javascript与css
目标:
1.使用style属性制作菜单特效;
2.使用className属性制作菜单特效;
3.使用scrollTop制作随鼠标滚动的广告图片;
一、通过js修改元素的样式
<style>
.va{border:1px solid #c00;}
.vb{border:0;}
</style>
<script type="text/javascript">
function mya(obj){
obj.className="va";
}
function myb(obj){
obj.className="vb";
}
</script>
<body>
<div οnmοuseοver="mya(this)" οnmοuseοut="myb(this)"></div>
</body>
二、js菜单
1.写好样式;
2.写好函数;
3.不同事件调用不同函数。
当有很多行代码相同时,例如:
<li οnmοuseοver="mya(this)" οnmοuseοut="myb(this)">第一行</li>
<li οnmοuseοver="mya(this)" οnmοuseοut="myb(this)">第二行</li>
<li οnmοuseοver="mya(this)" οnmοuseοut="myb(this)">第三行</li>
……
以上代码相同的,相同的<li> 和事件
JS简化写思路:
1.获得<li>标签对象集合
2.循环集合中的对象,对象.事件=function(){}
代码:
var arr=document.getElementByTagName("li");
for(var i=0; i<arr.length; i++){
arr[i].className="vb";
arr[i].οnmοuseοver=function(){
this.className="va";
};
arr[i].οnmοuseοut=function(){
this.className="vb";
}
}
三、动态改变文字样式
四、图片跟随滚动条
获取类样式的方法:
currentStyle
getComputedStyle()
网页的三维:x,y,z
层的浮动:position:absolute;
top:20px;
left:20px;
right:20px;
bottom:20px;
滚动事件:
currentStyle
//得到当前样式
currentStyle.top //当前与top
与top的距离
与left的距离
scrollTop滚动距离
<script type="text/javascript">
var va;
var vb;
//得到ID的位置
function myload(){
var da=document.getElementById("id");
va=da.currentStyle.top;
vb=da.currentStyle.left;
alert(va+" "+vb);
}
//得到滚动
function mymove(){
var ga=document.documentElement.scrollTop;
var gb=document.documentElement.scrollLeft;
alert(ga+" "+gb);
}
//window滚动事件
window.οnscrοll=mymove;
</script>
<body οnlοad="myload()">
……
选项框的使用
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
</head>
<body>
<!-- 上海某公司的面试题 全选 -->
<table border="1" cellpadding="0" cellspacing="0" width="60%" >
<tr>
<td><input id="all" type="checkbox">全选</td>
<td><input onclick="myCheck(this,'01',4,6);" type="checkbox">1月</td>
<td><input onclick="myCheck(this,'02',4,6);" type="checkbox">2月</td>
<td><input onclick="myCheck(this,'03',4,6);" type="checkbox">3月</td>
<td><input onclick="myCheck(this,'04',4,6);" type="checkbox">4月</td>
<td><input onclick="myCheck(this,'05',4,6);" type="checkbox">5月</td>
<td><input onclick="myCheck(this,'06',4,6);" type="checkbox">6月</td>
</tr>
<tr>
<td><input onclick="myCheck(this,'2008',0,4)" type="checkbox">2008年</td>
<td><input type="checkbox" value="200801"></td>
<td><input type="checkbox" value="200802"></td>
<td><input type="checkbox" value="200803"></td>
<td><input type="checkbox" value="200804"></td>
<td><input type="checkbox" value="200805"></td>
<td><input type="checkbox" value="200806"></td>
</tr>
<tr>
<td><input onclick="myCheck(this,'2007',0,4)" type="checkbox">2007</td>
<td><input type="checkbox" value="200701"></td>
<td><input type="checkbox" value="200702"></td>
<td><input type="checkbox" value="200703"></td>
<td><input type="checkbox" value="200704"></td>
<td><input type="checkbox" value="200705"></td>
<td><input type="checkbox" value="200706"></td>
</tr>
</table>
<script type="text/javascript">
function $(id){
return document.getElementById(id);
}
// 实现全选 |全不选
$("all").onclick = function(){
var checks = document.querySelectorAll('input');
//遍历
for(var i = 1;i<checks.length;i++){
checks[i].checked = this.checked;
}
};
// 1月 点击事件
// myCheck
