- 一副扑克牌的每张牌表示一个数(J、Q、K 分别表示 11、12、13,两个司令都表示 6)。任取4 张牌,即得到 4 个 1~13 的数,请添加运算符(规定为加+ 减- 乘* 除/ 四种)使之成为一个运算式。每个数只能参与一次运算,4 个数顺序可以任意组合,4 个运算符任意取 3 个且可以重复取。运算遵从一定优先级别,可加括号控制,最终使运算结果为 24。请输出一种解决方案的表达式,用括号表示运算优先。如果没有一种解决方案,则输出 -1 表示无解。
输入格式:
- 输入在一行中给出 4 个整数,每个整数取值在 [1, 13]。
输出格式:
- 输出任一种解决方案的表达式,用括号表示运算优先。如果没有解决方案,请输出 -1。
输入样例:
2 3 12 12
结尾无空行
输出样例:
((3-2)*12)+12
结尾无空行
WA:
#include <bits/stdc++.h>
using namespace std;
char op[4] = {'+', '-', '*', '/'};
float res(float x,float y,char op)
{
if(op == '+')
{
return x + y;
}
if(op == '-')
{
return x - y;
}
if(op == '*')
{
return x * y;
}
if(op == '/')
{
return x / y;
}
return 0;
}
float module1(float s1,float s2,float s3,float s4,char op1,char op2,char op3)
{
float x, y, z;
x = res(s1, s2, op1);
y = res(x, s3, op2);
z = res(y, s4, op3);
return z;
}
float module2(float s1,float s2,float s3,float s4,char op1,char op2,char op3)
{
float x, y, z;
x = res(s1, s2, op1);
y = res(s3, s4, op3);
z = res(x, y, op2);
return z;
}
float module3(float s1,float s2,float s3,float s4,char op1,char op2,char op3)
{
float x, y, z;
x = res(s2, s3, op2);
y = res(s1, x, op1);
z = res(y, s4, op3);
return z;
}
float module4(float s1,float s2,float s3,float s4,char op1,char op2,char op3)
{
float x, y, z;
x = res(s2, s3, op2);
y = res(x, s4, op3);
z = res(s1, y, op1);
return z;
}
float module5(float s1,float s2,float s3,float s4,char op1,char op2,char op3)
{
float x, y, z;
x = res(s3, s4, op3);
y = res(s2, x, op2);
z = res(s1, y, op1);
return z;
}
int solve(int a,int b,int c,int d)
{
int i, j, k;
int flag = 0;
for (i = 0; i < 4;i++)
{
for (j = 0; j < 4;j++)
{
for (k = 0; k < 4;k++)
{
char op1 = op[i];
char op2 = op[j];
char op3 = op[k];
if(module1(a,b,c,d,op1,op2,op3) == 24)
{
printf("((%d%c%d)%c%d)%c%d\n", a, op1, b, op2, c, op3, d);
flag = 1;
break;
}
if(module2(a,b,c,d,op1,op2,op3) == 24)
{
printf("(%d%c%d)%c(%d%c%d)\n", a, op1, b, op2, c, op3, d);
flag = 1;
break;
}
if(module3(a,b,c,d,op1,op2,op3) == 24)
{
printf("(%d%c(%d%c%d))%c%d\n", a, op1, b, op2, c, op3, d);
flag = 1;
break;
}
if(module4(a,b,c,d,op1,op2,op3) == 24)
{
printf("%d%c((%d%c%d)%c%d)\n", a, op1, b, op2, c, op3, d);
flag = 1;
break;
}
if(module5(a,b,c,d,op1,op2,op3) == 24)
{
printf("%d%c(%d%c(%d%c%d))\n", a, op1, b, op2, c, op3, d);
flag = 1;
break;
}
}
}
}
if(!flag)
{
cout << -1 << endl;
return 1;
}
return 0;
}
int main()
{
int a, b, c, d;
cin >> a >> b >> c >> d;
solve(a, b, c, d);
return 0;
}
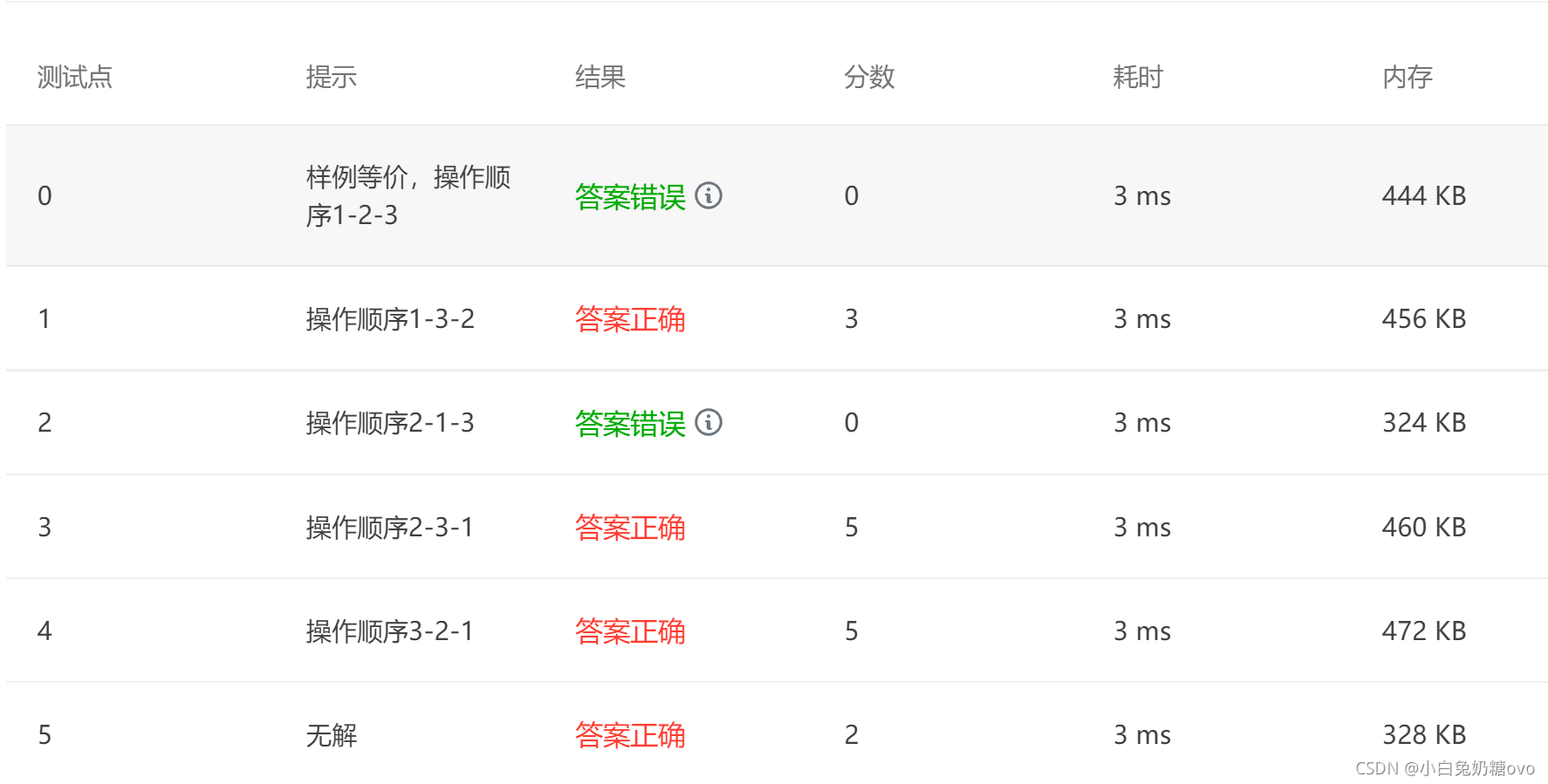
- 出错的原因很简单,直接主观地固定了题目给出的顺序!
