支持pc和移动端
支持进度条拖动
一、安装:
yarn add @liripeng/vue-audio-player 或 npm i -S @liripeng/vue-audio-player
二、全局引入
1.import AudioPlayer from '@liripeng/vue-audio-player'
2.Vue.use(AudioPlayer)
三、使用
<template>
<div class="aBOx">
<div class="audio-tex">
<span>录音的标题</span>
<span>这是一段录音的描述</span>
</div>
<audio-player :audio-list="audioList.map(elm => elm)" :before-play="handleBeforePlay"
theme-color="red" continuation="false" />
</div>
</template>
<script>
import { ref } from "vue";
export default {
setup() {
let currentAudioName = ref('')
let audioList = ref(['//downsc.chinaz.net/Files/DownLoad/sound1/201906/11670.mp3' ])
const handleBeforePlay = (next) => {
next()
}
return {
currentAudioName,
audioList,
handleBeforePlay
}
},
}
</script>
四、改造
思路:通过css进行隐藏不需要的功能,通过定位改变到想要的位置,新增功能通过定位进行遮罩
改造前
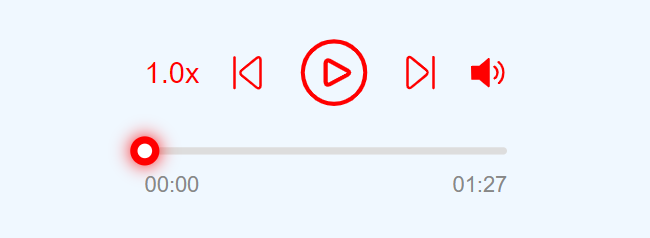
改造后
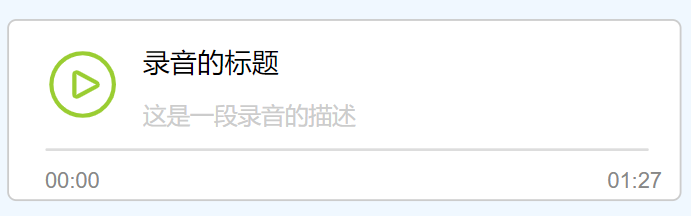
代码:
<template>
<div class="aBOx">
<div class="audio-tex">
<span>录音的标题</span>
<span>这是一段录音的描述</span>
</div>
<audio-player :audio-list="audioList.map(elm => elm)" :before-play="handleBeforePlay"
theme-color="rgb(255,255,255,.1)" continuation="false" />
</div>
</template>
<script>
import { ref } from "vue";
export default {
setup() {
let currentAudioName = ref('')
let audioList = ref(['//downsc.chinaz.net/Files/DownLoad/sound1/201906/11670.mp3'])
const handleBeforePlay = (next) => {
next()
}
return {
currentAudioName,
audioList,
handleBeforePlay
}
},
}
</script>
<style>
.aBOx {
width: 95%;
height: 100px;
background: white;
border: 1px solid #ccc;
border-radius: 5px;
position: relative;
}
.audio-tex {
height: 60%;
width: 80%;
color: black;
position: absolute;
left: 20%;
top: 8%;
display: flex;
flex-direction: column;
justify-content: space-around;
}
.audio-tex>span:nth-child(1) {
font-size: 15px;
color: black;
font-family: 'Franklin Gothic Medium', 'Arial Narrow', Arial, sans-serif;
}
.audio-tex>span:nth-child(2) {
font-size: 13px;
color: #ccc;
}
.audio-player {
height: 100% !important;
width: 100% !important;
display: flex;
flex-direction: column;
}
.audio__btn-wrap {
width: 90% !important;
height: 70% !important;
}
.audio__play-start {
position: absolute;
left: 0px;
top: 20%;
width: 15% !important;
height: 100% !important;
display: flex;
align-items: center;
justify-content: center;
color: yellowgreen !important;
}
.audio__play-pause {
position: absolute;
left: 0px;
top: 20%;
width: 15% !important;
height: 100% !important;
display: flex;
align-items: center;
justify-content: center;
color: yellowgreen !important;
}
.audio__play-rate {
display: none !important;
}
.audio__play-prev {
display: none !important;
}
.audio__play-next {
display: none !important;
}
.audio__play-volume-icon-wrap {
display: none !important;
}
.audio__progress-wrap {
width: 90% !important;
height: 10px !important;
margin-left: 20px;
height: 2px !important;
}
.audio__progress {
background: yellowgreen !important;
height: 2px;
}
.audio__progress-point {
display: none;
}
.audio__time-wrap {
width: 92% !important;
height: 20px !important;
margin-left: 20px;
}
</style>