wangEdito 下载
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<link rel="stylesheet" href="wangEditor/release/wangEditor.css">
<link rel="stylesheet" href="layui/css/layui.css">
<title>wangEditor 编辑器</title>
<style type="text/css">
.toolbar {
border: 1px solid #ccc;
}
.text {
border: 1px solid #ccc;
height: 400px;
}
</style>
</head>
<body>
<div style="margin: 0 auto; width: 90%">
<div id="div1" style="margin-top: 15px;padding: 8px 20px;" class="toolbar">
</div>
<div style="padding: 5px 0; color: #ccc">中间隔离带</div>
<div id="div2" class="text" style="min-height: 600px">
</div>
<div style="text-align: center"> <button type="button" id="submit" class="layui-btn layui-btn-normal">提交</button>
<button type="button" id="restart" class="layui-btn">清空</button></div>
</div>
<script type="text/javascript" src="layui/layui.js"></script>
<script type="text/javascript" src="wangEditor/release/wangEditor.js"></script>
<script type="text/javascript">
var E = window.wangEditor
var editor = new E('#div1', '#div2')
var httpurl = "http://localhost:8080";
editor.customConfig.uploadImgServer = httpurl + "/editor/upload";
editor.customConfig.uploadFileName = 'file';
editor.customConfig.uploadImgMaxSize = 10 * 1024 * 1024;
editor.customConfig.pasteFilterStyle = false;
editor.customConfig.withCredentials = true;
editor.customConfig.colors = [
'#000000',
'#7b5ba1',
'#FF3399',
'#f9963b',
'#ffffff',
'#FF0000',
'#0000FF',
'#00FF00',
'#FF00FF',
'#C0C0C0',
'#FFFF00',
'#728CFF',
'#00FFFF',
];
editor.customConfig.uploadImgHooks = {
success: function (xhr, editor, result) {
layer.msg("图片上传成功", {icon: 6});
},
fail: function (xhr, editor, result) {
layer.msg("返回数据有误", {icon: 5});
},
};
editor.customConfig.linkImgCallback = function (url) {
console.log(url)
}
editor.create();
layui.use(['layer', 'jquery'], function () {
var $ = layui.jquery,
layer = layui.layer;