AC:
#include <bits/stdc++.h>
using namespace std;
char op[4] = {'+', '-', '*', '/'};
float res(float x, float y, char op)
{
if (op == '+')
return x + y;
if (op == '-')
return x - y;
if (op == '*')
return x * y;
if (op == '/')
return x / y;
}
float module1(float a, float b, float c, float d, char op1, char op2, char op3)
{
float x, y, z;
x = res(a, b, op1);
y = res(x, c, op2);
z = res(y, d, op3);
return z;
}
float module2(float a, float b, float c, float d, char op1, char op2, char op3)
{
float x, y, z;
x = res(b, c, op2);
y = res(a, x, op1);
z = res(y, d, op3);
return z;
}
float module3(float a, float b, float c, float d, char op1, char op2, char op3)
{
float x, y, z;
x = res(c, d, op3);
y = res(b, x, op2);
z = res(a, y, op1);
return z;
}
float module4(float a, float b, float c, float d, char op1, char op2, char op3)
{
float x, y, z;
x = res(b, c, op2);
y = res(x, d, op3);
z = res(a, y, op1);
return z;
}
float module5(float a, float b, float c, float d, char op1, char op2, char op3)
{
float x, y, z;
x = res(a, b, op1);
y = res(c, d, op3);
z = res(x, y, op2);
return z;
}
int solve(int a, int b, int c, int d)
{
int i, j, k;
int flag = 0;
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
for (k = 0; k < 4; k++)
{
char op1 = op[i], op2 = op[j], op3 = op[k];
if (module1(a, b, c, d, op1, op2, op3) == 24)
{
printf("((%d%c%d)%c%d)%c%d", a, op1, b, op2, c, op3, d);
flag = 1;
break;
}
if (module2(a, b, c, d, op1, op2, op3) == 24)
{
printf("(%d%c(%d%c%d))%c%d", a, op1, b, op2, c, op3, d);
flag = 1;
break;
}
if (module3(a, b, c, d, op1, op2, op3) == 24)
{
printf("%d%c(%d%c(%d%c%d))", a, op1, b, op2, c, op3, d);
flag = 1;
break;
}
if (module4(a, b, c, d, op1, op2, op3) == 24)
{
printf("%d%c((%d%c%d)%c%d)", a, op1, b, op2, c, op3, d);
flag = 1;
break;
}
if (module5(a, b, c, d, op1, op2, op3) == 24)
{
printf("(%d%c%d)%c(%d%c%d)", a, op1, b, op2, c, op3, d);
flag = 1;
break;
}
}
if (flag)
break;
}
if (flag)
break;
}
if (flag)
return 1;
return 0;
}
int main()
{
int x[4];
scanf("%d %d %d %d", &x[0], &x[1], &x[2], &x[3]);
int i, j, k, t;
int a, b, c, d;
int flag = 0;
for (i = 0; i < 4; i++)
{
for (j = 0; j < 4; j++)
{
if (j == i)
continue;
for (k = 0; k < 4; k++)
{
if (k == i || k == j)
continue;
for (t = 0; t < 4; t++)
{
if (t == i || t == j || t == k)
continue;
int a = x[i];
int b = x[j];
int c = x[k];
int d = x[t];
flag = solve(a, b, c, d);
if (flag)
break;
}
if (flag)
break;
}
if (flag)
break;
}
if (flag)
break;
}
if (!flag)
cout << -1 << endl;
return 0;
}