一、输出指定要求的回文日期
- 2020 年春节期间,有一个特殊的日期引起了大家的注意:
2020 年 2 月 2 日
。因为如果将这个日期按“yyyymmdd
”的格式写成一个8
位数是20200202
,恰好是一个回文数。我们称这样的日期是回文日期(palindromic date)
。有人表示20200202
是“千年一遇”的特殊日子。对此小明很不认同,因为不到 2 年之后就是下一个回文日期:20211202
即 2021年 12月2日。也有人表示 20200202 并不仅仅是一个回文日期,还是一个 ABABBABA
型的回文日期。对此小明也不认同,因为大约 100 年后就能遇到下一个ABABBABA
型的回文日期:21211212
即2121 年 12 月 12 日。算不上“千年一遇”,顶多算“千年两遇”。 - 给定一个 8 位数的日期,请你计算该日期之后下一个回文日期和下一个 ABABBABA 型回文日期各是哪一天。
- 输入描述:输入包含一个八位整数N,表示日期。对于所有评测用例,10000101 ≤ N ≤ 89991231,保证 N 是一个合法日期的 8 位数
- 输出描述:输出两行,每行 1 个八位数。第一行表示下一个回文日期,第二行表示下一个
ABABBABA
型回文日期。
(一)编程实现
1、判断输入日期是否合法
- 创建静态方法
isLegalDate(String strDate)
package p03.t05;
/**
* 功能;输出指定要求的回文日期
* 作者:刘金花
* 日期:2022 年06月08日
*/
public class PalindromicDate {
/**
* 判断日期是否合法
*
* @param strDate
* @return true-合法,false-非法
*/
private static boolean isLegalDate(String strDate) {
int year, month, day;
year = Integer.parseInt(strDate.substring(0, 4));
month = Integer.parseInt(strDate.substring(4, 6));
day = Integer.parseInt(strDate.substring(6));
// 利用反向思维来处理
if (year < 1000 || year > 8999) return false;
if (month < 1 || month > 12) return false;
if (month == 1 || month == 3 || month == 5 || month == 7 || month == 8 || month == 10 || month == 12) {
if (day < 1 || day > 31) return false;
} else if (month == 2) {
if (year % 4 == 0 && year % 100 != 0 || year % 400 == 0) { // 闰年判断