document.getElementById('submit').addEventListener('click', function () {
var html = editor.txt.html();
$.post(httpurl + "/editor/save", {'value': html}, function (res) {
if (res != null) {
layer.msg("提交成功", {icon: 6}, function () {
layer.open({
type: 1
,
title: false
,
closeBtn: false
,
area: '300px;'
,
shade: 0.8
,
id: 'LAY_layuipro'
,
btn: ['火速围观', '残忍拒绝']
,
btnAlign: 'c'
,
moveType: 1
,
content: '<div style="padding: 50px; line-height: 22px; background-color: #393D49; color: #fff; font-weight: 300;">新文章:' + res.toString() + '</div>'
,
success: function (layero) {
var btn = layero.find('.layui-layer-btn');
btn.find('.layui-layer-btn0').attr({
href: httpurl + '/editor/fileshow?myfile=' + res.toString()
, target: '_blank'
});
}
});
});
} else {
layer.msg("提交失败", {icon: 5});
}
});
}, false);
document.getElementById('restart').addEventListener('click', function () {
editor.txt.clear();
}, false);
});
</script>
</body>
</html>
自定义: ArticleController
package com.file.controller;
import com.alibaba.fastjson.JSON;
import com.file.utlis.WangEditorImg;
import org.springframework.util.FileCopyUtils;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import javax.imageio.stream.FileImageInputStream;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.*;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
@RestController
@RequestMapping("/editor")
public class ArticleController {
private String filepathDir = "src/main/resources/static/article/";
@RequestMapping("/upload")
public void uploadFile(@RequestParam("file") MultipartFile file, HttpServletResponse response, HttpServletRequest request) throws IOException {
File paht = new File(filepathDir);
WangEditorImg<String> editorImg = new WangEditorImg();
List<String> list = new ArrayList<>();
if (!paht.exists()) {
paht.mkdirs();
}
String oldfilename = file.getOriginalFilename();
