题目:
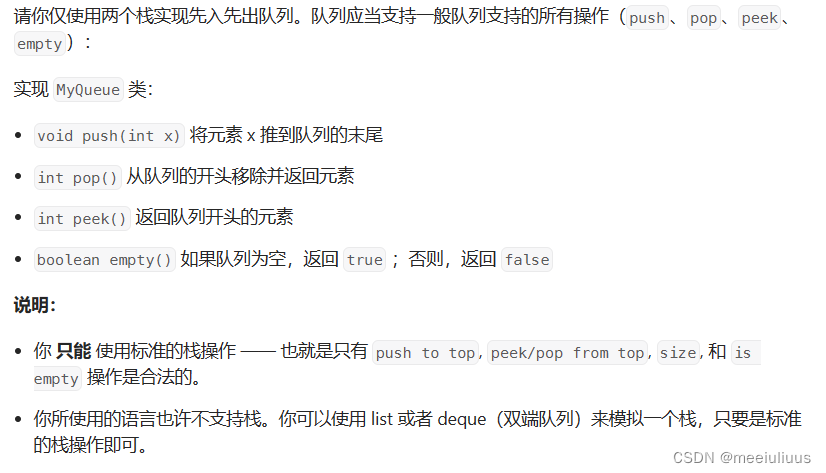
代码(首刷自解 2024年1月18日):
class MyQueue {
private:
stack<int> stack1;
stack<int> stack2;
public:
MyQueue() {
stack<int> stack1;
stack<int> stack2;
}
void push(int x) {
stack1.push(x);
}
int pop() {
while(!stack1.empty()){
stack2.push(stack1.top());
stack1.pop();
}
int a = stack2.top();
stack2.pop();
while(!stack2.empty()){
stack1.push(stack2.top());
stack2.pop();
}
return a;
}
int peek() {
int res = this->pop();
stack2.push(res);
return res;
}
bool empty() {
if(stack1.empty()) return true;
else return false;
}
};
代码(二刷自解 2024年5月4日 10min)
class MyQueue {
private:
stack<int> _stk1;//入栈
stack<int> _stk2;//出栈
void judge() {
if (_stk2.empty()) {
while(!_stk1.empty()) {
_stk2.push(_stk1.top());
_stk1.pop();
}
}
}
public:
MyQueue() {
}
void push(int x) {
_stk1.push(x);
}
int pop() {
judge();
int res = _stk2.top();
_stk2.pop();
return res;
}
int peek() {
judge();
return _stk2.top();
}
bool empty() {
judge();
return _stk2.empty();
}
};
/**
* Your MyQueue object will be instantiated and called as such:
* MyQueue* obj = new MyQueue();
* obj->push(x);
* int param_2 = obj->pop();
* int param_3 = obj->peek();
* bool param_4 = obj->empty();
*/