if (day < 1 || day > 29) return false;
} else {
if (day < 1 || day > 28) return false;
}
} else {
if (day < 1 || day > 30) return false;
}
return true;
}
}
2、创建主方法,测试输入日期是否合法
- 判断输入日期是否合法,给出相应的提示
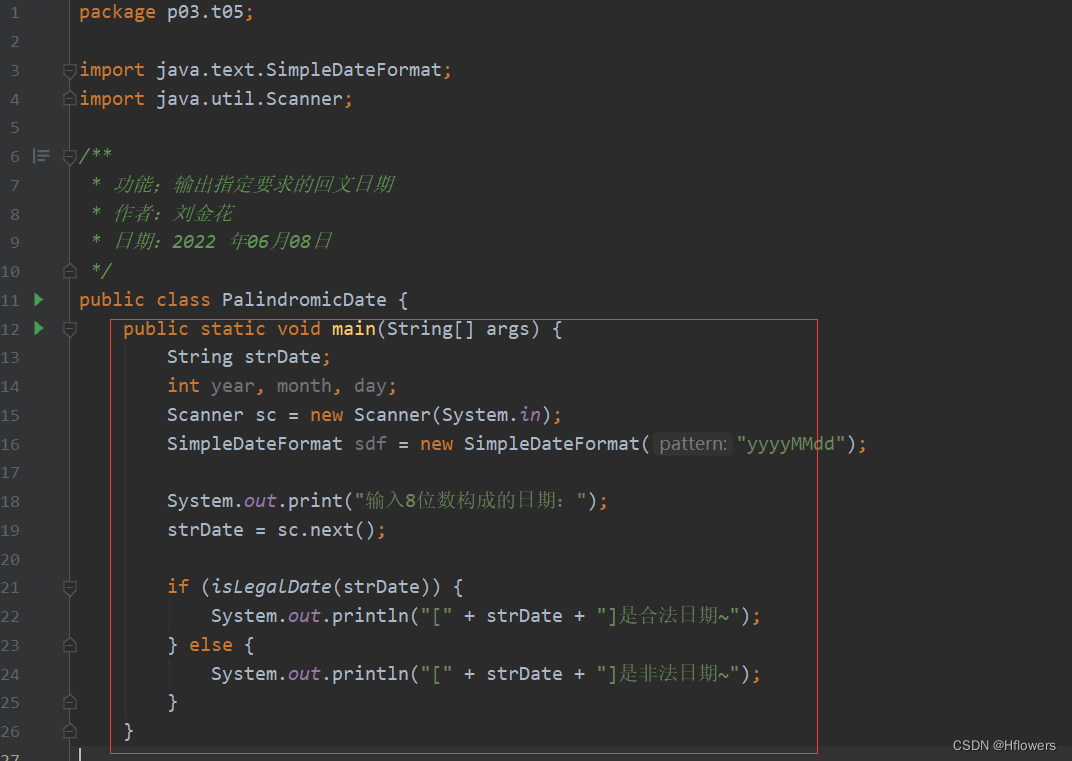
package p03.t05;
import java.text.SimpleDateFormat;
import java.util.Scanner;
/**
* 功能;输出指定要求的回文日期
* 作者:刘金花
* 日期:2022 年06月08日
*/
public class PalindromicDate {
public static void main(String[] args) {
String strDate;
int year, month, day;
Scanner sc = new Scanner(System.in);
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd");
System.out.print("输入8位数构成的日期:");
strDate = sc.next();
if (isLegalDate(strDate)) {
System.out.println("[" + strDate + "]是合法日期~");
} else {
System.out.println("[" + strDate + "]是非法日期~");
}
}
- 运行程序,查看结果:
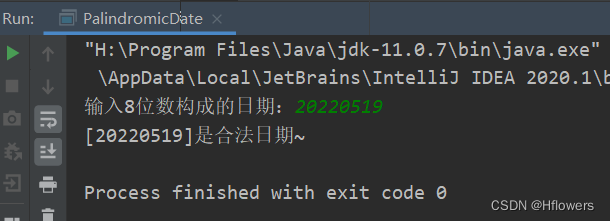
- 月份和天日都不对
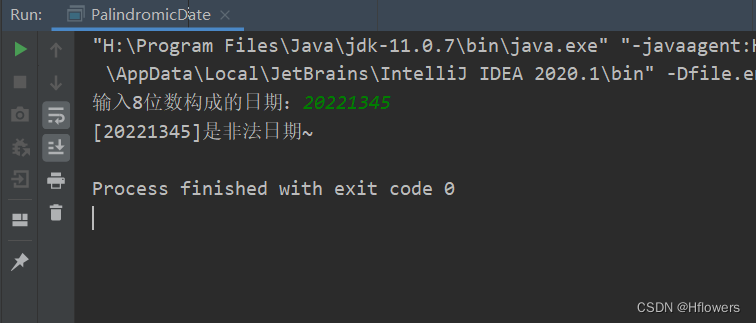
- 年份、月份和天日都对,但是长度不对
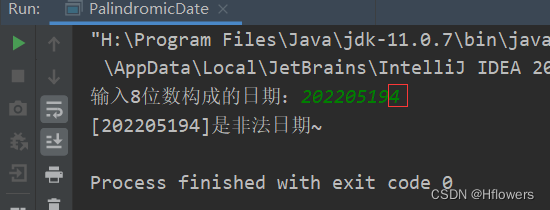
3、判断日期是否是回文日期
- 定义静态方法
isPalindromicDate()
方法
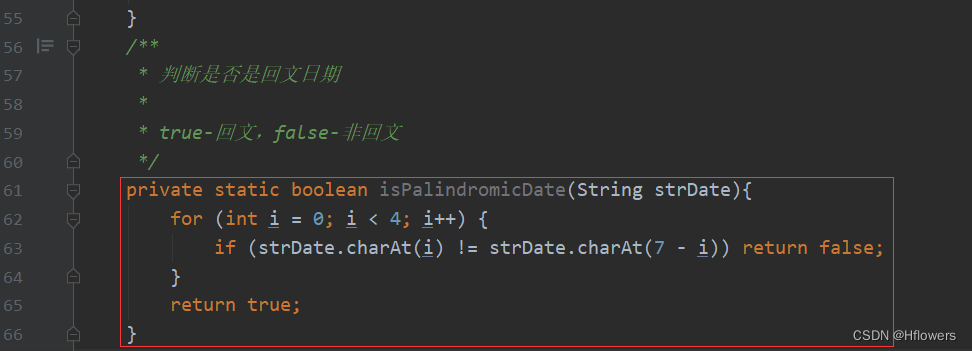
/**
* 判断是否是回文日期
*
* true-回文,false-非回文
*/
private static boolean isPalindromicDate(String strDate){
for (int i = 0; i < 4; i++) {
// 采用反向思维
if (strDate.charAt(i) != strDate.charAt(7 - i)) return false;
}
return true;
}
4、修改主方法,输出该日期之后的第一个回文日期
- 完成第一个任务:输出该日期之后的第一个回文日期
