// Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Notepad
{
static class Program
{
/// <summary>
/// 应用程序的主入口点。
/// </summary>
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new frmNotepad());
}
}
}
//Form1.Designer.cs
namespace Notepad
{
partial class frmNotepad
{
/// <summary>
/// 必需的设计器变量。
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// 清理所有正在使用的资源。
/// </summary>
/// <param name="disposing">如果应释放托管资源,为 true;否则为 false。</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows 窗体设计器生成的代码
/// <summary>
/// 设计器支持所需的方法 - 不要修改
/// 使用代码编辑器修改此方法的内容。
/// </summary>
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
System.ComponentModel.ComponentResourceManager resources = new System.ComponentModel.ComponentResourceManager(typeof(frmNotepad));
this.mnusNotepad = new System.Windows.Forms.MenuStrip();
this.tsmiFile = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiNew = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiOpen = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiSave = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiSaveAs = new System.Windows.Forms.ToolStripMenuItem();
this.toolStripMenuItem1 = new System.Windows.Forms.ToolStripSeparator();
this.tsmiClose = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiEdit = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiUndo = new System.Windows.Forms.ToolStripMenuItem();
this.toolStripMenuItem2 = new System.Windows.Forms.ToolStripSeparator();
this.tsmiCopy = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiCut = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiPaste = new System.Windows.Forms.ToolStripMenuItem();
this.toolStripMenuItem3 = new System.Windows.Forms.ToolStripSeparator();
this.tsmiSelectAll = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiDate = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiFormat = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiAuto = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiFont = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiView = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiToolStrip = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiStatusStrip = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiHelp = new System.Windows.Forms.ToolStripMenuItem();
this.tsmiAbout = new System.Windows.Forms.ToolStripMenuItem();
this.tlsNotepad = new System.Windows.Forms.ToolStrip();
this.新建NToolStripButton = new System.Windows.Forms.ToolStripButton();
this.打开OToolStripButton = new System.Windows.Forms.ToolStripButton();
this.保存SToolStripButton = new System.Windows.Forms.ToolStripButton();
this.打印PToolStripButton = new System.Windows.Forms.ToolStripButton();
this.toolStripSeparator = new System.Windows.Forms.ToolStripSeparator();
this.剪切UToolStripButton = new System.Windows.Forms.ToolStripButton();
this.复制CToolStripButton = new System.Windows.Forms.ToolStripButton();
this.粘贴PToolStripButton = new System.Windows.Forms.ToolStripButton();
this.toolStripSeparator1 = new System.Windows.Forms.ToolStripSeparator();
this.帮助LToolStripButton = new System.Windows.Forms.ToolStripButton();
this.rtxtNotepad = new System.Windows.Forms.RichTextBox();
this.stsNotepad = new System.Windows.Forms.StatusStrip();
this.tssLbl1 = new System.Windows.Forms.ToolStripStatusLabel();
this.tssLbl2 = new System.Windows.Forms.ToolStripStatusLabel();
