#include<graphics.h>
#include<cstdio>
#include<cstring>
#include<cstdlib>
#include<iostream>
#include<string>
#include<vector>
using namespace std;
#define SCREENWIDTH GetSystemMetrics(SM_CXSCREEN)
#define SCREENHEIGHT GetSystemMetrics(SM_CYSCREEN)
#define SHOWMAPLINE 1
struct BlockDataNode {
int x, y;//块坐标
int sideStatus[4] = { 0,0,0,0 };//上下左右
};
class MAP {
public:
int** matrix;
void showMatrix(void) {
for (int i = 0; i < _widthNum; ++i) {
for (int j = 0; j < _heightNum; ++j) {
printf(" %d ", matrix[i][j]);
}
printf("\n");
}
}
void initView(int width, int height) {
_width = width;
_height = height;
initgraph(_width, _height, EW_SHOWCONSOLE);
setbkcolor(RGB(255, 255, 255));
// 用背景色清空屏幕
cleardevice();
}
void drawLine(int width_num, int height_num) {
//初始化矩阵
matrix = (int**)malloc(sizeof(int*) * width_num);
for (int i = 0; i < width_num; ++i) {
*(matrix + i) = (int*)malloc(sizeof(int) * height_num);
}
for (int i = 0; i < width_num; ++i) {
for (int j = 0; j < height_num; ++j) {
matrix[i][j] = 0;
}
}
_widthNum = width_num;
_heightNum = height_num;
_widthStep = (float)_width / (float)height_num;
_heightStep = (float)_height / (float)width_num;
//画竖线
setlinecolor(RGB(0, 0, 0));
float x_now = 0, y_now = 0;
while (x_now <= _width) {
if (SHOWMAPLINE)
line(x_now, 0, x_now, _height);
x_now += _widthStep;
}
//画横线
while (y_now <= _height) {
if (SHOWMAPLINE)
line(0, y_now, _width, y_now);
y_now += _heightStep;
}
}
void setBlockColor(int x, int y, int r, int g, int b, int matrix_num) {
if (SHOWMAPLINE == 0)
setlinecolor(RGB(r, g, b));
setfillcolor(RGB(r, g, b));
fillrectangle(y * _widthStep, x * _heightStep, y * _widthStep + _widthStep, x * _heightStep + _heightStep);
this->matrix[x][y] = matrix_num;
}
void findRoad(class MAP& map, vector<struct BlockDataNode>& BlockStack, struct BlockDataNode& endNode);
void showPrivate(void) {
cout << "_width" << _width << endl;
cout << "_height" << _height << endl;
cout << "_widthStep" << _widthStep << endl;
cout << "_heightStep" << _heightStep << endl;
cout << "_widthNum" << _widthNum << endl;
cout << "_heightNum" << _heightNum << endl;
}
private:
int _width;
int _height;
float _widthStep;
float _heightStep;
int _widthNum;
int _heightNum;
};
void MAP::findRoad(class MAP& map, vector<struct BlockDataNode>& BlockStack, struct BlockDataNode& endNode) {
//栈为空或者栈顶的块是终点则结束
if (BlockStack.size() == 0 || (BlockStack[BlockStack.size() - 1].x == endNode.x && BlockStack[BlockStack.size() - 1].y == endNode.y)) {
printf("yes\n");
return;
}
//迭代现在位置的四个方向
for (int i = 0; i < 4; ++i) {
//如果方向状态都为1,则将其出栈,并把颜色改为原来的色,break
if (BlockStack[BlockStack.size() - 1].sideStatus[0] == 1 && BlockStack[BlockStack.size() - 1].sideStatus[1] == 1 && BlockStack[BlockStack.size() - 1].sideStatus[2] == 1 && BlockStack[BlockStack.size() - 1].sideStatus[3] == 1) {
map.setBlockColor(BlockStack[BlockStack.size() - 1].x, BlockStack[BlockStack.size() - 1].y, 255, 255, 255, 2);
//map.showMatrix();
//BlockStack.erase(BlockStack.end());
printf("del--");
cout << BlockStack[BlockStack.size() - 1].x << "," << BlockStack[BlockStack.size() - 1].y << endl;
BlockStack.pop_back();
MAP::findRoad(map, BlockStack, endNode);
printf("del over\n");
return;
}
Sleep(3);
//判断方向上点是否可以走
if (i == 0 && BlockStack[BlockStack.size() - 1].sideStatus[0] == 0) {//上