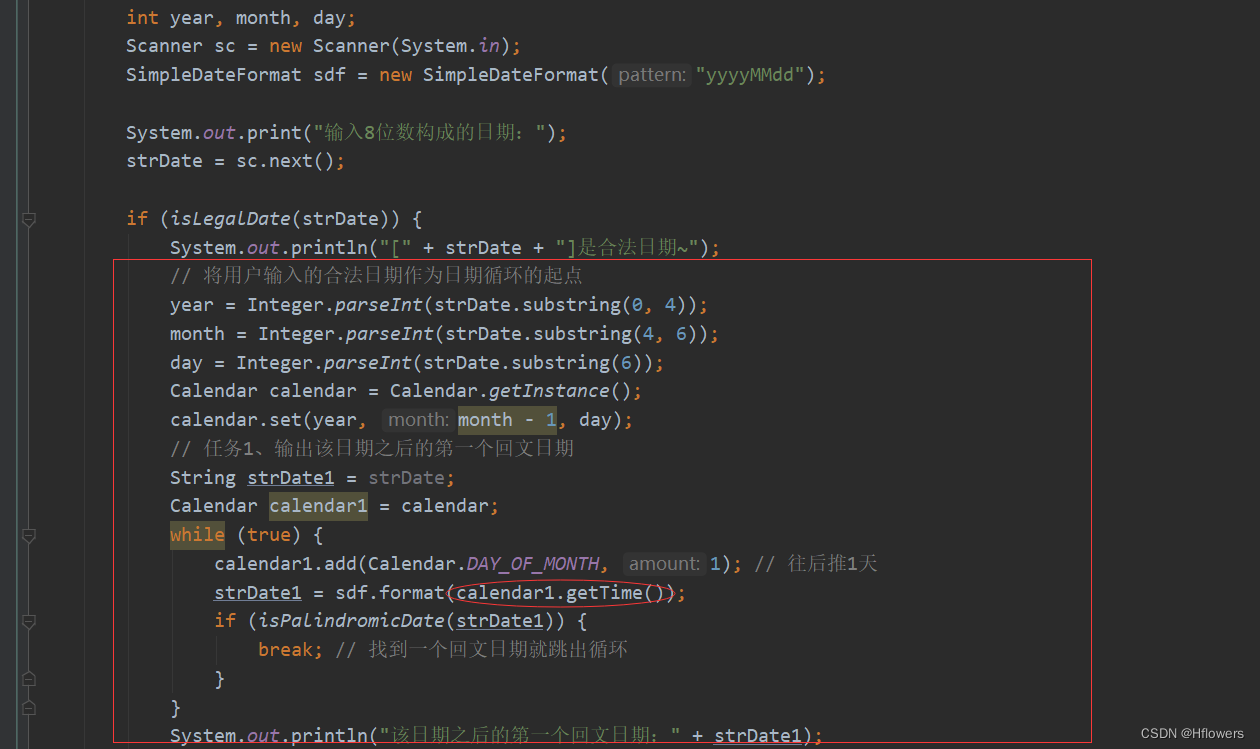
// 将用户输入的合法日期作为日期循环的起点
year = Integer.parseInt(strDate.substring(0, 4));
month = Integer.parseInt(strDate.substring(4, 6));
day = Integer.parseInt(strDate.substring(6));
Calendar calendar = Calendar.getInstance();
calendar.set(year, month - 1, day);
// 任务1、输出该日期之后的第一个回文日期
String strDate1 = strDate;
Calendar calendar1 = calendar;
while (true) {
calendar1.add(Calendar.DAY_OF_MONTH, 1); // 往后推1天
strDate1 = sdf.format(calendar1.getTime());
if (isPalindromicDate(strDate1)) {
break; // 找到一个回文日期就跳出循环
}
}
System.out.println("该日期之后的第一个回文日期:" + strDate1);
- 运行程序,查看结果:
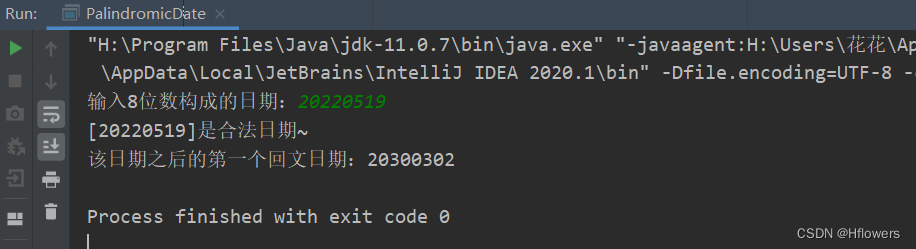
5、判断是否是ABABBABA型回文日期
- 定义静态方法
isABABBABAPalindromicDate()
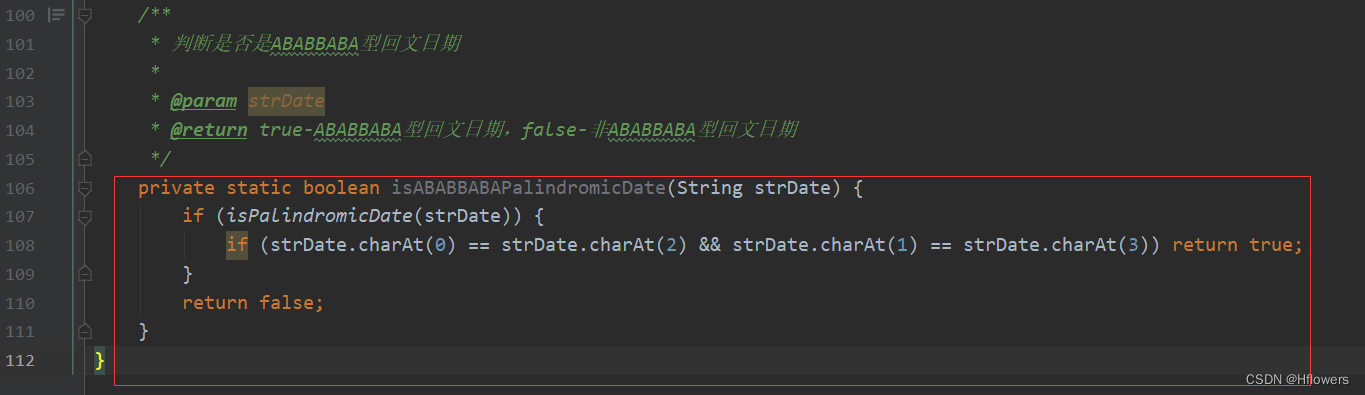
/**
* 判断是否是ABABBABA型回文日期
*
* @param strDate
* @return true-ABABBABA型回文日期,false-非ABABBABA型回文日期
*/
private static boolean isABABBABAPalindromicDate(String strDate) {
if (isPalindromicDate(strDate)) {
if (strDate.charAt(0) == strDate.charAt(2) && strDate.charAt(1) == strDate.charAt(3)) return true;
}
return false;
}
6、修改主方法,输出该日期之后的第一个ABABBABA型回文日期
- 完成第二个任务:输出该日期之后的第一个ABABBABA型回文日期
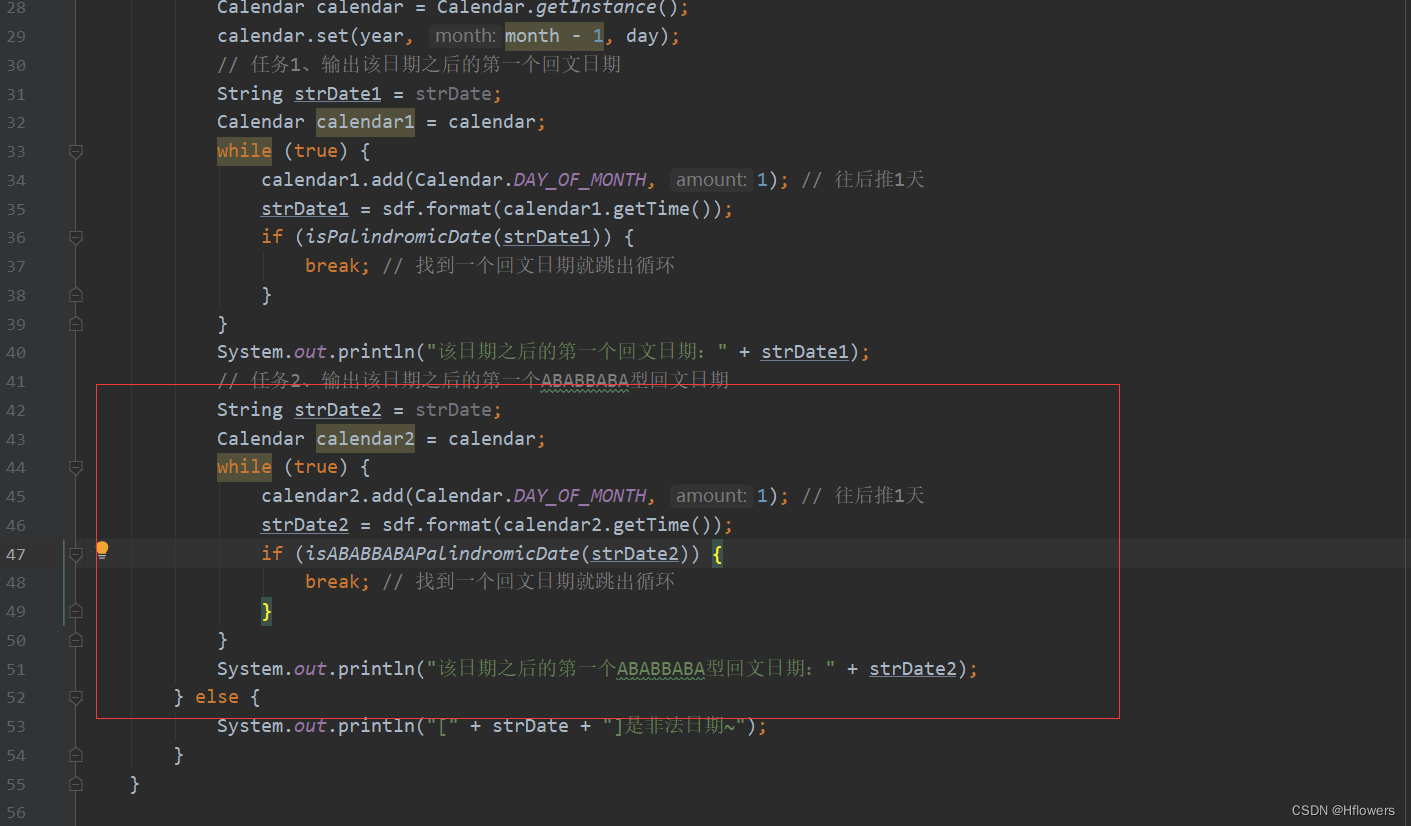
// 任务2、输出该日期之后的第一个ABABBABA型回文日期
String strDate2 = strDate;
Calendar calendar2 = calendar;
while (true) {
calendar2.add(Calendar.DAY_OF_MONTH, 1); // 往后推1天