this.odlgNotepad = new System.Windows.Forms.OpenFileDialog();
this.sdlgNotepad = new System.Windows.Forms.SaveFileDialog();
this.fdlgNotepad = new System.Windows.Forms.FontDialog();
this.tmrNotepad = new System.Windows.Forms.Timer(this.components);
this.tmrNotepad2 = new System.Windows.Forms.Timer(this.components);
this.mnusNotepad.SuspendLayout();
this.tlsNotepad.SuspendLayout();
this.stsNotepad.SuspendLayout();
this.SuspendLayout();
//
// mnusNotepad
//
this.mnusNotepad.ImageScalingSize = new System.Drawing.Size(20, 20);
this.mnusNotepad.Items.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.tsmiFile,
this.tsmiEdit,
this.tsmiFormat,
this.tsmiView,
this.tsmiHelp});
this.mnusNotepad.Location = new System.Drawing.Point(0, 0);
this.mnusNotepad.Name = "mnusNotepad";
this.mnusNotepad.Size = new System.Drawing.Size(600, 28);
this.mnusNotepad.TabIndex = 1;
this.mnusNotepad.Text = "menuStrip2";
//
// tsmiFile
//
this.tsmiFile.DropDownItems.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.tsmiNew,
this.tsmiOpen,
this.tsmiSave,
this.tsmiSaveAs,
this.toolStripMenuItem1,
this.tsmiClose});
this.tsmiFile.Name = "tsmiFile";
this.tsmiFile.ShowShortcutKeys = false;
this.tsmiFile.Size = new System.Drawing.Size(71, 24);
this.tsmiFile.Text = "文件(F)";
this.tsmiFile.Click += new System.EventHandler(this.文件ToolStripMenuItem_Click);
//
// tsmiNew
//
this.tsmiNew.Name = "tsmiNew";
this.tsmiNew.ShortcutKeys = ((System.Windows.Forms.Keys)((System.Windows.Forms.Keys.Control | System.Windows.Forms.Keys.N)));
this.tsmiNew.Size = new System.Drawing.Size(224, 26);
this.tsmiNew.Text = "新建(&N)";
this.tsmiNew.Click += new System.EventHandler(this.tsmiNew_Click);
//
// tsmiOpen
//
this.tsmiOpen.Name = "tsmiOpen";
this.tsmiOpen.ShortcutKeys = ((System.Windows.Forms.Keys)((System.Windows.Forms.Keys.Control | System.Windows.Forms.Keys.O)));
this.tsmiOpen.Size = new System.Drawing.Size(224, 26);
this.tsmiOpen.Text = "打开(&O)";
this.tsmiOpen.Click += new System.EventHandler(this.新建NCtrlNToolStripMenuItem_Click);
//
// tsmiSave
//
this.tsmiSave.Name = "tsmiSave";
this.tsmiSave.ShortcutKeys = ((System.Windows.Forms.Keys)((System.Windows.Forms.Keys.Control | System.Windows.Forms.Keys.S)));
this.tsmiSave.Size = new System.Drawing.Size(224, 26);
this.tsmiSave.Text = "保存(&S) ";
this.tsmiSave.Click += new System.EventHandler(this.tsmiSave_Click);
//
// tsmiSaveAs
//
this.tsmiSaveAs.Name = "tsmiSaveAs";
this.tsmiSaveAs.Size = new System.Drawing.Size(224, 26);
this.tsmiSaveAs.Text = "另存为(&A)";
this.tsmiSaveAs.Click += new System.EventHandler(this.tsmiSaveAs_Click);
//
// toolStripMenuItem1
//
this.toolStripMenuItem1.Name = "toolStripMenuItem1";
this.toolStripMenuItem1.Size = new System.Drawing.Size(221, 6);
//
// tsmiClose
//
this.tsmiClose.Name = "tsmiClose";
this.tsmiClose.ShowShortcutKeys = false;
this.tsmiClose.Size = new System.Drawing.Size(224, 26);
this.tsmiClose.Text = "退出(&X)";
this.tsmiClose.Click += new System.EventHandler(this.tsmiClose_Click);
//
// tsmiEdit
//
this.tsmiEdit.DropDownItems.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.tsmiUndo,
this.toolStripMenuItem2,
this.tsmiCopy,
this.tsmiCut,
this.tsmiPaste,
this.toolStripMenuItem3,
this.tsmiSelectAll,
this.tsmiDate});
this.tsmiEdit.Name = "tsmiEdit";
this.tsmiEdit.ShowShortcutKeys = false;
