 0){
// 不正常
char * errstr = strerror(ret);
printf("error : %s \n",errstr);
}
for(int i = 0;i<5;++i){
printf("%d \n",i);
}
sleep(1);
return 0;
}
3终止线程
/*
#include<pthread.h>
void pthread_exit(void *retval);
功能:终止一个线程,在哪个线程中调用,就表示终止哪个线程
参数:
retval:需要传递一个指针,作为一个返回值,可以在pthread_join()中获取到
pthread_t pthread_self(void);
功能:获取当前的线程的线程ID
int pthread_equal(pthread_t t1, pthread_t t2);
功能:比较两个线程ID是否相等
不同的操作系统,pthread_t类型的实现不一样,有的是无符号的长整型,有的
是使用结构体去实现的。
*/
#include<stdio.h>
#include<pthread.h>
#include<string.h>
void *callback(void * arg){
printf("child thread id : %ld\n",pthread_self());
return NULL; //pthread_exit(NULL);
}
int main(){
//创建一个子线程
// pthread_t 用来声明线程的id
pthread_t tid;
int ret = pthread_create(&tid,NULL,callback,NULL);
if(ret != 0){
char *errstr = strerror(ret);
printf("error : %s\n",errstr);
}
//主线程
for(int i =0;i<5;++i){
printf("%d\n",i);
}
printf("tid : %ld, main thread id : %ld \n",tid,pthread_self());
//让主线程退出,当主线程退出时,不会影响其他正常运行的线程
pthread_exit(NULL);
printf("main thread exit\n");
return 0;
}
4 连接已终止的线程
/*
#include<pthread.h>
int pthread_join(pthread_t thread, void **retval);
功能:和一个已经终止的线程进行连接
回收子线程的资源
这个函数是阻塞函数,调用一次只能回收一个子线程
一般在主线程中使用
参数:
thread:需要回收的子线程的ID
retval:接收子线程退出时的返回值
返回值:
0:成功
非0:失败,返回的错误号
*/
#include<stdio.h>
#include<pthread.h>
#include<string.h>
#include<unistd.h>
int value = 10;
void *callback(void * arg){
printf("child thread id : %ld\n",pthread_self());
sleep(3);
//return NULL; //pthread_exit(NULL);
//int value = 10; // 局部变量,在子线程结束的时候,栈空间会释放掉,数据也就消失了,应该定义一个全局变量
pthread_exit((void *)&value);
}
int main(){
//创建一个子线程
// pthread_t 用来声明线程的id
pthread_t tid;
int ret = pthread_create(&tid,NULL,callback,NULL);
if(ret != 0){
char *errstr = strerror(ret);
printf("error : %s\n",errstr);
}
//主线程
for(int i =0;i<5;++i){
printf("%d\n",i);
}
printf("tid : %ld, main thread id : %ld \n",tid,pthread_self());
// 主线程调用pthread_join()回收子线程的资源
// 如果子线程还没有结束,pthread_join会一直阻塞在这里
int * thread_retval;
ret = pthread_join(tid,(void **)&thread_retval);
if(ret != 0){
char *errstr = strerror(ret);
printf("error : %s\n",errstr);
}else {
printf("success\n");
}
printf("exit data : %d\n",*thread_retval);
//让主线程退出,当主线程退出时,不会影响其他正常运行的线程
pthread_exit(NULL);
return 0;
}
5 线程的分离
/*
#include<pthread.h>
int pthread_detach(pthread_t thread);
功能:分离一个线程。被分离的线程在终止的时候,会自动释放资源返回给系统。
1、不能多次分离,会产生不可预料的行为。
2、不能去连接一个已经分离的线程,否则会报错。
参数:需要分离的线程的ID
返回值:成功0
失败:返回错误号
*/
#include<stdlib.h>
#include<pthread.h>
#include<string.h>
#include<unistd.h>
#include<stdio.h>
void *callback(void *arg){
printf("child thread : %ld",pthread_self());
return NULL;
}
int main(){
//创建一个子线程
pthread_t tid;
