(一)作者瞎叨叨:
这个代码真的我一直写到现在,足足近一千八百行,但我依旧坚持靠自己完成了这个代码的编写,实在是有点累了,话不多说直接上代码!
——Agoni酱
(二)设计要求:
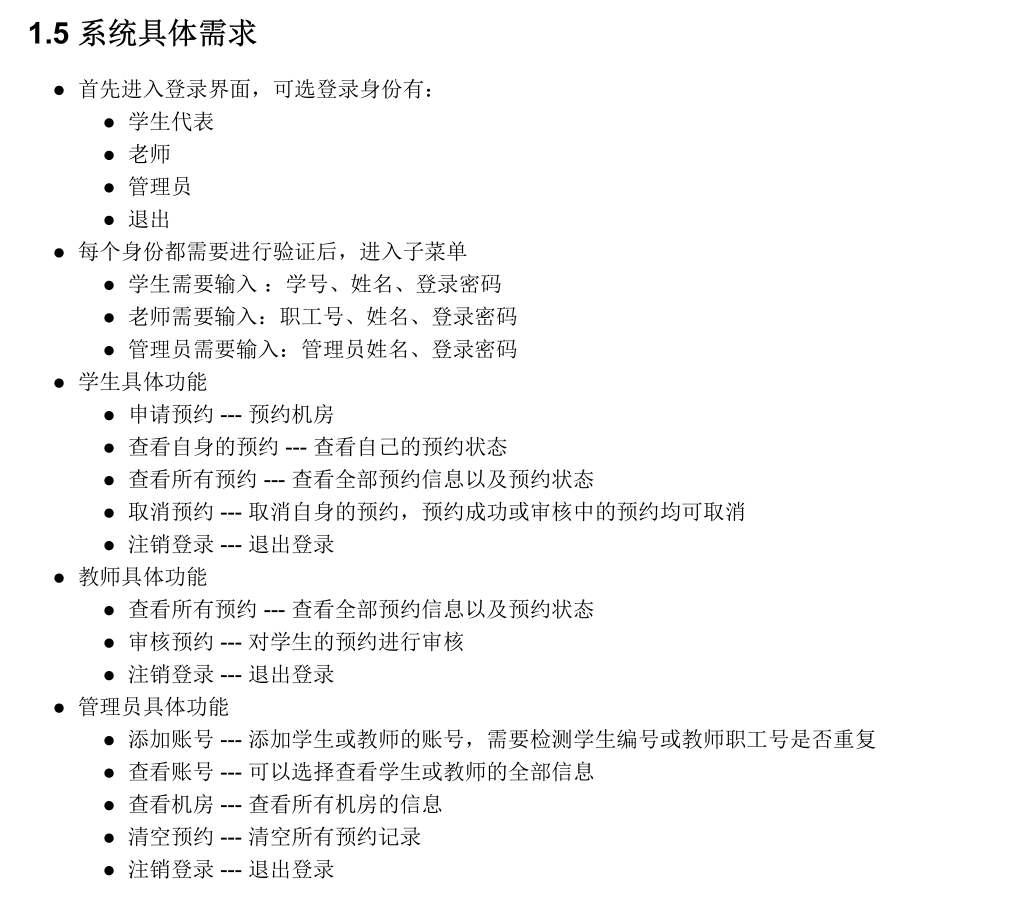
PS:看起来真的好复杂啊!!!
(三)程序相关事项(可见于main.cpp程序文件开头)
/*
开发项目:【黑马程序员~C++结课程序大设计】机房预约系统
程序结构:main.cpp (主程序) manager.h(Manager类的头文件) manager.cpp(Manager的成员函数存储)
开发语言:C++开发环境:Windows Visual Studio 2022
程序实现:可以实现对三个机房一周每天三个时间段的空位预约,可以记录学生,教师,管理员账号和密码,利用STL容器实现,可以存储至本地文件,可完成写入与读取,管理员支持添加账号,删除账号,查看特定账号的信息及其预约情况,更改账号信息,查看所有用户信息,查看特定时间段机房预约情况,可以清空预约,清除用户,更改管理员账号密码,教师支持查看学生的所有预约,审批学生的预约以及更改自己账户的密码,学生则支持申请预约,查看指定预约,查看所有预约,取消预约,更改自身密码
程序特性:本程序重心在于STL容器的应用,类的使用与文件写入读取,本程序逻辑复杂,写入数据不易实现,文件写入原理如下:管理员:只有一位,直接在admin.txt放入两行数据即可(第一行账号第二行密码)教师:由于教师不需要预约,所以教师的数据是两两一组,一行账号一行密码;但学生就较为复杂,我先读取一个标识符“account”为一个学生的开端,紧接着的是用户名和密码,在接下来是预约数据,两两一组,到下一个account为止;在机房文件中是一个由星期和时间段换算而来的序号和剩余空位的结合体,换算规则为number=(week - 1)*3 +time ;其实最重要的是学生预约数据的存储,我采用数字存储的方式完成,第一行为三到四位数,最后一位是审核状态(0 失败 1 未审核 2 成功)倒数第二位是机房号(1 sl501 2 sl502 3 sl503)剩下的便是前面星期和时间段换算而来的结果,第二行是人数(因为位数不固定,故单独储存)在设计完成后我又加入了文件实时检测,是由不断把内存容器内的数据输出完成的,这样可以有效避免在程序运行时的恶意纂改
*/
/*
完成时间:2023年1月14日00:27:16 当前版本:V1.0
发布时间:2023年1月14日00:39:48
更新日志:无
*/
(四)嫑废话,上代码!