strDate2 = sdf.format(calendar2.getTime());
if (isABABBABAPalindromicDate(strDate2)) {
break; // 找到一个回文日期就跳出循环
}
}
System.out.println("该日期之后的第一个ABABBABA型回文日期:" + strDate2);
7、查看完整源代码
package p03.t05;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Scanner;
/**
* 功能;输出指定要求的回文日期
* 作者:刘金花
* 日期:2022 年06月08日
*/
public class PalindromicDate {
public static void main(String[] args) {
String strDate;
int year, month, day;
Scanner sc = new Scanner(System.in);
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd");
System.out.print("输入8位数构成的日期:");
strDate = sc.next();
if (isLegalDate(strDate)) {
System.out.println("[" + strDate + "]是合法日期~");
// 将用户输入的合法日期作为日期循环的起点
year = Integer.parseInt(strDate.substring(0, 4));
month = Integer.parseInt(strDate.substring(4, 6));
day = Integer.parseInt(strDate.substring(6));
Calendar calendar = Calendar.getInstance();
calendar.set(year, month - 1, day);
// 任务1、输出该日期之后的第一个回文日期
String strDate1 = strDate;
Calendar calendar1 = calendar;
while (true) {
calendar1.add(Calendar.DAY_OF_MONTH, 1); // 往后推1天
strDate1 = sdf.format(calendar1.getTime());
if (isPalindromicDate(strDate1)) {
break; // 找到一个回文日期就跳出循环
}
}
System.out.println("该日期之后的第一个回文日期:" + strDate1);
// 任务2、输出该日期之后的第一个ABABBABA型回文日期
String strDate2 = strDate;
Calendar calendar2 = calendar;
while (true) {