if (BlockStack[BlockStack.size() - 1].x >= 1) {//坐标限制
int x = BlockStack[BlockStack.size() - 1].x - 1;
int y = BlockStack[BlockStack.size() - 1].y;
cout << "[" << x << "," << y << "]" << endl;
if (map.matrix[x][y] == 0) {//墙限制
//将图像变红,将矩阵点改为2
map.setBlockColor(x, y, 255, 0, 0, 2);
printf("上\n");
//map.showMatrix();
BlockStack[BlockStack.size() - 1].sideStatus[i] = 1;
//建立一个新的节点入栈
struct BlockDataNode newNode;
newNode.x = x;
newNode.y = y;
if (newNode.x == endNode.x && newNode.y == endNode.y) {
printf("***************************************************************************\n");
exit(0);
return;
}
else
BlockStack.push_back(newNode);
//递归
MAP::findRoad(map, BlockStack, endNode);
}
else {
BlockStack[BlockStack.size() - 1].sideStatus[i] = 1;
}
}
else {
BlockStack[BlockStack.size() - 1].sideStatus[i] = 1;
}
}
else if (i == 1 && BlockStack[BlockStack.size() - 1].sideStatus[1] == 0) {//下
if (BlockStack[BlockStack.size() - 1].x < map._widthNum - 1) {//坐标限制
int x = BlockStack[BlockStack.size() - 1].x + 1;
int y = BlockStack[BlockStack.size() - 1].y;
cout << "[" << x << "," << y << "]" << endl;
if (map.matrix[x][y] == 0) {//墙限制
//将图像变红,将矩阵点改为2
map.setBlockColor(x, y, 255, 0, 0, 2);
printf("下\n");
//map.showMatrix();
BlockStack[BlockStack.size() - 1].sideStatus[i] = 1;
//建立一个新的节点入栈
struct BlockDataNode newNode;
newNode.x = x;
newNode.y = y;
if (newNode.x == endNode.x && newNode.y == endNode.y) {
printf("***************************************************************************\n");
exit(0);
return;
}
else
BlockStack.push_back(newNode);
//递归
MAP::findRoad(map, BlockStack, endNode);
}
else {
BlockStack[BlockStack.size() - 1].sideStatus[i] = 1;
}
}
else {
BlockStack[BlockStack.size() - 1].sideStatus[i] = 1;
}
}
else if (i == 2 && BlockStack[BlockStack.size() - 1].sideStatus[2] == 0) {//左
if (BlockStack[BlockStack.size() - 1].y >= 1) {//坐标限制
int x = BlockStack[BlockStack.size() - 1].x;
int y = BlockStack[BlockStack.size() - 1].y - 1;
cout << "[" << x << "," << y << "]" << endl;
if (map.matrix[x][y] == 0) {//墙限制
//将图像变红,将矩阵点改为2
printf("左\n");
map.setBlockColor(x, y, 255, 0, 0, 2);
//map.showMatrix();
BlockStack[BlockStack.size() - 1].sideStatus[i] = 1;
//建立一个新的节点入栈
struct BlockDataNode newNode;
newNode.x = x;
newNode.y = y;
if (newNode.x == endNode.x && newNode.y == endNode.y) {
printf("***************************************************************************\n");
exit(0);
return;
}
else
BlockStack.push_back(newNode);
//递归
MAP::findRoad(map, BlockStack, endNode);
}
else {
BlockStack[BlockStack.size() - 1].sideStatus[i] = 1;
}
}
else {
BlockStack[BlockStack.size() - 1].sideStatus[i] = 1;
}
}
else if (i == 3 && BlockStack[BlockStack.size() - 1].sideStatus[3] == 0) {//右
if (BlockStack[BlockStack.size() - 1].y < map._heightNum - 1) {//坐标限制
int x = BlockStack[BlockStack.size() - 1].x;
int y = BlockStack[BlockStack.size() - 1].y + 1;
cout << "[" << x << "," << y << "]" << endl;
if (map.matrix[x][y] == 0) {//墙限制
printf("右\n");
//将图像变红,将矩阵点改为2
map.setBlockColor(x, y, 255, 0, 0, 2);
//map.showMatrix();
BlockStack[BlockStack.size() - 1].sideStatus[i] = 1;
//建立一个新的节点入栈
struct BlockDataNode newNode;
newNode.x = x;
newNode.y = y;
if (newNode.x == endNode.x && newNode.y == endNode.y) {
printf("***************************************************************************\n");
