This module defines functions directly available in high-level programs, in particularly providing flow control and output.、
这个模块定义了好多好多函数方法,像一个宝库。
目录
(2)print_str_if(), print_ln_if()
1. while循环
Do-while loop. The loop is stopped if the return value is zero. It must be public. The following executes exactly once:
@do_while
def _():
...
return regint(0)
from Compiler import types
i = sint()
i = sint.get_random_int(3)
print_ln("%s", i.reveal())
@do_while
def _():
print_ln("hello!!")
s = sint()
s = sint.get_random_int(3)
match = s == i
return match.reveal()
这个程序定义了一个变量i,随机赋值。while循环里定义一个随机变量s,打印hello!!,如果s和i相等就退出循环。s和i都是0到7之间的随机数。
注意,return返回的值必须是一个公开值,不可以是秘密值。return返回值为0时,结束循环。下面是运行结果,因为取得随机数,所以下图运行结果不唯一。
2. for循环
(1)for_range()
for_range
(start, stop=None, step=None)
Decorator to execute loop bodies consecutively. Arguments work as in Python range()
, but they can by any public integer. Information has to be passed out via container types such as Array or declaring registers as global
. Note that changing Python data structures such as lists within the loop is not possible, but the compiler cannot warn about this.
Parameters: | start/stop/step – regint/cint/int |
---|
n = 5
a = sint.Array(n)
x = sint(0)
@for_range(n)
def _(i):
a[i] = i
global x
x += 1
print_ln("%s",a.reveal())
这个程序比较简单,为数组a赋值i。
(2) for_range_opt
Execute loop bodies in parallel up to an optimization budget. This prevents excessive loop unrolling. The budget is respected even with nested loops. Note that optimization is rather rudimentary for runtime n_loops
(regint/cint). Consider using for_range_parallel() in this case.
Parameters: |
|
---|
与for_range类似,但是这个循环是经过优化的。在某种情况下应该比for_range要好。用法也与for_range类似。
n = 5
a = sint.Array(n)
x = sint(0)
@for_range_opt(n)
def _(i):
a[i] = i
global x
x += 1
print_ln("%s",a.reveal())
执行结果与for_range那个类似,这里就不放了。
(3)foreach_enumerate
Run-time loop over public data. This uses Player-Data/Public-Input/<progname>
.
@foreach_enumerate([2, 8, 3])
def _(i, j):
print_ln('%s: %s', i, j)
3. if条件语句
(1)if_
(condition)
Conditional execution without else block.
Parameters: | condition – regint/cint/int |
---|
注意,if后面还有个下划线。
x = sint(0)
@if_(x.reveal() == 0)
def _():
print_ln("%s",x.reveal())
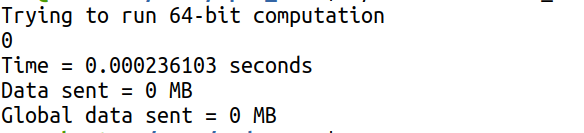
(2)if_e
(condition)
Conditional execution with else block.
Parameters: | condition – regint/cint/int |
---|
x = sint(10)
x = sint.get_random_int(4)
@if_e(x.reveal() > 7)
def _():
print_ln("x = %s > 7", x.reveal())
@else_
def _():
print_ln("x = %s < 7", x.reveal())
(3)if_then()
用法:
if_then(cond)
...
else_then()
...
end_if()
for i in range(5):
a = sint.get_random_int(10)
print_str("a = %s, ", a.reveal())
if_then(a.reveal() >= 512)
print_ln("a >= 512")
else_then()
print_ln("a < 512")
end_if()
(4) if_statement()
if_statement(condition, if_fn, else_fn=None)
def p1():
print_ln("hehe, a >= 512")
def p2():
print_ln("haha, a < 512")
for i in range(5):
a = sint.get_random_int(10)
print_str("a = %s, ", a.reveal())
if_statement(a.reveal() >= 512, p1, p2)
4.print语句
(1)print_str(), print_ln()
区别在于print_ln比print_str多了一个\n。一般用这两个的比较多。
(2)print_str_if(), print_ln_if()
只有满足条件才会触发print。
print_ln_if(cond, ss, *args)
print_str_if(cond, ss, *args)
n = 20
a = Array(n, sint)
t = [1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20]
a.assign(t)
print_ln("%s", a.reveal())
@for_range(n)
def _(i):
print_str("%s ", a[i].reveal())
print_ln()
@for_range(n)
def _(i):
print_str_if(i != 2, "%s ", a[i].reveal())
print_ln()
@for_range(n)
def _(i):
print_ln_if(i == 2, "%s", a[i].reveal())
(3)print_ln_to()
print_ln_to(player, ss, *args)
Print line at player
only. Note that printing is disabled by default except at player 0. Activate interactive mode with -I to enable it for all players.
Parameters: |
|
---|
print_ln_to(1, "%s", a[1].reveal())
运行代码什么也不显示,因为他打印给了player。我们看不到。
(4)print_float_precision(n)
设置浮点数的打印精度。注意这个函数不是打印什么,而是设置什么。
a = sfloat(5.655643)
print_ln("a = %s",a.reveal())
print_float_precision(2)
print_ln("a = %s",a.reveal())
5.排序
(1)sort()
sort(a): 普通排序,看不出来用的什么算法。只能升序排。
n = 20
a = Array(n, sint)
t = [2,15,21,33,41,100,101,200,12,90,34,122,234,23,177,32,3434,236,97,5]
a.assign(t)
print_ln("a = %s", a.reveal())
b = sort(a)
print_ln("b = %s", b.reveal())
6.
杂项
(1)cond_swap()
cond_swap(x,y):有条件的交换两个元素的值
当x >= y 时 交换两个元素的值。
@for_range(5)
def _(i):
a = sint.get_random_int(10)
b = sint.get_random_int(10)
print_str("a = %s, b = %s; After swap: ", a.reveal(), b.reveal())
a, b = cond_swap(a,b)
print_ln("a = %s, b = %s", a.reveal(), b.reveal())
(2)get_number_of_players()
获取参与方的总数
a = get_number_of_players()
print_ln("a = %s", a)
(3)get_player_id()
获取参与方的id
b = get_player_id()
print_ln("b = %s", b)
(4) public_input()
获得一个公共输入。
a = public_input()
print_ln("a = %s", a)
这个公共输入文件在Programs/Public-Input/文件夹,编译过后会在这个文件夹生成一个与你程序名一样的文件,这个文件就是存储公共输入的文件,你在里面写入数据,通过这个函数就可以读到程序里。
(5)runtime_error()
runtime_error(msg='', *args) Print an error message and abort the runtime. Parameters work as in :py:func:`print_ln`
中断程序,并打印错误信息。
这个程序是遍历数组,当某元素等于200时,中断程序并打印信息。
n = 20
a = Array(n, sint)
t = [2,15,21,33,41,100,101,200,12,90,34,122,234,23,177,32,3434,236,97,5]
a.assign(t)
print_ln("a = %s", a.reveal())
@for_range(n)
def _(i):
@if_(a[i].reveal()==200)
def _():
runtime_error("Stop!! a = %s", a[i].reveal())