效果
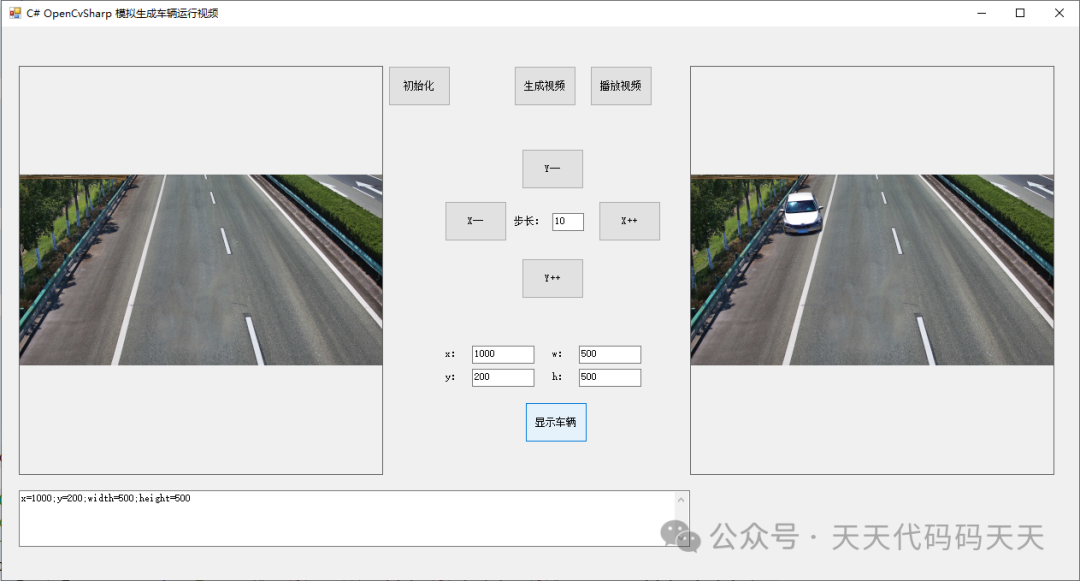
项目
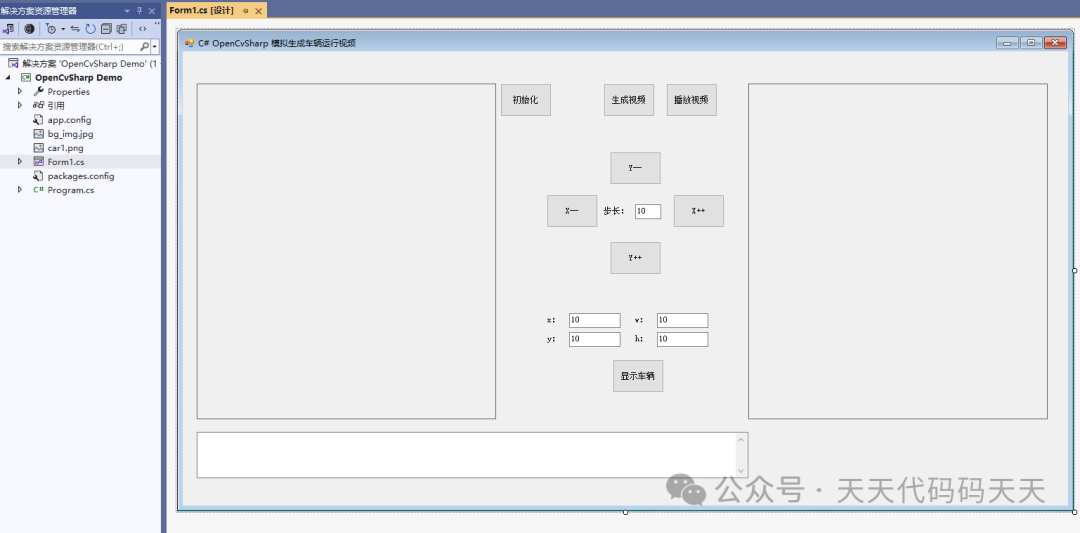
代码
using OpenCvSharp;
using OpenCvSharp.Extensions;
using System;
using System.Diagnostics;
using System.Drawing;
using System.Windows.Forms;
namespace OpenCvSharp_Demo
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
string image_path;
Stopwatch stopwatch = new Stopwatch();
private void Form1_Load(object sender, EventArgs e)
{
image_path = "bg_img.jpg";
pictureBox1.Image = new Bitmap(image_path);
}
Mat bgImg;
Mat car_bgra;
Mat car;
Mat mask;
Rect roi;
Mat pos;
int x, y, width, height, step;
private void button6_Click(object sender, EventArgs e)
{
x = Convert.ToInt32(txtX.Text);
y = Convert.ToInt32(txtY.Text);
width = Convert.ToInt32(txtW.Text);
height = Convert.ToInt32(txtH.Text);
Cv2.Resize(mask, mask, new OpenCvSharp.Size(width, height));
Cv2.Resize(car, car, new OpenCvSharp.Size(width, height));
textBox1.Text = string.Format("x={0};y={1}", x, y);
ShowCar();
}
private void button5_Click(object sender, EventArgs e)
{
step = Convert.ToInt32(txtStep.Text);
y = y + step;
ShowCar();
}
private void button7_Click(object sender, EventArgs e)
{
step = Convert.ToInt32(txtStep.Text);
x = x - step;
ShowCar();
}
private void button1_Click(object sender, EventArgs e)
{
step = Convert.ToInt32(txtStep.Text);
y = y - step;
ShowCar();
}
VideoCapture capture;
Mat currentFrame = new Mat();
private void button8_Click(object sender, EventArgs e)
{
capture = new VideoCapture("out.mp4");
if (!capture.IsOpened())
{
MessageBox.Show("打开视频文件失败");
return;
}
capture.Read(currentFrame);
if (!currentFrame.Empty())
{
pictureBox1.Image = BitmapConverter.ToBitmap(currentFrame);
timer1.Interval = (int)(1000.0 / capture.Fps);
timer1.Enabled = true;
}
}
private void timer1_Tick(object sender, EventArgs e)
{
capture.Read(currentFrame);
if (currentFrame.Empty())
{
//pictureBox1.Image = null;
timer1.Enabled = false;
capture.Release();
textBox1.Text = "播放完毕。";
return;
}
pictureBox1.Image = BitmapConverter.ToBitmap(currentFrame);
}
bool ShowCar()
{
textBox1.Text = string.Format("x={0};y={1};width={2};height={3}", x, y, width, height);
txtX.Text = x.ToString();
txtY.Text = y.ToString();
txtW.Text = width.ToString();
txtH.Text = height.ToString();
roi = new Rect(x, y, width, height);
if (x < 0)
{
x = 0;
textBox1.Text = "位置越界";
return false;
}
if (y < 0)
{
y = 0;
textBox1.Text = "位置越界";
return false;
}
bgImg = Cv2.ImRead(image_path, ImreadModes.Color);