calendar2.add(Calendar.DAY_OF_MONTH, 1); // 往后推1天
strDate2 = sdf.format(calendar2.getTime());
if (isABABBABAPalindromicDate(strDate2)) {
break; // 找到一个回文日期就跳出循环
}
}
System.out.println("该日期之后的第一个ABABBABA型回文日期:" + strDate2);
} else {
System.out.println("[" + strDate + "]是非法日期~");
}
}
/**
* 判断日期是否合法
*
* @param strDate
* @return true-合法,false-非法
*/
private static boolean isLegalDate(String strDate) {
int year, month, day;
year = Integer.parseInt(strDate.substring(0, 4));
month = Integer.parseInt(strDate.substring(4, 6));
day = Integer.parseInt(strDate.substring(6));
// 利用反向思维来处理
if (year < 1000 || year > 8999) return false;
if (month < 1 || month > 12) return false;
if (month == 1 || month == 3 || month == 5 || month == 7 || month == 8 || month == 10 || month == 12) {
if (day < 1 || day > 31) return false;
} else if (month == 2) {
if (year % 4 == 0 && year % 100 != 0 || year % 400 == 0) { // 闰年判断
if (day < 1 || day > 29) return false;
} else {
if (day < 1 || day > 28) return false;
}
} else {
if (day < 1 || day > 30) return false;
}
return true;
}
/**
* 判断是否是回文日期
*
* @param strDate
* @return true-回文日期,false-非回文日期
*/
private static boolean isPalindromicDate(String strDate) {
for (int i = 0; i < 4; i++) {
// 采用反向思维
if (strDate.charAt(i) != strDate.charAt(7 - i)) return false;
}
return true;
