本文介绍 java 如何使用 jdbc 连接数据库以及连接数据库后的基本操作。
JDBC介绍
首先来了解 JDBC(Java Database Connectivity),java数据库连接。是 Java 提供一套用于操作数据库的接口 API,是 Java 中的数据库连接规范,由 java.sql.* , javax.sql.* 包中的一些类和接口组成,为开发人员操作数据库提供了一个标准的 API,提供统一访问,我们只需面向这套接口编程即可。
现如今有多种类型数据库,如果采用对各种类型数据库进行直接操作,这不利于程序管理,也增大程序员学习成本,如果哪个数据库升级,那么代码可能就对升级后的数据库不起作用。所以 Java 提供了标准的 API,各数据库厂商只需实现 Java 提供的 API 即可。
准备工作
- 首先下载 MySQL 的驱动包(下载网址:https://mvnrepository.com/)
查看 mysql 版本:
- 把 jar 包导入到项目中:
先新建一个文件夹 lib:
在把刚刚下载的 jar 包复制进来:
把驱动添加到库后,就可以看到 jar 包里的内容了:
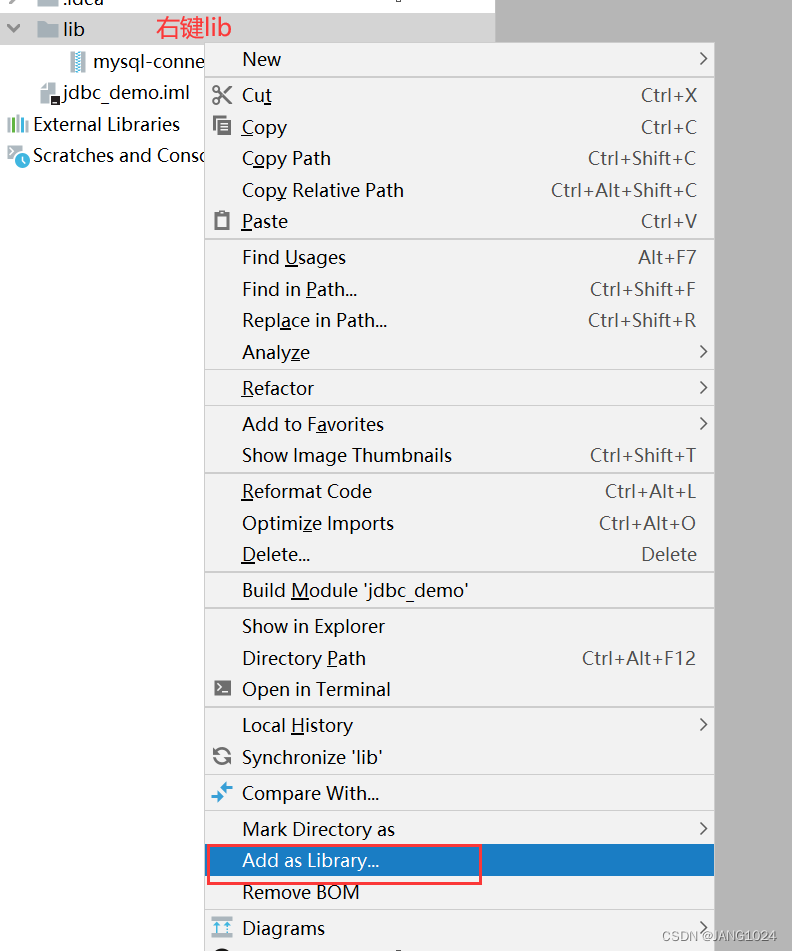
操作数据库
将 jar 包导入后,就可以编写代码来操作数据库了。
- 创建数据源:
//1. 创建并初始化一个数据源
DataSource dataSource = new MysqlDataSource();
((MysqlDataSource) dataSource).setUrl("jdbc:mysql://127.0.0.1:3306/java1?characterEncoding=utf8&useSSL=false");
((MysqlDataSource) dataSource).setUser("root");
((MysqlDataSource) dataSource).setPassword("666666");
- 建立连接:
建立连接时可能会抛出异常,需要处理。
//2. 和数据库服务器建立连接
Connection connection = dataSource.getConnection();
//java.sql下的Connection
- 构造 SQL 语句:
//编写SQL代码
String sql = "insert into students value('张三',18)";
//使用 PreparedStatement预编译 是为了减轻服务器的负担
PreparedStatement statement = connection.prepareStatement(sql);
此时表里没有数据:
- 执行 SQL 语句:
//执行SQL语句 delete,update,insert语句都是使用executeUpdate方法,
//返回值为int,表示影响到的行数
int res = statement.executeUpdate();
System.out.println("res=" + res);
- 释放资源:
//释放资源-----数据库和客户端进行通信的时候,是需要占用一定资源的,
//所以操作完成后,需要释放资源,并且创建的顺序和释放的顺序是相反的
//先创建的后释放,后创建的先释放
statement.close();
connection.close();
运行结果:
完整代码:(注意:数据库名、用户名、密码以及 SQL 语句,需要根据自己的情况来更改)
import com.mysql.cj.jdbc.MysqlDataSource;
import javax.sql.DataSource;
import java.sql.*;
public class test {
public static void main(String[] args) throws SQLException {
//1. 创建并初始化一个数据源
DataSource dataSource = new MysqlDataSource();
((MysqlDataSource) dataSource).setUrl("jdbc:mysql://127.0.0.1:3306/java1?characterEncoding=utf8&useSSL=false");
((MysqlDataSource) dataSource).setUser("root");
((MysqlDataSource) dataSource).setPassword("666666");
//2. 和数据库服务器建立连接
Connection connection = dataSource.getConnection();
//3.编写SQL代码
String sql = "insert into students value('张三',18)";
PreparedStatement statement = connection.prepareStatement(sql);
//4.执行SQL语句
int res = statement.executeUpdate();
System.out.println("res = " + res);
//5.释放资源
statement.close();
connection.close();
}
}
上面是通过将 SQL 语句写死来完成对表的插入操作的,但是我们更多的是通过用户输入字段 来完成插入功能的,所以可以使用 prepareStatement 的拼装功能来完成。
//输入要插入的信息
Scanner sc = new Scanner(System.in);
System.out.println("请输入姓名:");
