问题及代码:
头文件SqString.h和源文件SqString.cpp详见第八周项目1--建立顺序串的算法库
注:在头文件中加上相应的函数声明。
并且在源文件中加上相应的函数实现。
(1)函数实现:
- void Trans(SqString &s, char c1, char c2)
- {
- int i;
- for (i=0; i<s.length; i++)
- if (s.data[i]==c1)
- s.data[i]=c2;
- }
(1)主函数main.cpp函数:
- #include <stdio.h>
- #include "sqString.h"
- int main()
- {
- SqString s;
- StrAssign(s, "messages");
- Trans(s, 'e', 'a');
- DispStr(s);
- return 0;
- }
(1)运行结果:
原来:
运行结果:
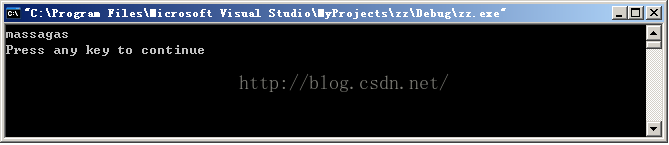
(2)函数实现:
- void Invert(SqString &s)
- {
- int i;
- char temp;
- for (i=0; i<s.length/2; i++)
- {
- temp = s.data[i];
- s.data[i]=s.data[s.length-i-1];
- s.data[s.length-i-1] = temp;
- }
- }
(2)主函数main.cpp函数:
- #include <stdio.h>
- #include "sqString.h"
- int main()
- {
- SqString s;
- StrAssign(s, "abcdefg");
- Invert(s);
- DispStr(s);
- return 0;
- }
(2)运行结果:
原来:
运行后:

(3)函数实现:
- void DellChar(SqString &s, char c)
- {
- int k=0, i=0;
- while(i<s.length)
- {
- if(s.data[i]==c)
- k++;
- else
- s.data[i-k]=s.data[i];
- i++;
- }
- s.length -= k;
- }
(3)主函数main.cpp函数:
- #include <stdio.h>
- #include "sqString.h"
- int main()
- {
- SqString s;
- StrAssign(s, "message");
- DellChar(s, 'e');
- DispStr(s);
- return 0;
- }
(3)运行结果:
原来:

运行后:

(4)的main函数:
- #include <stdio.h>
- #include "sqString.h"
- int main()
- {
- SqString s1, s2, s;
- StrAssign(s1, "message");
- StrAssign(s2, "agent");
- s = CommChar(s1, s2);
- DispStr(s);
- return 0;
- }
(4)的运行结果:
知识点总结:
串的操作。
学习心得:
通过顺序串的算法,让我对其有了更深的理解。