初用string解题
字符串整形数字
字符串相加【6,24日】
实现大数运算
字符串最后一个单词的长度【6.24日】
里面有刷题知识点见博客第二点刷oj题隐藏知识点
验证子串是否为回文
用string刷题的知识点
string arr[10]={"","","abc","def"}------但参数隐式类型转换
有时候也可以用用,让其作为返回值等
string& operator+
s1 + "abv";
s1 + s2;
string的使用
✳️在使用string类时,必须包含#include头文件以及using namespace std;
✳️string:管理动态增长字符数组,这个字符串以\0结尾
string边边角角
遍历string
法一:下标+【】
for(size_t I = 0; i<s1.size(); I++)
{
cout << s1[i] << " ";-------➡️s1.operator[](i);运算符重载
}
法二:迭代器
迭代器是什么?–➡️像指针一样的东西或者就是指针
string::iterator it = s1.begin();--------➡️要指定类域;
while(it != s1.end())
{
cout << *it << " ";
it++;
}
vector<int>::iterator it
✳️begin与end符合“左闭右开”;
✳️尽量写成“!=”,因为后面其他数据结构的地址并不连续;
法三:范围for-------原理就是迭代器
for (auto ch : s1)
{
cout << ch << " " ;
}
仅仅反转字母题目【6,1日】
string的构造函数
✳️:npos表示是无符号整型的最大数字
string s1;---------➡️常用构造
string s2("hello world");---------➡️常用构造
s2 += "!!!!!";
string s3(s2);---------➡️常用构造
string s6(10, 'x')----➡️10个x来初始化
string s7(s2, 6, 3);----➡️从s2点第六个位置取3个字符来初始化
若不给3则是缺省值npos表示有多少取多少
string s8;还可用迭代器初始化后面讲!
string::operator=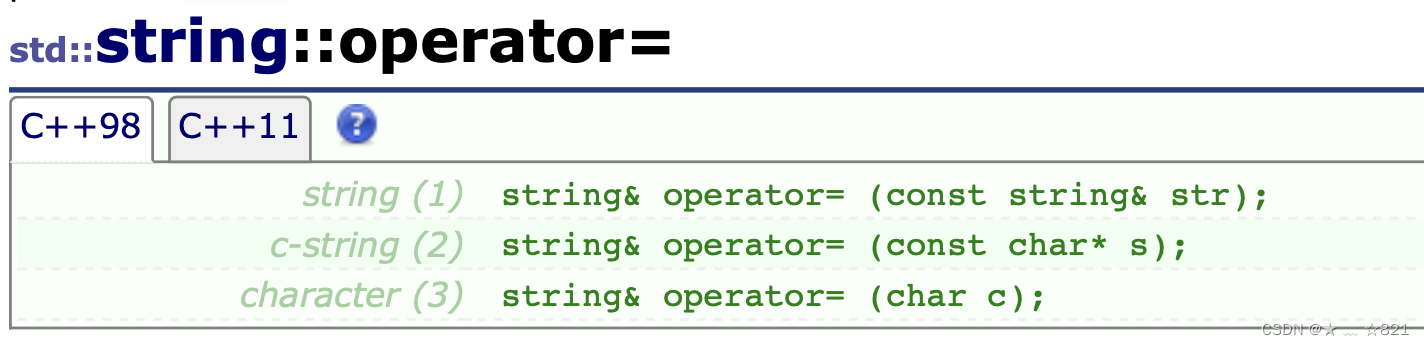
s1 = s2;
s1 = “yyy”;
s1 = 'x';
char& operator[](函数重载)
✳️当然该函数还有其他形式的重载
const char& operator[](int pos)const
✳️支持修改返回对象
string s1("hellow");
s1[2] = 'x';
char& tmp = s1[0];
✳️越界一定会有assert断言错误;
string的迭代器
普通/const迭代器(也有正向/反向)
Func(const string& s)
{
string::iterator it = s.begin();-----❌:因为传来的参数有const
所以迭代器也应该是const类型
string::const_iterator it = s.begin();
}
string s1="hello";
Func(s1);
const string
正向迭代器
string::iterator b_it=s1.begin();
string::iterator e_it=s1.end();
反向迭代器
string::reverse_iterator r_b_it=s1.rebegin();
string::reverse_iterator r_e_it=s1.reend();
利用“auto”
auto it = s1.begin();
sting里的“size()”函数
✳️一般不用:s.lenth(),用s.size()可以与其他的容器用法相同;
✳️:不会算‘\0’;
push_back()函数
验证VS下capacity扩容机制
✳️分析: 插入的时候是要扩容的 ,扩容是有代价的,扩容的话会开新的空间,释放旧的空间,因为不一定能原地扩容,会有性能的损耗若想要减少扩容带来的代价,则下面给出了方法:reserve()函数
✳️在V S下大概是1.5倍的扩容
扩容函数:reserve/resize
string::reserve()【扩容函数】
1.用法:
void reserve (size_t n =0);
2.分析
可以预先扩一定空间,若s.reserve(1000)不一定就只开1000空间,编译器会优化使得内存对齐,但一定会满足你所需要的扩容空间(1000);
string::resize()【扩容➕初始化函数】
1.用法:
void resize (size_t n);
void resize (size_t n, char c);