(1)manager.h
#pragma once
#include <iostream>
#include <map>
#include <vector>
using namespace std;
class Manager {
public:
void readsl(map<int, int>&sl, string file_name);
void writesl(map<int, int>& sl, string file_name);
void reloadsl(string room_name);
void readadmin();
void writeadmin();
void readteacher();
void writeteacher();
void readstudent();
void writestudent();
void readfile(string file_name);
void showmenu();
void studentshowmenu();
void teachershowmenu();
void managershowmenu();
string now_name;
string now_password;
string admin_name;
string admin_password;
void text01();
void studentsystem();
void student1();
int searchrecord(int num);
string printfroom(int room);
string printfresult(int result);
void student2();
int searchrecord2(int num);
void student3();
void student4();
void student5();
void text02();
void teachersystem();
void teacher1();
void teacher2();
void teacher3();
void text03();
void managersystem();
void manager1();
void manager2();
void manager3();
void manager4();
void manager5();
void manager6();
void manager7();
void manager8();
void manager9();
map<string, vector<string>> student;
map<string, string> teacher;
map<int, int> sl501;
map<int, int> sl502;
map<int, int> sl503;
map<int, string>audit;
map<int, string>auditnext;
void RELOADING();
string get_time(int week, int time);
void Protect();
};
(2)manager.cpp
#include "manager.h"
#include <fstream>
#include <map>
#include <string>
void Manager::readsl(map<int,int>&sl,string file_name) {
ifstream ifs;
ifs.open(file_name, ios::in);
int i, j;
for (int m = 0; m < 21; m++) {
ifs >> i;
ifs >> j;
sl.insert(make_pair(i, j));
}
ifs.close();
}
void Manager::writesl(map<int, int>& sl, string file_name) {
ofstream ofs;
ofs.open(file_name, ios::out);
map<int, int>::iterator it = sl.begin();
for (int m = 0; m < 21; m++) {
ofs << it->first << endl ;
ofs << it->second << endl;
it++;
}
ofs.close();
}
void Manager::reloadsl(string room_name) {
ofstream ofs;
ofs.open(room_name, ios::out);
for (int i = 1; i <= 21; i++) {
ofs << i << endl;
ofs << 120 << endl;
}
ofs.close();
}
void Manager::readadmin() {
ifstream ifs;
ifs.open("admin.txt", ios::in);
string a;
char buf[1024] = { 0 };
ifs.getline(buf, sizeof(buf));
a = buf;
this->admin_name = a;
ifs.getline(buf, sizeof(buf));
this->admin_password = buf;
ifs.close();
}
void Manager::writeadmin() {
ofstream ofs;
ofs.open("admin.txt", ios::out);
ofs << this->admin_name << endl;
ofs << this->admin_password << endl;
ofs.close();
}
void Manager::readteacher(){
teacher.clear();
ifstream ifs;
ifs.open("teacher.txt", ios::in);
char buf[1024] = { 0 };
char account[1024] = { 0 };
while (ifs.getline(account, sizeof(account))) {
ifs.getline(buf, sizeof(buf));
teacher.insert(make_pair(account, buf));
}
ifs.close();
}
void Manager::writeteacher() {
ofstream ofs;
ofs.open("teacher.txt", ios::out);
map<string, string>::iterator it = this->teacher.begin();
while (it != this->teacher.end()) {
ofs << it->first << endl;
ofs << it->second << endl;
it++;
}
ofs.close();
}
void Manager::readstudent() {
student.clear();
ifstream ifs;
vector<string> s;
ifs.open("student.txt", ios::in);
char buf[1024] = { 0 };
char account[1024] = { 0 };
string a = buf;
if (!ifs.getline(account, sizeof(account))) {
ifs.close();
return;
}
ifs.getline(account, sizeof(account));
while (ifs.getline(buf, sizeof(buf))) {
a = buf;
if (a == "account") {
this->student.insert(make_pair(account, s));
s.clear();
ifs.getline(account, sizeof(account));
}
else {
s.push_back(buf);
}
}
this->student.insert(make_pair(account, s));
ifs.close();
}
void Manager::writestudent() {
ofstream ofs;
ofs.open("student.txt", ios::out );
map<string, vector<string>>::iterator it = this->student.begin();
while (it != this->student.end()) {
ofs << "account" << endl;
ofs << it->first << endl;
vector<string>::iterator itrecord = it->second.begin();
while (itrecord != it->second.end()) {
ofs << *itrecord << endl;
itrecord++;
}
it++;
}
ofs.close();
}
void Manager::readfile(string file_name) {
ifstream ifs;
ifs.open(file_name, ios::in );
if (!ifs.is_open()) {
cout << "system:" << file_name << "文件丢失,正在为您初始化......" << endl;
ofstream ofs;
ofs.open(file_name, ios::out );
if (file_name == "admin.txt"){
ofs << "admin" << endl << "123456";
ofs.close();
cout << "system:admin.txt文件初始化成功,您的管理员账号为:admin 密码为:123456" << endl;
}
else if(file_name == "sl501.txt" || file_name == "sl502.txt" || file_name == "sl503.txt") {
reloadsl(file_name);
cout << "system:" << file_name << "文件初始化成功,文件默认为每个机房容量120人" << endl;
}
else {
ofs.close();
cout << "system:"<< file_name <<"文件初始化成功,文件默认为空" << endl;
}
}
ifs.close();
}
void Manager::showmenu() {
cout << "**************************************" << endl;
cout << "** 欢迎使用机房预约系统 **" << endl;
cout << "** 请选择您的身份 **" << endl;
cout << "** **" << endl;
cout << "** 1 学生 **" << endl;
cout << "** 2 教师 **" << endl;
cout << "** 3 管理员 **" << endl;
cout << "** **" << endl;
cout << "** 0 退出系统 **" << endl;
cout << "** **" << endl;
cout << "** 制作:CSDN agoni酱 **" << endl;
cout << "** **" << endl;
cout << "** 文件实时保护系统已启用 **" << endl;
cout << "**************************************" << endl;
}
void Manager::studentshowmenu() {
cout << "欢迎学生" << now_name << "! 祝您学习进步!!!" << endl;
cout << "**************************************" << endl;