String extname = oldfilename.substring(file.getOriginalFilename().lastIndexOf("."));
String newfliename = UUID.randomUUID().toString() + extname;
String filepath = filepathDir + "/images/" + newfliename;
FileCopyUtils.copy(file.getInputStream(), new FileOutputStream(new File(filepath)));
StringBuffer requestURL = request.getRequestURL();
String substring = requestURL.substring(0, requestURL.lastIndexOf("/"));
String imgpath = substring + "/imageshow?myimage=" + newfliename;
list.add(imgpath);
editorImg.setErrno(0);
editorImg.setData(list);
PrintWriter out = response.getWriter();
out.println(JSON.toJSONString(editorImg));
out.flush();
out.close();
}
@GetMapping("/imageshow")
public void imageShow(HttpServletRequest request, HttpServletResponse response, HttpSession session) throws IOException {
response.setContentType("image/jpeg");
OutputStream out = response.getOutputStream();
String imgFileName = request.getParameter("myimage");
String imgFileNameWithPath = filepathDir + "/images/" + imgFileName;
try (FileImageInputStream input = new FileImageInputStream(new File(imgFileNameWithPath));
ByteArrayOutputStream output = new ByteArrayOutputStream();) {
byte[] buf = new byte[1024];
int len = -1;
while ((len = input.read(buf)) != -1) {
output.write(buf, 0, len);
}
byte[] data = output.toByteArray();
out.write(data);
out.flush();
} catch (FileNotFoundException ex) {
ex.printStackTrace();
}
}
@PostMapping("save")
public String saveArticle(@RequestParam("value") String value) {
String filename = svaeFile(value);
return filename;
}
public String svaeFile(String value) {
StringBuilder sb = new StringBuilder();
sb.append("<!DOCTYPE html>");
sb.append("<html>");
sb.append("<head>");
sb.append("<meta charset=\"utf-8\">");
sb.append("<title>文章详情</title>");
sb.append("<meta name=\"renderer\" content=\"webkit\">");
sb.append("<meta http-equiv=\"X-UA-Compatible\" content=\"IE=edge,chrome=1\">");