function myCheck(obj,m,start,end){
// 获取所有的input复选框
var inputs = document.querySelectorAll("input");
//筛选 获取到value值后如果都是以01结尾的 都是属于每个年份的1月份
for(var i = 0;i<inputs.length;i++){
// console.log(inputs[i].value);
// substring substr 200801
var month = inputs[i].value.substring(start,end);
// console.log(month);
if(month === m){
// console.log(inputs[i])
inputs[i].checked = obj.checked;
}
}
}
</script>
<!-- js -->
<script type="text/javascript">
var result = "akjshdjaasd";
// 从第二个位置开始截取,截取后面四位数
console.log(result.substr(2,4));
// 从第二个位置开始截取,截取到下标为4结束
console.log(result.substring(2,4));
</script>
</body>
</html>
表格与 选项按钮的应用
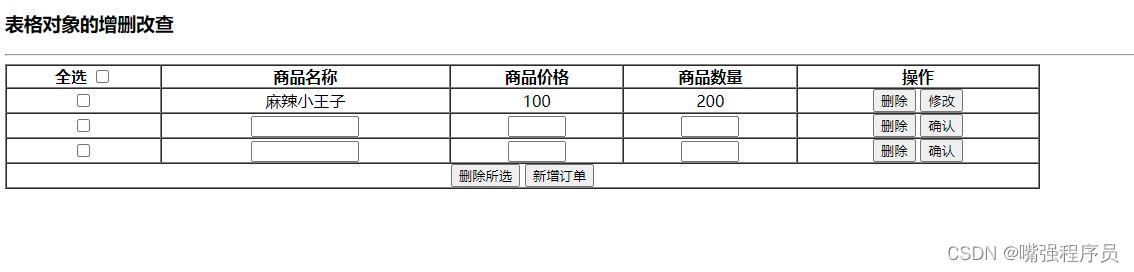
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
table tr {
text-align: center;
}
</style>
</head>
<body>
<h3>表格对象的增删改查</h3>
<hr>
<table border="1" cellpadding="0" cellspacing="0" width="80%">
<tr>
<th>全选 <input type="checkbox"></th>
<th>商品名称</th>
<th>商品价格</th>
<th>商品数量</th>
<th>操作</th>
</tr>
<tr id="1">
<td><input name='sb' type="checkbox" value='1'></td>
<td>麻辣小王子</td>
<td>100</td>
<td>200</td>
<td>
<button onclick="mydel('1')">删除</button>
<button onclick="myEdit('1')">修改</button>
</td>
</tr>
<tr>
<td colspan="5">
<button onclick="plDel();">删除所选</button>
<button onclick="addOrder();">新增订单</button>
</td>
</tr>
</table>
<script type="text/javascript">
//新增订单的点击事件
/*
1.获取表格对象
2.获取指定的位置(tr的下标)
3.调用insertRow(index);
*/
function addOrder() {
// 获取表格对象
var table = document.querySelector("table");
// 获取指定的位置下标
var length = table.rows.length - 1;
// 调用新增tr的方法并且会将tr对象返回
var tr = table.insertRow(length);
tr.id = length;
// 第1个单元格
var td1 = tr.insertCell(0);
td1.innerHTML = "<input name = 'sb' type = 'checkbox' value = '" + tr.id + "'/>"
// 第2个单元格
var td2 = tr.insertCell(1);
td2.innerHTML = "<input type = 'text' style = 'width:100px'>";
// 第3个单元格
var td3 = tr.insertCell(2);
td3.innerHTML = "<input type = 'text' style = 'width:50px'>";
// 第4个单元格
var td4 = tr.insertCell(3);
td4.innerHTML = "<input type = 'text' style = 'width:50px'>";
// 第5个单元格
var td5 = tr.insertCell(4);
td5.innerHTML = "<button onclick = 'mydel(\"" + tr.id + "\")'>删除</button> <button onclick = 'myConfirm(\"" + tr
.id + "\")'>确认</button>";
}
// 确认事件
function myConfirm(id) {
// console.log(typeof(id));
//根据参数id获取当前所点击的tr
var tr = document.getElementById(id);
// console.log(tr);
// 获取第2个单元格中的输入项中的value属性
var gname = tr.cells[1].lastElementChild.value;
// 获取第3个单元格中的输入项中的value属性
var gprice = tr.cells[2].lastElementChild.value;
// 获取第4个单元格中的输入项中的value属性
var gcount = tr.cells[3].lastElementChild.value;
//将获取的结果重新赋值到每一个单元格的文本内容中
tr.cells[1].innerHTML = gname;
tr.cells[2].innerHTML = gprice;
tr.cells[3].innerHTML = gcount;
//将确认按钮改变内容以及事件监听
var confirmbtn = tr.cells[4].lastElementChild;
confirmbtn.innerHTML = "修改";
confirmbtn.setAttribute("onclick", "myEdit('" + id + "')");
}
// 修改事件
function myEdit(id) {
//根据参数id获取当前所点击的tr
var tr = document.getElementById(id);
// 名称
var gname = tr.cells[1].innerHTML;
// 价格
var gprice = tr.cells[2].innerHTML;
// 名称
var gcount = tr.cells[3].innerHTML;
// 将内容设置到每个单元格中输入项中
tr.cells[1].innerHTML = "<input type = 'text' value = '" + gname + "' style = 'width:100px'>";