cout << "** 欢迎使用机房预约系统 **" << endl;
cout << "** 请选择您的操作 **" << endl;
cout << "** **" << endl;
cout << "** 1 申请预约 **" << endl;
cout << "** 2 查看预约 **" << endl;
cout << "** 3 查看所有预约 **" << endl;
cout << "** 4 撤销预约 **" << endl;
cout << "** 5 更改密码 **" << endl;
cout << "** 0 退出登录 **" << endl;
cout << "** **" << endl;
cout << "** 制作:CSDN agoni酱 **" << endl;
cout << "** **" << endl;
cout << "** 文件实时保护系统已启用 **" << endl;
cout << "**************************************" << endl;
}
void Manager::teachershowmenu() {
cout << "欢迎教师" << now_name << "! 祝您工作顺利!!!" << endl;
cout << "**************************************" << endl;
cout << "** 欢迎使用机房预约系统 **" << endl;
cout << "** 请选择您的操作 **" << endl;
cout << "** **" << endl;
cout << "** 1 查看所有预约 **" << endl;
cout << "** 2 审核预约 **" << endl;
cout << "** 3 更改密码 **" << endl;
cout << "** 0 退出登录 **" << endl;
cout << "** **" << endl;
cout << "** 制作:CSDN agoni酱 **" << endl;
cout << "** **" << endl;
cout << "** 文件实时保护系统已启用 **" << endl;
cout << "**************************************" << endl;
}
void Manager::managershowmenu() {
cout << "欢迎管理员" << this->admin_name << "! 祝您工作顺利!!!" << endl;
cout << "**************************************" << endl;
cout << "** 欢迎使用机房预约系统 **" << endl;
cout << "** 请选择您的操作 **" << endl;
cout << "** **" << endl;
cout << "** 1 添加账号 **" << endl;
cout << "** 2 删除账号 **" << endl;
cout << "** 3 查看账号及其预约 **" << endl;
cout << "** 4 更改密码 **" << endl;
cout << "** 5 查看所有用户 **" << endl;
cout << "** 6 查看机房余量 **" << endl;
cout << "** 7 清空预约 **" << endl;
cout << "** 8 清空用户 **" << endl;
cout << "** 9 更改管理员账户 **" << endl;
cout << "** 0 退出登录 **" << endl;
cout << "** **" << endl;
cout << "** 制作:CSDN agoni酱 **" << endl;
cout << "** **" << endl;
cout << "** 文件实时保护系统已启用 **" << endl;
cout << "**************************************" << endl;
}
void Manager::text01() {
cout << "system:请登录学生账号:";
string account;
Protect();
cin >> account;
if (student.find(account)!=student.end()) {
Protect();
cout << "system:请输入学生" << account << "的密码:";
string password;
cin >> password;
if (password == student.find(account)->second.front()) {
cout << "system:登录成功!欢迎学生" << account << "!!!" << endl;
this->now_name = account;
this->now_password = password;
system("pause");
system("cls");
studentsystem();
}
else {
cout << "system:登录失败!密码错误!!!" << endl;
}
}
else {
cout << "system:登录失败!不存在账号为 " << account << " 的学生账户!!!" << endl;
}
}
void Manager::studentsystem() {
int in = 0;
while (true) {
studentshowmenu();
Protect();
cout << "system:请输入您的操作代码:";
cin >> in;
switch (in) {
case 1:
Protect();
student1();
break;
case 2:
Protect();
student2();
break;
case 3:
Protect();
student3();
break;
case 4:
Protect();
student4();
break;
case 5:
Protect();
student5();
break;
case 0:
cout << "system:感谢使用,下次再会!" << endl;
cout << "system:学生 " << this->now_name << " 登出成功!!!" << endl;
system("pause");
system("cls");
return;
default:
Protect();
cout << "system:输入有误,请检查后重新输入!" << endl;
system("pause");
system("cls");
break;
}
}
}
void Manager::student1() {
int week = 0, time = 0, num = 0 , sum = 0, number = 0, num1 = 0;
string s;
cout << "system:请输入星期( 1 ~ 7 ):";
Protect();
cin >> week;
while (week > 7 || week < 1) {
cout << "system:星期输入错误,请检查后重新输入!" << endl;
cout << "system:请输入星期( 1 ~ 7 ):";
Protect();
cin >> week;
}
cout << "system:请输入时间段(1 上午 2 下午 3 晚上):";
Protect();
cin >> time;
while (time > 3 || time < 1) {
cout << "system:时间段输入错误,请检查后重新输入!" << endl;
cout << "system:请输入时间段(1 上午 2 下午 3 晚上):";
Protect();
cin >> time;
}
if (sum=searchrecord(time + (week - 1) * 3)) {
Protect();
cout << "system: " << get_time(week, time) << "的申请记录如下:" << endl;
cout << "预约时间: " << get_time(week, time) << " 人数: " << sum / 100 << "\t机房: " << printfroom(sum / 10 % 10) << " 审核情况: " << printfresult(sum % 10) << endl;
system("pause");
system("cls");
return;
}
cout << "system:请输入机房编号(1 sl501 2 sl502 3 sl503):";
Protect();
cin >> num;
while (num > 3 || num < 1) {
cout << "system:机房编号输入错误,请检查后重新输入!" << endl;
cout << "system:请输入机房编号(1 sl501 2 sl502 3 sl503):";
Protect();
cin >> num;
}
Protect();
switch (num) {
case 1:
number = sl501.find(num)->second;
break;
case 2:
number = sl502.find(num)->second;
break;
case 3:
number = sl503.find(num)->second;
break;
}
cout << "system:请输入您想要预定的座位数:";
Protect();
cin >> num1;
while (number < num1) {
cout << "system: " << get_time(week, time) << " "<< printfroom(num)<< "机房空位余量不足!当前剩余: " << number<< endl;
cout << "system:请输入您想要预定的座位数:";
Protect();
cin >> num1;
}
Protect();
cout << "system:预约成功!请等待教师审核!!!" << endl;
student.find(now_name)->second.push_back(to_string((time + (week - 1) * 3 ) * 100 + num * 10 + 1));
student.find(now_name)->second.push_back(to_string(num1));
writestudent();
cout << "system:写入student.txt成功!!!" << endl;
system("pause");
system("cls");
}
int Manager::searchrecord(int num) {
int size = 0, sum = 0;
vector<string>::iterator it = student.find(now_name)->second.begin();
if ((size=student.find(now_name)->second.size()) == 1) {
return 0;
}
else {
sum = (size - 1) / 2;
for (int i = 0; i < sum; i++) {
it++;
if (stoi(*it)/100 == num && stoi(*it) %10 != 0) {
return (stoi(*it) % 100) + stoi(*(++it)) * 100;
}
else {
it++;
}
}
return 0;
}
}
string Manager::printfroom(int room) {
string s = "sl50";
s.push_back(room+'0');
return s;
}
