前言
- C# 中的 字典 Dictionary的用法,可以查看微软MSDN的在线文档
方法验证
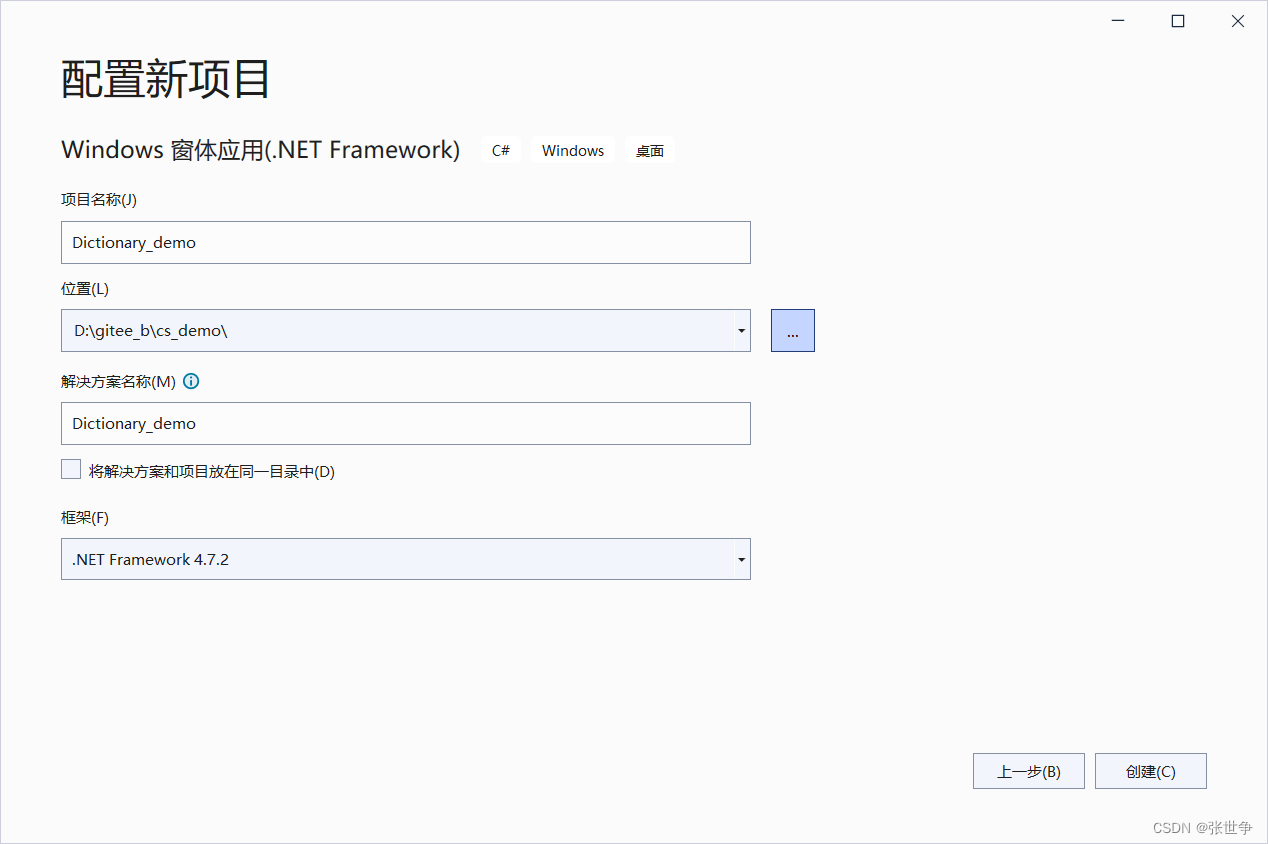
字典
- 字典 Dictionary 就是【键值对】的管理,可以添加多个【键值对】,用处还是比较的广泛的
- 如学号与姓名的键值对的管理
- 键(Key)必须是唯一的,值(Value)可以相同可以不相同
- 为了表达方便,尽量不使用空值(NULL)最为Key 或者 Value
测试代码
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace Dictionary_demo
{
public partial class Form1 : Form
{
Dictionary<string, string> dictDemo = new Dictionary<string, string>();
public Form1()
{
InitializeComponent();
}
private void add_dict_Click(object sender, EventArgs e)
{
this.textBoxInfo.AppendText("------------- Add item -------------\r\n");
if (dictDemo.ContainsKey(textKey.Text))
{
this.textBoxInfo.AppendText("Key : " + textKey.Text + " already exits!\r\n");
}
else
{
dictDemo.Add(textKey.Text, textValue.Text);
this.textBoxInfo.AppendText("Add Key : " + textKey.Text + " \r\n");
this.textBoxInfo.AppendText("Add Value : " + textValue.Text + " \r\n");
}
}
private void btnLogClean_Click(object sender, EventArgs e)
{
this.textBoxInfo.Clear();
}
private void btnFindFromKey_Click(object sender, EventArgs e)
{
this.textBoxInfo.AppendText("------------- find item with Key -------------\r\n");
textValue.Text = "";
if (dictDemo.ContainsKey(textKey.Text))
{
string value = "";
this.textBoxInfo.AppendText("Key : " + textKey.Text + " exits!\r\n");
if (dictDemo.TryGetValue(textKey.Text, out value))
{
textValue.Text = value;
this.textBoxInfo.AppendText("Find : " + "Key = " + textKey.Text + "Value = " + value + "\r\n");
}
else
{
this.textBoxInfo.AppendText("Key : " + textKey.Text + " find error!\r\n");
}
}
else
{
this.textBoxInfo.AppendText("Key : " + textKey.Text + " not exits!\r\n");
}
}
private void btnFindFromValue_Click(object sender, EventArgs e)
