1 创建以下内容
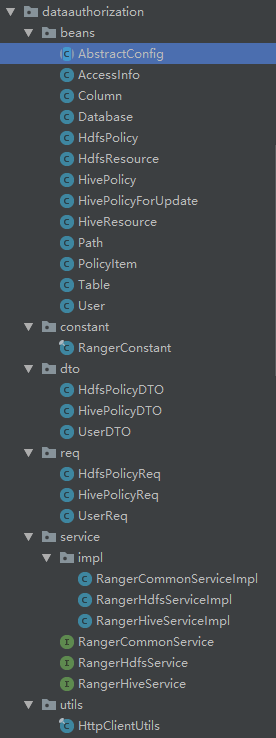
2 AbstractConfig
package com.test.datamanagement.dataauthorization.beans;
import org.codehaus.jackson.annotate.JsonProperty;
import java.io.Serializable;
import java.util.List;
/**
* @author tuzuoquan
* @version 1.0
* @ClassName AbstractConfig
* @description TODO
* @date 2020/4/21 9:57
**/
public abstract class AbstractConfig implements Serializable {
private static final long serialVersionUID = 6544357528018619278L;
private List<String> values;
private Boolean isRecursive;
private Boolean isExcludes;
protected AbstractConfig() {
}
public List<String> getValues() {
return values;
}
public void setValues(List<String> values) {
this.values = values;
}
@JsonProperty(value = "isRecursive")
public Boolean getIsRecursive() {
return isRecursive;
}
public void setRecursive(Boolean recursive) {
isRecursive = recursive;
}
@JsonProperty(value = "isExcludes")
public Boolean getIsExcludes() {
return isExcludes;
}
public void setExcludes(Boolean excludes) {
isExcludes = excludes;
}
}
3 AccessInfo
package com.test.datamanagement.dataauthorization.beans;
import org.codehaus.jackson.annotate.JsonProperty;
import java.io.Serializable;
/**
* @author tuzuoquan
* @version 1.0
* @ClassName AccessInfo
* @description TODO
* @date 2020/4/21 10:25
**/
public class AccessInfo implements Serializable {
private static final long serialVersionUID = -4167952102358620895L;
/**
* 权限类型
*/
private String type;
/**
* 是否允许
*/
private Boolean isAllowed;
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
@JsonProperty(value = "isAllowed")
public Boolean getIsAllowed() {
return isAllowed;
}
public void setAllowed(Boolean allowed) {
isAllowed = allowed;
}
}
4 Column
package com.test.datamanagement.dataauthorization.beans;
/**
* @author tuzuoquan
* @version 1.0
* @ClassName Column
* @description TODO
* @date 2020/4/21 9:47
**/
public class Column extends AbstractConfig {
private static final long serialVersionUID = 1094741866960414562L;
public Column() {
}
}
5 Database
package com.test.datamanagement.dataauthorization.beans;
/**
* @author tuzuoquan
* @version 1.0
* @ClassName Database
* @description TODO
* @date 2020/4/21 9:45
**/
public class Database extends AbstractConfig {
private static final long serialVersionUID = -2619315946039623847L;
public Database() {
}
}
6 HdfsPolicy
package com.test.datamanagement.dataauthorization.beans;
import org.codehaus.jackson.annotate.JsonProperty;
import java.io.Serializable;
import java.util.List;
/**
* @author tuzuoquan
* @version 1.0
* @ClassName HdfsPolicy
* @description TODO
* @date 2020/4/17 16:48
**/
public class HdfsPolicy implements Serializable {
private static final long serialVersionUID = -8946810855575126349L;
/**
* 策略类型
*/
private String policyType;
/**
* 策略名称
*/
private String name;
/**
* is_enabled
*/
private Boolean isEnabled;
/**
* policy_priority
*/
private Integer policyPriority;
private List<String> policyLabels;
private String description;
/**
* is_audit_enabled
*/
private Boolean isAuditEnabled;
private HdfsResource resources;
private List<PolicyItem> policyItems;
private List<PolicyItem> denyPolicyItems;
private List<PolicyItem> allowExceptions;
private List<PolicyItem> denyExceptions;
/**
* 所属服务(服务名称)
*/
private String service;
public HdfsPolicy() {
}
public String getPolicyType() {
return policyType;
}
public void setPolicyType(String policyType) {
this.policyType = policyType;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@JsonProperty(value = "isEnabled")
public Boolean getIsEnabled() {
return isEnabled;
}
public void setEnabled(Boolean enabled) {
isEnabled = enabled;
}
public Integer getPolicyPriority() {
return policyPriority;
}
public void setPolicyPriority(Integer policyPriority) {