this.tsmiEdit.Size = new System.Drawing.Size(71, 24);
this.tsmiEdit.Text = "编辑(E)";
this.tsmiEdit.Click += new System.EventHandler(this.编辑EToolStripMenuItem_Click);
//
// tsmiUndo
//
this.tsmiUndo.Name = "tsmiUndo";
this.tsmiUndo.ShortcutKeys = ((System.Windows.Forms.Keys)((System.Windows.Forms.Keys.Control | System.Windows.Forms.Keys.Z)));
this.tsmiUndo.Size = new System.Drawing.Size(200, 26);
this.tsmiUndo.Text = "撤销(&U)";
this.tsmiUndo.Click += new System.EventHandler(this.tsmiUndo_Click);
//
// toolStripMenuItem2
//
this.toolStripMenuItem2.Name = "toolStripMenuItem2";
this.toolStripMenuItem2.Size = new System.Drawing.Size(197, 6);
//
// tsmiCopy
//
this.tsmiCopy.Name = "tsmiCopy";
this.tsmiCopy.ShortcutKeys = ((System.Windows.Forms.Keys)((System.Windows.Forms.Keys.Control | System.Windows.Forms.Keys.C)));
this.tsmiCopy.Size = new System.Drawing.Size(200, 26);
this.tsmiCopy.Text = "复制(&C)";
this.tsmiCopy.Click += new System.EventHandler(this.tsmiCopy_Click);
//
// tsmiCut
//
this.tsmiCut.Name = "tsmiCut";
this.tsmiCut.ShortcutKeys = ((System.Windows.Forms.Keys)((System.Windows.Forms.Keys.Control | System.Windows.Forms.Keys.X)));
this.tsmiCut.Size = new System.Drawing.Size(200, 26);
this.tsmiCut.Text = "剪切(&T)";
this.tsmiCut.Click += new System.EventHandler(this.tsmiCut_Click);
//
// tsmiPaste
//
this.tsmiPaste.Name = "tsmiPaste";
this.tsmiPaste.ShortcutKeys = ((System.Windows.Forms.Keys)((System.Windows.Forms.Keys.Control | System.Windows.Forms.Keys.V)));
this.tsmiPaste.Size = new System.Drawing.Size(200, 26);
this.tsmiPaste.Text = "粘贴(&P)";
this.tsmiPaste.Click += new System.EventHandler(this.tsmiPaste_Click);
//
// toolStripMenuItem3
//
this.toolStripMenuItem3.Name = "toolStripMenuItem3";
this.toolStripMenuItem3.Size = new System.Drawing.Size(197, 6);
//
// tsmiSelectAll
//
this.tsmiSelectAll.Name = "tsmiSelectAll";
this.tsmiSelectAll.ShortcutKeys = ((System.Windows.Forms.Keys)((System.Windows.Forms.Keys.Control | System.Windows.Forms.Keys.A)));
this.tsmiSelectAll.Size = new System.Drawing.Size(200, 26);
this.tsmiSelectAll.Text = "全选(&A)";
this.tsmiSelectAll.Click += new System.EventHandler(this.tsmiSelectAll_Click);
//
// tsmiDate
//
this.tsmiDate.Name = "tsmiDate";
this.tsmiDate.ShortcutKeys = System.Windows.Forms.Keys.F5;
this.tsmiDate.Size = new System.Drawing.Size(200, 26);
this.tsmiDate.Text = "日期(&D)";
this.tsmiDate.Click += new System.EventHandler(this.tsmiDate_Click);
//
// tsmiFormat
//
this.tsmiFormat.DropDownItems.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.tsmiAuto,
this.tsmiFont});
this.tsmiFormat.Name = "tsmiFormat";
this.tsmiFormat.Size = new System.Drawing.Size(75, 24);
this.tsmiFormat.Text = "格式(O)";
//
// tsmiAuto
//
this.tsmiAuto.Checked = true;
this.tsmiAuto.CheckState = System.Windows.Forms.CheckState.Checked;
this.tsmiAuto.Name = "tsmiAuto";
this.tsmiAuto.Size = new System.Drawing.Size(177, 26);
this.tsmiAuto.Text = "自动换行(&W)";
this.tsmiAuto.Click += new System.EventHandler(this.tsmiAuto_Click);
//
// tsmiFont
//
this.tsmiFont.Name = "tsmiFont";
this.tsmiFont.Size = new System.Drawing.Size(177, 26);
this.tsmiFont.Text = "字体(&F)";
this.tsmiFont.Click += new System.EventHandler(this.tsmiFont_Click);
//
// tsmiView
//
this.tsmiView.DropDownItems.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.tsmiToolStrip,
this.tsmiStatusStrip});
