public interface ILinearList<T>
{
int Length();
bool IsEmpty();
void Clear();
T GetItem(int index);
int LocateItem(T t);
void Insert(T item, int index);
void Add(T item);
void Delete(int index);
}
public class Node<T>
{
private T data;
private Node<T> next;
public Node(T val, Node<T> p)
{
data = val;
next = p;
}
public Node(Node<T> p)
{
next = p;
}
public Node(T val)
{
data = val;
next = null;
}
public Node()
{
data = default(T);
next = null;
}
public T Data
{
get { return data; }
set
{
data = value;
}
}
public Node<T> Next
{
get { return next; }
set { next = value; }
}
}
public class LinkList<T> : ILinearList<T>
{
private Node<T> head;
public LinkList(Node<T> val)
{
head = val;
}
public int Length()
{
Node<T> p = head;
int len = 0;
while (p != null)
{
len++;
p = p.Next;
}
return len;
}
public void Add(T item)
{
Node<T> q = new Node<T>(item);
Node<T> p = new Node<T>();
if (head == null)
{
head = q;
}
p = head;
while (p.Next != null)
{
p = p.Next;
}
p.Next = q;
}
public void Clear()
{
head = null;
}
public void Delete(int index)
{
if (IsEmpty() || index < 1)
{
throw new Exception("链表为空或删除位置不允许");
}
if (index == 1)
{
head = head.Next;
}
Node<T> p = head;
Node<T> q = new Node<T>();
int j = 1;
while (p.Next != null && j < index)
{
j++;
q = p;
p = p.Next;
}
if (j == index)
{
q.Next = p.Next;
}
else
{
throw new Exception("删除节点不存在");
}
}
public T GetItem(int index)
{
if (IsEmpty() || index < 1)
{
throw new Exception("链表为空或获取位置不允许");
}
Node<T> p = new Node<T>();
p = head;
int j = 1;
while (p.Next != null && j < index)
{
p = p.Next;
j++;
}
if (j == index)
{
return p.Data;
}
else
{
throw new Exception("要获取的节点不存在");
}
}
public void Insert(T item, int index)
{
if (IsEmpty() || index < 1)
{
throw new Exception("链表为空或插入位置不允许");
}
if (index == 1)
{
Node<T> newHead = new Node<T>(item);
newHead.Next = head;
head = newHead;
}
Node<T> p = head;
Node<T> r = new Node<T>();
int j = 1;
while (p.Next != null && j < index)
{
r = p;
p = p.Next;
j++;
}
if (j == index)
{
Node<T> q = new Node<T>(item);
q.Next = p;
r.Next = q;
}
}
public bool IsEmpty()
{
return head == null;
}
public int LocateItem(T t)
{
if (IsEmpty())
{
throw new Exception("链表为空");
}
Node<T> p = new Node<T>();
p = head;
int result = -1;
int j = 1;
do
{
if (p.Data.Equals(t))
{
result = j;
break;
}
p = p.Next;
j++;
} while (p.Next != null);
return result;
}
/// <summary>
/// 反转链表
/// </summary>
public Node<T> Inverse()
{
Node<T> r = null;
Node<T> q = null;
Node<T> p = head;
while (p != null)
{
r = q;
q = p;
p = p.Next;
q.Next = r;
}
return q;
}
}
数据结构-单链表
最新推荐文章于 2024-03-12 20:44:15 发布
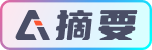