}
/**
* 判断是否是ABABBABA型回文日期
*
* @param strDate
* @return true-ABABBABA型回文日期,false-非ABABBABA型回文日期
*/
private static boolean isABABBABAPalindromicDate(String strDate) {
if (isPalindromicDate(strDate)) {
if (strDate.charAt(0) == strDate.charAt(2) && strDate.charAt(1) == strDate.charAt(3)) return true;
}
return false;
}
}
8、运行程序,查看结果
- 输入一个合法日期
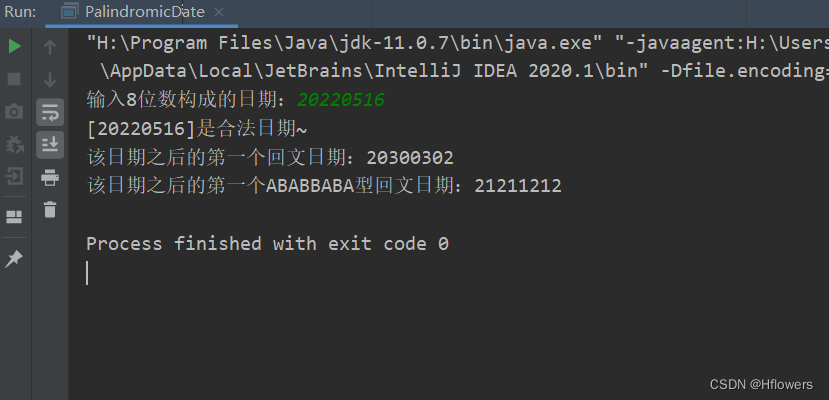
- 输入一个非法日期
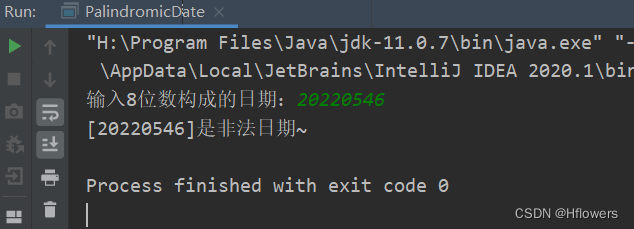
(二)知识点讲解
Data类
- Date 类来封装当前的日期和时间。 Date 类提供两个构造函数来实例化 Date 对象。
Calendar类:
- Calendar类的功能要比Date类强大很多,可以方便的进行日期的计算,获取日期中的信息时考虑了时区等问题。而且在实现方式上也比Date类要复杂一些
SimpleDateFormat类:
- SimpleDateFormat是位于java.text包下,继承自DateFormat类,专门用于格式化时间、字符串时间与日期对象相互转换的一个工具类。
案列:计算中华人民共和国成立了多少天
编程实现:
package p03.t05;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
/**
* 功能;计算中华人民共和国成立了多少天
* 作者:刘金花
* 日期:2022 年05月19日
*/
public class LifeOfPRC {
public static void main(String[] args) {
Date date1 = new Date();
System.out.println("当前日期:" + date1);
System.out.println("日期对应的毫秒数:" + date1.getTime());
// 创建简单日期格式对象
SimpleDateFormat sdf = new SimpleDateFormat("yyyy年MM月dd日 hh:mm:ss");
System.out.println("方法一:采用Date类");
Date foundDate = new Date(1949 - 1900,9,1,8,0,0); // 1949-10-01
System.out.println(sdf.format(foundDate));
Date currentDate = new Date();
System.out.println("当前日期:" + sdf.format(currentDate));