this.tsmiView.Name = "tsmiView";
this.tsmiView.Size = new System.Drawing.Size(73, 24);
this.tsmiView.Text = "查看(V)";
this.tsmiView.Click += new System.EventHandler(this.tsmiView_Click);
//
// tsmiToolStrip
//
this.tsmiToolStrip.Checked = true;
this.tsmiToolStrip.CheckState = System.Windows.Forms.CheckState.Checked;
this.tsmiToolStrip.Name = "tsmiToolStrip";
this.tsmiToolStrip.Size = new System.Drawing.Size(156, 26);
this.tsmiToolStrip.Text = "工具栏(&T)";
this.tsmiToolStrip.Click += new System.EventHandler(this.tsmiToolStrip_Click);
//
// tsmiStatusStrip
//
this.tsmiStatusStrip.Checked = true;
this.tsmiStatusStrip.CheckState = System.Windows.Forms.CheckState.Checked;
this.tsmiStatusStrip.Name = "tsmiStatusStrip";
this.tsmiStatusStrip.Size = new System.Drawing.Size(156, 26);
this.tsmiStatusStrip.Text = "状态栏(&S)";
this.tsmiStatusStrip.Click += new System.EventHandler(this.tsmiStatusStrip_Click);
//
// tsmiHelp
//
this.tsmiHelp.DropDownItems.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.tsmiAbout});
this.tsmiHelp.Name = "tsmiHelp";
this.tsmiHelp.Size = new System.Drawing.Size(75, 24);
this.tsmiHelp.Text = "帮助(H)";
//
// tsmiAbout
//
this.tsmiAbout.Name = "tsmiAbout";
this.tsmiAbout.Size = new System.Drawing.Size(188, 26);
this.tsmiAbout.Text = "关于记事本(&A)";
this.tsmiAbout.Click += new System.EventHandler(this.tsmiAbout_Click);
//
// tlsNotepad
//
this.tlsNotepad.ImageScalingSize = new System.Drawing.Size(20, 20);
this.tlsNotepad.Items.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.新建NToolStripButton,
this.打开OToolStripButton,
this.保存SToolStripButton,
this.打印PToolStripButton,
this.toolStripSeparator,
this.剪切UToolStripButton,
this.复制CToolStripButton,
this.粘贴PToolStripButton,
this.toolStripSeparator1,
this.帮助LToolStripButton});
this.tlsNotepad.Location = new System.Drawing.Point(0, 28);
this.tlsNotepad.Name = "tlsNotepad";
this.tlsNotepad.Size = new System.Drawing.Size(600, 27);
this.tlsNotepad.TabIndex = 2;
this.tlsNotepad.Text = "toolStrip1";
this.tlsNotepad.ItemClicked += new System.Windows.Forms.ToolStripItemClickedEventHandler(this.tlsNotepad_ItemClicked);
//
// 新建NToolStripButton
//
this.新建NToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image;
this.新建NToolStripButton.Image = ((System.Drawing.Image)(resources.GetObject("新建NToolStripButton.Image")));
this.新建NToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta;
this.新建NToolStripButton.Name = "新建NToolStripButton";
this.新建NToolStripButton.Size = new System.Drawing.Size(29, 24);
this.新建NToolStripButton.Text = "新建(&N)";
this.新建NToolStripButton.Click += new System.EventHandler(this.新建NToolStripButton_Click);
//
// 打开OToolStripButton
//
this.打开OToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image;
this.打开OToolStripButton.Image = ((System.Drawing.Image)(resources.GetObject("打开OToolStripButton.Image")));
this.打开OToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta;
this.打开OToolStripButton.Name = "打开OToolStripButton";
this.打开OToolStripButton.Size = new System.Drawing.Size(29, 24);
this.打开OToolStripButton.Text = "打开(&O)";
//
// 保存SToolStripButton
//
this.保存SToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image;
this.保存SToolStripButton.Image = ((System.Drawing.Image)(resources.GetObject("保存SToolStripButton.Image")));
this.保存SToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta;
