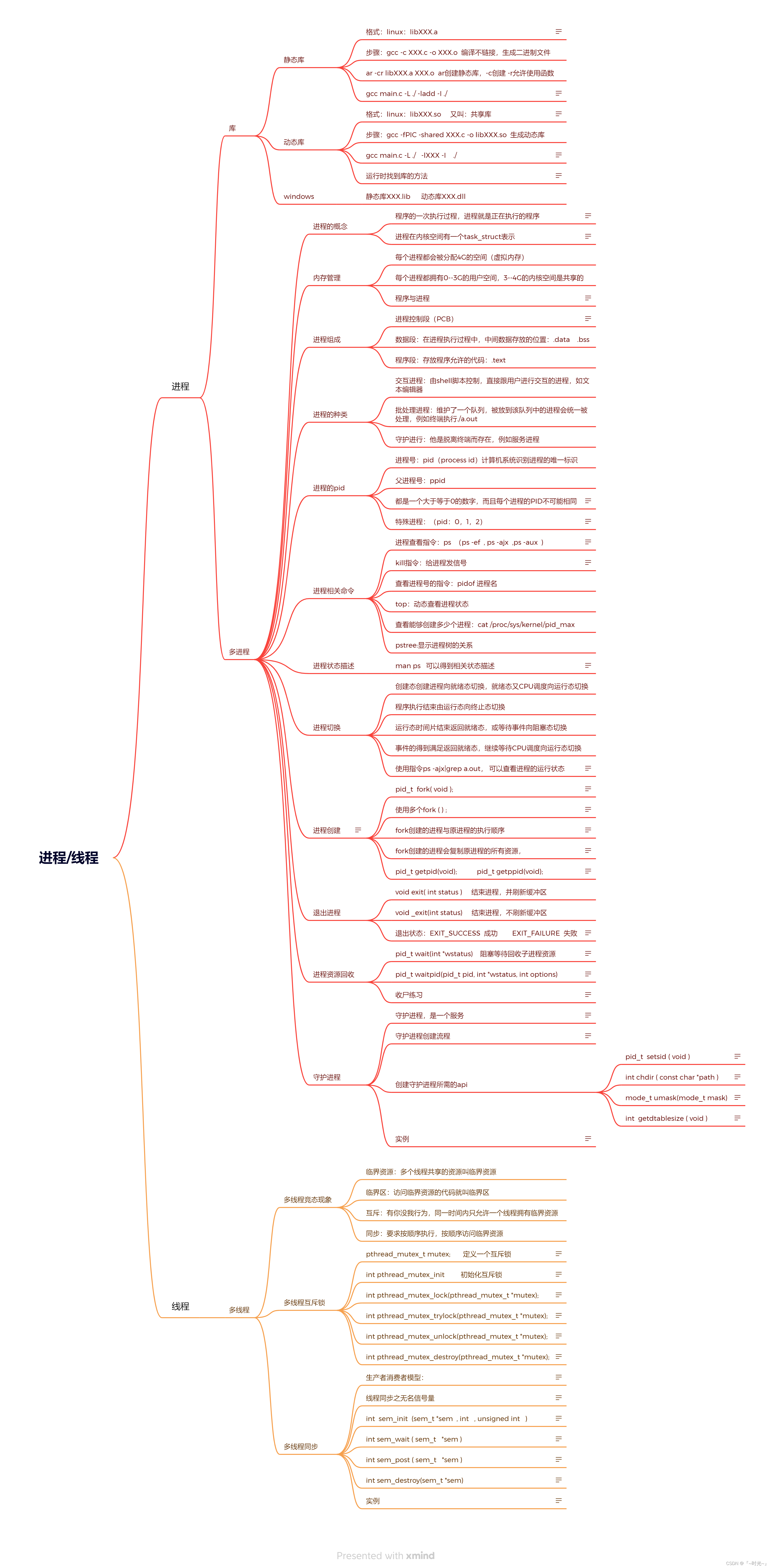
使用管道文件实现两个进程通信
main.c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <unistd.h>
#include <errno.h>
#include <fcntl.h>
#include <pthread.h>
#include <semaphore.h>
void *task1(void *arg)
{
int fd;
char Buf[256];
if((fd=open("./myfifo",O_WRONLY))==1)
{
perror("open error");
pthread_exit(NULL);
}
while(1)
{
memset(Buf,0,sizeof(Buf));
fgets(Buf,sizeof(Buf),stdin);
if(strlen(Buf)!=0)
{
Buf[strlen(Buf)-1]='\0';
write(fd,Buf,strlen(Buf));
}
if(strcmp(Buf,"quit")==0)
{
break;
}
}
close(fd);
pthread_cancel(*((pthread_t *)arg));
pthread_exit(NULL);
}
void *task2(void *arg)
{
int fd1;
char Buf[256];
if((fd1=open("./myfifo1",O_RDONLY))==1)
{
perror("open error");
pthread_exit(NULL);
}
while(1)
{
memset(Buf,0,sizeof(Buf));
ssize_t n=read(fd1,Buf,sizeof(Buf));
if(n!=0)
{
printf("对方:%s\n",Buf);
}
if(strcmp(Buf,"quit")==0)
{
break;
}
}
close(fd1);
pthread_cancel(*((pthread_t *)arg));
pthread_exit(NULL);
}
int main()
{
pthread_t tid1,tid2;
if(mkfifo("./myfifo",0664))
{
perror("mkfifo error");
return -1;
}
if(mkfifo("./myfifo1",0664))
{
perror("mkfifo1 error");
return -1;
}
if(pthread_create(&tid1,NULL,task1,&tid2))
{
perror("create error");
return -1;
}
if(pthread_create(&tid2,NULL,task2,&tid1))
{
perror("create error");
return -1;
}
pthread_join(tid1,NULL);
pthread_join(tid2,NULL);
system("rm myfifo");
system("rm myfifo1");
return 0;
}
main1.c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <unistd.h>
#include <errno.h>
#include <fcntl.h>
#include <pthread.h>
#include <semaphore.h>
void *task1(void *arg)
{
int fd;
char Buf1[256];
if((fd=open("./myfifo",O_RDONLY))==1)
{
perror("open error");
pthread_exit(NULL);
}
while(1)
{
memset(Buf1,0,sizeof(Buf1));
ssize_t n=read(fd,Buf1,sizeof(Buf1));
if(n!=0)
{
printf("对方:%s\n",Buf1);
}
if(strcmp(Buf1,"quit")==0)
{
break;
}
}
close(fd);
pthread_cancel(*((pthread_t *)arg));
pthread_exit(NULL);
}
void *task2(void *arg)
{
int fd1;
char Buf[256];
if((fd1=open("./myfifo1",O_WRONLY))==1)
{
perror("open error");
pthread_exit(NULL);
}
while(1)
{
memset(Buf,0,sizeof(Buf));
fgets(Buf,sizeof(Buf),stdin);
if(strlen(Buf)!=0)
{
Buf[strlen(Buf)-1]='\0';
write(fd1,Buf,strlen(Buf));
}
if(strcmp(Buf,"quit")==0)
{
break;
}
}
close(fd1);
pthread_cancel(*((pthread_t *)arg));
pthread_exit(NULL);
}
int main()
{
pthread_t tid1,tid2;
if(pthread_create(&tid1,NULL,task1,&tid2))
{
perror("create error");
return -1;
}
if(pthread_create(&tid2,NULL,task2,&tid1))
{
perror("create error");
return -1;
}
pthread_join(tid1,NULL);
pthread_join(tid2,NULL);
return 0;
}