✳️resize()函数不仅会开空间,还会给你初始化并改变“size”的大小
共异点
a.他们在vs下都不能够缩容;
append()【尾插函数】
✳️同push_back()都是尾插函数;
✳️append是可以加常量字符串;
但是push_back()只能是一个字符;
用法
string& append (const string& str);
string& append (const char* s);
string& append (size_t n, char c);
void push_back (char c);
operator +=() 【最好用的尾插函数】
1.用法:
string& operator+= (const string& str);
string& operator+= (const char* s);
string& operator+= (char c);
2.打样
string s1;
s1 += "hello world";
s1 += s2;
string::insert()函数 【用的少】
1.用法
basic_string& insert (size_type pos, const basic_string& str);
basic_string& insert (size_type pos, size_type n, charT c);
string::erase()函数【用得少】
1.用法:
basic_string& erase (size_type pos = 0, size_type len = npos);
iterator erase (iterator first, iterator last);
string::swap()函数
1.用法:
void swap (basic_string& str);
2.打样
string s1 = "hello world";
string s2 = 'x';
s1.swap(s2);
std中的swap()函数区别
✳️std中的swapC++98中效率低一些,因为它是深拷贝,但C++11引入“右值引用”就不会了
✳️string::swap()是直接交换指针,效率更高
string::c_str()函数
✳️返回字符串的指针,配合C语言很多地方使用;
string::find()/rfind()函数
1.用法:
size_type find (charT c, size_type pos = 0) const;
size_type find (const charT* s, size_type pos, size_type n) const;
size_type find (const charT* s, size_type pos = 0) const;
size_type rfind (charT c, size_type pos = npos) const;
size_type rfind (const charT* s, size_type pos = npos) const;
2.打样
// 要求取出文件的后缀
//string file("string.cpp");
//string file("string.c");
string file("string.c.tar.zip");
//size_t pos = file.find('.');
size_t pos = file.rfind('.');
string::substr()函数【取子串】
1.用法
basic_string substr (size_type pos = 0, size_type len = npos) const;
2.打样
if (pos != string::npos)
{
//string suffix = file.substr(pos, file.size() - pos);
string suffix = file.substr(pos);
cout << file << "后缀:" << suffix << endl;
}
else
{
cout << "没有后缀" << endl;
}
string的深浅拷贝问题
bit::string s1("hello world");
bit::string s2(s1);
cout << s1.c_str() << endl;
cout << s2.c_str() << endl;
我们没写拷贝构造则编译器自己调用默认构造函数,只会完成值拷贝,即为浅拷贝;
浅拷贝危害:同一个空间被析构两次
一个对象修改影响另外一个
string(const string& s)//------一定要用上“&(引用)”
:_str(new char[strlen(s.c_str())+1])
{
strcpy(_str, s.c_str());
}
深拷贝:有一样大的空间,但不是同一个空间、