long interval = 0; // 时间间隔(毫秒数)
interval = currentDate.getTime() - foundDate.getTime();
System.out.println("中华人民共和国成立了" + interval + "毫秒");
System.out.println("中华人民共和国成立了" + (interval / 1000) + "秒");
System.out.println("中华人民共和国成立了" + (interval / 1000 / 60) + "分");
System.out.println("中华人民共和国成立了" + (interval / 1000 / 60 / 60) + "小时");
System.out.println("中华人民共和国成立了" + (interval / 1000 / 60 / 24) + "天");
System.out.println();
System.out.println("方法二:采用Calendar类");
Calendar calendar1 = Calendar.getInstance(); // 单例模式
calendar1.set(Calendar.YEAR,1949);
calendar1.set(Calendar.MONTH,9);
calendar1.set(Calendar.DAY_OF_MONTH,1);
calendar1.set(Calendar.HOUR,8);
calendar1.set(Calendar.MINUTE,0);
calendar1.set(Calendar.SECOND,0);
System.out.println("成立日期:" + sdf.format(calendar1.getTime()));
Calendar calendar2 = Calendar.getInstance();
System.out.println("当前日期:" + sdf.format(calendar2.getTime()));
interval = 0; // 时间间隔(毫秒数)
interval = calendar2.getTime().getTime() - calendar1.getTime().getTime();
System.out.println("中华人民共和国成立了" + interval + "毫秒");
System.out.println("中华人民共和国成立了" + (interval / 1000) + "秒");
System.out.println("中华人民共和国成立了" + (interval / 1000 / 60) + "分");
System.out.println("中华人民共和国成立了" + (interval / 1000 / 60 / 60) + "小时");
System.out.println("中华人民共和国成立了" + (interval / 1000 / 60 / 24) + "天");
