(一)作者瞎叨叨:
今天有点事儿耽搁了一下~但还是来更新啦,今天呢其实已经把黑马程序员C++课程刷完啦,共计三百多集,很有成就感呢!这次完成的是C++提高编程中的结课程序设计,依旧是那句!初来乍到,请大家多多关照啦~
——Agoni酱
(二)设计要求:
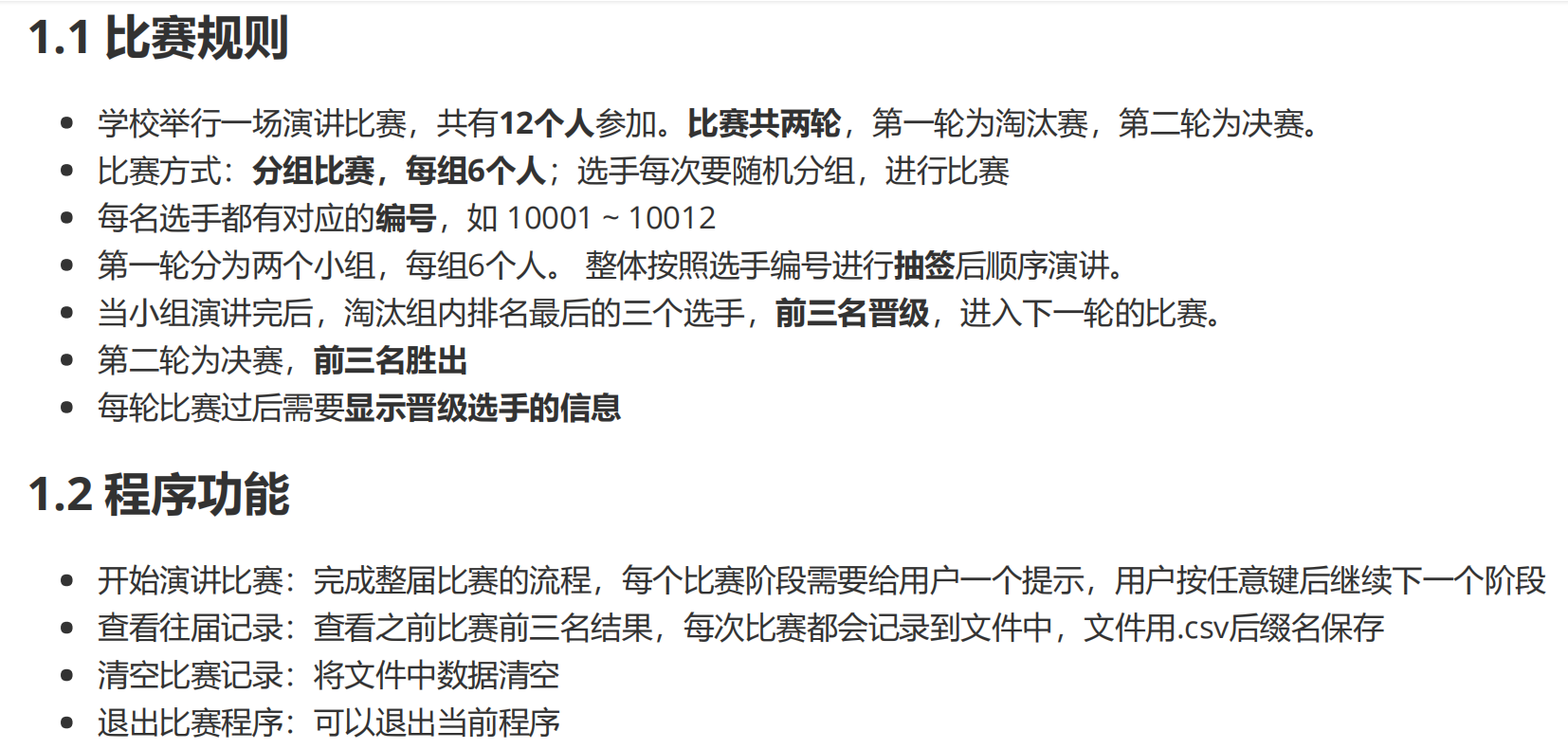
PS:是不是看起来很复杂~但实际上都是自动完成的,不需要使用人有过多操作的
(三)程序相关事项(可见于main.cpp程序文件开头)
/*
开发项目:【黑马程序员~C++提高编程程序设计】基于STL的演讲比赛流程管理系统
程序结构:main.cpp (主程序) manager.h(Manager类的头文件) manager.cpp(Manager的成员函数存储)
开发语言:C++开发环境:Windows Visual Studio 2022
程序实现:可以自动生成12位员工(10001~10012)并自动随机排序完成出场顺序以及分组,并且使用STL中的各类容器存储数据,结合algorithm、numeric算法库与STL容器,完成评委评价(十位评委,去掉最高分和最低分求平均,完全随机化)以及筛选晋级人员,公示晋级人员进入下一轮,并且再次随机排序决定出场顺序,随后继续完成评委评价,并且排序后颁发奖项,写入文件,并且可以随时调取文件查看历届比赛记录,可以清空记录
程序特性:本程序重心在于STL容器的应用,类的使用,algorithm、numeric算法库的使用,以及对文件的操作,拥有较为完善的检测机制,可以确保文件的有效性,有着较完善的交互机制,能够显而易见地阅览数据
*/
/*
完成时间:2023年1月11日22:39:43 当前版本:V1.0
发布时间:2023年1月11日22:41:19
更新日志:无
*/
(四)嫑废话,上代码!(qq_yang的第一节C语言编程课)
(1)manager.h
#pragma once
#include <iostream>
#include <fstream>
using namespace std;
class Manager {
public:
void showmenu();
void writefile();
void searchfile();
double score(int num);
void error();
bool file_empty;
int line = 0;
void test01();
void test02();
void test03();
};
(2)manager.cpp
#include "manager.h"
#include <map>
#include <ctime>
#include <algorithm>
#include <vector>
#include <set>
#include <numeric>
void Manager::showmenu() {
cout << "****************************************************************************" << endl;
cout << "************************** 1 开始演讲比赛 **********************************" << endl;
cout << "************************** 2 查看往届记录 **********************************" << endl;
cout << "************************** 3 清空比赛记录 **********************************" << endl;
cout << "************************** 0 退出比赛程序 **********************************" << endl;
cout << "************************ 制作:CSDN agoni酱 ********************************" << endl;
cout << "****************************************************************************" << endl;
}
void Manager::error() {
ifstream ifs;
ifs.open("competition.csv", ios::in);
if (!ifs.is_open()) {
cout << "system:[error]程序运行过程中发生文件丢失!请重启此系统!" << endl;
exit(0);
}
ifs.close();
}
double Manager::score(int num) {
multiset<int> score;
if (num == 1) {
for (int i = 0; i < 10; i++) {
score.insert(rand() % 30 + 70);
}
}
else {
for (int i = 0; i < 10; i++) {
score.insert(rand() % 20 + 80);
}
}
return accumulate(++score.begin(), --score.end(), 0) / 8.0;
}
void Manager::test01() {
srand((unsigned int)time(NULL));
vector<int> record, promote;
multimap<double, int> r1, r2, result;
for (int i = 10001; i < 10013; i++) {
record.push_back(i);
}
