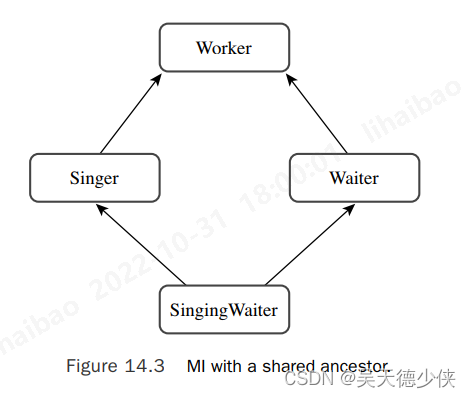
workermi.h
#pragma once
#include<string>
class Worker
{
private:
std::string fullname;
long id;
protected:
virtual void Data() const;
virtual void Get();
public:
Worker() :fullname("no one"), id(0L) {}
Worker(const std::string& s,long n):fullname(s),id(n){}
virtual ~Worker() = 0;
virtual void Set() = 0;
virtual void Show() const = 0;
};
class Waiter :virtual public Worker
{
private:
int panache;
protected:
void Data() const;
void Get();
public:
Waiter():Worker(),panache(0){}
Waiter(const std::string& s,long n,int p=0):Worker(s,n),panache(p){}
Waiter(const Worker& wk,int p=0):Worker(wk),panache(p){}
void Set();
void Show() const;
};
class Singer :virtual public Worker
{
protected:
enum { other, alto, contralto, soprano, bass, baritone, tenor };
enum { Vtypes = 7 };
void Data() const;
void Get();
private:
static char* pv[Vtypes];
int voice;
public:
Singer():Worker(),voice(other){}
Singer(const std::string& s,long n,int v=other):Worker(s,n),voice(v){}
Singer(const Worker& wk,int v=other):Worker(wk),voice(v){}
void Set();
void Show() const;
};
class SingingWaiter :public Singer, public Waiter
{
protected:
void Data() const;
void Get();
public:
SingingWaiter(){}
SingingWaiter(const std::string& s,long n,int p=0,int v=other):
Worker(s,n),Waiter(s,n,p),Singer(s,n,v){}
SingingWaiter(const Worker& wk,int p=0,int v=other):Worker(wk),Waiter(wk,v){}
SingingWaiter(const Waiter& wt,int v=other):Worker(wt),Waiter(wt),Singer(wt,v){}
SingingWaiter(const Singer& sg,int p=0):Worker(sg),Waiter(sg,p),Singer(sg){}
void Set();
void Show() const;
};
workermi.cpp
#include "workermi.h"
#include<iostream>
using std::cout;
using std::cin;
using std::endl;
Worker::~Worker(){}
void Worker::Data() const
{
cout << "Name: " << fullname << endl;
cout << "Employee ID: " << id << endl;
}
void Worker::Get()
{
getline(cin, fullname);
cout << "enter worker's ID: ";
cin >> id;
while (cin.get() != '\n') continue;
}
void Waiter::Set()
{
cout << "enter waiter's name: ";
Worker::Get();
Get();
}
void Waiter::Show() const
{
cout << "Category: waiter\n";
Worker::Data();
Data();
}
void Waiter::Data() const
{
cout << "panache rating: " << panache << endl;
}
void Waiter::Get()
{
cout << "enter waiter's panache rating: ";
cin >> panache;
while (cin.get() != '\n') continue;
}
char* Singer::pv[Singer::Vtypes] =
{ "other","alto","contralto","soprano","bass","baritone","tenor" };
void Singer::Set()
{
cout << "enter singer's name: ";
Worker::Get();
Get();
}
void Singer::Show() const
{
cout << "Category: singer\n";
Worker::Data();
Data();
}
void Singer::Data() const
{
cout << "Vocal range: " << pv[voice] << endl;
}
void Singer::Get()
{
cout << "enter number for singer's vocal range:\n";
int i;
for (i = 0; i < Vtypes; i++) {
cout << i << ": " << pv[i] << " ";
if (i % 4 == 3) cout << endl;
}
if (i % 4 != 0) cout << "\n";
cin >> voice;
while (cin.get() != '\n') continue;
}
void SingingWaiter::Data() const
{
Singer::Data();
Waiter::Data();
}
void SingingWaiter::Get()
{
Waiter::Get();
Singer::Get();
}
void SingingWaiter::Set()
{
cout << "enter singing waiter's name: ";
Worker::Get();
Get();
}
void SingingWaiter::Show() const
{
cout << "Category: Singing waiter\n";
Worker::Data();
Data();
}
main.cpp
#include<iostream>
#include<cstring>
#include"workermi.h"
const int SIZE = 5;
int main()
{
using std::cin;
using std::cout;
using std::endl;
using std::strchr;
Worker* lolas[SIZE];
int ct;
for (ct = 0; ct < SIZE; ct++) {
char choice;
cout << "enter the employee category:\n"
<< "w: waiter s: singer "
<< "t: singing waiter q: quit\n";
cin >> choice;
while (strchr("wstq", choice) == NULL) {
cout << "please enter a w,s,t,or q: ";
cin >> choice;
}
if (choice == 'q') break;
switch (choice)
{
case 'w':
lolas[ct] = new Waiter;
break;
case 's':
lolas[ct] = new Singer;
break;
case 't':
lolas[ct] = new SingingWaiter;
}
cin.get();
lolas[ct]->Set();
}
cout << "\nHere is your staff:\n";
int i;
for (i = 0; i < ct; i++) {
cout << endl;
lolas[i]->Show();
}
for (i = 0; i < ct; i++) {
delete lolas[i];
}
cout << "Bye.\n";
return 0;
}
结果
enter the employee category:
w: waiter s: singer t: singing waiter q: quit
w
enter waiter's name: wally slipshod
enter worker's ID: 1040
enter waiter's panache rating: 4
enter the employee category:
w: waiter s: singer t: singing waiter q: quit
s
enter singer's name: sinclair parma
enter worker's ID: 1044
enter number for singer's vocal range:
0: other 1: alto 2: contralto 3: soprano
4: bass 5: baritone 6: tenor
5
enter the employee category:
w: waiter s: singer t: singing waiter q: quit
t
enter singing waiter's name: natasha gargalova
enter worker's ID: 1021
enter waiter's panache rating: 6
enter number for singer's vocal range:
0: other 1: alto 2: contralto 3: soprano
4: bass 5: baritone 6: tenor
3
enter the employee category:
w: waiter s: singer t: singing waiter q: quit
q
Here is your staff:
Category: waiter
Name: wally slipshod
Employee ID: 1040
panache rating: 4
Category: singer
Name: sinclair parma
Employee ID: 1044
Vocal range: baritone
Category: Singing waiter
Name: natasha gargalova
Employee ID: 1021
Vocal range: soprano
panache rating: 6
Bye.