this.保存SToolStripButton.Name = "保存SToolStripButton";
this.保存SToolStripButton.Size = new System.Drawing.Size(29, 24);
this.保存SToolStripButton.Text = "保存(&S)";
//
// 打印PToolStripButton
//
this.打印PToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image;
this.打印PToolStripButton.Image = ((System.Drawing.Image)(resources.GetObject("打印PToolStripButton.Image")));
this.打印PToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta;
this.打印PToolStripButton.Name = "打印PToolStripButton";
this.打印PToolStripButton.Size = new System.Drawing.Size(29, 24);
this.打印PToolStripButton.Text = "打印(&P)";
//
// toolStripSeparator
//
this.toolStripSeparator.Name = "toolStripSeparator";
this.toolStripSeparator.Size = new System.Drawing.Size(6, 27);
//
// 剪切UToolStripButton
//
this.剪切UToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image;
this.剪切UToolStripButton.Image = ((System.Drawing.Image)(resources.GetObject("剪切UToolStripButton.Image")));
this.剪切UToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta;
this.剪切UToolStripButton.Name = "剪切UToolStripButton";
this.剪切UToolStripButton.Size = new System.Drawing.Size(29, 24);
this.剪切UToolStripButton.Text = "剪切(&U)";
//
// 复制CToolStripButton
//
this.复制CToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image;
this.复制CToolStripButton.Image = ((System.Drawing.Image)(resources.GetObject("复制CToolStripButton.Image")));
this.复制CToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta;
this.复制CToolStripButton.Name = "复制CToolStripButton";
this.复制CToolStripButton.Size = new System.Drawing.Size(29, 24);
this.复制CToolStripButton.Text = "复制(&C)";
//
// 粘贴PToolStripButton
//
this.粘贴PToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image;
this.粘贴PToolStripButton.Image = ((System.Drawing.Image)(resources.GetObject("粘贴PToolStripButton.Image")));
this.粘贴PToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta;
this.粘贴PToolStripButton.Name = "粘贴PToolStripButton";
this.粘贴PToolStripButton.Size = new System.Drawing.Size(29, 24);
this.粘贴PToolStripButton.Text = "粘贴(&P)";
//
// toolStripSeparator1
//
this.toolStripSeparator1.Name = "toolStripSeparator1";
this.toolStripSeparator1.Size = new System.Drawing.Size(6, 27);
//
// 帮助LToolStripButton
//
this.帮助LToolStripButton.DisplayStyle = System.Windows.Forms.ToolStripItemDisplayStyle.Image;
this.帮助LToolStripButton.Image = ((System.Drawing.Image)(resources.GetObject("帮助LToolStripButton.Image")));
this.帮助LToolStripButton.ImageTransparentColor = System.Drawing.Color.Magenta;
this.帮助LToolStripButton.Name = "帮助LToolStripButton";
this.帮助LToolStripButton.Size = new System.Drawing.Size(29, 24);
this.帮助LToolStripButton.Text = "帮助(&L)";
//
// rtxtNotepad
//
this.rtxtNotepad.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
| System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.rtxtNotepad.Location = new System.Drawing.Point(0, 58);
this.rtxtNotepad.Name = "rtxtNotepad";
this.rtxtNotepad.Size = new System.Drawing.Size(600, 318);
this.rtxtNotepad.TabIndex = 3;
this.rtxtNotepad.Text = "";
this.rtxtNotepad.TextChanged += new System.EventHandler(this.rtxtNotepad_TextChanged);
//
// stsNotepad
//
this.stsNotepad.ImageScalingSize = new System.Drawing.Size(20, 20);
this.stsNotepad.Items.AddRange(new System.Windows.Forms.ToolStripItem[] {
this.tssLbl1,
this.tssLbl2});