int ret = pthread_create(&tid,NULL,callback,NULL);
if(ret != 0){
char *errstr = strerror(ret);
printf("error data : %s \n",errstr);
}
//输出主线程和子线程的ID
printf("parent thread : %ld , child tread : %ld \n",pthread_self(),tid);
//设置子线程分离,子线程分离后,子线程结束时对应的资源就不需要主线程释放
pthread_detach(tid);
//退出主线程,对子线程没有影响
pthread_exit(NULL);
return 0;
}
6 线程取消
/*
#include<pthread.h>
int pthread_cancel(pthread_t thread);
功能:取消线程(让线程终止)
取消某个线程,可以终止某个线程的运行,
但是并不是立马终止,而是当子线程执行到一个取消点,线程才会终止。
取消点:系统规定好的一些系统调用,我们可以粗略的理解为用户区到内核区的切换,这个位置称为取消点。
*/
#include<stdlib.h>
#include<pthread.h>
#include<string.h>
#include<unistd.h>
#include<stdio.h>
void *callback(void *arg){
printf("child thread : %ld\n",pthread_self());
for(int i = 0; i< 5; i++){
printf("child : %d\n",i);
}
return NULL;
}
int main(){
//创建一个子线程
pthread_t tid;
int ret = pthread_create(&tid,NULL,callback,NULL);
if(ret != 0){
char *errstr = strerror(ret);
printf("error data : %s \n",errstr);
}
// 取消线程
pthread_cancel(tid);
for(int i = 0; i< 5; i++){
printf("%d\n",i);
}
//输出主线程和子线程的ID
printf("parent thread : %ld , child tread : %ld \n",pthread_self(),tid);
//退出主线程,对子线程没有影响
pthread_exit(NULL);
return 0;
}
程序运行多次发现运行结果并不相同,这说明pthread_cancel取消一个线程并不是立马终止,而是到达一个取消点之后才会终止
7 线程属性
跟属性相关的线程的一些函数 (按两次tab键)
/*
int pthread_attr_init(pthread_attr_t *attr);
- 初始化线程属性变量
int pthread_attr_destroy(pthread_attr_t *attr);
- 释放线程属性的资源
int pthread_attr_getdetachstate(const pthread_attr_t *attr, int *detachstate);
- 获取线程分离的状态属性
int pthread_attr_setdetachstate(pthread_attr_t *attr, int detachstate);
- 设置线程分离的状态属性
*/
#include<stdlib.h>
#include<pthread.h>
#include<string.h>
#include<unistd.h>
#include<stdio.h>
void *callback(void *arg){
printf("child thread : %ld\n",pthread_self());
return NULL;
}
int main(){
//创建一个线程属性变量
pthread_attr_t attr;
//初始化属性变量
pthread_attr_init(&attr);
//设置属性
pthread_attr_setdetachstate(&attr,PTHREAD_CREATE_DETACHED);
//创建一个子线程
pthread_t tid;
int ret = pthread_create(&tid,&attr,callback,NULL);
if(ret != 0){
char *errstr = strerror(ret);
printf("error data : %s \n",errstr);
}
//获取线程的栈的大小
size_t size;
pthread_attr_getstacksize(&attr,&size);
printf("thread satck size : %ld \n",size);
//输出主线程和子线程的ID
printf("parent thread : %ld , child tread : %ld \n",pthread_self(),tid);
//释放线程属性资源
pthread_attr_destroy(&attr);
//退出主线程,对子线程没有影响
pthread_exit(NULL);
return 0;
}
8 线程同步
/*
使用多线程实现买票的案例
有3个窗口,一共是100张票
*/
#include<stdio.h>
#include<pthread.h>
#include<unistd.h>
//门票不能放到sellticket函数里面,不然三个子线程都会卖100张,不合题意,一共就100张
static int tickets = 100;
void * selltickets(void *arg){
//卖票
while(tickets > 0){
//睡眠多少微秒
usleep(3000);
printf("%ld 正在卖第 %d 张门票\n",pthread_self(),tickets);
