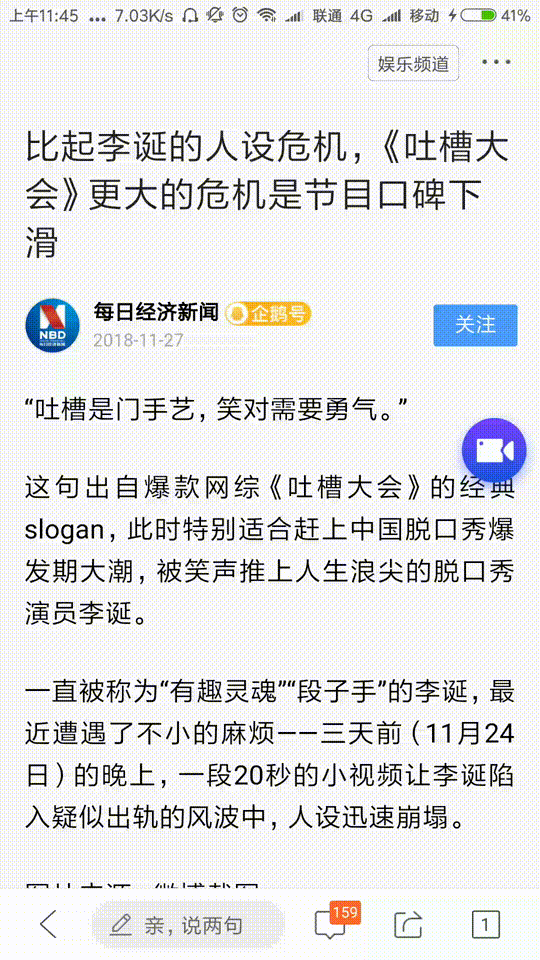
大概就这种效果
额,突然发现UC被我卸载了,这个是QQ浏览器的效果,不过都一样,如果当前页面不是全屏的话,把根布局设为相对布局,然后设置评论布局为处于底部,这样在点击评论时弹开键盘会触发布局重绘,底部的评论也会处于软键盘的上方,键盘消息,布局下移,但是我们要说的是全屏的情况下我们如何处理。
首先要监听到软件盘弹起,然后再设置评论的popupWindow
import android.app.Activity;
import android.graphics.Rect;
import android.support.v4.app.Fragment;
import android.view.View;
import android.view.ViewTreeObserver;
public class KeyboardStatusDetector {
private static final int SOFT_KEY_BOARD_MIN_HEIGHT = 100;
private KeyboardVisibilityListener mVisibilityListener;
boolean keyboardVisible = false;
public KeyboardStatusDetector registerFragment(Fragment f) {
return registerView(f.getView());
}
public KeyboardStatusDetector registerActivity(Activity a) {
return registerView(a.getWindow().getDecorView().findViewById(android.R.id.content));
}
public KeyboardStatusDetector registerView(final View v) {
v.getViewTreeObserver().addOnGlobalLayoutListener(new ViewTreeObserver.OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
Rect r = new Rect();
v.getWindowVisibleDisplayFrame(r);
//int heightDiff = v.getRootView().getHeight() - (r.bottom - r.top);
int heightDiff = v.getRootView().getHeight() - r.bottom;
LogUtils.e("键盘A--->", v.getRootView().getHeight() +" "+ r.bottom +" "+ r.top);
if (heightDiff > SOFT_KEY_BOARD_MIN_HEIGHT) { // if more than 100 pixels, its probably a keyboard...
if (!keyboardVisible) {
keyboardVisible = true;
if (mVisibilityListener != null) {
mVisibilityListener.onVisibilityChanged(true, heightDiff);
}
}
} else {
if (keyboardVisible) {
keyboardVisible = false;
if (mVisibilityListener != null) {
mVisibilityListener.onVisibilityChanged(false, heightDiff);
}
}
}
}
});
return this;
}
public KeyboardStatusDetector setVisibilityListener(KeyboardVisibilityListener listener) {
mVisibilityListener = listener;
return this;
}
public interface KeyboardVisibilityListener {
void onVisibilityChanged(boolean keyboardVisible, int heightDiff);
}
}
然后再对popupWindow进行设置位置
private void showSendMsgPop() {
if (null == sendMsgPopup) {
sendMsgPopup = new SendMsgPopupWindow(NewsDetailsActivity.this);
}
//设置处于底部
sendMsgPopup.showAtLocation(getWindow().getDecorView(), Gravity.BOTTOM, 0, 0);
}
当然,最后最关键的是popupWindow里的配置,要不然popupWindow会被软键盘遮挡。
@Override
public void dismiss() {
super.dismiss();
// 评论框消息的同时把软键盘关闭
KeyBoardUtils.closeKeybord(editText, mContext);
}
public void showAtLocation(View parent, int gravity, int x, int y) {
// 这三行配置是关键
this.setSoftInputMode(WindowManager.LayoutParams.SOFT_INPUT_ADJUST_RESIZE);
this.setSoftInputMode(WindowManager.LayoutParams.SOFT_INPUT_STATE_VISIBLE | WindowManager.LayoutParams.SOFT_INPUT_ADJUST_RESIZE);
this.setInputMethodMode(PopupWindow.INPUT_METHOD_NEEDED); // 在显示输入法之后调用,否则popupwindow会在窗口底层
super.showAtLocation(parent, gravity, x, y);
}