tr.cells[2].innerHTML = "<input type = 'text' value = '" + gprice + "' style = 'width:50px'>";
tr.cells[3].innerHTML = "<input type = 'text' value = '" + gcount + "' style = 'width:50px'>";
//将修改的按钮的内容以及事件监听
var editbtn = tr.cells[4].lastElementChild;
editbtn.innerHTML = '确认';
editbtn.setAttribute("onclick", "myConfirm('" + id + "')")
}
// 删除的点击事件
function mydel(id) {
//根据参数id获取当前所点击的tr
var tr = document.getElementById(id);
// 获取改行对应的下标
var index = tr.rowIndex;
//获取表格对象
var table = document.querySelector("table");
// 调用deleteRow
table.deleteRow(index);
}
// 批量删除
function plDel() {
// 获取除了全选的所有子复选框
var inputs = document.getElementsByName('sb');
// console.log(inputs);
for (var i = 0; i < inputs.length; i++) {
if (inputs[i].checked == true) {
// console.log(inputs[i]);
// console.log(inputs[i].value);
// 调用删除单个的方法
mydel(inputs[i].value);
// 删除一个后 循环的次数需要减少一次
i--;
}
}
}
</script>
</body>
</html>
js与css 的交互 鼠标触碰变更样式
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
img{width: 100px;height: 100px; border: 3px solid red;}
.over{border: 3px solid yellow;}
.out{border: 3px solid red;}
.oBox{
width: 100%;
height: 40px;
}
*{
margin: 0px;padding: 0px;list-style: none;
}
.oBox ul li{float: left;}
.oBox ul li a{
display: inline-block;
width: 100px;
border: 1px solid red;
text-align: center;
text-decoration: none;
color: black;
background-color: skyblue;
}
.oBox ul li .over1{
background-color: red;
color: blue;
}
.oBox ul li .out1{
background-color: bisque;
color: blue;
}
/* 纯css手法 设置鼠标触碰 */
/* .oBox ul li a:hover{
background-color: yellow;
color: red;
} */
</style>
<script type="text/javascript">
// js与css的交互
// css样式:选择器(ID,class,标签)|属性(字体,边框,背景,列表等)
// 通过js的手段去设置标签的css样式
// 1.通过style对象设置样式,获取(行内式)
// 2.通过className属性设置嵌入式class样式
// style对象手段
window.onload = function(){
//获取所有的img
var oImgs = document.querySelectorAll('img');
//遍历
for(var i = 0;i<oImgs.length;i++){
oImgs[i].setAttribute("index",i);
// 设置点击触碰事件
oImgs[i].onmouseover = function(){
// this.style.border = "3px solid blue";
// oImgs[this.getAttribute('index')].className = "over";
this.className = "over";
};
oImgs[i].onmouseout = function(){
// this.style.border = "3px solid red";
oImgs[this.getAttribute('index')].className = "out";
};
}
};
</script>
</head>
<body>
<img src="img/1.jpg" alt="">
<img src="img/2.jpg" alt="">
<img src="img/3.jpg" alt="">
<img src="img/4.jpg" alt="">
<hr>
<div class="oBox">
<ul>
<li><a href="">恐怖</a></li>
<li><a href="">言情</a></li>
<li><a href="">青春</a></li>
<li><a href="">校园</a></li>
<li><a href="">穿越</a></li>
</ul>
</div>
<script type="text/javascript">
var as = document.querySelectorAll('a');
// console.log(as)
for(var i = 0;i<as.length;i++){
as[i].onmouseover = function(){
console.log(1)
this.className = "over1";
// this.style.backgroundColor = 'red'
};
as[i].onmouseout = function(){
console.log(1)
this.className = "out1";
// this.style.backgroundColor = 'red'
};
}
</script>
</body>
</html>
获取非行装元素的值
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
*{margin: 0px;}
div{
width: 100px;
height: 100px;
background-color: red;
/* 内填充 */
padding: 10px;
border: 10px solid blue;
/* 外边距 */
margin-top: 100px;
/* 定位 */
position: absolute;/* 绝对定位 */