this.stsNotepad.Location = new System.Drawing.Point(0, 377);
this.stsNotepad.Name = "stsNotepad";
this.stsNotepad.Size = new System.Drawing.Size(600, 26);
this.stsNotepad.TabIndex = 4;
this.stsNotepad.Text = "stsNotepad";
this.stsNotepad.ItemClicked += new System.Windows.Forms.ToolStripItemClickedEventHandler(this.stsNotepad_ItemClicked);
//
// tssLbl1
//
this.tssLbl1.Name = "tssLbl1";
this.tssLbl1.Size = new System.Drawing.Size(39, 20);
this.tssLbl1.Text = "就绪";
//
// tssLbl2
//
this.tssLbl2.Name = "tssLbl2";
this.tssLbl2.Size = new System.Drawing.Size(114, 20);
this.tssLbl2.Text = "显示日期、时间";
this.tssLbl2.Click += new System.EventHandler(this.tssLbl2_Click);
//
// odlgNotepad
//
this.odlgNotepad.FileName = "openFileDialog1";
this.odlgNotepad.Filter = "RTF文件|*.rtf|所有文件|*.*";
//
// sdlgNotepad
//
this.sdlgNotepad.FileName = "无标题";
this.sdlgNotepad.Filter = "RTF文件|*.rtf";
//
// tmrNotepad
//
this.tmrNotepad.Enabled = true;
this.tmrNotepad.Interval = 1000;
//
// tmrNotepad2
//
this.tmrNotepad2.Enabled = true;
this.tmrNotepad2.Interval = 1000;
this.tmrNotepad2.Tick += new System.EventHandler(this.tmrNotepad2_Tick);
//
// frmNotepad
//
this.AutoScaleDimensions = new System.Drawing.SizeF(8F, 15F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(600, 403);
this.Controls.Add(this.stsNotepad);
this.Controls.Add(this.rtxtNotepad);
this.Controls.Add(this.tlsNotepad);
this.Controls.Add(this.mnusNotepad);
this.Name = "frmNotepad";
this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen;
this.Text = "记事本";
this.Load += new System.EventHandler(this.frmNotepad_Load);
this.mnusNotepad.ResumeLayout(false);
this.mnusNotepad.PerformLayout();
this.tlsNotepad.ResumeLayout(false);
this.tlsNotepad.PerformLayout();
this.stsNotepad.ResumeLayout(false);
this.stsNotepad.PerformLayout();
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.MenuStrip mnusNotepad;
private System.Windows.Forms.ToolStripMenuItem tsmiFile;
private System.Windows.Forms.ToolStripMenuItem tsmiNew;
private System.Windows.Forms.ToolStripMenuItem tsmiEdit;
private System.Windows.Forms.ToolStripMenuItem tsmiFormat;
private System.Windows.Forms.ToolStripMenuItem tsmiView;
private System.Windows.Forms.ToolStripMenuItem tsmiHelp;
private System.Windows.Forms.ToolStripMenuItem tsmiOpen;
private System.Windows.Forms.ToolStripMenuItem tsmiSave;
private System.Windows.Forms.ToolStripMenuItem tsmiSaveAs;
private System.Windows.Forms.ToolStripSeparator toolStripMenuItem1;
private System.Windows.Forms.ToolStripMenuItem tsmiClose;
private System.Windows.Forms.ToolStripMenuItem tsmiUndo;
private System.Windows.Forms.ToolStripSeparator toolStripMenuItem2;
private System.Windows.Forms.ToolStripMenuItem tsmiCopy;
private System.Windows.Forms.ToolStripMenuItem tsmiCut;
private System.Windows.Forms.ToolStripMenuItem tsmiPaste;
private System.Windows.Forms.ToolStripSeparator toolStripMenuItem3;
private System.Windows.Forms.ToolStripMenuItem tsmiSelectAll;
private System.Windows.Forms.ToolStripMenuItem tsmiDate;
private System.Windows.Forms.ToolStripMenuItem tsmiAuto;
private System.Windows.Forms.ToolStripMenuItem tsmiFont;
private System.Windows.Forms.ToolStripMenuItem tsmiToolStrip;
private System.Windows.Forms.ToolStripMenuItem tsmiStatusStrip;
private System.Windows.Forms.ToolStripMenuItem tsmiAbout;
private System.Windows.Forms.ToolStrip tlsNotepad;