random_shuffle(record.begin(), record.end());
vector<int>::iterator it = record.begin();
cout << "****************************************************************************" << endl;
cout << "** 第" << line+1 <<"届演讲比赛开始 **" << endl;
cout << "** **" << endl;
cout << "** 第一轮初赛 **" << endl;
cout << "** 第一组 第二组 **" << endl;
cout << "** **" << endl;
for (int i = 0; i < 6; i++) {
r1.insert(make_pair(score(1), *it));
cout << "** 选手编号:" << *it;
it++;
r2.insert(make_pair(score(1), *(it)));
cout << " 选手编号:" << *it << " **" << endl;
it++;
}
cout << "** **" << endl;
multimap<double, int>::iterator it1 = r1.end();
multimap<double, int>::iterator it2 = r2.end();
cout << "** 初赛成绩 **" << endl;
cout << "** 第一组 第二组 **" << endl;
cout << "** **" << endl;
for (int i = 0; i < 6; i++) {
it1--;
it2--;
if (i == 3) {
cout << "** -------------------------------------------------------------------- **" << endl;
}
if (i > 2) {
cout << "** 选手编号:" << it1->second << " 成绩:" << it1->first << "\t | 选手编号:" << it2->second << "\t成绩:" << it2->first << " \t **" << endl;
}
else {
cout << "** 选手编号:" << it1->second << " 成绩:" << it1->first << "\t | 选手编号:" << it2->second << "\t成绩:" << it2->first << " \t **" << endl;
promote.push_back(it1->second);
promote.push_back(it2->second);
}
}
cout << "** **" << endl;
cout << "****************************************************************************" << endl;
cout << endl << "system:按任意键开始第二轮演讲比赛" << endl;
system("pause");
cout << "****************************************************************************" << endl;
cout << "** 第二轮决赛 **" << endl;
cout << "** 决赛名单 **" << endl;
cout << "** **" << endl;
random_shuffle(promote.begin(), promote.end());
it = promote.begin();
for (int i = 0; i < 6; i++) {
result.insert(make_pair(score(2), *it));
cout << "** "<< "选手编号:" << *it <<" **" << endl;
it++;
}
cout << "** **" << endl;
multimap<double, int>::iterator itresult = result.end(),first,second,third;
cout << "** 决赛成绩 **" << endl;
cout << "** **" << endl;
for (int i = 0; i < 6; i++) {
itresult--;
if (i == 3) {
cout << "** -------------------------------------------------------------------- **" << endl;
}
cout << "**\t\t\t选手编号:" << itresult->second << "\t最终成绩:" << itresult->first << "\t\t\t **" << endl;
}
cout << "** **" << endl;
cout << "****************************************************************************" << endl;
first = result.end();
second = --first;
third = --second;
third--;
cout << "system:开始写入competition.csv" << endl;
this->error();
ofstream ofs;
ofs.open("competition.csv", ios::out | ios::app);