this.policyPriority = policyPriority;
}
public List<String> getPolicyLabels() {
return policyLabels;
}
public void setPolicyLabels(List<String> policyLabels) {
this.policyLabels = policyLabels;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
@JsonProperty(value = "isAuditEnabled")
public Boolean getIsAuditEnabled() {
return isAuditEnabled;
}
public void setAuditEnabled(Boolean auditEnabled) {
isAuditEnabled = auditEnabled;
}
public HdfsResource getResources() {
return resources;
}
public void setResources(HdfsResource resources) {
this.resources = resources;
}
public List<PolicyItem> getPolicyItems() {
return policyItems;
}
public void setPolicyItems(List<PolicyItem> policyItems) {
this.policyItems = policyItems;
}
public List<PolicyItem> getDenyPolicyItems() {
return denyPolicyItems;
}
public void setDenyPolicyItems(List<PolicyItem> denyPolicyItems) {
this.denyPolicyItems = denyPolicyItems;
}
public List<PolicyItem> getAllowExceptions() {
return allowExceptions;
}
public void setAllowExceptions(List<PolicyItem> allowExceptions) {
this.allowExceptions = allowExceptions;
}
public List<PolicyItem> getDenyExceptions() {
return denyExceptions;
}
public void setDenyExceptions(List<PolicyItem> denyExceptions) {
this.denyExceptions = denyExceptions;
}
public String getService() {
return service;
}
public void setService(String service) {
this.service = service;
}
public static final class HdfsPolicyBuilder {
private String policyType;
private String name;
private Boolean isEnabled;
private Integer policyPriority;
private List<String> policyLabels;
private String description;
private Boolean isAuditEnabled;
private HdfsResource resources;
private List<PolicyItem> policyItems;
private List<PolicyItem> denyPolicyItems;
private List<PolicyItem> allowExceptions;
private List<PolicyItem> denyExceptions;
private String service;
private HdfsPolicyBuilder() {
}
public static HdfsPolicyBuilder builder() {
return new HdfsPolicyBuilder();
}
public HdfsPolicyBuilder withPolicyType(String policyType) {
this.policyType = policyType;
return this;
}
public HdfsPolicyBuilder withName(String name) {
this.name = name;
return this;
}
public HdfsPolicyBuilder withIsEnabled(Boolean isEnabled) {
this.isEnabled = isEnabled;
return this;
}
public HdfsPolicyBuilder withPolicyPriority(Integer policyPriority) {
this.policyPriority = policyPriority;
return this;
}
public HdfsPolicyBuilder withPolicyLabels(List<String> policyLabels) {
this.policyLabels = policyLabels;
return this;
}
public HdfsPolicyBuilder withDescription(String description) {
this.description = description;
return this;
}
public HdfsPolicyBuilder withIsAuditEnabled(Boolean isAuditEnabled) {
this.isAuditEnabled = isAuditEnabled;
return this;
}
public HdfsPolicyBuilder withResources(HdfsResource resources) {
this.resources = resources;
return this;
}
public HdfsPolicyBuilder withPolicyItems(List<PolicyItem> policyItems) {
this.policyItems = policyItems;
return this;
}
public HdfsPolicyBuilder withDenyPolicyItems(List<PolicyItem> denyPolicyItems) {
this.denyPolicyItems = denyPolicyItems;
return this;
}
public HdfsPolicyBuilder withAllowExceptions(List<PolicyItem> allowExceptions) {
this.allowExceptions = allowExceptions;
return this;
}
public HdfsPolicyBuilder withDenyExceptions(List<PolicyItem> denyExceptions) {
this.denyExceptions = denyExceptions;
return this;
}
public HdfsPolicyBuilder withService(String service) {
this.service = service;
return this;
}
public HdfsPolicy build() {
HdfsPolicy hdfsPolicy = new HdfsPolicy();
hdfsPolicy.setPolicyType(policyType);
hdfsPolicy.setName(name);
hdfsPolicy.setPolicyPriority(policyPriority);
hdfsPolicy.setPolicyLabels(policyLabels);
hdfsPolicy.setDescription(description);