string Manager::printfresult(int result) {
switch (result) {
case 1:
return "未审核!";
case 2:
return "审核通过!";
case 0:
return "审核不通过!";
}
}
void Manager::student2() {
if (student.find(now_name)->second.size() == 1) {
cout << "system:您的申请记录为空!!!";
system("pause");
system("cls");
return;
}
int week = 0, time = 0, num = 0, sum = 0, number = 0, num1 = 0;
string s;
Protect();
cout << "system:请输入星期( 1 ~ 7 ):";
cin >> week;
while (week > 7 || week < 1) {
cout << "system:星期输入错误,请检查后重新输入!" << endl;
cout << "system:请输入星期( 1 ~ 7 ):";
Protect();
cin >> week;
}
cout << "system:请输入时间段(1 上午 2 下午 3 晚上):";
Protect();
cin >> time;
while (time > 3 || time < 1) {
cout << "system:时间段输入错误,请检查后重新输入!" << endl;
cout << "system:请输入时间段(1 上午 2 下午 3 晚上):";
Protect();
cin >> time;
}
Protect();
searchrecord2((week - 1) * 3 + time);
system("pause");
system("cls");
}
int Manager::searchrecord2(int num) {
int size = 0, sum = 0, number = 0, flag = 1;
vector<string>::iterator it = student.find(now_name)->second.begin();
size = student.find(now_name)->second.size();
sum = (size - 1) / 2;
for (int i = 0; i < sum; i++) {
it++;
if (stoi(*it) / 100 == num) {
number = stoi(*it);
if (flag) {
Protect();
cout << "system: " << get_time(num / 3 + 1, (num - 1) % 3 + 1) << "的申请记录如下:" << endl;
}
flag = 0;
Protect();
cout << "预约时间: " << get_time(num / 3 + 1, (num - 1) % 3 + 1) << " 人数: " << *(++it) << "\t机房: " << printfroom(number/10%10) << " 审核情况: " << printfresult(number % 10) << endl;
}
else {
it++;
}
}
if (flag) {
Protect();
cout << "system:不存在 " << get_time(num / 3 + 1, (num - 1) % 3 + 1) << "的申请记录!!!" << endl;
return 1;
}
return 0;
}
void Manager::student3() {
Protect();
if (student.find(now_name)->second.size() == 1) {
cout << "system:您的申请记录为空!!!";
system("pause");
system("cls");
return;
}
int first = 0, second = 0;
cout << "system:您的申请记录如下:" << endl;
Protect();
vector<string>::iterator it = ++student.find(now_name)->second.begin();
while (it != student.find(now_name)->second.end()) {
first = stoi(*it);
second = stoi(*(++it));
cout << "system:预约时间: " << get_time(first/100/3+1,(first/100-1)%3+1) << " 人数: " << second << "\t机房: " << printfroom(first / 10 % 10) << " 审核情况: " << printfresult(first % 10) << endl;
it++;
}
system("pause");
system("cls");
return;
}
void Manager::student4() {
if (student.find(now_name)->second.size() == 1) {
cout << "system:您的申请记录为空!!!";
system("pause");
system("cls");
return;
}
Protect();
int week = 0, time = 0, num = 0, sum = 0, number = 0 , people = 0;
string s;
cout << "system:请输入星期( 1 ~ 7 ):";
cin >> week;
while (week > 7 || week < 1) {
cout << "system:星期输入错误,请检查后重新输入!" << endl;
cout << "system:请输入星期( 1 ~ 7 ):";
Protect();
cin >> week;
}
cout << "system:请输入时间段(1 上午 2 下午 3 晚上):";
Protect();
cin >> time;
while (time > 3 || time < 1) {
cout << "system:时间段输入错误,请检查后重新输入!" << endl;
cout << "system:请输入时间段(1 上午 2 下午 3 晚上):";
Protect();
cin >> time;
}
if (num = searchrecord((week - 1) * 3 + time)) {
cout << "system: " << get_time(week, time) << "的申请记录如下:" << endl;
cout << "预约时间: " << get_time(week, time) << " 人数: " << num / 100 << "\t机房: " << printfroom(num / 10 % 10) << " 审核情况: " << printfresult(num % 10) << endl;
cout << "system:确认撤销?(1 确认 其他 取消):";
Protect();
cin >> number;
if (number != 1) {
Protect();
cout << "system:取消撤销成功!!!" << endl;
}
sum = num % 100 + ((week - 1) * 3 + time) * 100;
student.find(now_name)->second.erase(student.find(now_name)->second.erase(find(student.find(now_name)->second.begin(), student.find(now_name)->second.end(), to_string(sum))));
Protect();
cout << "system:撤销成功!!!" << endl;
writestudent();
cout << "system:写入student.txt成功!!!" << endl;
if (num % 10 == 2) {
switch (num / 10 % 10) {
case 1:
people = (sl501.find((week - 1) * 3 + time)->second);
sl501.erase(sl501.find((week - 1) * 3 + time));
sl501.insert(make_pair((week - 1) * 3 + time, people + num / 100));
writesl(sl501, "sl501.txt");
cout << "system:写入sl501.txt成功!!!" << endl;
break;
case 2:
people = (sl502.find((week - 1) * 3 + time)->second);
sl502.erase(sl502.find((week - 1) * 3 + time));
sl502.insert(make_pair((week - 1) * 3 + time, people + num / 100));
writesl(sl502, "sl502.txt");
cout << "system:写入sl502.txt成功!!!" << endl;
break;
case 3:
people = (sl503.find((week - 1) * 3 + time)->second);
sl503.erase(sl503.find((week - 1) * 3 + time));
sl503.insert(make_pair((week - 1) * 3 + time, people + num / 100));
writesl(sl503, "sl503.txt");
cout << "system:写入sl503.txt成功!!!" << endl;
break;
}
}
}
else {
Protect();
cout << "system:不存在 " << get_time(week, time) << "可以撤销的记录!!!" << endl;
}
system("pause");
system("cls");
}
void Manager::student5() {
cout << "system:请输入学生" << now_name << "的密码:";
string password;
Protect();
cin >> password;
if (password == student.find(now_name)->second.front()) {
cout << "system:学生账号 " << now_name << " 密码正确,请输入您更改后的密码:" ;
Protect();
cin >> password;
now_password = password;
Protect();
student.find(now_name)->second.insert(student.find(now_name)->second.erase(student.find(now_name)->second.begin()),password);
cout << "system:更改成功!!!"<< endl;
writestudent();
cout << "system:写入student.txt成功!!!" << endl;
}
else {
Protect();
cout << "system:更改失败!密码错误!!!" << endl;
}
system("pause");
system("cls");
}
void Manager::text02() {
cout << "system:请登录教师账号:";
string account;
Protect();
cin >> account;