tickets --;
}
}
int main(){
//创建3个子线程
pthread_t tid1,tid2,tid3;
pthread_create(&tid1,NULL,selltickets,NULL);
pthread_create(&tid2,NULL,selltickets,NULL);
pthread_create(&tid3,NULL,selltickets,NULL);
//回收子线程的资源,阻塞
pthread_join(tid1,NULL);
pthread_join(tid2,NULL);
pthread_join(tid3,NULL);
//回收子线程资源和设置线程分离 二选一
//设置线程分离
// pthread_detach(tid1);
// pthread_detach(tid2);
// pthread_detach(tid3);
//退出主线程
pthread_exit(NULL);//不影响子线程
return 0;
}
卖票案例遇到的问题
解决办法:线程同步
9 互斥锁
实现线程同步的手段:互斥量(互斥锁)
使用互斥量给某共享资源加锁
/*
互斥量的类型 pthread_mutex_t
int pthread_mutex_init(pthread_mutex_t *restrict mutex, const pthread_mutexattr_t *restrict attr);
- 初始化互斥量
- 参数 :
- mutex : 需要初始化的互斥量变量
- attr : 互斥量相关的属性,NULL
- restrict : C语言的修饰符,被修饰的指针,不能由另外的一个指针进行操作。
pthread_mutex_t *restrict mutex = xxx;
pthread_mutex_t * mutex1 = mutex; //报错
int pthread_mutex_destroy(pthread_mutex_t *mutex);
- 释放互斥量的资源
int pthread_mutex_lock(pthread_mutex_t *mutex);
- 加锁,阻塞的,如果有一个线程加锁了,那么其他的线程只能阻塞等待
int pthread_mutex_trylock(pthread_mutex_t *mutex);
- 尝试加锁,如果加锁失败,不会阻塞,会直接返回。
int pthread_mutex_unlock(pthread_mutex_t *mutex);
- 解锁
*/
#include<stdio.h>
#include<pthread.h>
#include<unistd.h>
//门票不能放到sellticket函数里面,不然三个子线程都会卖100张,不合题意,一共就100张
static int tickets = 1000;
//创建一个互斥量
pthread_mutex_t mutex;
void * selltickets(void *arg){
//加锁
//pthread_mutex_lock(&mutex);
//卖票 这样不行,虽然数据没有问题,但是都是一个线程卖的
// while(tickets > 0){
// //睡眠多少微秒
// usleep(3000);
// printf("%ld 正在卖第 %d 张门票\n",pthread_self(),tickets);
// tickets --;
// }
//改进
while(1){
//加锁
pthread_mutex_lock(&mutex);
if(tickets>0){
usleep(8000);
printf("%ld 正在卖第 %d 张门票\n",pthread_self(),tickets);
tickets --;
}else{
//解锁
pthread_mutex_unlock(&mutex);
break;
}
//解锁
pthread_mutex_unlock(&mutex);
}
//解锁
//pthread_mutex_unlock(&mutex);
}
int main(){
//初始化互斥量
pthread_mutex_init(&mutex,NULL);
//创建3个子线程
pthread_t tid1,tid2,tid3;
pthread_create(&tid1,NULL,selltickets,NULL);
pthread_create(&tid2,NULL,selltickets,NULL);
pthread_create(&tid3,NULL,selltickets,NULL);
//回收子线程的资源,阻塞
pthread_join(tid1,NULL);
pthread_join(tid2,NULL);
pthread_join(tid3,NULL);
//回收子线程资源和设置线程分离 二选一
//设置线程分离
// pthread_detach(tid1);
// pthread_detach(tid2);
// pthread_detach(tid3);
//退出主线程
pthread_exit(NULL);//不影响子线程
//释放互斥量的资源
pthread_mutex_destroy(&mutex);
return 0;
}
10 死锁
第一种情况,忘记释放锁,可以发现程序执行的时候阻塞了,除了第一个进程其他进程都被阻塞进不去。
第二种情况,重复加锁,第一个线程被阻塞在第二个锁外面,其他线程被阻塞在第一个锁外面(所有线程都被阻塞)
第三种情况多线程多锁,抢占锁资源。两个线程,其中一个线程执行worka,另外一个执行workb,当worka给mutex1加锁后,休眠一秒,与此同时,第二个函数,workb给mutex2加锁,休眠一秒,worka再给mutex2加锁,发现2被其他线程(b)加锁了,a不能给他加锁了,b想给mutex1加锁发现也不行,就这样陷入了僵局。
所以我们在使用互斥量的时候,要避免产生死锁。
11 读写锁
读写锁:读的时候可以多个线程同时读,但是如果有线程进行写操作,则其他线程的读,写要停止
/*