{
this.textBoxInfo.AppendText("------------- find item with value -------------\r\n");
foreach (KeyValuePair<string, string> kvp in dictDemo)
{
if (kvp.Value.Equals(textValue.Text))
{
this.textBoxInfo.AppendText("key = " + kvp.Key + " Value = " + kvp.Value + " \r\n");
}
}
this.textBoxInfo.AppendText("------------- print end -------------\r\n");
}
private void btnDictPrint_Click(object sender, EventArgs e)
{
this.textBoxInfo.AppendText("------------- print start -------------\r\n");
foreach (KeyValuePair<string, string> kvp in dictDemo)
{
this.textBoxInfo.AppendText("key = " + kvp.Key + " Value = " + kvp.Value + " \r\n");
}
this.textBoxInfo.AppendText("------------- print end -------------\r\n");
}
private void btnDictDelete_Click(object sender, EventArgs e)
{
this.textBoxInfo.AppendText("------------- remove item -------------\r\n");
dictDemo.Remove(textKey.Text);
this.textBoxInfo.AppendText("Remove itme : Key = " + textKey.Text + " \r\n");
}
}
}
测试方法
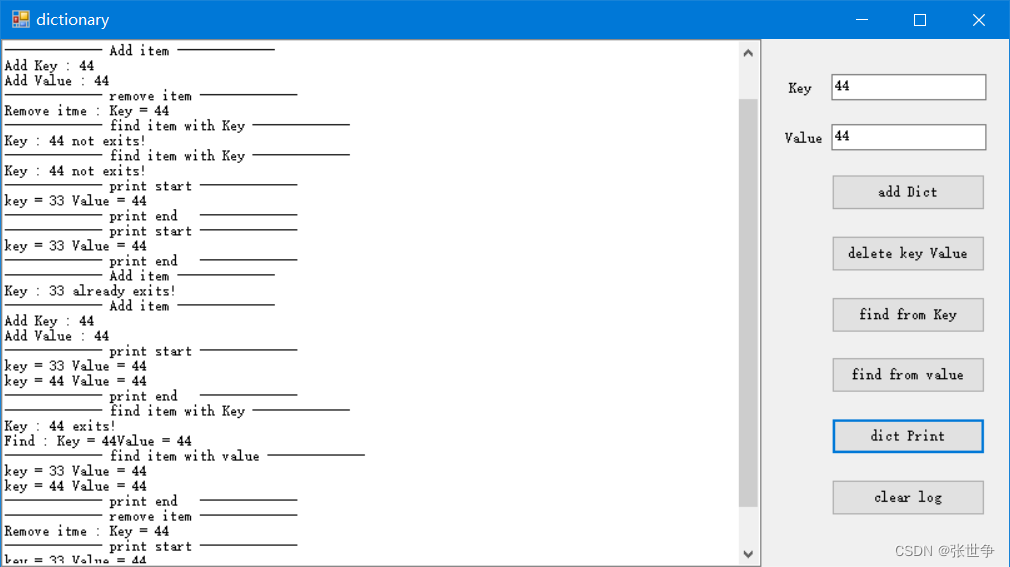
相关的API
初始化
Dictionary<string, string> dictDemo = new Dictionary<string, string>();
- 注意 Key 与 Value的类型,可以随便,不一定是string类型
添加
- 使用 Add 方法添加,如:
dictDemo.Add(textKey.Text, textValue.Text);
删除
- 使用 remove 方法,如
dictDemo.Remove(textKey.Text);
- 清空字典可以使用 clear方法
dictDemo.clear();
查找
- 按关键字查找,最好先判断关键字是否存在,如:
if (dictDemo.ContainsKey(textKey.Text))
- 如果key 存在,可以使用:TryGetValue 尝试获取值,或者直接通过索引:
string value = dictDemo[textKey.Text];
遍历
foreach (KeyValuePair<string, string> kvp in dictDemo)
{
if (kvp.Value.Equals(textValue.Text))
{
this.textBoxInfo.AppendText("key = " + kvp.Key + " Value = " + kvp.Value + " \r\n");
}
}
小结
- C# 的字典用的还是比较的简单,更多的操作可以详细查看微软官方的文档