private System.Windows.Forms.ToolStripButton 新建NToolStripButton;
private System.Windows.Forms.ToolStripButton 打开OToolStripButton;
private System.Windows.Forms.ToolStripButton 保存SToolStripButton;
private System.Windows.Forms.ToolStripButton 打印PToolStripButton;
private System.Windows.Forms.ToolStripSeparator toolStripSeparator;
private System.Windows.Forms.ToolStripButton 剪切UToolStripButton;
private System.Windows.Forms.ToolStripButton 复制CToolStripButton;
private System.Windows.Forms.ToolStripButton 粘贴PToolStripButton;
private System.Windows.Forms.ToolStripSeparator toolStripSeparator1;
private System.Windows.Forms.ToolStripButton 帮助LToolStripButton;
private System.Windows.Forms.RichTextBox rtxtNotepad;
private System.Windows.Forms.StatusStrip stsNotepad;
private System.Windows.Forms.ToolStripStatusLabel tssLbl1;
private System.Windows.Forms.ToolStripStatusLabel tssLbl2;
private System.Windows.Forms.OpenFileDialog odlgNotepad;
private System.Windows.Forms.SaveFileDialog sdlgNotepad;
private System.Windows.Forms.FontDialog fdlgNotepad;
private System.Windows.Forms.Timer tmrNotepad;
private System.Windows.Forms.Timer tmrNotepad2;
}
}
//formAbout.Designer.cs
namespace Notepad
{
partial class frmAbout
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.label1 = new System.Windows.Forms.Label();
this.btnOk = new System.Windows.Forms.Button();
this.linkLabel1 = new System.Windows.Forms.LinkLabel();
this.SuspendLayout();
//
// label1
//
this.label1.AutoSize = true;
this.label1.Font = new System.Drawing.Font("宋体", 18F);
this.label1.Location = new System.Drawing.Point(284, 92);
this.label1.Name = "label1";
this.label1.Size = new System.Drawing.Size(193, 30);
this.label1.TabIndex = 0;
this.label1.Text = "多功能记事本";
//
// btnOk
//
this.btnOk.Font = new System.Drawing.Font("宋体", 14F);
this.btnOk.Location = new System.Drawing.Point(626, 341);
this.btnOk.Name = "btnOk";
this.btnOk.Size = new System.Drawing.Size(150, 40);
this.btnOk.TabIndex = 1;
this.btnOk.Text = "确定";
this.btnOk.UseVisualStyleBackColor = true;
this.btnOk.Click += new System.EventHandler(this.btnOk_Click);
//
// linkLabel1
//
this.linkLabel1.AutoSize = true;
this.linkLabel1.Font = new System.Drawing.Font("宋体", 18F);
this.linkLabel1.Location = new System.Drawing.Point(253, 178);
this.linkLabel1.Name = "linkLabel1";
this.linkLabel1.Size = new System.Drawing.Size(283, 30);
this.linkLabel1.TabIndex = 2;
this.linkLabel1.TabStop = true;
this.linkLabel1.Text = "如有问题,点击这里";
this.linkLabel1.LinkClicked += new System.Windows.Forms.LinkLabelLinkClickedEventHandler(this.linkLabel1_LinkClicked);
//
// frmAbout
//
this.AutoScaleDimensions = new System.Drawing.SizeF(8F, 15F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.AutoSizeMode = System.Windows.Forms.AutoSizeMode.GrowAndShrink;
this.ClientSize = new System.Drawing.Size(800, 450);
this.Controls.Add(this.linkLabel1);
this.Controls.Add(this.btnOk);
this.Controls.Add(this.label1);
this.MaximizeBox = false;
this.Name = "frmAbout";
this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen;
this.Text = "关于记事本";
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label label1;
private System.Windows.Forms.Button btnOk;
private System.Windows.Forms.LinkLabel linkLabel1;
}
}
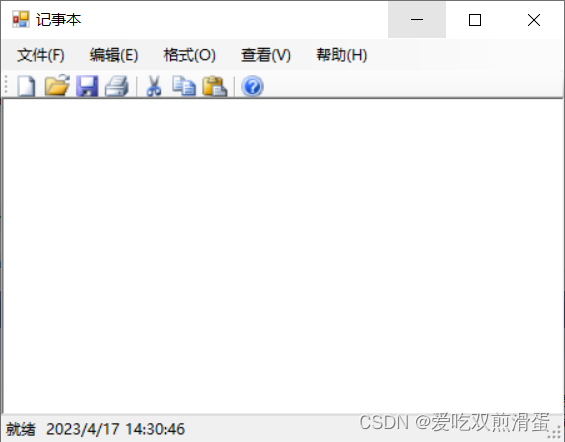
github:https://github.com/ybwell/Notepad/tree/main