hdfsPolicy.setResources(resources);
hdfsPolicy.setPolicyItems(policyItems);
hdfsPolicy.setDenyPolicyItems(denyPolicyItems);
hdfsPolicy.setAllowExceptions(allowExceptions);
hdfsPolicy.setDenyExceptions(denyExceptions);
hdfsPolicy.setService(service);
hdfsPolicy.isEnabled = this.isEnabled;
hdfsPolicy.isAuditEnabled = this.isAuditEnabled;
return hdfsPolicy;
}
}
}
7 HdfsResource
package com.test.datamanagement.dataauthorization.beans;
import java.io.Serializable;
/**
* @author tuzuoquan
* @version 1.0
* @ClassName HdfsResource
* @description TODO
* @date 2020/4/21 9:43
**/
public class HdfsResource implements Serializable {
private static final long serialVersionUID = 6518622425358356391L;
private Path path;
public Path getPath() {
return path;
}
public void setPath(Path path) {
this.path = path;
}
}
8 HivePolicy
package com.test.datamanagement.dataauthorization.beans;
import org.codehaus.jackson.annotate.JsonProperty;
import java.io.Serializable;
import java.util.List;
/**
* @author tuzuoquan
* @version 1.0
* @ClassName HivePolicy
* @description TODO
* @date 2020/4/17 16:48
**/
public class HivePolicy implements Serializable {
private static final long serialVersionUID = -5379925186824296473L;
/**
* 策略类型
*/
private String policyType;
/**
* 策略名称
*/
private String name;
/**
* is_enabled
*/
private Boolean isEnabled;
/**
* policy_priority
*/
private Integer policyPriority;
private List<String> policyLabels;
private String description;
/**
* is_audit_enabled
*/
private Boolean isAuditEnabled;
private HiveResource resources;
private List<PolicyItem> policyItems;
private List<PolicyItem> denyPolicyItems;
private List<PolicyItem> allowExceptions;
private List<PolicyItem> denyExceptions;
/**
* 所属服务(服务名称)
*/
private String service;
public HivePolicy() {
}
public HivePolicy(String policyType, String name,
Boolean isEnabled, Integer policyPriority,
String description, Boolean isAuditEnabled, String service) {
this.policyType = policyType;
this.name = name;
this.isEnabled = isEnabled;
this.policyPriority = policyPriority;
this.description = description;
this.isAuditEnabled = isAuditEnabled;
this.service = service;
}
public String getPolicyType() {
return policyType;
}
public void setPolicyType(String policyType) {
this.policyType = policyType;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@JsonProperty(value = "isEnabled")
public Boolean getIsEnabled() {
return isEnabled;
}
public void setEnabled(Boolean enabled) {
isEnabled = enabled;
}
public Integer getPolicyPriority() {
return policyPriority;
}
public void setPolicyPriority(Integer policyPriority) {
this.policyPriority = policyPriority;
}
public List<String> getPolicyLabels() {
return policyLabels;
}
public void setPolicyLabels(List<String> policyLabels) {
this.policyLabels = policyLabels;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
@JsonProperty(value = "isAuditEnabled")
public Boolean getIsAuditEnabled() {
return isAuditEnabled;
}
public void setAuditEnabled(Boolean auditEnabled) {
isAuditEnabled = auditEnabled;
}
public HiveResource getResources() {
return resources;
}
public void setResources(HiveResource resources) {
this.resources = resources;
}
public List<PolicyItem> getPolicyItems() {
return policyItems;
}
public void setPolicyItems(List<PolicyItem> policyItems) {
this.policyItems = policyItems;
}
public List<PolicyItem> getDenyPolicyItems() {
return denyPolicyItems;
}
public void setDenyPolicyItems(List<PolicyItem> denyPolicyItems) {
this.denyPolicyItems = denyPolicyItems;
}
public List<PolicyItem> getAllowExceptions() {
return allowExceptions;
}
public void setAllowExceptions(List<PolicyItem> allowExceptions) {
this.allowExceptions = allowExceptions;
}
public List<PolicyItem> getDenyExceptions() {
