一、链表概述
- 链表是一种物理存储结构上非连续,非顺序的存储结构,数据元素的逻辑顺序是通过链表中的指针链接次序实现的。
- 与数组区别:
链表是链式的存储结构,数组是顺序的存储结构。
链表通过指针来连接元素与元素,数组则是把所有元素按次序依次存储。
优缺点如图:
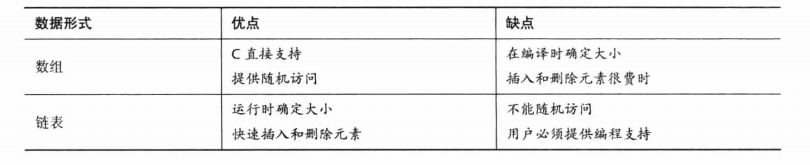
二、创建链表前的准备工作
1.结点构成
- 数据域:存放用户需要的实际数据
- 指针域:存放下一个结点的地址
2.malloc函数
- 函数原型:void *malloc(unsigned int size);
- 功能:在内存的动态存储区申请一个长度为size字节的连续存储空间,并返回该存储空间的起始地址。如果没有足够的内存空间可分配,则函数的返回值为空指针NULL。
- 说明:这个函数返回的是个void类型指针,所以在使用时应注意强制类型转换成需要的指针类型。
3.free函数
- 函数原型:void free(void *p);
- 功能:将指针变量p指向的存储空间释放,交换给系统。
三、创建链表
1.尾插法
- 特点:输入顺序(存储顺序)与输出顺序相同
- 图解:
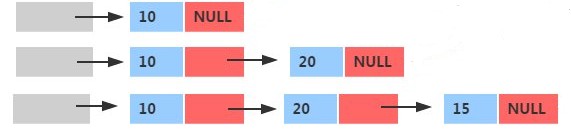
- 核心代码
end->next = p;
end = p;
2.头插法
- 特点:输入顺序(存储顺序)与输出顺序相反
- 图解:

- 核心代码:
p->next = head->next;
head->next = p;
3.完整代码
struct node *creat() {
struct node *head= NULL, *end = NULL, *p = NULL;
head = (struct node *)malloc(sizeof(strcut node));
end = head;
head->next = NULL