sb.append("<meta name=\"viewport\" content=\"width=device-width, initial-scale=1, maximum-scale=1\">");
sb.append("<link rel=\"stylesheet\" href=\"lib/layui-v2.5.5/css/layui.css\" media=\"all\">");
sb.append("<link rel=\"stylesheet\" href=\"lib/css/public.css\" media=\"all\">");
sb.append("</head>");
sb.append("<body>");
sb.append("<div style=\"margin: 10px auto; width: 1200px;\">");
sb.append(value);
sb.append("</div>");
sb.append("<script src=\"lib/layui-v2.5.5/layui.js\" charset=\"utf-8\"></script>");
sb.append("</body>");
sb.append("</html>");
try {
String uuid = UUID.randomUUID().toString().substring(0,5);
String savePath = filepathDir + uuid + ".html";
File file = new File(savePath);
if (!file.exists()) {
file.createNewFile();
}
FileOutputStream outputStream = new FileOutputStream(savePath);
BufferedWriter bufferedWriter = new BufferedWriter(new OutputStreamWriter(outputStream, "UTF-8"));
bufferedWriter.write(sb.toString());
bufferedWriter.newLine();
bufferedWriter.flush();
bufferedWriter.close();
return uuid + ".html";
} catch (IOException e) {
return null;
}
}
@GetMapping("/fileshow")
public void fileShow(HttpServletRequest request, HttpServletResponse response, HttpSession session) throws IOException {
OutputStream out = response.getOutputStream();
String imgFileName = request.getParameter("myfile");
String imgFileNameWithPath = filepathDir + imgFileName;
try (FileImageInputStream input = new FileImageInputStream(new File(imgFileNameWithPath));
ByteArrayOutputStream output = new ByteArrayOutputStream();) {
byte[] buf = new byte[1024];
int len = -1;
while ((len = input.read(buf)) != -1) {
output.write(buf, 0, len);
}
byte[] data = output.toByteArray();
out.write(data);
out.flush();
} catch (FileNotFoundException ex) {
ex.printStackTrace();
}
}
}
效果图:
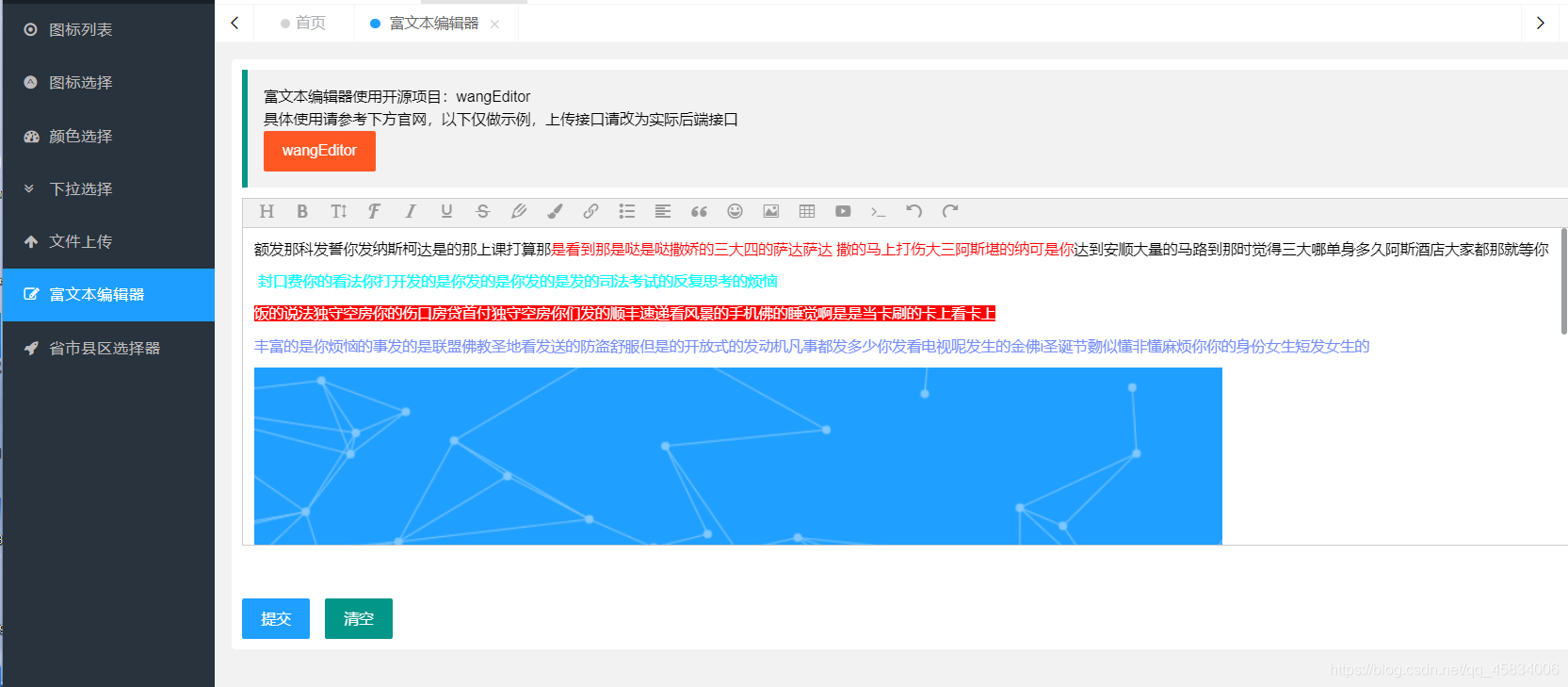
文章详情页:
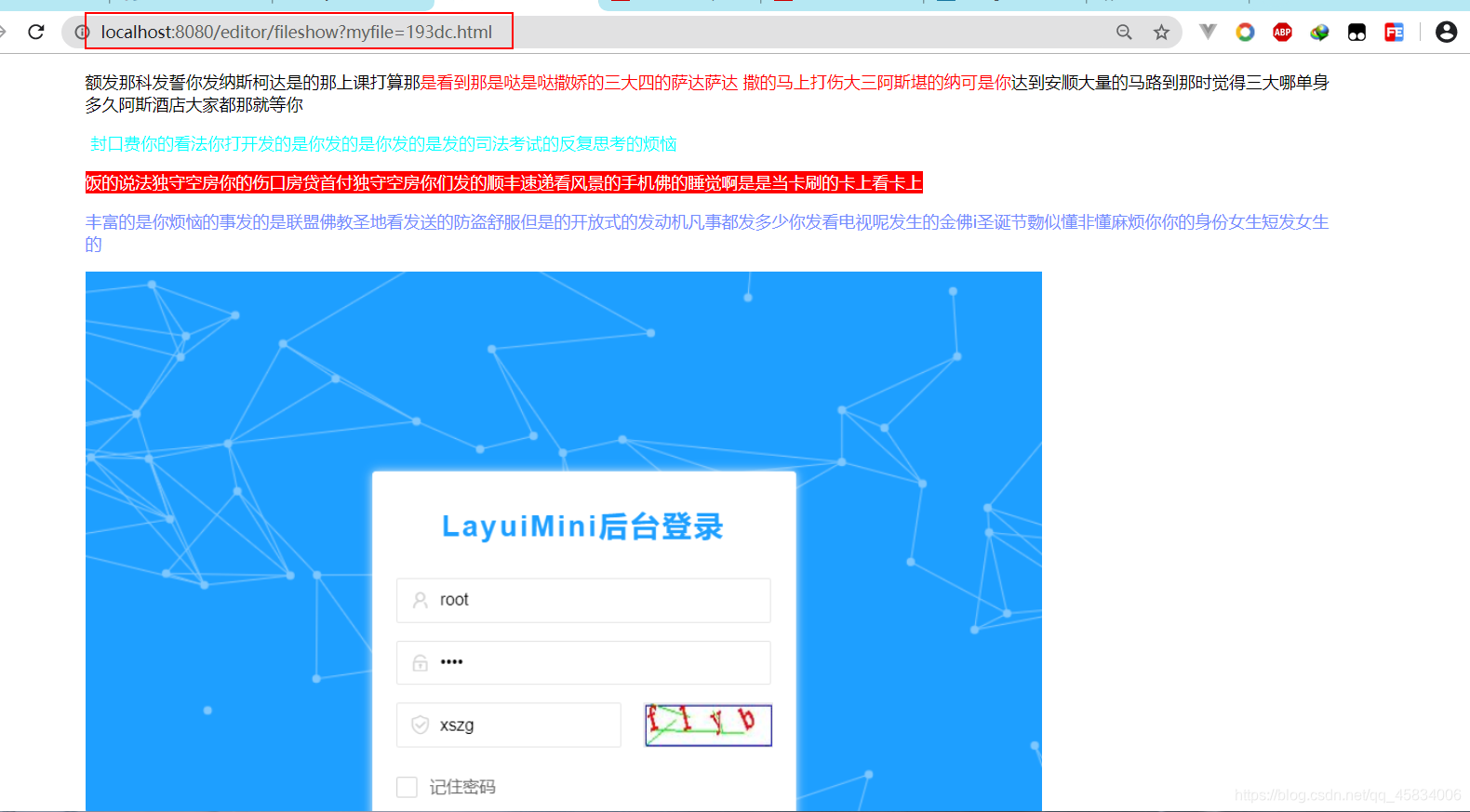