if (teacher.find(account)!=teacher.end()) {
cout << "system:请输入教师" << account << "的密码:";
string password;
Protect();
cin >> password;
if (password == teacher.find(account)->second) {
cout << "system:登录成功!欢迎教师" << account << "!!!" << endl;
Protect();
now_name = account;
now_password = password;
system("pause");
system("cls");
teachersystem();
}
else {
cout << "system:登录失败!密码错误!!!" << endl;
}
}
else {
cout << "system:登录失败!教师账号不存在: " << account << endl;
}
}
void Manager::teachersystem() {
int in = 0;
while (true) {
teachershowmenu();
cout << "system:请输入您的操作代码:";
cin >> in;
switch (in) {
case 1:
Protect();
teacher1();
break;
case 2:
Protect();
teacher2();
break;
case 3:
Protect();
teacher3();
break;
case 0:
cout << "system:感谢使用,下次再会!" << endl;
cout << "system:教师 " << this->now_name << " 登出成功!!!" << endl;
Protect();
system("pause");
system("cls");
return;
default:
Protect();
cout << "system:输入有误,请检查后重新输入!" << endl;
system("pause");
system("cls");
break;
}
}
}
void Manager::teacher1() {
map<string, vector<string>>::iterator it = student.begin();
vector<string>::iterator itrecord;
int num = 0,first = 0,second = 0;
Protect();
while (it != student.end()) {
itrecord = ++it->second.begin();
if ((num = it->second.size()) == 1) {
Protect();
cout << "system:学生 " << it->first << " 的预约记录为空!!!" << endl;
}
else {
Protect();
cout << "system:学生 " << it->first << " 的预约记录如下:" << endl;
while (itrecord != it->second.end()) {
first = stoi(*itrecord++);
second = stoi(*itrecord++);
cout << "预约时间: " << get_time(first / 100 / 3 + 1, (first / 100 - 1) % 3 + 1) << " 人数: " << second << "\t机房: " << printfroom(first / 10 % 10) << " 审核情况: " << printfresult(first % 10) << endl;
}
}
Protect();
cout << endl;
it++;
}
system("pause");
system("cls");
return;
}
void Manager::teacher2() {
while (true) {
audit.clear();
Protect();
map<string, vector<string>>::iterator it = student.begin();
vector<string>::iterator itrecord;
int flag = 1, first = 0, second = 0, num = 1, in = 0, in1 = 2, number = 0, flagmax = 1;
while (it != student.end()) {
itrecord = ++it->second.begin();
flag = 1;
while (itrecord != it->second.end()) {
first = stoi(*itrecord++);
second = stoi(*itrecord++);
if (first % 10 == 1) {
if (flagmax) {
cout << "system:待审核预约如下:" << endl;
flagmax = 0;
}
if (flag) {
cout << endl;
cout << "system: " << num << " 学生 " << it->first << " 的待审核预约记录如下:" << endl;
num++;
flag = 0;
Protect();
}
Protect();
cout << "system:预约时间: " << get_time(first / 100 / 3 + 1, (first / 100 - 1) % 3 + 1) << " 人数: " << second << "\t机房: " << printfroom(first / 10 % 10) << " 审核情况: " << printfresult(first % 10) << endl;
audit.insert(make_pair(num - 1, it->first));
}
}
it++;
}
if (flagmax) {
Protect();
cout << "system:暂无需要审核的审批!!!" << endl;
system("pause");
system("cls");
return;
}
cout << "system:请输入您想审核的学生编号(输入0可以退出审核系统):";
Protect();
cin >> in;
if (in == 0) {
cout << "system:成功退出审核系统!!!" << endl;
system("pause");
system("cls");
return;
}
while (audit.find(in) == audit.end()) {
cout << "system:输入学生编号有误,请重新输入!!!" << endl;
cout << "system:请输入您想审核的学生编号:";
Protect();
cin >> in;
Protect();
if (in == 0) {
cout << "system:成功退出审核系统!!!" << endl;
Protect();
system("pause");
system("cls");
return;
}
}
string name = audit.find(in)->second;
Protect();
cout << "system:请审核 " << name << "的预约申请:" << endl;
itrecord = ++student.find(name)->second.begin();
num = 1;
flag = 1;
while (itrecord != student.find(name)->second.end()) {
first = stoi(*itrecord++);
second = stoi(*itrecord++);
if (first % 10 == 1) {
if (flag) {
cout << "system: 学生 " << audit.find(in)->second << " 的待审核预约记录如下:" << endl;
flag = 0;
}
cout << "system: " << num << " 预约时间: " << get_time(first / 100 / 3 + 1, (first / 100 - 1) % 3 + 1) << " 人数: " << second << "\t机房: " << printfroom(first / 10 % 10) << " 审核情况: " << printfresult(first % 10) << endl;
auditnext.insert(make_pair(num, to_string(first)));
num++;
}
}
Protect();
cout << "system:请输入您想审核的预约编号(输入0可以退出审核系统):";
cin >> in;
if (in == 0) {
cout << "system:成功退出审核系统!!!" << endl;
system("pause");
system("cls");
return;
}
while (auditnext.find(in) == auditnext.end()) {
cout << "system:输入预约编号有误,请重新输入!!!" << endl;
cout << "system:请输入您想审核的预约编号(输入0可以退出审核系统):";
Protect();
cin >> in;
if (in == 0) {
cout << "system:成功退出审核系统!!!" << endl;
Protect();
system("pause");
system("cls");
return;
}
}
vector<string>::iterator its = ++student.find(name)->second.begin();
while (*its != auditnext.find(in)->second) {
its++;
}
Protect();
vector<string>::iterator itnext = its;
num = stoi(auditnext.find(in)->second);
int people = stoi(*(++itnext));
switch (num / 10 % 10) {
case 1:
if (sl501.find(num / 100)->second < people) {
Protect();
cout << "system: sl501 机房容量不足,已自动不通过审核!!!" << endl;
goto out;
}
break;
case 2:
if (sl502.find(num / 100)->second < people) {
Protect();
cout << "system: sl502 机房容量不足,已自动不通过审核!!!" << endl;
goto out;
}
break;
case 3:
if (sl503.find(num / 100)->second < people) {
Protect();
cout << "system: sl503 机房容量不足,已自动不通过审核!!!" << endl;
goto out;
}
break;
}
Protect();
cout << "system:是否通过该申请(1 通过 2 不通过 0 退出审核):";
cin >> in1;
if (in1 == 0) {
cout << "system:成功退出审核系统!!!" << endl;
Protect();
system("pause");
system("cls");
return;
}
Protect();
while (in1 != 1 && in1 != 2 && in1 != 0) {
cout << "system:输入编号有误,请重新输入!!!" << endl;