ofs << "第 " << line + 1 << " 届演讲比赛:\t冠军:" << first->second << " 最终成绩:" << first->first
<< "\t亚军:" << second->second << " 最终成绩:" << second->first
<< "\t季军:" << third->second << " 最终成绩:" << third->first << endl;
ofs.close();
this->file_empty = false;
this->line++;
cout << "system:写入competition.csv成功!" << endl;
system("pause");
system("cls");
this->showmenu();
}
void Manager::test02() {
this->error();
if (this->file_empty) {
cout << "system:文件为空!!!" << endl;
return;
}
ifstream ifs;
ifs.open("competition.csv", ios::in);
char buf[1024] = { 0 };
while (ifs.getline(buf,sizeof(buf)))
{
cout << buf << endl;
}
ifs.close();
system("pause");
system("cls");
this->showmenu();
}
void Manager::searchfile() {
ifstream ifs;
ifs.open("competition.csv", ios::in);
if (!ifs.is_open()) {
cout << "system:文件不存在,已为您创建competition.csv" << endl;
ofstream ofs;
ofs.open("competition.csv", ios::out);
ofs.close();
}
char buf[1024] = { 0 };
while (ifs.getline(buf, sizeof(buf)))
{
this->line++;
}
if (line > 0) {
this->file_empty = false;
}
else {
this->file_empty = true;
}
cout << "system:文件初始化加载成功!!!" << endl;
ifs.close();
}
void Manager::test03()
{
if (this->file_empty) {
cout << "system:文件为空!无需清除!!!" << endl;
return;
}
cout << "system:是否确定清空文件?(1 是 其他 否)" ;
int select = 0;
cin >> select;
if (select == 1)
{
ofstream ofs("competition.csv", ios::trunc);
ofs.close();
this->file_empty = true;
this->line = 0;
cout << "system:清空成功!" << endl;
}
system("pause");
system("cls");
this->showmenu();
}
(3)main.cpp
/*
开发项目:【黑马程序员~C++提高编程程序设计】基于STL的演讲比赛流程管理系统
程序结构:main.cpp (主程序) manager.h(Manager类的头文件) manager.cpp(Manager的成员函数存储)
开发语言:C++ 开发环境:Windows Visual Studio 2022
程序实现:可以自动生成12位员工(10001~10012)并自动随机排序完成出场顺序以及分组,并且使用STL中的各类容器存储数据,
结合algorithm、numeric算法库与STL容器,完成评委评价(十位评委,去掉最高分和最低分求平均,完全随机化)
以及筛选晋级人员,公示晋级人员进入下一轮,并且再次随机排序决定出场顺序,随后继续完成评委评价,并且排序后
颁发奖项,写入文件,并且可以随时调取文件查看历届比赛记录,可以清空记录
程序特性:本程序重心在于STL容器的应用,类的使用,algorithm、numeric算法库的使用,以及对文件的操作,拥有较为完善
的检测机制,可以确保文件的有效性,有着较完善的交互机制,能够显而易见地阅览数据
*/
/*
完成时间:2023年1月11日22:39:43 当前版本:V1.0
发布时间:2023年1月11日22:41:19
更新日志:无
*/
#include "manager.h"
int main() {
Manager manager;
manager.showmenu();
int in = 0;
manager.searchfile();
while (true) {
cout << "system:请输入您的操作指令:";
cin >> in;
switch (in) {
case 1:
manager.test01();
break;
case 2:
manager.test02();
break;
case 3:
manager.test03();
break;
case 0:
cout << "system:期待您下次使用!再见!" << endl ;
system("pause");
return 0;
default:
cout << "system:您输入操作指令有误!请重新输入!" << endl ;
break;
}
}
system("pause");
return 0;
}
// THE END
(五)版权声明:
此程序完全由本人制作,并且仅在CSDN平台发布,虽然我是个小菜鸡,但也是不能商用的哦嘿嘿
(六)结语
终于(自己背着qq_yang看的)课程结束了!!!明天完成课程大设计就可以啦!再接再厉!