读写锁的类型 pthread_rwlock_t
int pthread_rwlock_init(pthread_rwlock_t *restrict rwlock, const pthread_rwlockattr_t *restrict attr);
int pthread_rwlock_destroy(pthread_rwlock_t *rwlock);
int pthread_rwlock_rdlock(pthread_rwlock_t *rwlock);
int pthread_rwlock_tryrdlock(pthread_rwlock_t *rwlock);
int pthread_rwlock_wrlock(pthread_rwlock_t *rwlock);
int pthread_rwlock_trywrlock(pthread_rwlock_t *rwlock);
int pthread_rwlock_unlock(pthread_rwlock_t *rwlock);
案例:8个线程操作同一个全局变量。
3个线程不定时写这个全局变量,5个线程不定时的读这个全局变量
*/
#include<stdio.h>
#include<pthread.h>
#include<unistd.h>
//创建一个共享数据
int num = 1;
//互斥锁
pthread_mutex_t mutex;
//读写锁
pthread_rwlock_t rwlock;
void *writeNum(void * arg){
while(1){
//pthread_mutex_lock(&mutex);
pthread_rwlock_wrlock(&rwlock);
num++;
printf("----write,tid : %ld, num : %d \n",pthread_self(),num);
//pthread_mutex_unlock(&mutex);
pthread_rwlock_unlock(&rwlock);
usleep(100);
}
}
void *readNum(void * arg){
while(1){
//pthread_mutex_lock(&mutex);
pthread_rwlock_rdlock(&rwlock);
printf("+++++read, tid : %ld, num : %d\n",pthread_self(),num);
//pthread_mutex_unlock(&mutex);
pthread_rwlock_unlock(&rwlock);
usleep(100);
}
}
int main(){
//pthread_mutex_init(&mutex,NULL);
pthread_rwlock_init(&rwlock,NULL);
//创建3个写线程,5个读线程
pthread_t wtids[3],rtids[5];
for(int i=0;i<3;++i){
pthread_create(&wtids[i],NULL,writeNum,NULL);
}
for(int i=0;i<5;++i){
pthread_create(&rtids[i],NULL,readNum,NULL);
}
//设置线程分离
for(int i=0;i<3;++i){
pthread_detach(wtids[i]);
}
for(int i=0;i<5;++i){
pthread_detach(rtids[i]);
}
//pthread_mutex_destroy(&mutex);
pthread_rwlock_destroy(&rwlock);
//退出主线程
pthread_exit(NULL);
return 0;
}
12 生产者和消费者模型
生产者消费者粗略版本(有错误,未改,下面的视频里面会改)
/*
生产者消费者模型(粗略的版本)
*/
#include<stdio.h>
#include<pthread.h>
#include<stdlib.h>
#include<unistd.h>
struct Node{
int num;
struct Node *next;
};
//头结点
struct Node *head = NULL;
void *producer(void *arg){
//不断创建新的结点,添加到链表中(生产者)
while(1){
struct Node *newNode = (struct Node *)malloc(sizeof(struct Node));
newNode->next = head;
head = newNode;
newNode->num = rand()%1000;
printf("add node , num : %d ,tid : %ld\n",newNode->num,pthread_self());
usleep(100);
}
return NULL;
}
void *customer(void *arg){
while(1){
//保存头结点的指针
struct Node *temp = head;
head = head->next;
printf("del node , num : %d ,tid : %ld\n",temp->num,pthread_self());
free(temp);
usleep(100);
}
}
int main(){
//创建5个生产者线程, 5个消费者线程
pthread_t ptids[5],ctids[5];
for(int i=0; i<5; ++i){
pthread_create(&ptids[i],NULL,producer,NULL);
pthread_create(&ctids[i],NULL,customer,NULL);
}
//线程分离
for(int i=0;i<5;++i){
pthread_detach(ptids[i]);
pthread_detach(ctids[i]);
}
//线程退出,退出主线程,就不会执行return 0?