left: 100px;
top: 100px;
}
</style>
</head>
<body>
<div>
内容
</div>
<script type="text/javascript">
// offset 偏移量,获取元素的宽度和高度以及距离页面顶端与左侧端的间距
// 获取div
var oDiv = document.querySelector('div');
// 获取宽度和高度 (width+padding+border) 不包含外边距 不带单位
console.log(oDiv.offsetWidth);
console.log(oDiv.offsetHeight);
//
console.log(oDiv.offsetTop);
console.log(oDiv.offsetLeft)
// client 客户端 可以可视区宽度
// 获取标签的可视区宽度(width+padding)
console.log(oDiv.clientWidth);
console.log(document.body.clientWidth);
console.log(document.documentElement.clientWidth);
// scroll 滚动
window.onscroll = function(){
console.log("我触发了滚动条");
console.log(document.documentElement.scrollTop);
// console.log(document.body.scrollTop);
};
获取标签的 高 宽
var oDiv = document.querySelector('div');
// 获取行内式样式(带单位)
console.log(oDiv.style.width);
// 获取非行内式样式(null)
console.log(oDiv.style.height);
//针对非行内式样式获取的方式
// getComputeStyle(obj,false)[name] 非IE
// currentStyle[name] IE
// console.log(oDiv.currentStyle['height']);
// console.log(getComputedStyle(oDiv,false)['height']);
console.log(getStyle(oDiv,'height'));
// 为了获取非行内式样式 不管使用什么浏览器 封装
// function getStyle(obj,name){
// if(obj.currentStyle){//兼容IE
// return obj.currentStyle[name];
// }else{//非IE
// return getComputedStyle(obj,false)[name];
// }
// }
</script>
</body>
</html>
模拟静止广告
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style type="text/css">
div {
width: 100px;
height: 100px;
background-color: red;
position: absolute;
left: 0px;
top: 200px;
/* 设置和模型的圆角 */
/* border-radius: 5px; */
}
</style>
<!-- 引入封装的js插件 -->
<script src="myjs.js" type="text/javascript" charset="utf-8"></script>
</head>
<body>
<script type="text/javascript">
for (var i = 0; i < 1000; i++) {
document.write("<br>");
}
</script>
<div>
我是漂浮的广告窗口
</div>
<!-- js -->
<script type="text/javascript">
// 获取div
var oDiv = document.querySelector('div');
var mytop = getStyle(oDiv,'top');
console.log("mytop==="+mytop);
// var top = oDiv.offsetTop;
// 滚动监听事件
window.onscroll = function() {
console.log(mytop);
// 当滚动条被滚动时,获取被滚去的距离
var scrollObj = scroll();
console.log(scrollObj.top);
var myscrollTop = scrollObj.top;
// 将盒子div的top值与被滚去的距离相加
var result = parseInt(mytop) + myscrollTop;
console.log(result);
// 将最终的结果赋值给top属性
oDiv.style.top = result + "px";
};
</script>
</body>
</html>
封装的一些 js代码兼容个种浏览器 的方法
function $(id) {
return document.getElementById(id);
}
function getStyle(obj, name) {
if (obj.currentStyle) { //兼容IE
return obj.currentStyle[name];
} else { //非IE
return getComputedStyle(obj, false)[name];
}
}
//封装可视区宽度和高度的一个方法
function client() {
if (window.innerWidth != null) // ie9 + 最新浏览器
{
return {
width: window.innerWidth,
height: window.innerHeight
}
} else if (document.compatMode === "CSS1Compat") // 标准浏览器
{
return {
width: document.documentElement.clientWidth,
height: document.documentElement.clientHeight
}
}
return { // 怪异浏览器
width: document.body.clientWidth,
height: document.body.clientHeight
}
}
/**
* scroll()方法的封装
*/
function scroll() {
//直接return一个json对象
return {
"left": window.pageXOffset || document.documentElement.left || document.body.scrollLeft,
"top": window.pageYOffset || document.documentElement.top || document.body.scrollTop
};
}