exit(0);
return;
}
else
BlockStack.push_back(newNode);
//递归
MAP::findRoad(map, BlockStack, endNode);
}
else {
BlockStack[BlockStack.size() - 1].sideStatus[i] = 1;
}
}
else {
BlockStack[BlockStack.size() - 1].sideStatus[i] = 1;
}
}
}
//如果方向状态都为1,则将其出栈,并把颜色改为原来的色,break
if (BlockStack[BlockStack.size() - 1].sideStatus[0] == 1 && BlockStack[BlockStack.size() - 1].sideStatus[1] == 1 && BlockStack[BlockStack.size() - 1].sideStatus[2] == 1 && BlockStack[BlockStack.size() - 1].sideStatus[3] == 1) {
map.setBlockColor(BlockStack[BlockStack.size() - 1].x, BlockStack[BlockStack.size() - 1].y, 255, 255, 255, 2);
//map.showMatrix();
//BlockStack.erase(BlockStack.end());
printf("del--");
cout << BlockStack[BlockStack.size() - 1].x << "," << BlockStack[BlockStack.size() - 1].y << endl;
BlockStack.pop_back();
MAP::findRoad(map, BlockStack, endNode);
printf("del over\n");
return;
}
}
int main(int argc, char** argv) {
MAP map;
map.initView(500, 500);
map.drawLine(30, 30);
//画墙
map.setBlockColor(2, 1, 0, 0, 0, 1);
map.setBlockColor(2, 2, 0, 0, 0, 1);
map.setBlockColor(2, 3, 0, 0, 0, 1);
map.setBlockColor(2, 4, 0, 0, 0, 1);
map.setBlockColor(2, 5, 0, 0, 0, 1);
map.setBlockColor(2, 6, 0, 0, 0, 1);
map.setBlockColor(2, 7, 0, 0, 0, 1);
map.setBlockColor(2, 8, 0, 0, 0, 1);
map.setBlockColor(2, 6, 0, 0, 0, 1);
map.setBlockColor(2, 15, 0, 0, 0, 1);
map.setBlockColor(2, 17, 0, 0, 0, 1);
map.setBlockColor(4, 2, 0, 0, 0, 1);
map.setBlockColor(4, 0, 0, 0, 0, 1);
map.setBlockColor(5, 4, 0, 0, 0, 1);
map.setBlockColor(4, 5, 0, 0, 0, 1);
map.setBlockColor(5, 6, 0, 0, 0, 1);
map.setBlockColor(6, 7, 0, 0, 0, 1);
map.setBlockColor(4, 8, 0, 0, 0, 1);
map.setBlockColor(12, 8, 0, 0, 0, 1);
map.setBlockColor(12, 6, 0, 0, 0, 1);
map.setBlockColor(14, 15, 0, 0, 0, 1);
map.setBlockColor(12, 17, 0, 0, 0, 1);
map.setBlockColor(14, 4, 0, 0, 0, 1);
map.setBlockColor(12, 4, 0, 0, 0, 1);
map.setBlockColor(12, 5, 0, 0, 0, 1);
map.setBlockColor(12, 5, 0, 0, 0, 1);
map.setBlockColor(19, 4, 0, 0, 0, 1);
map.setBlockColor(19, 2, 0, 0, 0, 1);
map.setBlockColor(4, 12, 0, 0, 0, 1);
map.setBlockColor(4, 10, 0, 0, 0, 1);
map.setBlockColor(5, 14, 0, 0, 0, 1);
map.setBlockColor(4, 15, 0, 0, 0, 1);
map.setBlockColor(5, 16, 0, 0, 0, 1);
map.setBlockColor(6, 17, 0, 0, 0, 1);
map.setBlockColor(4, 18, 0, 0, 0, 1);
map.setBlockColor(12,18, 0, 0, 0, 1);
map.setBlockColor(12, 16, 0, 0, 0, 1);
map.setBlockColor(14, 15, 0, 0, 0, 1);
map.setBlockColor(12, 17, 0, 0, 0, 1);
map.setBlockColor(14, 14, 0, 0, 0, 1);
map.setBlockColor(12, 14, 0, 0, 0, 1);
map.setBlockColor(12, 15, 0, 0, 0, 1);
map.setBlockColor(12, 15, 0, 0, 0, 1);
map.setBlockColor(19, 14, 0, 0, 0, 1);
map.setBlockColor(19, 12, 0, 0, 0, 1);
vector<struct BlockDataNode>BlockStack;
struct BlockDataNode startNode;
struct BlockDataNode endNode;
startNode.x = 0;
startNode.y = 0;
map.setBlockColor(0, 0, 255, 0, 0, 1);//start block
endNode.x = 18;
endNode.y = 18;
map.setBlockColor(18, 18, 0, 255, 0, 0);//end block
//将起点入栈
map.setBlockColor(startNode.x, startNode.y, 255, 0, 0, 2);//end block
BlockStack.push_back(startNode);
map.findRoad(map, BlockStack, endNode);
map.showPrivate();
map.showMatrix();//矩阵显示
printf("程序结束\n");
char temp = getchar();
return 0;
}
迷宫问题 C++图形界面运行
最新推荐文章于 2021-05-24 15:00:29 发布
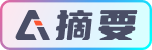