}
}
- 运行程序,查看结果:
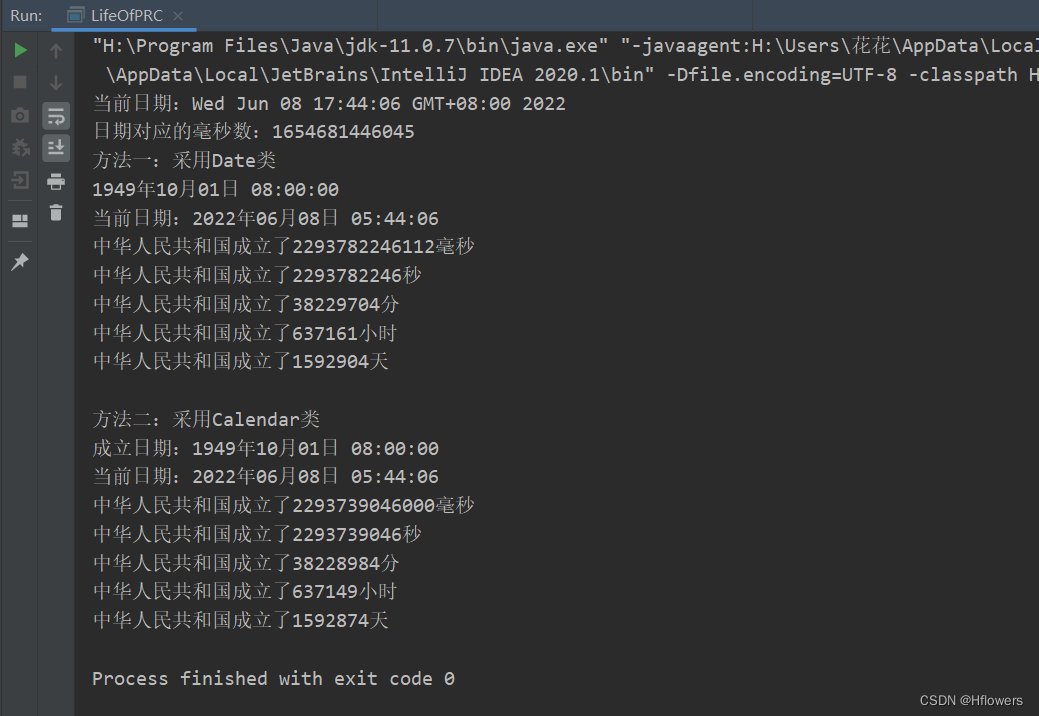
- 语句
calendar1.set(1949, 9, 1, 8, 0, 0);
相当于以下6
条语句
calendar1.set(Calendar.YEAR,1949);
calendar1.set(Calendar.MONTH,9);
calendar1.set(Calendar.DAY_OF_MONTH,1);
calendar1.set(Calendar.HOUR,8);
calendar1.set(Calendar.MINUTE,0);
calendar1.set(Calendar.SECOND,0);
(三)拓展练习
任务1、日期前推和日期后推
- 当前日期100天前是哪年哪月哪日星期几,当前日期100天后是哪年哪月哪日星期几
- 代码如下:
package p03.t05;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.Scanner;
/**
* 功能;日期后推
* 作者:刘金花
* 日期:2022 年06月08日
*/
public class renwu1 {
public static void main(String[] args) throws ParseException {
Scanner date = new Scanner(System.in);
System.out.println("请输入时间:YYYY-MM-DD");
String s1 = date.next();
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-mm-dd");
Date newdate = dateFormat.parse(s1);
Calendar calendar = Calendar.getInstance();
calendar.setTime(newdate);
calendar.add(Calendar.DAY_OF_MONTH,100);
int year = calendar.get(Calendar.YEAR);
int month = calendar.get(Calendar.MONTH);
int day = calendar.get(Calendar.DAY_OF_MONTH);
int week = calendar.get(Calendar.DAY_OF_WEEK);
System.out.println("一百天前:" + year + "年" + month + "月" + day + "日 星期" +week);
}
}
- 运行代码,查看结果:
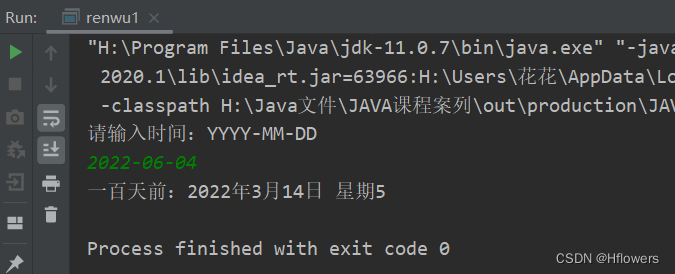
任务3、计算你活过的时间
- 计算你活了多少秒,多少分,多少小时,多少天,要求利用Calendar类来完成任务。