cout << "system:是否通过该申请(1 通过 2 不通过 0 退出审核):";
Protect();
cin >> in1;
Protect();
if (in1 == 0) {
cout << "system:成功退出审核系统!!!" << endl;
system("pause");
system("cls");
return;
}
}
out:
if (in1 == 1) {
Protect();
number = stoi(auditnext.find(in)->second) + 1;
student.find(name)->second.insert(student.find(name)->second.erase(its), to_string(number));
switch (num / 10 % 10) {
case 1:
Protect();
number = sl501.find(num / 100)->second - people;
sl501.erase(num / 100);
sl501.insert(make_pair(num / 100, number));
writesl(sl501, "sl501.txt");
break;
case 2:
Protect();
number = sl502.find(num / 100)->second - people;
sl502.erase(num / 100);
sl502.insert(make_pair(num / 100, number));
writesl(sl502, "sl502.txt");
break;
case 3:
Protect();
number = sl503.find(num / 100)->second - people;
sl503.erase(num / 100);
sl503.insert(make_pair(num / 100, number));
writesl(sl503, "sl503.txt");
break;
}
}
else {
Protect();
number = stoi(auditnext.find(in)->second) - 1;
student.find(name)->second.insert(student.find(name)->second.erase(its), to_string(number));
}
cout << "system:审核完成!!!" << endl;
writestudent();
cout << "system:写入文件student.txt成功!!!" << endl;
system("pause");
system("cls");
}
}
void Manager::teacher3() {
cout << "system:请输入教师" << now_name << "的密码:";
string password;
Protect();
cin >> password;
Protect();
if (password == teacher.find(now_name)->second) {
cout << "system:教师账号 " << now_name << " 密码正确,请输入您更改后的密码:";
Protect();
cin >> password;
now_password = password;
teacher.erase(now_name);
teacher.insert(make_pair(now_name, password));
cout << "system:更改成功!!!" << endl;
writeteacher();
cout << "system:写入teacher.txt成功!!!" << endl;
}
else {
cout << "system:更改失败!密码错误!!!" << endl;
}
system("pause");
system("cls");
}
void Manager::text03() {
cout << "system:请登录管理员账号:";
string account;
Protect();
cin >> account;
Protect();
if (account == this->admin_name) {
cout << "system:请输入管理员" << account << "的密码:";
Protect();
string password;
cin >> password;
if (password == this->admin_password) {
cout << "system:登录成功!欢迎管理员" << account << "!!!" << endl;
Protect();
system("pause");
system("cls");
this->managersystem();
}
else {
cout << "system:登录失败!密码错误!!!" << endl;
}
}
else {
cout << "system:登录失败!管理员账号不为:" << account << endl;
system("pause");
system("cls");
}
}
void Manager::managersystem() {
int in = 0;
while (true) {
this->managershowmenu();
Protect();
cout << "system:请输入您的操作代码:";
cin >> in;
Protect();
switch (in) {
case 1:
manager1();
break;
case 2:
manager2();
break;
case 3:
manager3();
break;
case 4:
manager4();
break;
case 5:
manager5();
break;
case 6:
manager6();
break;
case 7:
manager7();
break;
case 8:
manager8();
break;
case 9:
manager9();
break;
case 0:
Protect();
cout << "system:感谢使用,下次再会!" << endl;
cout << "system:管理员" << this->admin_name <<"登出成功!!!" << endl;
system("pause");
system("cls");
return;
default:
Protect();
cout << "system:输入有误,请检查后重新输入!" << endl;
system("pause");
system("cls");
break;
}
}
}
void Manager::manager1() {
int in;
vector<string> s;
string account, password;
while (true) {
Protect();
cout << "system:您要添加什么类型的账户(1 学生 2 教师):";
cin >> in;
switch (in) {
case 1:
cout << "system:请输入您想要添加的学生账号:";
Protect();
cin >> account;
while (this->student.find(account) != this->student.end()) {
cout << "system:已存在此学生!请重新输入:";
Protect();
cin >> account;
}
cout << "system:请输入学生 " << account << " 的密码:";
Protect();
cin >> password;
s.push_back(password);
this->student.insert(make_pair(account, s));
this->writestudent();
Protect();
cout << "system:学生 " << account << " 信息录入成功!!!" << endl;
system("pause");
system("cls");
return;
case 2:
cout << "system:请输入您想要添加的教师账号:";
Protect();
cin >> account;
Protect();
while (this->teacher.find(account) != this->teacher.end()) {
cout << "system:已存在此教师!请重新输入:";
cin >> account;
}
cout << "system:请输入教师 " << account << " 的密码:";
Protect();
cin >> password;
teacher.insert(make_pair(account, password));
writeteacher();
cout << "system:教师 " << account << " 信息录入成功!!!" << endl;
system("pause");
system("cls");
return;
default:
Protect();
cout << "system:输入不合法,请重新输入!!!" << endl;
break;
}
}
}
void Manager::manager2() {
int in;
string account;
Protect();
while (true) {
cout << "system:您要删除什么类型的账户(1 学生 2 教师):";
Protect();
cin >> in;
Protect();
switch (in) {
case 1:
if (this->student.empty()) {
cout << "system:文件student.txt为空,无法完成删除!!!" << endl;
system("pause");
system("cls");
return;
}
cout << "system:请输入对应的学生账号:";
cin >> account;
if (this->student.find(account) != this->student.end()) {
Protect();
cout << "system:成功查询到学生" << account << "信息,确认删除?(1 确认 其他 取消):";
cin >> in;
if (in == 1) {
this->student.erase(account);
cout << "system:学生" << account << "删除成功!!!" << endl;
writestudent();
cout << "system:写入文件student.txt成功!!!" <<endl;
}
else {
cout << "system:取消删除成功!!!" << endl;
}
}
else {
cout << "system:无法查询到学生" << account << "信息!!!" << endl;
}
system("pause");
system("cls");
return;
case 2:
if (this->teacher.empty()) {
cout << "system:文件teacher.txt为空,无法完成删除!!!" << endl;
system("pause");
system("cls");
return;
}
cout << "system:请输入对应的教师账号:";
cin >> account;
if (this->teacher.find(account) != this->teacher.end()) {
Protect();
cout << "system:成功查询到教师" << account << "信息,确认删除?(1 确认 其他 取消):";
cin >> in;
if (in == 1) {
this->teacher.erase(account);
cout << "system:教师" << account << "删除成功!!!" << endl;