pthread_exit(NULL);
return 0;
}
13 条件变量
条件变量不是锁,不能解决数据混乱的问题。是配合互斥锁或者读写锁使用的。
/*
条件变量的类型 pthread_cond_t
int pthread_cond_init(pthread_cond_t *restrict cond, const pthread_condattr_t *restrict attr);
int pthread_cond_destroy(pthread_cond_t *cond);
int pthread_cond_wait(pthread_cond_t *restrict cond, pthread_mutex_t *restrict mutex);
- 等待,调用了该函数,线程会阻塞。
int pthread_cond_timedwait(pthread_cond_t *restrict cond, pthread_mutex_t *restrict mutex, const struct timespec *restrict abstime);
- 等待多长时间,调用了这个函数,线程会阻塞,直到指定的时间结束。
int pthread_cond_signal(pthread_cond_t *cond);
- 唤醒一个或者多个等待的线程
int pthread_cond_broadcast(pthread_cond_t *cond);
- 唤醒所有的等待的线程
*/
/*
生产者消费者模型(粗略的版本)
*/
#include<stdio.h>
#include<pthread.h>
#include<stdlib.h>
#include<unistd.h>
//创建一个互斥量解决数据不同步问题
pthread_mutex_t mutex;
//创建条件变量
pthread_cond_t cond;
struct Node{
int num;
struct Node *next;
};
//头结点
struct Node *head = NULL;
void *producer(void *arg){
//不断创建新的结点,添加到链表中(生产者)
while(1){
pthread_mutex_lock(&mutex);
struct Node *newNode = (struct Node *)malloc(sizeof(struct Node));
newNode->next = head;
head = newNode;
newNode->num = rand()%1000;
printf("add node , num : %d ,tid : %ld\n",newNode->num,pthread_self());
//只要生产了一个,就通知消费者
pthread_cond_signal(&cond);
pthread_mutex_unlock(&mutex);
usleep(100);
}
return NULL;
}
void *customer(void *arg){
while(1){
pthread_mutex_lock(&mutex);
//保存头结点的指针
struct Node *temp = head;
//判断是否有数据
if(head != NULL){
head = head->next;
printf("del node , num : %d ,tid : %ld\n",temp->num,pthread_self());
free(temp);
pthread_mutex_unlock(&mutex);
usleep(100);
}else{
//没有数据,需要等待
//当生产者执行了pthread_cond_signal,wait就会被唤醒
// 当wait这个函数调用阻塞的时候,会对互斥锁进行解锁,当不阻塞时,继续向下执行,会重新加锁
pthread_cond_wait(&cond,&mutex);
pthread_mutex_unlock(&mutex);
}
}
}
int main(){
pthread_mutex_init(&mutex,NULL);
pthread_cond_init(&cond,NULL);
//创建5个生产者线程, 5个消费者线程
pthread_t ptids[5],ctids[5];
for(int i=0; i<5; ++i){
pthread_create(&ptids[i],NULL,producer,NULL);
pthread_create(&ctids[i],NULL,customer,NULL);
}
//线程分离
for(int i=0;i<5;++i){
pthread_detach(ptids[i]);
pthread_detach(ctids[i]);
}
while(1){
sleep(10);
}
//destroy写在exit后面会不执行,写在前面,线程分离之后就销毁,没有意义,解决办法写一个for循环让他睡眠
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
//线程退出,退出主线程,就不会执行return 0?