if (roi.Bottom > bgImg.Height)
{
textBox1.Text = "位置越界";
bgImg.Dispose();
return false;
}
if (roi.Right > bgImg.Width)
{
textBox1.Text = "位置越界";
bgImg.Dispose();
return false;
}
pos = new Mat(bgImg, roi);
car.CopyTo(pos, mask);
pictureBox2.Image = new Bitmap(bgImg.ToMemoryStream());
pos.Dispose();
bgImg.Dispose();
return true;
}
private void button3_Click(object sender, EventArgs e)
{
step = Convert.ToInt32(txtStep.Text);
x = x + step;
ShowCar();
}
private void button2_Click(object sender, EventArgs e)
{
bgImg = Cv2.ImRead(image_path, ImreadModes.Color);
car_bgra = Cv2.ImRead("car1.png", ImreadModes.Unchanged);
car = Cv2.ImRead("car1.png", ImreadModes.Color);
//透明图
//B,G,R,A B,G,R -> 0 黑色 255 白色
//A为透明度 -> 255为不透明,0为全透。
var alphaBgr = Cv2.Split(car_bgra);
mask = alphaBgr[3];
x = 0;
y = 0;
width = car.Cols;
height = car.Rows;
ShowCar();
}
private void button4_Click(object sender, EventArgs e)
{
x = 1120;
y = 0;
width = 400;
height = 400;
Cv2.Resize(mask, mask, new OpenCvSharp.Size(width, height));
Cv2.Resize(car, car, new OpenCvSharp.Size(width, height));
Cv2.Resize(car_bgra, car_bgra, new OpenCvSharp.Size(width, height));
bgImg = Cv2.ImRead(image_path, ImreadModes.Color);
VideoWriter vwriter = new VideoWriter("out.mp4", FourCC.X264, 30, new OpenCvSharp.Size(bgImg.Width, bgImg.Height));
int count = 0;
for (int i = 0; i < 200; i++)
{
y = y + 10;
width = width + 2;
height = height + 2;
car_bgra = Cv2.ImRead("car1.png", ImreadModes.Unchanged);
car = Cv2.ImRead("car1.png", ImreadModes.Color);
Cv2.Resize(car_bgra, car_bgra, new OpenCvSharp.Size(width, height));
Cv2.Resize(car, car, new OpenCvSharp.Size(width, height));
var alphaBgr = Cv2.Split(car_bgra);
mask = alphaBgr[3];
roi = new Rect(x, y, width, height);
if (roi.Bottom > bgImg.Height)
{
textBox1.Text = "位置越界";
break;
}
if (roi.Right > bgImg.Width)
{
textBox1.Text = "位置越界";
break;
}
bgImg = Cv2.ImRead(image_path, ImreadModes.Color);
pos = new Mat(bgImg, roi);
car.CopyTo(pos, mask);
Cv2.ImWrite("out/" + i + ".jpg", bgImg);
vwriter.Write(bgImg);
count++;
//pos.Dispose();
//bgImg.Dispose();
}
pictureBox2.Image = new Bitmap(bgImg.ToMemoryStream());
//Cv2.ImWrite("result.jpg", bgImg);
//double costTime = stopwatch.Elapsed.TotalMilliseconds;
//textBox1.Text = $"耗时:{costTime:F2}ms";
textBox1.Text = "生成完毕!count=" + count.ToString();
textBox1.Text += string.Format("x={0};y={1};width={2};height={3}", x, y, width, height);
vwriter.Release();
}
}
}
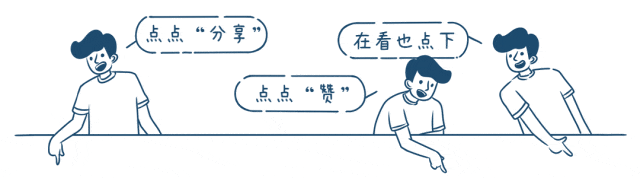