Stack.h
#pragma onece
#include <stdio.h>
#include <assert.h>
typedef int STDataType;
typedef struct Stack
{
STDataType *a;
int top;
int capacity;
}Stack;
void StackInit(Stack *pst);
void StackDestory(Stack *pst);
void StackPush(Stack *pst, STDataType x);
void StackPop(Stack *pst);
int StackSize(Stack *pst);
int StackEmpty(Stack *pst);
STDataType StackTop(Stack *pst);
Stack.c
#include "Stack.h"
void StackInit(Stack *pst)
{
assert(pst);
pst->a = (STDataType *)malloc(sizeof(STDataType)* 4);
if (pst->a == NULL)
{
printf("malloc fail\n");
exit(-1);
}
pst->capacity = 4;
pst->top = 0;
}
void StackDestory(Stack *pst)
{
assert(pst);
free(pst->a);
pst->a = NULL;
pst->top = pst->capacity = 0;
}
void StackPush(Stack *pst, STDataType x)
{
assert(pst);
if (pst->top == pst->capacity)
{
STDataType *tmp = realloc(pst->a, pst->capacity * 2 * sizeof(STDataType));
if (tmp == NULL)
{
printf("realloc fail\n");
exit(-1);
}
pst->a = tmp;
pst->capacity = pst->capacity * 2;
}
pst->a[pst->top] = x;
pst->top++;
}
void StackPop(Stack *pst)
{
assert(pst);
assert(!StackEmpty(pst));
--pst->top;
}
int StackSize(Stack *pst)
{
assert(pst);
return pst->top;
}
int StackEmpty(Stack *pst)
{
assert(pst);
return pst->top == 0 ? 1 : 0;
}
STDataType StackTop(Stack *pst)
{
assert(pst);
assert(!StackEmpty(pst));
return pst->a[pst->top-1];
}
Test.c
#include "Stack.h"
int main()
{
Stack S;
StackInit(&S);
StackPush(&S, 1);
StackPush(&S, 2);
StackPush(&S, 3);
StackPush(&S, 4);
while (!StackEmpty(&S))
{
printf("%d ", StackTop(&S));
StackPop(&S);
}
printf("\n");
StackDestory(&S);
return 0;
}
测试结果
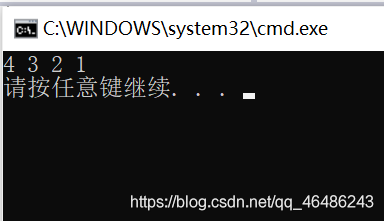