writeteacher();
cout << "system:写入文件teacher.txt成功!!!" << endl;
}
else {
cout << "system:取消删除成功!!!" << endl;
}
}
else {
cout << "system:无法查询到教师" << account << "信息!!!" << endl;
}
system("pause");
system("cls");
return;
default:
cout << "system:输入有误,请检查后重新输入!" << endl;
system("pause");
system("cls");
break;
}
}
}
void Manager::manager3() {
int in = 0, first = 0, second = 0;
string account;
while (true) {
Protect();
cout << "system:您要查看什么类型的账户(1 学生 2 教师):";
cin >> in;
switch (in) {
case 1:
if (this->student.empty()) {
Protect();
cout << "system:文件student.txt为空,无法完成读取!!!" << endl;
system("pause");
system("cls");
return;
}
cout << "system:请输入对应的学生账号:";
cin >> account;
if (this->student.find(account) != this->student.end()) {
Protect();
cout << "system:成功查询到学生 " << account << " 信息如下:" << endl;
vector<string>::iterator it = this->student.find(account)->second.begin();
cout << "system:学生账号: " << account << "\t密码:" << *it << endl;
if (this->student.find(account)->second.size() == 1) {
Protect();
cout << "system:学生 " << account << " 暂无预约记录!!!" << endl;
}
else {
it++;
Protect();
cout << "system:学生 " << account << " 预约记录如下:" << endl;
while (it != this->student.find(account)->second.end()) {
first = stoi(*(it++));
second = stoi(*(it++));
cout << "system:预约时间: " << get_time(first / 100 / 3 + 1, (first / 100 - 1) % 3 + 1) << " 人数: " << second << "\t机房: " << printfroom(first / 10 % 10) << " 审核情况: " << printfresult(first % 10) << endl;
}
}
}
else {
cout << "system:无法查询到学生" << account << "信息!!!" << endl;
}
system("pause");
system("cls");
return;
case 2:
if (this->teacher.empty()) {
Protect();
cout << "system:文件teacher.txt为空,无法完成读取!!!" << endl;
system("pause");
system("cls");
return;
}
cout << "system:请输入对应的教师账号:";
cin >> account;
if (this->teacher.find(account) != this->teacher.end()) {
cout << "system:成功查询到教师 " << account << " 信息如下:" << endl;
cout << "system:教师账号:" << account << " 密码:" << this->teacher.find(account)->second << endl;
}
else {
cout << "system:无法查询到教师" << account << "信息!!!" << endl;
}
Protect();
system("pause");
system("cls");
return;
default:
Protect();
cout << "system:输入有误,请检查后重新输入!" << endl;
system("pause");
system("cls");
break;
}
}
}
void Manager::manager4() {
int in;
string account,password;
while (true) {
cout << "system:您要修改什么类型的账户(1 学生 2 教师):";
Protect();
cin >> in;
switch (in) {
case 1:
if (this->student.empty()) {
cout << "system:文件student.txt为空,无法完成读取!!!" << endl;
system("pause");
system("cls");
return;
}
cout << "system:请输入对应的学生账号:";
cin >> account;
if (this->student.find(account) != this->student.end()) {
Protect();
cout << "system:成功查询到学生" << account << "信息如下:" << endl;
cout << "system:学生账号:" << account << " 密码:" << *(this->student.find(account)->second.begin()) << endl;
cout << "system:将学生 " << account << " 的密码修改为:";
cin >> password;
this->student.find(account)->second.erase(this->student.find(account)->second.begin());
this->student.find(account)->second.insert(this->student.find(account)->second.begin(), password);
cout << "system:学生 " << account << " 的密码修改成功!!!" << endl;
writestudent();
cout << "system:写入文件student.txt成功!!!" << endl;
}
else {
Protect();
cout << "system:无法查询到学生 " << account << " 信息!!!" << endl;
}
system("pause");
system("cls");
return;
case 2:
if (this->teacher.empty()) {
Protect();
cout << "system:文件teacher.txt为空,无法完成读取!!!" << endl;
system("pause");
system("cls");
return;
}
cout << "system:请输入对应的教师账号:";
cin >> account;
if (this->teacher.find(account) != this->teacher.end()) {
Protect();
cout << "system:成功查询到教师 " << account << " 信息如下:" << endl;
cout << "system:教师账号:" << account << " 密码:" << this->teacher.find(account)->second << endl;
cout << "system:将教师 " << account << " 的密码修改为:";
cin >> password;
teacher.erase(this->teacher.find(account));
teacher.insert(make_pair(account, password));
cout << "system:教师 " << account << " 的密码修改成功!!!" << endl;
writeteacher();
Protect();
cout << "system:写入文件teacher.txt成功!!!" << endl;
}
else {
Protect();
cout << "system:无法查询到教师" << account << "信息!!!" << endl;
}
system("pause");
system("cls");
return;
default:
Protect();
cout << "system:输入有误,请检查后重新输入!" << endl;
system("pause");
system("cls");
break;
}
}
}
void Manager::manager5() {
if (this->student.size() == 0) {
cout << "system:学生名单为空!" << endl;
}
else {
cout << "system:学生名单如下:" << endl;
Protect();
map<string, vector<string>>::iterator it = this->student.begin();
while (it != this->student.end()) {
cout << "账号:" << it->first << "\t\t\t密码:" << it->second.front() << endl;
it++;
}
}
if (this->teacher.size() == 0) {
Protect();
cout << endl << "system:教师名单为空!" << endl;
}
else{
cout << endl << "system:教师名单如下:" << endl;
map<string, string>::iterator it2 = this->teacher.begin();
Protect();
while (it2 != this->teacher.end()) {
cout << "账号:" << it2->first << "\t\t\t密码:" << it2->second << endl;
it2++;
}
}
cout << endl << "system:名单输出完成!!!" << endl;
Protect();
system("pause");
system("cls");
}
void Manager::manager6() {
int week = 0, time = 0, num = 0;
string s;
cout << "system:请输入星期( 1 ~ 7 ):";
Protect();
cin >> week;
while (week > 7 || week < 1) {
cout << "system:星期输入错误,请检查后重新输入!" << endl;
cout << "system:请输入星期( 1 ~ 7 ):";
cin >> week;
}
cout << "system:请输入时间段(1 上午 2 下午 3 晚上):";
cin >> time;
while (time > 3 || time < 1 ) {