String name = sc.nextLine();
System.out.println("请输入年龄:");
int age = sc.nextInt();
//3.编写SQL代码
String sql = "insert into students value(?,?)";
PreparedStatement statement = connection.prepareStatement(sql);
statement.setString(1, name);
statement.setInt(2, age);
System.out.println(statement);
运行效果:
代码:
import com.mysql.cj.jdbc.MysqlDataSource;
import javax.sql.DataSource;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Scanner;
public class test2 {
public static void main(String[] args) throws SQLException {
//1. 创建并初始化一个数据源
DataSource dataSource = new MysqlDataSource();
((MysqlDataSource) dataSource).setUrl("jdbc:mysql://127.0.0.1:3306/java1?characterEncoding=utf8&useSSL=false");
((MysqlDataSource) dataSource).setUser("root");
((MysqlDataSource) dataSource).setPassword("666666");
//2. 和数据库服务器建立连接
Connection connection = dataSource.getConnection();
//输入要插入的信息
Scanner sc = new Scanner(System.in);
System.out.println("请输入姓名:");
String name = sc.nextLine();
System.out.println("请输入年龄:");
int age = sc.nextInt();
//3.编写SQL代码
String sql = "insert into students value(?,?)";
PreparedStatement statement = connection.prepareStatement(sql);
statement.setString(1, name);
statement.setInt(2, age);
System.out.println(statement);
//4.执行SQL语句
int res = statement.executeUpdate();
System.out.println("res=" + res);
//5.释放资源
statement.close();
connection.close();
}
}
更新数据也类似:
查询数据:
代码:
import com.mysql.cj.jdbc.MysqlDataSource;
import javax.sql.DataSource;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class test3 {
public static void main(String[] args) throws SQLException {
//1. 创建并初始化一个数据源
DataSource dataSource = new MysqlDataSource();
((MysqlDataSource) dataSource).setUrl("jdbc:mysql://127.0.0.1:3306/java1?characterEncoding=utf8&useSSL=false");
((MysqlDataSource) dataSource).setUser("root");
((MysqlDataSource) dataSource).setPassword("666666");
//2. 和数据库服务器建立连接
Connection connection = dataSource.getConnection();
//3.编写SQL代码
String sql = "select * from students";
PreparedStatement statement = connection.prepareStatement(sql);
//4.执行SQL语句
//查询使用 executeQuery 方法,返回查询后的结果集
ResultSet resultSet = statement.executeQuery();
//遍历结果集合
while (resultSet.next()) {
int age = resultSet.getInt("age");
String name = resultSet.getString("name");
System.out.println("name: " + name + " age: " + age);
}
//5.释放资源
resultSet.close();
statement.close();
connection.close();
}
}
注意:如果有如下报错是因为 MySQL 服务没有开启。
打开 MySQL 服务后即可正常运行:Win + S(搜索)-> 输入服务 找到 MySQL ,点击启动此服务既可。
启动后即可正常运行。