return denyExceptions;
}
public void setDenyExceptions(List<PolicyItem> denyExceptions) {
this.denyExceptions = denyExceptions;
}
public String getService() {
return service;
}
public void setService(String service) {
this.service = service;
}
}
9 HivePolicyForUpdate
package com.test.datamanagement.dataauthorization.beans;
/**
* @author tuzuoquan
* @version 1.0
* @ClassName HivePolicyForUpdate
* @description 更新策略的时候
* @date 2020/5/6 16:14
**/
public class HivePolicyForUpdate extends HivePolicy {
private static final long serialVersionUID = -313323408326714792L;
private Long id;
/** 策略guid **/
private String guid;
/** 创建者 **/
private String createdBy;
/** 更新者 **/
private String updatedBy;
/** 新的版本值 **/
private Long version;
/** 资源签名 **/
private String resourceSignature;
/** create_time */
private Long createTime;
/**
* update_time
*/
private Long updateTime;
public HivePolicyForUpdate() {
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getGuid() {
return guid;
}
public void setGuid(String guid) {
this.guid = guid;
}
public String getCreatedBy() {
return createdBy;
}
public void setCreatedBy(String createdBy) {
this.createdBy = createdBy;
}
public String getUpdatedBy() {
return updatedBy;
}
public void setUpdatedBy(String updatedBy) {
this.updatedBy = updatedBy;
}
public Long getVersion() {
return version;
}
public void setVersion(Long version) {
this.version = version;
}
public String getResourceSignature() {
return resourceSignature;
}
public void setResourceSignature(String resourceSignature) {
this.resourceSignature = resourceSignature;
}
public Long getCreateTime() {
return createTime;
}
public void setCreateTime(Long createTime) {
this.createTime = createTime;
}
public Long getUpdateTime() {
return updateTime;
}
public void setUpdateTime(Long updateTime) {
this.updateTime = updateTime;
}
}
10 HiveResource
package com.test.datamanagement.dataauthorization.beans;
import java.io.Serializable;
/**
* @author tuzuoquan
* @version 1.0
* @ClassName HiveResource
* @description TODO
* @date 2020/4/21 9:43
**/
public class HiveResource implements Serializable {
private static final long serialVersionUID = -1430242627355290592L;
/**
* 数据库
*/
private Database database;
/**
* 可访问的表
*/
private Table table;
/**
* 可访问的列
*/
private Column column;
public Database getDatabase() {
return database;
}
public void setDatabase(Database database) {
this.database = database;
}
public Table getTable() {
return table;
}
public void setTable(Table table) {
this.table = table;
}
public Column getColumn() {
return column;
}
public void setColumn(Column column) {
this.column = column;
}
}
11 Path
package com.test.datamanagement.dataauthorization.beans;
import org.codehaus.jackson.annotate.JsonProperty;
import java.io.Serializable;
import java.util.List;
/**
* @author tuzuoquan
* @version 1.0
* @ClassName Path
* @description TODO
* @date 2020/7/8 15:00
**/
public class Path implements Serializable {
private static final long serialVersionUID = -6778742922797146458L;
private Boolean isExcludes;
private Boolean isRecursive;
/** 路径列表,类似:["/cececece_20", "/user/cececece_20"] **/
private List<String> values;
@JsonProperty(value = "isExcludes")
public Boolean getIsExcludes() {
return isExcludes;
}
public void setExcludes(Boolean excludes) {
isExcludes = excludes;
}
@JsonProperty(value = "isRecursive")
public Boolean getIsRecursive() {
return isRecursive;
}
public void setRecursive(Boolean recursive) {
isRecursive = recursive;
}
public List<String> getValues() {
return values;
}
public void setValues(List<String> values) {
this.values = values;
}
}
12 PolicyItem
package com.test.datamanagement.dataauthorization.beans;
import java.io.Serializable;
import java.util.List;
/**
* @author tuzuoquan
