前提
当我们使用vuex时,我们会发现所有的状态都存储在state中,当状态很多时store对象就会变得很臃肿。这是因为vuex使用的时使用单一状态树,应用的所有状态会集中到一个比较大的对象。为了解决store对象臃肿的问题,提升可维护性等,我们使用module将需要存储的状态分到不同的模块中。
文件目录
在src文件夹下的store文件夹下新建一个modules文件夹,在这个文件夹下新建一个模块,例如:user.js
模块拆分
user.js的内容可以包含另外四个核心概念并将其抛出,然后在index.js中导入子模块并使用,这里推荐将state作为函数返回,当然也可以用另外三个概念的写法。注意namespaced不要写错了
const mutations = {
updateAge (state, newage) {
state.userInfo.userage = newage
}
}
const actions = {
updateAge2 (context, newage) {
setTimeout(() => {
context.commit('updateAge', newage)
}, 1000)
}
}
const getters = {
filterList (state) {
return state.list.filter(item => item > 5)
}
}
export default {
namespaced: true, // 默认挂载到全局,开启命名空间后挂载到子模块
state () {
return {
userInfo: {
username: '张三',
userage: 8
},
list: [1, 2, 3, 4, 5, 6, 7, 8, 9]
}
},
mutations,
actions,
getters
}
index.js的内容只需要导入user.js并挂载
import { createStore } from 'vuex'
import user from '@/store/modules/user'
export default createStore({
state: {
},
getters: {
},
mutations: {
},
actions: {
},
modules: {
user
}
})
子模块内容使用
state的使用
①直接使用模块名访问
// <div>{{$store.state.模块名.需要使用的状态名}}<div>
<div>
<P>我的用户名:{{$store.state.user.userInfo.username}}</P>
</div>
②通过mapState映射
<template>
<div>
<P>我的用户名:{{$store.state.user.userInfo.username}}</P>
<p>我的用户名:{{ userInfo.username }}</p>
</div>
</template>
<script>
import { mapState } from 'vuex'
export default {
computed: {
...mapState('user', ['userInfo'])
}
}
</script>
<style>
</style>
运行结果
getters的使用
①直接使用模块名访问
// $store.getters['模块名/方法名']
<p>{{ $store.getters['user/filterList'] }}</p>
②通过mapGetters映射
<template>
<div>
<p>{{ filterList }}</p>
</div>
</template>
<script>
import { mapGetters } from 'vuex'
export default {
computed: {
...mapGetters('user', ['filterList'])
},
methods: {
}
}
</script>
<style>
</style>
运行结果
mutations的使用
①直接使用模块名访问
// $store.commit('模块名/方法名', 参数)
<template>
<div>
<P>我的用户名:{{$store.state.user.userInfo.username}}</P>
<p>我的年龄:{{ userInfo.userage }}</p>
<button @click="handleAdd()">改变年龄</button>
</div>
</template>
<script>
import { mapState } from 'vuex'
export default {
computed: {
...mapState('user', ['userInfo'])
},
methods: {
handleAdd () {
this.$store.commit('user/updateAge', 20)
}
}
}
</script>
<style>
</style>
运行结果
②通过mapMutations映射
<template>
<div>
<P>我的用户名:{{$store.state.user.userInfo.username}}</P>
<p>我的年龄:{{ userInfo.userage }}</p>
<button @click="handleAdd()">改变年龄</button>
<button @click="updateAge(25)">改变年龄</button>
</div>
</template>
<script>
import { mapMutations, mapState } from 'vuex'
export default {
computed: {
...mapState('user', ['userInfo'])
},
methods: {
...mapMutations('user', ['updateAge']),
handleAdd () {
this.$store.commit('user/updateAge', 20)
}
}
}
</script>
<style>
</style>
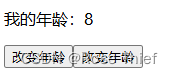
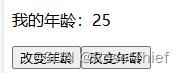
actions的使用
①直接使用模块名访问
<template>
<div>
<P>我的用户名:{{$store.state.user.userInfo.username}}</P>
<p>我的年龄:{{ userInfo.userage }}</p>
<button @click="handleAdd2()">延时改变年龄</button>
</div>
</template>
<script>
import { mapState } from 'vuex'
export default {
computed: {
...mapState('user', ['userInfo'])
},
methods: {
handleAdd2 () {
this.$store.dispatch('user/updateAge2', 20)
}
}
}
</script>
<style>
</style>
②通过mapActions映射
<template>
<div>
<P>我的用户名:{{$store.state.user.userInfo.username}}</P>
<p>我的年龄:{{ userInfo.userage }}</p>
<button @click="updateAge2(25)">延时改变年龄</button>
</div>
</template>
<script>
import { mapActions, mapState } from 'vuex'
export default {
computed: {
...mapState('user', ['userInfo'])
},
methods: {
...mapActions('user', ['updateAge2']),
}
}
</script>
<style>
</style>
完整代码
<template>
<div>
<p>我的用户名:{{$store.state.user.userInfo.username}}</p>
<p>我的年龄:{{ userInfo.userage }}</p>
<button @click="handleAdd()">改变年龄</button>
<button @click="updateAge(25)">改变年龄</button>
<button @click="handleAdd2()">延时改变年龄</button>
<button @click="updateAge2(25)">延时改变年龄</button>
<p>{{ $store.getters['user/filterList'] }}</p>
<p>{{ filterList }}</p>
</div>
</template>
<script>
import { mapActions, mapGetters, mapMutations, mapState } from 'vuex'
export default {
computed: {
...mapState('user', ['userInfo']),
...mapGetters('user', ['filterList'])
},
methods: {
...mapMutations('user', ['updateAge']),
...mapActions('user', ['updateAge2']),
handleAdd () {
this.$store.commit('user/updateAge', 20)
},
handleAdd2 () {
this.$store.dispatch('user/updateAge2', 20)
}
}
}
</script>
<style>
</style>