package com.example.java_algorithms.javaBasic.img;
import org.apache.commons.io.FileUtils;
import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLConnection;
/**
* 下载图片:字节流写入,三种方法
*/
public class picture {
private static String filePath="https://img3.doubanio.com/view/photo/l/public/p2647478420.webp";
public static void main(String[] args) throws Exception {
// testDownload01();
// testDownload02();
testDownload03();
}
//下载-URLConnection
public static void testDownload01 () throws Exception {
URL url = new URL(filePath);//定义一个url对象
URLConnection urlConnection=url.openConnection();
InputStream inputStream=urlConnection.getInputStream();
OutputStream outputStream=new FileOutputStream("Copy04.jpg");
int temp=0;
//循环
while ((temp=inputStream.read())!=-1){
outputStream.write(temp);
}
outputStream.close();
inputStream.close();
}
//下载-HttpURLConnection
public static void testDownload02() throws Exception {
URL url = new URL(filePath);//定义一个url对象
HttpURLConnection conn = (HttpURLConnection) url.openConnection();//打开链接
conn.setRequestMethod("GET");//设置请求方式为get请求
conn.setConnectTimeout(10 * 100);//设置请求超时为10秒
InputStream is = conn.getInputStream();//通过数据流获取图片
byte[] data = readInputStream(is);//得到图片数据流二进制数据
File imageFile = new File("Copy03.jpg");//创建文件保存图片
FileOutputStream outputStream = new FileOutputStream(imageFile);//创建输出流
outputStream.write(data);//写入数据
outputStream.close();//关闭数据流
}
//用的是apache的包commons-io
public static void testDownload03() throws Exception {
System.out.println(filePath);
//拷贝一个网页文件到一个文件
try {
FileUtils.copyURLToFile(new URL(filePath),new File("test_02_1.jpg"));
} catch (IOException e) {
e.printStackTrace();
System.out.println("IO异常,downloader方法异常!");
}
}
public static byte[] readInputStream(InputStream inSream) throws Exception{
ByteArrayOutputStream outStream=new ByteArrayOutputStream();
byte[] buffer=new byte[6024];//创建一个buffer字符串
int len;
//使用一个输入流从buffer里把数据读取出来
while ((len =inSream.read(buffer)) != -1) {
//用输出流往buffer里写入数据,中间参数代表从哪个位置开始读,len代表读取的长度
outStream.write(buffer, 0, len);
}
//关闭输入流
inSream.close();
//把outStream里的数据写入内存
return outStream.toByteArray();
}
}
Java之图片下载
于 2022-05-27 23:43:14 首次发布
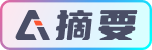