pthread_exit(NULL);
return 0;
}
生产者消费者遇到的问题在这边得以解决
14 信号量
信号量(信号灯)做的事情与条件变量类似,都是用来阻塞线程。
信号灯,顾名思义,灯一共有两种状态,灯亮表明资源可用,灯灭表明资源不可用。
使用信号量实现生产者和消费者模型
/*
信号量的类型 sem_t
int sem_init(sem_t *sem, int pshared, unsigned int value);
- 初始化信号量
- 参数:
- sem : 信号量变量的地址
- pshared : 0 用在线程间 ,非0 用在进程间
- value : 信号量中的值
int sem_destroy(sem_t *sem);
- 释放资源
int sem_wait(sem_t *sem);
- 对信号量加锁,调用一次对信号量的值-1,如果值为0,就阻塞
int sem_trywait(sem_t *sem);
int sem_timedwait(sem_t *sem, const struct timespec *abs_timeout);
int sem_post(sem_t *sem);
- 对信号量解锁,调用一次对信号量的值+1
int sem_getvalue(sem_t *sem, int *sval);
sem_t psem;
sem_t csem;
init(psem, 0, 8);
init(csem, 0, 0);
producer() {
sem_wait(&psem);
sem_post(&csem)
}
customer() {
sem_wait(&csem);
sem_post(&psem)
}
*/
#include<stdio.h>
#include<pthread.h>
#include<stdlib.h>
#include<unistd.h>
#include<semaphore.h>
//创建一个互斥量解决数据不同步问题
pthread_mutex_t mutex;
//创建信号量
sem_t psem,csem;
struct Node{
int num;
struct Node *next;
};
//头结点
struct Node *head = NULL;
void *producer(void *arg){
//不断创建新的结点,添加到链表中(生产者)
while(1){
sem_wait(&psem);//调用一次,psem值会减一
pthread_mutex_lock(&mutex);
struct Node *newNode = (struct Node *)malloc(sizeof(struct Node));
newNode->next = head;
head = newNode;
newNode->num = rand()%1000;
printf("add node , num : %d ,tid : %ld\n",newNode->num,pthread_self());
pthread_mutex_unlock(&mutex);
sem_post(&csem);//调用一次,csem值会加一
usleep(100);
}
return NULL;
}
void *customer(void *arg){
while(1){
sem_wait(&csem);//生产者生产之后,上面的sem_post会给csem加一,表明容器里面有一个数据,wait调用之后会减一,表示消费一个数据
pthread_mutex_lock(&mutex);
//保存头结点的指针
struct Node *temp = head;
head = head->next;
printf("del node , num : %d ,tid : %ld\n",temp->num,pthread_self());
free(temp);
pthread_mutex_unlock(&mutex);
sem_post(&psem);//对生产者信号量的值加一
usleep(100);
}
}
int main(){
pthread_mutex_init(&mutex,NULL);
sem_init(&psem,0,8);
sem_init(&csem,0,0);
//创建5个生产者线程, 5个消费者线程
pthread_t ptids[5],ctids[5];
for(int i=0; i<5; ++i){
pthread_create(&ptids[i],NULL,producer,NULL);
pthread_create(&ctids[i],NULL,customer,NULL);
}
//线程分离
for(int i=0;i<5;++i){
pthread_detach(ptids[i]);
pthread_detach(ctids[i]);
}
while(1){
sleep(10);
}
//destroy写在exit后面会不执行,写在前面,线程分离之后就销毁,没有意义,解决办法写一个for循环让他睡眠
pthread_mutex_destroy(&mutex);
sem_destroy(&csem);
sem_destroy(&psem);
//线程退出,退出主线程,就不会执行return 0?
pthread_exit(NULL);
return 0;
}