* @version 1.0
* @ClassName PolicyItem
* @description TODO
* @date 2020/4/21 10:10
**/
public class PolicyItem implements Serializable {
private static final long serialVersionUID = 7698059281969319356L;
private List<String> groups;
private List<String> users;
private List<AccessInfo> accesses;
private Boolean delegateAdmin;
private List<Object> conditions;
public List<String> getGroups() {
return groups;
}
public void setGroups(List<String> groups) {
this.groups = groups;
}
public List<String> getUsers() {
return users;
}
public void setUsers(List<String> users) {
this.users = users;
}
public List<AccessInfo> getAccesses() {
return accesses;
}
public void setAccesses(List<AccessInfo> accesses) {
this.accesses = accesses;
}
public Boolean getDelegateAdmin() {
return delegateAdmin;
}
public void setDelegateAdmin(Boolean delegateAdmin) {
this.delegateAdmin = delegateAdmin;
}
public List<Object> getConditions() {
return conditions;
}
public void setConditions(List<Object> conditions) {
this.conditions = conditions;
}
}
13 Table
package com.test.datamanagement.dataauthorization.beans;
/**
* @author tuzuoquan
* @version 1.0
* @ClassName Table
* @description TODO
* @date 2020/4/21 9:45
**/
public class Table extends AbstractConfig {
private static final long serialVersionUID = -8352040569163490242L;
public Table() {
}
}
14 User
package com.test.datamanagement.dataauthorization.beans;
import java.io.Serializable;
import java.util.List;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
/**
* @author tuzuoquan
* @version 1.0
* @ClassName User
* @description TODO
* @date 2020/4/17 14:58
**/
public class User implements Serializable {
private static final long serialVersionUID = -4396396612633542455L;
/**
* 所属组,即当前命名空间对应的group,例如:["13"]
*/
private List<String> groupIdList;
/**
* 默认值为:1
*/
private Integer status;
/**
* 用户角色,例如:["ROLE_USER"],此处是固定的
*/
private List<String> userRoleList;
/**
* 用户名,例如:"toto_2"
*/
private String name;
/**
* 密码,例如:"toto_2_20200417"
*/
private String password;
/**
* 例如:toto_2
*/
private String firstName;
/**
* 例如:toto_2,调用的时候填写空串:""
*/
private String lastName;
/**
* 邮箱地址,此处填写""
*/
private String emailAddress;
public User() {
}
public User(List<String> groupIdList, Integer status, List<String> userRoleList, String name, String password,
String firstName, String lastName, String emailAddress) {
this.groupIdList = groupIdList;
this.status = status;
this.userRoleList = userRoleList;
this.name = name;
this.password = password;
this.firstName = firstName;
this.lastName = lastName;
this.emailAddress = emailAddress;
}
public List<String> getGroupIdList() {
return groupIdList;
}
public void setGroupIdList(List<String> groupIdList) {
this.groupIdList = groupIdList;
}
public Integer getStatus() {
return status;
}
public void setStatus(Integer status) {
this.status = status;
}
public List<String> getUserRoleList() {
return userRoleList;
}
public void setUserRoleList(List<String> userRoleList) {
this.userRoleList = userRoleList;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmailAddress() {
return emailAddress;
}
public void setEmailAddress(String emailAddress) {
this.emailAddress = emailAddress;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.MULTI_LINE_STYLE);
}
}
15 RangerConstant
package com.test.datamanagement.dataauthorization.constant;
/**
* @author tuzuoquan
* @version 1.0
* @ClassName RangerConstant
* @description TODO
* @date 2020/4/21 16:36
**/
public final class RangerConstant {
/** 登录地址 **/
public static final String LOGIN_URL = "/login";
/** 添加用户接口 **/
public static final String USER_ADD_URL = "/service/xusers/secure/users";
/** 删除用户的接口前缀 **/
public static final String USER_DELETE_URL_PREFIX = "/service/xusers/secure/users/id/";
/** 删