ExpandableListView的使用
1)、功能Activity:
public class Fourth extends Activity {
private String url = "http://www.jcpeixun.com/app_client_api/course_lesson_list.aspx?courseid=2168&uid=450894";
private ExpandableListView ex;
private TextView text_fourth;
private RequestQueue mRequestQueue;
private Handler mHandler;
private List<Item> course_name_list = new ArrayList<Item>();
private List<Item> chapter_list = new ArrayList<Item>();
private List<Item> name_list;
private List<ArrayList> list_item = new ArrayList<ArrayList>();
private
class Item {
String course_name;
String chapter;
String name;
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
this.setContentView(R.layout.fourth);
mRequestQueue = Volley.newRequestQueue(this);
init();
getData(url);
}
public void init() {
text_fourth = (TextView) findViewById(R.id.text_fourth);
ex = (ExpandableListView) findViewById(R.id.expandablelistview);
mHandler = new Handler() {
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
if (msg.what == 0x001) {
assign((JSONObject) msg.obj);
}
}
};
}
public void assign(JSONObject object) {
try {
Item item = new Item();
item.course_name = object.getString("course_name");
course_name_list.add(item);
TextPaint tp = text_fourth.getPaint();
tp.setFakeBoldText(true);
text_fourth.setText(course_name_list.get(0).course_name);
JSONArray outline = object.getJSONArray("outline");
for(int i=0;i<outline.length();i++){
JSONObject object1 = outline.getJSONObject(i);
Item item1 = new Item();
item1.chapter = object1.getString("chapter");
chapter_list.add(item1);
JSONArray array = object1.getJSONArray("lesson_list");
name_list = new ArrayList<Item>();
for(int j=0;j<array.length();j++){
JSONObject object2 = array.getJSONObject(j);
Item item2 = new Item();
item2.name = object2.getString("name");
name_list.add(item2);
Log.e("name_list "+j, "" + name_list.get(j).name);
}
list_item.add((ArrayList) name_list);
}
} catch (JSONException e) {
e.printStackTrace();
}
ExpandableAdapter mExpandableAdapter = new ExpandableAdapter();
ex.setAdapter(mExpandableAdapter);
}
public void getData(String url) {
JsonObjectRequest js = new JsonObjectRequest(Request.Method.GET, url, null,
new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject jsonObject) {
if (jsonObject.toString() != null) {
Message message = mHandler.obtainMessage(0x001);
message.obj = jsonObject;
mHandler.sendMessage(message);
}
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError volleyError) {
}
});
mRequestQueue.add(js);
}
public class ExpandableAdapter extends BaseExpandableListAdapter{
@Override
public int getGroupCount() {
return chapter_list.size();
}
@Override
public int getChildrenCount(int groupPosition) {
return list_item.get(groupPosition).size();
}
@Override
public Object getGroup(int groupPosition) {
return chapter_list.get(groupPosition);
}
@Override
public Object getChild(int groupPosition, int childPosition) {
return list_item.get(groupPosition).get(childPosition);
}
@Override
public long getGroupId(int groupPosition) {
return groupPosition;
}
@Override
public long getChildId(int groupPosition, int childPosition) {
return childPosition;
}
@Override
public boolean hasStableIds() {
return true;
}
@Override
public View getGroupView(int groupPosition, boolean isExpanded, View convertViewGroup, ViewGroup parent) {
convertViewGroup = getLayoutInflater().inflate(R.layout.fourth_groupview,null);
TextView text_groupView = (TextView) convertViewGroup.findViewById(R.id.text_groupView);
text_groupView.setText(chapter_list.get(groupPosition).chapter);
return convertViewGroup;
}
@Override
public View getChildView(int groupPosition, int childPosition, boolean isLastChild, View convertViewChild, ViewGroup parent) {
convertViewChild = getLayoutInflater().inflate(R.layout.fourth_childview, null);
TextView text_childView = (TextView) convertViewChild.findViewById(R.id.text_childView);
name_list = list_item.get(groupPosition);
text_childView.setText(name_list.get(childPosition).name);
return convertViewChild;
}
@Override
public boolean isChildSelectable(int groupPosition, int childPosition) {
return true;
}
}
}
2)、activity布局fourth.xml:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/text_fourth"
android:layout_width="match_parent"
android:gravity="center"
android:layout_marginTop="14dp"
android:textSize="22dp"
android:layout_height="44dp" />
<ExpandableListView
android:id="@+id/expandablelistview"
android:layout_width="match_parent"
android:layout_height="fill_parent"
android:layout_gravity="center_horizontal"
android:layout_marginTop="1dp"
android:background="#ffffffff"
android:isScrollContainer="false"/>
</LinearLayout>
3)、GroupView布局文件fourth_groupview.xml:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal" android:layout_width="match_parent"
android:layout_height="70dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textAppearance="?android:attr/textAppearanceMedium"
android:text="Medium Text"
android:id="@+id/text_groupView"
android:layout_gravity="center_vertical"
android:layout_marginLeft="35dp"
android:textSize="24dp"
android:textColor="#FF0000" />
</LinearLayout>
4)、ChildView布局fourth_childview.xml:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="37dp"
android:orientation="horizontal">
<TextView
android:id="@+id/text_childView"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical"
android:layout_weight="1"
android:text="Small Text"
android:textAppearance="?android:attr/textAppearanceSmall"
android:textColor="#666"
android:layout_marginLeft="14dp"
android:textSize="20dp" />
<Button
android:id="@+id/play_video"
style="?android:attr/buttonStyleSmall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginRight="5dp"
android:background="#ffffffff"
android:focusable="false"
android:text="播放"
android:textColor="#169dde" />
</LinearLayout>
5)、效果图:
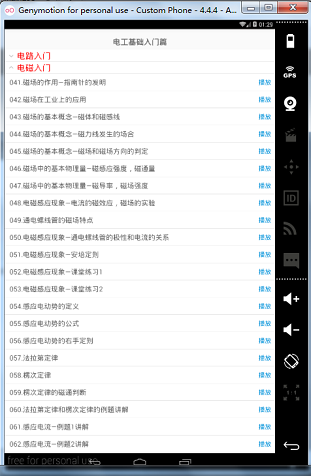