cout << "system:时间段输入错误,请检查后重新输入!" << endl;
cout << "system:请输入时间段(1 上午 2 下午 3 晚上):";
cin >> time;
Protect();
}
while (true) {
cout << "system:请输入机房编号(1 sl501 2 sl502 3 sl503):";
Protect();
cin >> num;
switch (num) {
case 1:
s = get_time(week, time);
num = (week - 1) * 3 + time;
cout << "system: "<< s <<" sl501 还剩下:" << sl501.find(num)->second << " 个空位!" << endl;
system("pause");
system("cls");
return;
case 2:
s = get_time(week, time);
num = (week - 1) * 3 + time;
cout << "system: " << s << " sl502 还剩下:" << sl502.find(num)->second << " 个空位!" << endl;
system("pause");
system("cls");
return;
case 3:
s = get_time(week, time);
num = (week - 1) * 3 + time;
cout << "system: " << s << " sl503 还剩下:" << sl503.find(num)->second << " 个空位!" << endl;
system("pause");
system("cls");
return;
default:
cout << "system:机房编号输入有误,请检查后重新输入!" << endl;
break;
}
}
}
void Manager::manager7() {
cout << "system:确认要清除所有预约吗?(1 确认 其他 取消):";
Protect();
int num = 0;
cin >> num;
if (num != 1) {
Protect();
cout << "system:取消清除成功!!!" << endl;
system("pause");
system("cls");
return;
}
Protect();
map<string, vector<string>> ::iterator it = student.begin();
while (it != student.end()) {
it->second.erase(++it->second.begin(), it->second.end());
it++;
}
cout << "system:清除成功!!!" << endl;
writestudent();
cout << "system:写入student.txt成功!!!" << endl;
reloadsl("sl501.txt");
reloadsl("sl502.txt");
reloadsl("sl503.txt");
sl501.clear();
sl502.clear();
sl503.clear();
readsl(sl501, "sl501.txt");
readsl(sl502, "sl502.txt");
readsl(sl503, "sl503.txt");
cout << "system:重载sl501.txt成功!!!" << endl;
cout << "system:重载sl502.txt成功!!!" << endl;
cout << "system:重载sl503.txt成功!!!" << endl;
system("pause");
system("cls");
}
void Manager::manager8() {
cout << "system:确认要清除所有用户吗?(1 确认 其他 取消):";
Protect();
int num = 0;
cin >> num;
if (num != 1) {
cout << "system:取消清除成功!!!" << endl;
system("pause");
system("cls");
return;
}
Protect();
student.clear();
teacher.clear();
cout << "system:清除成功!!!" << endl;
writestudent();
cout << "system:写入student.txt成功!!!" << endl;
writeteacher();
cout << "system:写入teacher.txt成功!!!" << endl;
reloadsl("sl501.txt");
reloadsl("sl502.txt");
reloadsl("sl503.txt");
sl501.clear();
sl502.clear();
sl503.clear();
readsl(sl501, "sl501.txt");
readsl(sl502, "sl502.txt");
readsl(sl503, "sl503.txt");
cout << "system:重载sl501.txt成功!!!" << endl;
cout << "system:重载sl502.txt成功!!!" << endl;
cout << "system:重载sl503.txt成功!!!" << endl;
system("pause");
system("cls");
}
void Manager::manager9() {
cout << "system:请输入新的管理员账号:";
string s;
cin >> s;
this->admin_name = s;
Protect();
cout << "system:请输入管理员 "<< s << " 的密码:";
Protect();
cin >> s;
Protect();
this->admin_password = s;
cout << "system:修改成功!!!" << endl;
writeadmin();
cout << "system:写入文件admin.txt成功!!!" << endl;
system("pause");
system("cls");
}
void Manager::RELOADING() {
cout << "system:系统初始化ing......" << endl;
readfile("admin.txt");
readfile("teacher.txt");
readfile("student.txt");
readfile("sl501.txt");
readfile("sl502.txt");
readfile("sl503.txt");
readstudent();
readteacher();
readadmin();
readsl(sl501, "sl501.txt");
readsl(sl502, "sl502.txt");
readsl(sl503, "sl503.txt");
cout << "system:系统初始化完成!!!" << endl;
system("pause");
system("cls");
}
string Manager::get_time(int week, int time) {
string s = "星期";
switch (week) {
case 1:
s += "一";
break;
case 2:
s += "二";
break;
case 3:
s += "三";
break;
case 4:
s += "四";
break;
case 5:
s += "五";
break;
case 6:
s += "六";
break;
case 7:
s += "日";
break;
}
switch (time) {
case 1:
s += "上午";
break;
case 2:
s += "下午";
break;
case 3:
s += "晚上";
break;
}
return s;
}
void Manager::Protect() {
writeadmin();
writestudent();
writeteacher();
writesl(sl501, "sl501.txt");
writesl(sl502, "sl502.txt");
writesl(sl503, "sl503.txt");
}
// THE END
(3)main.cpp
/*
开发项目:【黑马程序员~C++结课程序大设计】机房预约系统
程序结构:main.cpp (主程序) manager.h(Manager类的头文件) manager.cpp(Manager的成员函数存储)
开发语言:C++ 开发环境:Windows Visual Studio 2022
程序实现:可以实现对三个机房一周每天三个时间段的空位预约,可以记录学生,教师,管理员账号和密码,利用STL容器实现,
可以存储至本地文件,可完成写入与读取,管理员支持添加账号,删除账号,查看特定账号的信息及其预约情况,更
改账号信息,查看所有用户信息,查看特定时间段机房预约情况,可以清空预约,清除用户,更改管理员账号密码,
教师支持查看学生的所有预约,审批学生的预约以及更改自己账户的密码,学生则支持申请预约,查看指定预约,查
看所有预约,取消预约,更改自身密码
程序特性:本程序重心在于STL容器的应用,类的使用与文件写入读取,本程序逻辑复杂,写入数据不易实现,文件写入原理如
下:管理员:只有一位,直接在admin.txt放入两行数据即可(第一行账号第二行密码)教师:由于教师不需要预约
,所以教师的数据是两两一组,一行账号一行密码;但学生就较为复杂,我先读取一个标识符“account”为一个学生
的开端,紧接着的是用户名和密码,在接下来是预约数据,两两一组,到下一个account为止;在机房文件中是一个
由星期和时间段换算而来的序号和剩余空位的结合体,换算规则为number=(week - 1)*3 +time ;其实最重要的
是学生预约数据的存储,我采用数字存储的方式完成,第一行为三到四位数,最后一位是审核状态(0 失败 1 未审
核 2 成功)倒数第二位是机房号(1 sl501 2 sl502 3 sl503)剩下的便是前面星期和时间段换算而来的结果,第
二行是人数(因为位数不固定,故单独储存)在设计完成后我又加入了文件实时检测,是由不断把内存容器内的数据
输出完成的,这样可以有效避免在程序运行时的恶意纂改
*/
/*
完成时间:2023年1月14日00:27:16 当前版本:V1.0
发布时间:2023年1月14日00:39:48
更新日志:无
*/
#include "manager.h"
int main() {
Manager manager;
int in = 0;
manager.RELOADING();
while (true) {
manager.showmenu();
manager.Protect();
cout << "system:请输入您的操作代码:";
cin >> in;
switch (in) {
case 1 :
manager.text01();
break;
case 2:
manager.text02();
break;
case 3:
manager.text03();
break;
case 0:
cout << "system:感谢使用,下次再会!" << endl;
system("pause");
system("cls");
return 0;
default:
cout << "system:输入有误,请检查后重新输入!" << endl;
system("pause");
system("cls");
break;
}
}
}
(五)版权声明:
此程序完全由本人制作,并且仅在CSDN平台发布,虽然我是个小菜鸡,但也是不能商用的哦嘿嘿
(六)结语
C++黑马程序员三百多节课程正式完结撒花!!!接下来准备把书通读一遍,年前完成!!加油!!!!!