手写unique_ptr指针指针
代码:
#include <iostream>
#include <stdexcept>
template <typename T>
class unique_ptr {
public:
// 构造函数
explicit unique_ptr(T* ptr = nullptr) : m_ptr(ptr) {}
// 析构函数
~unique_ptr() {
delete m_ptr;
}
// 禁止复制构造函数
unique_ptr(const unique_ptr&) = delete;
// 禁止复制赋值运算符
unique_ptr& operator=(const unique_ptr&) = delete;
// 移动构造函数
unique_ptr(unique_ptr&& other) noexcept : m_ptr(other.m_ptr) {
other.m_ptr = nullptr;
}
// 移动赋值运算符
unique_ptr& operator=(unique_ptr&& other) noexcept {
if (this != &other) {
delete m_ptr;
m_ptr = other.m_ptr;
other.m_ptr = nullptr;
}
return *this;
}
// 重载*和->运算符
T& operator*() const {
return *m_ptr;
}
T* operator->() const {
return m_ptr;
}
// 获取原始指针
T* get() const {
return m_ptr;
}
// 释放所有权
T* release() {
T* temp = m_ptr;
m_ptr = nullptr;
return temp;
}
// 重置智能指针
void reset(T* ptr = nullptr) {
T* old_ptr = m_ptr;
m_ptr = ptr;
delete old_ptr;
}
private:
T* m_ptr;
};
int main() {
unique_ptr<int> ptr1(new int(10));
std::cout << *ptr1 << std::endl;
unique_ptr<int> ptr2 = std::move(ptr1);
std::cout << *ptr2 << std::endl; // 输出 10
std::cout << *ptr1 << std::endl; // 不会输出什么东西
return 0;
}
手写登录界面,不允许拖拽,要求尽可能的美观
代码:
#ifndef WIDGET_H
#define WIDGET_H
#include <QWidget>
#include <QLineEdit>
#include <QPushButton>
class Widget : public QWidget
{
Q_OBJECT
public:
Widget(QWidget *parent = nullptr);
~Widget();
private slots:
void on_loginButton_clicked();
private:
QLineEdit *usernameEdit;
QLineEdit *passwordEdit;
QPushButton *loginButton;
};
#endif // WIDGET_H
#include<QDebug>
#include "widget.h"
#include "ui_widget.h"
#include <QLineEdit>
#include <QPushButton>
#include <QVBoxLayout>
#include <QLabel>
#include <QIcon>
Widget::Widget(QWidget *parent)
: QWidget(parent)
{
setFixedSize(900, 600); // 设置窗口大小
setWindowTitle("登录界面");
setWindowIcon(QIcon("D:\\Qt\\msg\\homework\\png\\1.png")); // 设置窗口图标
QWidget *window = new QWidget;
window->setAutoFillBackground(true); // 确保背景图像填充整个窗口
window->setStyleSheet("QWidget { background-image: url(D:/Qt/msg/homework/png/2.png); }");
QVBoxLayout *layout = new QVBoxLayout(this); // 创建布局
usernameEdit = new QLineEdit;
usernameEdit->setPlaceholderText("用户名");
layout->addWidget(usernameEdit);
passwordEdit = new QLineEdit;
passwordEdit->setPlaceholderText("密码");
passwordEdit->setEchoMode(QLineEdit::Password);
layout->addWidget(passwordEdit);
loginButton = new QPushButton("登录");
layout->addWidget(loginButton);
connect(loginButton, &QPushButton::clicked, this, &Widget::on_loginButton_clicked);
// 应用样式表
this->setStyleSheet("* { "
" font-size: 30px; " // 为所有控件设置字体大小为14px
"} "
"QLineEdit { border: 3px solid #ccc; padding: 15px; }"
"QPushButton { background-color: #4CAF50; color: white; border: none; padding: 10px; border-radius: 5px; }"
"QPushButton:hover { background-color: #45a049; }");
}
Widget::~Widget()
{
}
void Widget::on_loginButton_clicked()
{
// 获取行编辑器中的内容
QString account = usernameEdit->text();
QString password = passwordEdit->text();
// 判断账号和密码是否正确
// 这里需要根据实际情况来验证账号和密码,以下只是一个示例
if (account == "123456" && password == "123456") {
// 登录成功
qDebug() << "登录成功";
this->close();
} else {
// 登录失败
qDebug() << "登录失败";
passwordEdit->clear();
}
}
#include "widget.h"
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
Widget w;
w.show();
return a.exec();
}
结果:
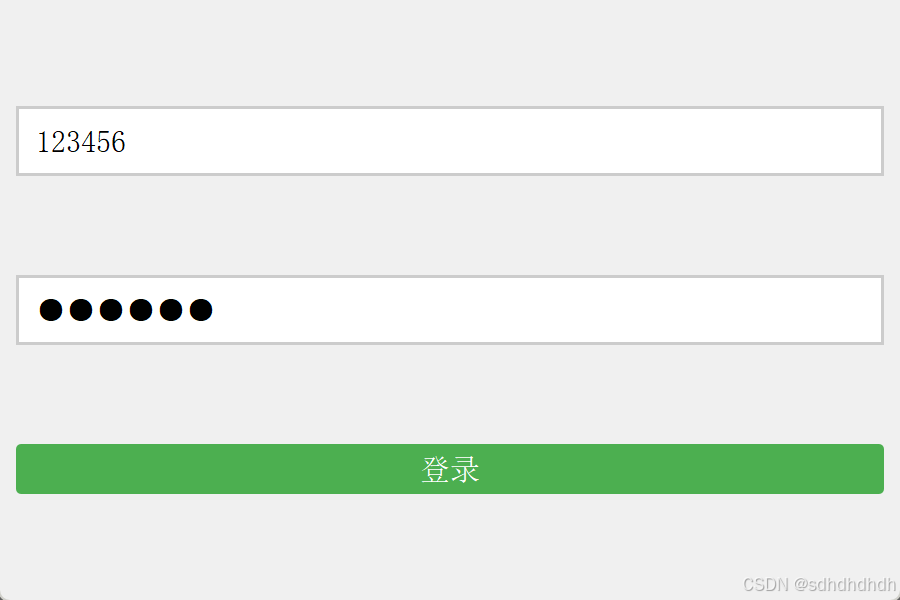
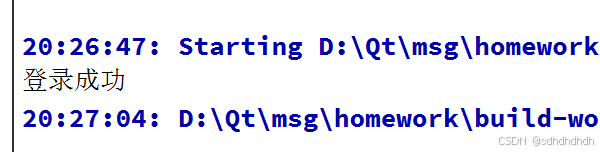